Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / XPath / Internal / followingquery.cs / 1 / followingquery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; internal sealed class FollowingQuery : BaseAxisQuery { private XPathNavigator input; private XPathNodeIterator iterator; public FollowingQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest) : base(qyInput, name, prefix, typeTest) {} private FollowingQuery(FollowingQuery other) : base(other) { this.input = Clone(other.input); this.iterator = Clone(other.iterator); } public override void Reset() { iterator = null; base.Reset(); } public override XPathNavigator Advance() { if (iterator == null) { input = qyInput.Advance(); if (input == null) { return null; } XPathNavigator prev; do { prev = input.Clone(); input = qyInput.Advance(); } while (prev.IsDescendant(input)); input = prev; iterator = XPathEmptyIterator.Instance; } while (! iterator.MoveNext()) { bool matchSelf; if (input.NodeType == XPathNodeType.Attribute || input.NodeType == XPathNodeType.Namespace) { input.MoveToParent(); matchSelf = false; } else { while (! input.MoveToNext()) { if (! input.MoveToParent()) { return null; } } matchSelf = true; } if (NameTest) { iterator = input.SelectDescendants(Name, Namespace, matchSelf); } else { iterator = input.SelectDescendants(TypeTest, matchSelf); } } position++; currentNode = iterator.Current; return currentNode; } public override XPathNodeIterator Clone() { return new FollowingQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
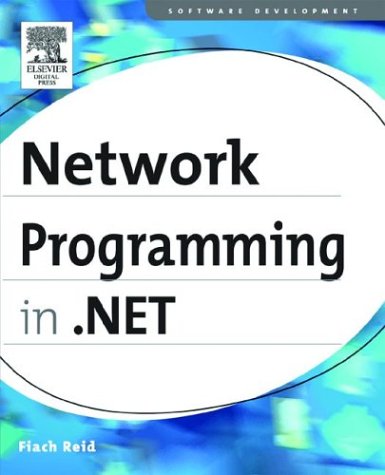
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventSourceCreationData.cs
- DataGridPagerStyle.cs
- TimeZoneInfo.cs
- IBuiltInEvidence.cs
- BitStack.cs
- AddInActivator.cs
- securitymgrsite.cs
- HtmlTitle.cs
- ObjectIDGenerator.cs
- ObjectStateEntry.cs
- AddInToken.cs
- CompilationUtil.cs
- Int32.cs
- CodeTypeMember.cs
- EventLogPermission.cs
- RenderData.cs
- Win32PrintDialog.cs
- CannotUnloadAppDomainException.cs
- EventArgs.cs
- _ConnectStream.cs
- WebZone.cs
- WebPartHelpVerb.cs
- TaskSchedulerException.cs
- DataTableNewRowEvent.cs
- XmlWriterTraceListener.cs
- X509PeerCertificateElement.cs
- ReaderWriterLock.cs
- CodeRemoveEventStatement.cs
- Attributes.cs
- SmiEventSink_Default.cs
- RunWorkerCompletedEventArgs.cs
- NgenServicingAttributes.cs
- VirtualDirectoryMappingCollection.cs
- ClientUtils.cs
- IpcManager.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- WebPartUserCapability.cs
- Switch.cs
- WsrmMessageInfo.cs
- VectorCollectionValueSerializer.cs
- SecurityManager.cs
- LinqDataSourceValidationException.cs
- Duration.cs
- StrongNameIdentityPermission.cs
- TableRowCollection.cs
- SqlHelper.cs
- XmlCharCheckingWriter.cs
- QilVisitor.cs
- ReflectPropertyDescriptor.cs
- Menu.cs
- LicenseManager.cs
- WebServiceData.cs
- DataGridViewAccessibleObject.cs
- TextureBrush.cs
- CachedPathData.cs
- OleDbException.cs
- HyperLink.cs
- PlatformCulture.cs
- NamespaceInfo.cs
- DataSourceCache.cs
- RoutingService.cs
- IntAverageAggregationOperator.cs
- RoleBoolean.cs
- ResXBuildProvider.cs
- WindowsStatusBar.cs
- ItemContainerGenerator.cs
- ResourceReferenceExpression.cs
- IncrementalHitTester.cs
- BoundColumn.cs
- IssuanceLicense.cs
- VisualCollection.cs
- SqlCommandSet.cs
- ImpersonateTokenRef.cs
- ParagraphResult.cs
- UserNamePasswordServiceCredential.cs
- QilReplaceVisitor.cs
- HtmlAnchor.cs
- TextParagraphView.cs
- WindowCollection.cs
- WindowsListView.cs
- Adorner.cs
- SystemIPv4InterfaceProperties.cs
- XmlHierarchicalDataSourceView.cs
- loginstatus.cs
- DataControlButton.cs
- SpeechSynthesizer.cs
- Byte.cs
- ManagedFilter.cs
- RowBinding.cs
- MobilePage.cs
- LineGeometry.cs
- SqlDependency.cs
- TagElement.cs
- UIElementIsland.cs
- DeleteMemberBinder.cs
- XmlMapping.cs
- HtmlGenericControl.cs
- PrintPreviewDialog.cs
- LambdaCompiler.Address.cs
- RadioButtonFlatAdapter.cs