Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Runtime / Serialization / Formatters / Binary / BinaryObjectInfo.cs / 1 / BinaryObjectInfo.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SerObjectInfo ** ** ** Purpose: Holds information about an objects Members ** ** ===========================================================*/ namespace System.Runtime.Serialization.Formatters.Binary { using System.Runtime.Remoting; using System.Runtime.Serialization; using System; using System.Collections; using System.Reflection; using System.Diagnostics; using System.Globalization; // This class contains information about an object. It is used so that // the rest of the Formatter routines can use a common interface for // a normal object, an ISerializable object, and a surrogate object // // The methods in this class are for the internal use of the Formatters. // There use will be restricted when signing is supported for assemblies internal sealed class WriteObjectInfo { internal int objectInfoId; internal Object obj; internal Type objectType; internal bool isSi = false; internal bool isNamed = false; internal bool isTyped = false; internal bool isArray = false; internal SerializationInfo si = null; internal SerObjectInfoCache cache = null; internal Object[] memberData = null; internal ISerializationSurrogate serializationSurrogate = null; internal StreamingContext context; internal SerObjectInfoInit serObjectInfoInit = null; // Writing and Parsing information internal long objectId; internal long assemId; internal WriteObjectInfo() { } internal void ObjectEnd() { SerTrace.Log( this, objectInfoId," objectType ",objectType," ObjectEnd"); PutObjectInfo(serObjectInfoInit, this); } private void InternalInit() { SerTrace.Log( this, objectInfoId," objectType ",objectType," InternalInit"); obj = null; objectType = null; isSi = false; isNamed = false; isTyped = false; isArray = false; si = null; cache = null; memberData = null; // Writing and Parsing information objectId = 0; assemId = 0; } internal static WriteObjectInfo Serialize(Object obj, ISurrogateSelector surrogateSelector, StreamingContext context, SerObjectInfoInit serObjectInfoInit, IFormatterConverter converter, ObjectWriter objectWriter) { WriteObjectInfo soi = GetObjectInfo(serObjectInfoInit); soi.InitSerialize(obj, surrogateSelector, context, serObjectInfoInit, converter, objectWriter); return soi; } // Write constructor internal void InitSerialize(Object obj, ISurrogateSelector surrogateSelector, StreamingContext context, SerObjectInfoInit serObjectInfoInit, IFormatterConverter converter, ObjectWriter objectWriter) { SerTrace.Log( this, objectInfoId," Constructor 1 ",obj); this.context = context; this.obj = obj; this.serObjectInfoInit = serObjectInfoInit; ISurrogateSelector surrogateSelectorTemp; if (RemotingServices.IsTransparentProxy(obj)) objectType = Converter.typeofMarshalByRefObject; else objectType = obj.GetType(); if (objectType.IsArray) { isArray = true; InitNoMembers(); return; } SerTrace.Log( this, objectInfoId," Constructor 1 trace 2"); objectWriter.ObjectManager.RegisterObject(obj); if (surrogateSelector != null && (serializationSurrogate = surrogateSelector.GetSurrogate(objectType, context, out surrogateSelectorTemp)) != null) { SerTrace.Log( this, objectInfoId," Constructor 1 trace 3"); si = new SerializationInfo(objectType, converter); if (!objectType.IsPrimitive) serializationSurrogate.GetObjectData(obj, si, context); InitSiWrite(); } else