Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Synthesis / TTSEngine / SAPIEngineTypes.cs / 1 / SAPIEngineTypes.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Xml; namespace System.Speech.Synthesis.TtsEngine { //******************************************************************* // // Public Enums // //******************************************************************* #region Public Enums [ComImport, Guid ("A74D7C8E-4CC5-4F2F-A6EB-804DEE18500E"), InterfaceType (ComInterfaceType.InterfaceIsIUnknown)] internal interface ITtsEngine { [PreserveSig] int Speak (SPEAKFLAGS dwSpeakFlags, ref Guid rguidFormatId, IntPtr pWaveFormatEx, IntPtr pTextFragList, IntPtr pOutputSite); [PreserveSig] int GetOutputFormat (ref Guid pTargetFmtId, IntPtr pTargetWaveFormatEx, out Guid pOutputFormatId, out IntPtr ppCoMemOutputWaveFormatEx); } [StructLayout (LayoutKind.Sequential)] internal class SPVTEXTFRAG { public IntPtr pNext; public SPVSTATE State; public IntPtr pTextStart; public int ulTextLen; public int ulTextSrcOffset; // must be the last element, it is passed to the TTS engine that // does not see thess fields public GCHandle gcText; public GCHandle gcNext; public GCHandle gcPhoneme; public GCHandle gcSayAsCategory; } internal enum SPVSKIPTYPE { SPVST_SENTENCE = 1, } // Disable warning CS0649 in this block #pragma warning disable 649 [ComConversionLossAttribute] [TypeLibTypeAttribute (16)] internal struct SPVSTATE { //--- Action public SPVACTIONS eAction; //--- Running state values public Int16 LangID; public Int16 wReserved; public int EmphAdj; public int RateAdj; public int Volume; public SPVPITCH PitchAdj; public int SilenceMSecs; public IntPtr pPhoneIds; public SPPARTOFSPEECH ePartOfSpeech; public SPVCONTEXT Context; } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal struct SPVCONTEXT { //[MarshalAs (UnmanagedType.LPWStr)] public IntPtr pCategory; //[MarshalAs (UnmanagedType.LPWStr)] public IntPtr pBefore; //[MarshalAs (UnmanagedType.LPWStr)] public IntPtr pAfter; } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal struct SPVPITCH { public int MiddleAdj; public int RangeAdj; } internal static class SAPIGuids { static internal readonly Guid SPDFID_WaveFormatEx = new Guid ("C31ADBAE-527F-4ff5-A230-F62BB61FF70C"); #if SPEECHSERVER static internal readonly Guid SPDFID_Text = new Guid ("7CEEF9F9-3D13-11d2-9EE7-00C04F797396"); #endif } #pragma warning restore 649 [Flags] internal enum SPEAKFLAGS : int { SPF_DEFAULT = 0x0000, // Synchronous, no purge, xml auto detect SPF_ASYNC = 0x0001, // Asynchronous call SPF_PURGEBEFORESPEAK = 0x0002, // Purge current data prior to speaking this SPF_IS_FILENAME = 0x0004, // The string passed to Speak() is a file name SPF_IS_XML = 0x0008, // The input text will be parsed for XML markup SPF_IS_NOT_XML = 0x0010, // The input text will not be parsed for XML markup SPF_PERSIST_XML = 0x0020, // Persists XML global state changes SPF_NLP_SPEAK_PUNC = 0x0040, // The normalization processor should speak the punctuation SPF_PARSE_SAPI = 0x0080, // Force XML parsing as MS SAPI SPF_PARSE_SSML = 0x0100 // Force XML parsing as W3C SSML } [Flags] internal enum SPVESACTIONS { SPVES_CONTINUE = 0, SPVES_ABORT = 1, SPVES_SKIP = 2, SPVES_RATE = 4, SPVES_VOLUME = 8 } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal enum SPVACTIONS { SPVA_Speak = 0, SPVA_Silence = 1, SPVA_Pronounce = 2, SPVA_Bookmark = 