Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / QilReplaceVisitor.cs / 1 / QilReplaceVisitor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Xml.Xsl; namespace System.Xml.Xsl.Qil { ////// Base internal class for visitors that replace the graph as they visit it. /// internal abstract class QilReplaceVisitor : QilVisitor { protected QilFactory f; public QilReplaceVisitor(QilFactory f) { this.f = f; } //----------------------------------------------- // QilVisitor overrides //----------------------------------------------- ////// Visit all children of "parent", replacing each child with a copy of each child. /// protected override QilNode VisitChildren(QilNode parent) { XmlQueryType oldParentType = parent.XmlType; bool recalcType = false; // Visit children for (int i = 0; i < parent.Count; i++) { QilNode oldChild = parent[i], newChild; XmlQueryType oldChildType = oldChild != null ? oldChild.XmlType : null; // Visit child if (IsReference(parent, i)) newChild = VisitReference(oldChild); else newChild = Visit(oldChild); // Only replace child and recalculate type if oldChild != newChild or oldChild.XmlType != newChild.XmlType if (!Ref.Equals(oldChild, newChild) || (newChild != null && !Ref.Equals(oldChildType, newChild.XmlType))) { recalcType = true; parent[i] = newChild; } } if (recalcType) RecalculateType(parent, oldParentType); return parent; } //----------------------------------------------- // QilReplaceVisitor methods //----------------------------------------------- ////// Once children have been replaced, the Xml type is recalculated. /// protected virtual void RecalculateType(QilNode node, XmlQueryType oldType) { XmlQueryType newType; newType = f.TypeChecker.Check(node); // Note the use of AtMost to account for cases when folding of Error nodes in the graph cause // cardinality to be recalculated. // For example, (Sequence (TextCtor (Error "error")) (Int32 1)) => (Sequence (Error "error") (Int32 1)) // In this case, cardinality has gone from More to One Debug.Assert(newType.IsSubtypeOf(XmlQueryTypeFactory.AtMost(oldType, oldType.Cardinality)), "Replace shouldn't relax original type"); node.XmlType = newType; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Xml.Xsl; namespace System.Xml.Xsl.Qil { ////// Base internal class for visitors that replace the graph as they visit it. /// internal abstract class QilReplaceVisitor : QilVisitor { protected QilFactory f; public QilReplaceVisitor(QilFactory f) { this.f = f; } //----------------------------------------------- // QilVisitor overrides //----------------------------------------------- ////// Visit all children of "parent", replacing each child with a copy of each child. /// protected override QilNode VisitChildren(QilNode parent) { XmlQueryType oldParentType = parent.XmlType; bool recalcType = false; // Visit children for (int i = 0; i < parent.Count; i++) { QilNode oldChild = parent[i], newChild; XmlQueryType oldChildType = oldChild != null ? oldChild.XmlType : null; // Visit child if (IsReference(parent, i)) newChild = VisitReference(oldChild); else newChild = Visit(oldChild); // Only replace child and recalculate type if oldChild != newChild or oldChild.XmlType != newChild.XmlType if (!Ref.Equals(oldChild, newChild) || (newChild != null && !Ref.Equals(oldChildType, newChild.XmlType))) { recalcType = true; parent[i] = newChild; } } if (recalcType) RecalculateType(parent, oldParentType); return parent; } //----------------------------------------------- // QilReplaceVisitor methods //----------------------------------------------- ////// Once children have been replaced, the Xml type is recalculated. /// protected virtual void RecalculateType(QilNode node, XmlQueryType oldType) { XmlQueryType newType; newType = f.TypeChecker.Check(node); // Note the use of AtMost to account for cases when folding of Error nodes in the graph cause // cardinality to be recalculated. // For example, (Sequence (TextCtor (Error "error")) (Int32 1)) => (Sequence (Error "error") (Int32 1)) // In this case, cardinality has gone from More to One Debug.Assert(newType.IsSubtypeOf(XmlQueryTypeFactory.AtMost(oldType, oldType.Cardinality)), "Replace shouldn't relax original type"); node.XmlType = newType; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
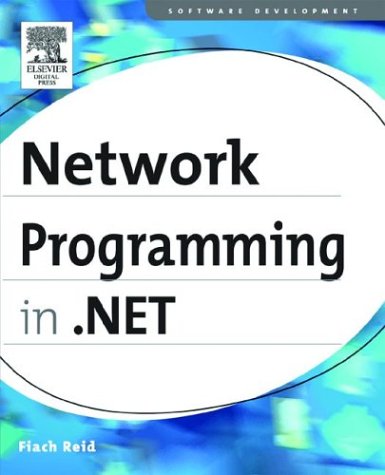
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CatalogPart.cs
- WebPartMenuStyle.cs
- UpdateRecord.cs
- AudioSignalProblemOccurredEventArgs.cs
- ResXFileRef.cs
- TextServicesCompartment.cs
- DataRowView.cs
- XomlCompilerHelpers.cs
- Roles.cs
- DataBindingHandlerAttribute.cs
- WmfPlaceableFileHeader.cs
- WebPartAddingEventArgs.cs
- CustomCategoryAttribute.cs
- Parallel.cs
- DiscreteKeyFrames.cs
- ServiceDeploymentInfo.cs
- MemberPath.cs
- EnumerableCollectionView.cs
- RegionInfo.cs
- XmlTextWriter.cs
- Version.cs
- BrushValueSerializer.cs
- WhiteSpaceTrimStringConverter.cs
- StylusPlugInCollection.cs
- PageMediaSize.cs
- RoutedEvent.cs
- ModuleBuilder.cs
- MetadataSerializer.cs
- TypeNameConverter.cs
- PowerModeChangedEventArgs.cs
- Activator.cs
- PreviewPageInfo.cs
- HtmlInputReset.cs
- RemoteTokenFactory.cs
- RoamingStoreFile.cs
- Opcode.cs
- DisplayInformation.cs
- StoreContentChangedEventArgs.cs
- SendAgentStatusRequest.cs
- Rect.cs
- SystemResourceKey.cs
- SplitterEvent.cs
- StringBlob.cs
- AuthenticationSection.cs
- MemberAssignment.cs
- ElementHostPropertyMap.cs
- Path.cs
- ChangeBlockUndoRecord.cs
- RawKeyboardInputReport.cs
- ColorAnimation.cs
- XPathDescendantIterator.cs
- PropertySourceInfo.cs
- BuildProviderCollection.cs
- SelectionEditingBehavior.cs
- LZCodec.cs
- KeyProperty.cs
- ImageMapEventArgs.cs
- IPHostEntry.cs
- ConfigurationSectionGroupCollection.cs
- DocumentOrderQuery.cs
- Propagator.JoinPropagator.cs
- Bitmap.cs
- SettingsSection.cs
- PasswordRecoveryDesigner.cs
- SqlTypeSystemProvider.cs
- IdentifierService.cs
- DataGridViewCellPaintingEventArgs.cs
- ImageSource.cs
- WindowsScrollBar.cs
- SettingsSection.cs
- ScrollViewer.cs
- EndPoint.cs
- ImageBrush.cs
- HTMLTagNameToTypeMapper.cs
- ToolstripProfessionalRenderer.cs
- PictureBox.cs
- CatalogZone.cs
- PersonalizationStateQuery.cs
- SafePointer.cs
- DiscardableAttribute.cs
- NetworkInterface.cs
- InputLanguageEventArgs.cs
- DNS.cs
- CustomMenuItemCollection.cs
- XmlLinkedNode.cs
- ColorAnimationUsingKeyFrames.cs
- XmlSiteMapProvider.cs
- ProxyFragment.cs
- MobileControlsSection.cs
- fixedPageContentExtractor.cs
- Table.cs
- SqlDataReaderSmi.cs
- TypedRowGenerator.cs
- CriticalFinalizerObject.cs
- TransactionProxy.cs
- CounterCreationData.cs
- loginstatus.cs
- DBSchemaTable.cs
- SamlAuthenticationStatement.cs
- HttpStreamMessageEncoderFactory.cs