if (obj is ISerializable) { if (!objectType.IsSerializable) { throw new SerializationException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("Serialization_NonSerType"), objectType.FullName, objectType.Assembly.FullName)); } si = new SerializationInfo(objectType, converter); ((ISerializable)obj).GetObjectData(si, context); SerTrace.Log( this, objectInfoId," Constructor 1 trace 4 ISerializable "+objectType); InitSiWrite(); } else { SerTrace.Log(this, objectInfoId," Constructor 1 trace 5"); InitMemberInfo(); } } [Conditional("SER_LOGGING")] private void DumpMemberInfo() { for (int i=0; i0 && cache.memberNames[lastPosition].Equals(name)) { return lastPosition; } else if ((++lastPosition < cache.memberNames.Length) && (cache.memberNames[lastPosition].Equals(name))) { return lastPosition; } else { // Search for name SerTrace.Log( this, objectInfoId," Position miss search for name "+name); for (int i=0; i
Link Menu
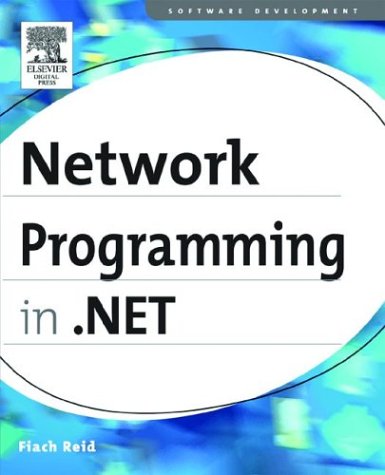
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SessionPageStateSection.cs
- SequenceFullException.cs
- compensatingcollection.cs
- CircleEase.cs
- WebDisplayNameAttribute.cs
- Run.cs
- PathSegmentCollection.cs
- Popup.cs
- EntityModelSchemaGenerator.cs
- BuildDependencySet.cs
- CodeGroup.cs
- HtmlInputRadioButton.cs
- SqlXmlStorage.cs
- ToolboxItem.cs
- InputReportEventArgs.cs
- StringOutput.cs
- GlobalProxySelection.cs
- CodeDomSerializationProvider.cs
- KeyValueConfigurationCollection.cs
- SystemIPInterfaceProperties.cs
- CompModSwitches.cs
- HttpCacheVary.cs
- ParseElement.cs
- HandlerFactoryWrapper.cs
- ResourcePart.cs
- FeatureSupport.cs
- SQLMoneyStorage.cs
- HierarchicalDataBoundControl.cs
- AvTrace.cs
- TreeIterator.cs
- SelectQueryOperator.cs
- EditingCommands.cs
- WindowsFont.cs
- StringDictionary.cs
- ButtonRenderer.cs
- Vector.cs
- TemplatePartAttribute.cs
- GetWorkflowTree.cs
- CodeBlockBuilder.cs
- BorderGapMaskConverter.cs
- ColumnHeaderCollectionEditor.cs
- MouseOverProperty.cs
- TextRangeAdaptor.cs
- BasicBrowserDialog.cs
- NextPreviousPagerField.cs
- CodeCatchClause.cs
- BinaryObjectWriter.cs
- ManipulationInertiaStartingEventArgs.cs
- GetFileNameResult.cs
- ActiveDocumentEvent.cs
- RepeaterItemEventArgs.cs
- AmbientValueAttribute.cs
- SingleBodyParameterMessageFormatter.cs
- _DisconnectOverlappedAsyncResult.cs
- XmlSchemaComplexType.cs
- WebPartPersonalization.cs
- DrawListViewSubItemEventArgs.cs
- SvcMapFile.cs
- EntityProviderServices.cs
- Localizer.cs
- UIElementParagraph.cs
- SqlStream.cs
- UdpUtility.cs
- Events.cs
- IsolatedStorageFilePermission.cs
- RoutingSection.cs
- SequenceDesigner.cs
- VersionConverter.cs
- XmlSchemaSet.cs
- CryptoSession.cs
- TransformConverter.cs
- TemplateBindingExtensionConverter.cs
- ConfigurationSchemaErrors.cs
- QueryComponents.cs
- BaseCodeDomTreeGenerator.cs
- ListBindingHelper.cs
- RedBlackList.cs
- GridViewSelectEventArgs.cs
- TabControl.cs
- StylusPointPropertyInfo.cs
- XsdCachingReader.cs
- SpotLight.cs
- HttpProfileBase.cs
- EntityViewGenerator.cs
- ping.cs
- XmlSchemaSimpleTypeList.cs
- InvokeProviderWrapper.cs
- CustomErrorsSectionWrapper.cs
- FileChangesMonitor.cs
- MemberCollection.cs
- HTTPNotFoundHandler.cs
- OutputScope.cs
- ErrorInfoXmlDocument.cs
- WorkflowRuntime.cs
- RichTextBoxAutomationPeer.cs
- SystemBrushes.cs
- Command.cs
- MdImport.cs
- ToolStrip.cs
- Int32AnimationUsingKeyFrames.cs