Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / PropertyGeneratedEventArgs.cs / 1305376 / PropertyGeneratedEventArgs.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Metadata.Edm; namespace System.Data.Services.Design { ////// This class encapsulates the EventArgs dispatched as part of the event /// raised when a property is generated. /// public sealed class PropertyGeneratedEventArgs : EventArgs { #region Private Data private MetadataItem _propertySource; private string _backingFieldName; private CodeTypeReference _returnType; private List_additionalGetStatements = new List (); private List _additionalSetStatements = new List (); private List _additionalSetStatements2 = new List (); private List _additionalAttributes = new List (); #endregion #region Constructors /// /// Default constructor /// public PropertyGeneratedEventArgs() { } ////// Constructor /// /// The event source /// The name of the field corresponding to the property /// The property return type public PropertyGeneratedEventArgs(MetadataItem propertySource, string backingFieldName, CodeTypeReference returnType) { this._propertySource = propertySource; this._backingFieldName = backingFieldName; this._returnType = returnType; } #endregion #region Properties ////// The Metadata object that is the source of the property /// public MetadataItem PropertySource { get { return this._propertySource; } } ////// The name of the field that backs the property; can be null in the case of /// navigation property /// public string BackingFieldName { get { return this._backingFieldName; } } ////// The type of the property by default; if changed by the user, the new value /// will be used by the code generator /// public CodeTypeReference ReturnType { get { return this._returnType; } set { this._returnType = value; } } ////// Statements to be included in the property's getter /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1002", Justification = "Same as System.Data.Entity.Design")] public ListAdditionalGetStatements { get { return this._additionalGetStatements; } } /// /// Statements to be included in the property's setter /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1002", Justification = "Same as System.Data.Entity.Design")] public ListAdditionalSetStatements { get { return _additionalSetStatements; } } /// /// Statements to be included in the property's setter /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1002", Justification = "Same as System.Data.Entity.Design")] internal ListAdditionalAfterSetStatements { get { return _additionalSetStatements2; } } /// /// Attributes to be added to the property's CustomAttributes collection /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1002", Justification = "Same as System.Data.Entity.Design")] public ListAdditionalAttributes { get { return this._additionalAttributes; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
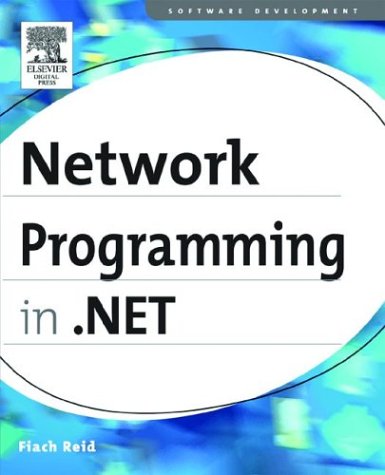
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Emitter.cs
- SafeNativeMethods.cs
- RemotingAttributes.cs
- HttpModuleActionCollection.cs
- HtmlInputHidden.cs
- mactripleDES.cs
- ResourceKey.cs
- ChameleonKey.cs
- SafeEventHandle.cs
- CopyNamespacesAction.cs
- PropertyMappingExceptionEventArgs.cs
- ContainerFilterService.cs
- RadioButtonRenderer.cs
- AnimationStorage.cs
- ProfileServiceManager.cs
- Stacktrace.cs
- initElementDictionary.cs
- TextServicesCompartmentEventSink.cs
- HtmlAnchor.cs
- ReferenceSchema.cs
- ItemsPresenter.cs
- EventlogProvider.cs
- ComNativeDescriptor.cs
- TreeNodeStyleCollection.cs
- DataBinding.cs
- RuntimeHandles.cs
- DrawingCollection.cs
- IisTraceWebEventProvider.cs
- CheckBoxField.cs
- ScriptDescriptor.cs
- SafeRightsManagementPubHandle.cs
- ItemsControl.cs
- Soap.cs
- MenuScrollingVisibilityConverter.cs
- ValidationEventArgs.cs
- TreePrinter.cs
- WindowsListViewItem.cs
- WebPartUtil.cs
- ViewLoader.cs
- ToolStripItemClickedEventArgs.cs
- CustomValidator.cs
- WebPartVerbsEventArgs.cs
- UnionCodeGroup.cs
- SupportsPreviewControlAttribute.cs
- SortedList.cs
- ParallelActivityDesigner.cs
- SendKeys.cs
- UTF8Encoding.cs
- ListViewItemSelectionChangedEvent.cs
- WindowsFormsSynchronizationContext.cs
- ObjectViewQueryResultData.cs
- DatatypeImplementation.cs
- MissingSatelliteAssemblyException.cs
- RegisteredArrayDeclaration.cs
- SharedStatics.cs
- AbandonedMutexException.cs
- ScrollableControl.cs
- SessionStateItemCollection.cs
- FreezableOperations.cs
- ValueTypeFixupInfo.cs
- XmlCharType.cs
- ReadContentAsBinaryHelper.cs
- Error.cs
- OletxResourceManager.cs
- SessionStateSection.cs
- LayoutSettings.cs
- PeerCollaborationPermission.cs
- TrackingWorkflowEventArgs.cs
- HMACMD5.cs
- DisplayInformation.cs
- ToolBar.cs
- Double.cs
- ExpressionVisitor.cs
- Stack.cs
- IntegrationExceptionEventArgs.cs
- securitymgrsite.cs
- WindowShowOrOpenTracker.cs
- SequenceRangeCollection.cs
- ProfileModule.cs
- Brush.cs
- QueryReaderSettings.cs
- SelectingProviderEventArgs.cs
- MDIWindowDialog.cs
- DataTrigger.cs
- TailPinnedEventArgs.cs
- XsltArgumentList.cs
- XmlEntityReference.cs
- LiteralDesigner.cs
- SecurityState.cs
- Point3DCollection.cs
- DiscreteKeyFrames.cs
- FormsAuthenticationEventArgs.cs
- ImageListUtils.cs
- PrintingPermissionAttribute.cs
- TypeResolver.cs
- documentation.cs
- ExcCanonicalXml.cs
- PlatformCulture.cs
- OleDbConnectionFactory.cs
- XmlSchemaValidationException.cs