Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / TableRowCollection.cs / 1305376 / TableRowCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; ////// [ Editor("System.Web.UI.Design.WebControls.TableRowsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public sealed class TableRowCollection : IList { ///Encapsulates the collection of ///objects within a control. /// A protected field of type private Table owner; ///. Represents the collection internally. /// /// internal TableRowCollection(Table owner) { this.owner = owner; } ////// Gets the /// count of public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///in the collection. /// /// public TableRow this[int index] { get { return(TableRow)owner.Controls[index]; } } ////// Gets a ///referenced by the /// specified ordinal index value. /// /// public int Add(TableRow row) { AddAt(-1, row); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableRow row) { owner.Controls.AddAt(index, row); if (row.TableSection != TableRowSection.TableBody) { owner.HasRowSections = true; } } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableRow[] rows) { if (rows == null) { throw new ArgumentNullException("rows"); } foreach(TableRow row in rows) { Add(row); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); owner.HasRowSections = false; } } ///Removes all ///controls from the collection. /// public int GetRowIndex(TableRow row) { if (owner.HasControls()) { return owner.Controls.IndexOf(row); } return -1; } ///Returns an ordinal index value that denotes the position of the specified /// ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableRow row) { owner.Controls.Remove(row); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ///Removes the ///from the collection at the specified /// index location. object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableRow)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableRow) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableRow)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableRow)o); } /// void IList.Insert(int index, object o) { AddAt(index, (TableRow)o); } /// void IList.Remove(object o) { Remove((TableRow)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; ////// [ Editor("System.Web.UI.Design.WebControls.TableRowsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] public sealed class TableRowCollection : IList { ///Encapsulates the collection of ///objects within a control. /// A protected field of type private Table owner; ///. Represents the collection internally. /// /// internal TableRowCollection(Table owner) { this.owner = owner; } ////// Gets the /// count of public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///in the collection. /// /// public TableRow this[int index] { get { return(TableRow)owner.Controls[index]; } } ////// Gets a ///referenced by the /// specified ordinal index value. /// /// public int Add(TableRow row) { AddAt(-1, row); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableRow row) { owner.Controls.AddAt(index, row); if (row.TableSection != TableRowSection.TableBody) { owner.HasRowSections = true; } } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableRow[] rows) { if (rows == null) { throw new ArgumentNullException("rows"); } foreach(TableRow row in rows) { Add(row); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); owner.HasRowSections = false; } } ///Removes all ///controls from the collection. /// public int GetRowIndex(TableRow row) { if (owner.HasControls()) { return owner.Controls.IndexOf(row); } return -1; } ///Returns an ordinal index value that denotes the position of the specified /// ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the collection. In /// this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableRow row) { owner.Controls.Remove(row); } ///Removes the specified ///from the collection. /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ///Removes the ///from the collection at the specified /// index location. object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableRow)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableRow) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableRow)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableRow)o); } /// void IList.Insert(int index, object o) { AddAt(index, (TableRow)o); } /// void IList.Remove(object o) { Remove((TableRow)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
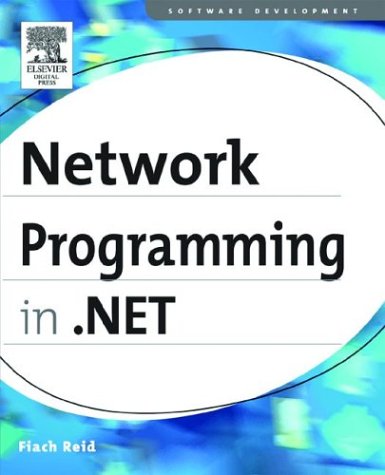
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaAny.cs
- SkewTransform.cs
- GetMemberBinder.cs
- FloaterBaseParagraph.cs
- AsyncDataRequest.cs
- BlobPersonalizationState.cs
- DataServiceHost.cs
- SplitterEvent.cs
- SmiConnection.cs
- ObjectDataSourceView.cs
- ObjectKeyFrameCollection.cs
- LabelDesigner.cs
- AsyncCompletedEventArgs.cs
- GuidTagList.cs
- DependencyPropertyAttribute.cs
- JulianCalendar.cs
- webclient.cs
- UIElementCollection.cs
- FontConverter.cs
- PointIndependentAnimationStorage.cs
- CodeSubDirectory.cs
- SqlBooleanMismatchVisitor.cs
- PageParserFilter.cs
- UnsafeNativeMethods.cs
- OracleParameterBinding.cs
- Wizard.cs
- WebPartExportVerb.cs
- LocationChangedEventArgs.cs
- XmlChoiceIdentifierAttribute.cs
- GridItemProviderWrapper.cs
- DeviceFilterEditorDialog.cs
- TraceEventCache.cs
- RichTextBox.cs
- _AutoWebProxyScriptEngine.cs
- EditorZoneBase.cs
- _TimerThread.cs
- GeneralTransform3D.cs
- DeferredElementTreeState.cs
- PropertyOrder.cs
- WinEventQueueItem.cs
- DataGridViewColumnEventArgs.cs
- DragDeltaEventArgs.cs
- Psha1DerivedKeyGenerator.cs
- WasAdminWrapper.cs
- PatternMatcher.cs
- GridPatternIdentifiers.cs
- AspProxy.cs
- Point3DAnimation.cs
- WebPartAuthorizationEventArgs.cs
- FileController.cs
- SqlNamer.cs
- InlinedAggregationOperatorEnumerator.cs
- PointLightBase.cs
- BindingObserver.cs
- AppManager.cs
- TextElementEnumerator.cs
- MSAAWinEventWrap.cs
- COM2ColorConverter.cs
- ResolveNameEventArgs.cs
- GC.cs
- ListenerSessionConnection.cs
- LineProperties.cs
- HandlerFactoryWrapper.cs
- CodeAccessPermission.cs
- InvokePatternIdentifiers.cs
- NativeMethods.cs
- SimpleWorkerRequest.cs
- XmlSortKey.cs
- CollectionBase.cs
- SqlParameter.cs
- StrongNamePublicKeyBlob.cs
- SqlUserDefinedAggregateAttribute.cs
- Literal.cs
- ListViewItem.cs
- MDIWindowDialog.cs
- SettingsPropertyWrongTypeException.cs
- ValidationErrorCollection.cs
- TraceSwitch.cs
- InputScopeConverter.cs
- CheckoutException.cs
- ExeConfigurationFileMap.cs
- BroadcastEventHelper.cs
- ProgressiveCrcCalculatingStream.cs
- ProfileSection.cs
- ConnectionPoint.cs
- MessageSecurityException.cs
- XmlObjectSerializerReadContextComplex.cs
- UnsafeNativeMethods.cs
- CryptoStream.cs
- ComponentResourceManager.cs
- KeyboardDevice.cs
- ArgumentException.cs
- AudioFormatConverter.cs
- RelativeSource.cs
- GlyphShapingProperties.cs
- XamlReader.cs
- ParserContext.cs
- ProjectionRewriter.cs
- SqlTypesSchemaImporter.cs
- UnsafeNativeMethods.cs