3, SPVA_SpellOut = 4, SPVA_Section = 5, SPVA_ParseUnknownTag = 6, } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal enum SPPARTOFSPEECH { //--- SAPI5 public POS category values (bits 28-31) SPPS_NotOverriden = -1, SPPS_Unknown = 0, SPPS_Noun = 0x1000, SPPS_Verb = 0x2000, SPPS_Modifier = 0x3000, SPPS_Function = 0x4000, SPPS_Interjection = 0x5000, SPPS_SuppressWord = 0xF000, // Special flag to indicate this word should not be recognized } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Xml; namespace System.Speech.Synthesis.TtsEngine { //******************************************************************* // // Public Enums // //******************************************************************* #region Public Enums [ComImport, Guid ("A74D7C8E-4CC5-4F2F-A6EB-804DEE18500E"), InterfaceType (ComInterfaceType.InterfaceIsIUnknown)] internal interface ITtsEngine { [PreserveSig] int Speak (SPEAKFLAGS dwSpeakFlags, ref Guid rguidFormatId, IntPtr pWaveFormatEx, IntPtr pTextFragList, IntPtr pOutputSite); [PreserveSig] int GetOutputFormat (ref Guid pTargetFmtId, IntPtr pTargetWaveFormatEx, out Guid pOutputFormatId, out IntPtr ppCoMemOutputWaveFormatEx); } [StructLayout (LayoutKind.Sequential)] internal class SPVTEXTFRAG { public IntPtr pNext; public SPVSTATE State; public IntPtr pTextStart; public int ulTextLen; public int ulTextSrcOffset; // must be the last element, it is passed to the TTS engine that // does not see thess fields public GCHandle gcText; public GCHandle gcNext; public GCHandle gcPhoneme; public GCHandle gcSayAsCategory; } internal enum SPVSKIPTYPE { SPVST_SENTENCE = 1, } // Disable warning CS0649 in this block #pragma warning disable 649 [ComConversionLossAttribute] [TypeLibTypeAttribute (16)] internal struct SPVSTATE { //--- Action public SPVACTIONS eAction; //--- Running state values public Int16 LangID; public Int16 wReserved; public int EmphAdj; public int RateAdj; public int Volume; public SPVPITCH PitchAdj; public int SilenceMSecs; public IntPtr pPhoneIds; public SPPARTOFSPEECH ePartOfSpeech; public SPVCONTEXT Context; } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal struct SPVCONTEXT { //[MarshalAs (UnmanagedType.LPWStr)] public IntPtr pCategory; //[MarshalAs (UnmanagedType.LPWStr)] public IntPtr pBefore; //[MarshalAs (UnmanagedType.LPWStr)] public IntPtr pAfter; } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal struct SPVPITCH { public int MiddleAdj; public int RangeAdj; } internal static class SAPIGuids { static internal readonly Guid SPDFID_WaveFormatEx = new Guid ("C31ADBAE-527F-4ff5-A230-F62BB61FF70C"); #if SPEECHSERVER static internal readonly Guid SPDFID_Text = new Guid ("7CEEF9F9-3D13-11d2-9EE7-00C04F797396"); #endif } #pragma warning restore 649 [Flags] internal enum SPEAKFLAGS : int { SPF_DEFAULT = 0x0000, // Synchronous, no purge, xml auto detect SPF_ASYNC = 0x0001, // Asynchronous call SPF_PURGEBEFORESPEAK = 0x0002, // Purge current data prior to speaking this SPF_IS_FILENAME = 0x0004, // The string passed to Speak() is a file name SPF_IS_XML = 0x0008, // The input text will be parsed for XML markup SPF_IS_NOT_XML = 0x0010, // The input text will not be parsed for XML markup SPF_PERSIST_XML = 0x0020, // Persists XML global state changes SPF_NLP_SPEAK_PUNC = 0x0040, // The normalization processor should speak the punctuation SPF_PARSE_SAPI = 0x0080, // Force XML parsing as MS SAPI SPF_PARSE_SSML = 0x0100 // Force XML parsing as W3C SSML } [Flags] internal enum SPVESACTIONS { SPVES_CONTINUE = 0, SPVES_ABORT = 1, SPVES_SKIP = 2, SPVES_RATE = 4, SPVES_VOLUME = 8 } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal enum SPVACTIONS { SPVA_Speak = 0, SPVA_Silence = 1, SPVA_Pronounce = 2, SPVA_Bookmark = 3, SPVA_SpellOut = 4, SPVA_Section = 5, SPVA_ParseUnknownTag = 6, } [System.Runtime.InteropServices.TypeLibTypeAttribute (16)] internal enum SPPARTOFSPEECH { //--- SAPI5 public POS category values (bits 28-31) SPPS_NotOverriden = -1, SPPS_Unknown = 0, SPPS_Noun = 0x1000, SPPS_Verb = 0x2000, SPPS_Modifier = 0x3000, SPPS_Function = 0x4000, SPPS_Interjection = 0x5000, SPPS_SuppressWord = 0xF000, // Special flag to indicate this word should not be recognized } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
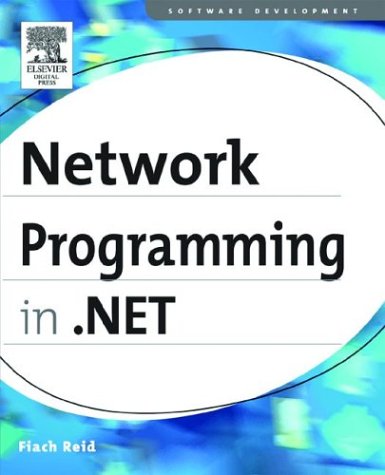
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateControlCodeDomTreeGenerator.cs
- TypeConverter.cs
- BackgroundFormatInfo.cs
- XslVisitor.cs
- TemplateXamlParser.cs
- CasesDictionary.cs
- PropertyConverter.cs
- StateItem.cs
- DataServiceKeyAttribute.cs
- ScrollChrome.cs
- SmiConnection.cs
- BrowserCapabilitiesCodeGenerator.cs
- Helper.cs
- ConfigurationLoaderException.cs
- unsafeIndexingFilterStream.cs
- XmlUtf8RawTextWriter.cs
- _MultipleConnectAsync.cs
- Utils.cs
- EncryptedPackage.cs
- PropertyGridCommands.cs
- TrimSurroundingWhitespaceAttribute.cs
- PolyLineSegment.cs
- TcpTransportSecurityElement.cs
- ServiceOperationParameter.cs
- ISAPIApplicationHost.cs
- NavigatorInvalidBodyAccessException.cs
- StyleBamlTreeBuilder.cs
- Switch.cs
- DataView.cs
- Column.cs
- SqlDataSourceCache.cs
- UserPreferenceChangingEventArgs.cs
- SHA512Managed.cs
- ModifierKeysValueSerializer.cs
- HttpHandlerAction.cs
- XmlTextWriter.cs
- MessageDescription.cs
- TreeViewCancelEvent.cs
- WebPartsPersonalization.cs
- UserControl.cs
- InfocardExtendedInformationCollection.cs
- _Connection.cs
- querybuilder.cs
- SQLGuid.cs
- CustomActivityDesigner.cs
- WebZone.cs
- ConfigXmlWhitespace.cs
- IntPtr.cs
- DataBoundControlHelper.cs
- PositiveTimeSpanValidator.cs
- WindowsFont.cs
- ReceiveMessageContent.cs
- ConfigurationValidatorBase.cs
- NullReferenceException.cs
- Highlights.cs
- FontInfo.cs
- RangeValuePatternIdentifiers.cs
- X509CertificateRecipientServiceCredential.cs
- SqlGenerator.cs
- XmlLanguage.cs
- PropertyGeneratedEventArgs.cs
- HtmlEmptyTagControlBuilder.cs
- DataGridViewCellStyleConverter.cs
- MediaSystem.cs
- XMLUtil.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- Validator.cs
- ServiceModelExtensionElement.cs
- EasingFunctionBase.cs
- FormatterConverter.cs
- DbException.cs
- ReversePositionQuery.cs
- CoreChannel.cs
- NumericUpDownAccelerationCollection.cs
- ConfigXmlText.cs
- IndependentAnimationStorage.cs
- ButtonAutomationPeer.cs
- Identity.cs
- DbConnectionPool.cs
- ConfigurationConverterBase.cs
- ComNativeDescriptor.cs
- ValidationRuleCollection.cs
- ProfileSettingsCollection.cs
- NativeMethods.cs
- XmlArrayItemAttributes.cs
- DataTableNewRowEvent.cs
- FormViewDeleteEventArgs.cs
- Stream.cs
- HttpValueCollection.cs
- DataReaderContainer.cs
- MailWriter.cs
- HtmlMeta.cs
- RequiredFieldValidator.cs
- ErrorHandler.cs
- FontUnitConverter.cs
- NullableDoubleMinMaxAggregationOperator.cs
- FileResponseElement.cs
- SmiEventSink_Default.cs
- cookieexception.cs
- KeyGestureConverter.cs