Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Shared / MS / Internal / SecurityCriticalDataForSet.cs / 1 / SecurityCriticalDataForSet.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a helper class to facilate the storage of data that's Critical for set. // The data itself is not information disclosure but the value controls a critical // operation. // // For example a filepath variable might control what part of the file system the // code gets access to. // // History: // 01/30/05 : [....] Created. // //--------------------------------------------------------------------------- using System ; using System.Security ; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif PRESENTATIONUI using MS.Internal.PresentationUI; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Internal { [FriendAccessAllowed] // Built into Base, also used by Core and Framework. [Serializable] internal struct SecurityCriticalDataForSet{ /// /// Critical - "by definition" - this class is intended only for data that's /// Critical for setting. /// [SecurityCritical] internal SecurityCriticalDataForSet(T value) { _value = value; } ////// Critical - Setter is Critical "by definition" - this class is intended only /// for data that's Critical for setting. /// Safe - get is safe by definition. /// Not Safe - set is not safe by definition. /// internal T Value { #if DEBUG [System.Diagnostics.DebuggerStepThrough] #endif [SecurityCritical, SecurityTreatAsSafe] get { return _value; } #if DEBUG [System.Diagnostics.DebuggerStepThrough] #endif [SecurityCritical] set { _value = value; } } ////// Critical - by definition as this data is Critical for set. /// > [SecurityCritical] private T _value; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This is a helper class to facilate the storage of data that's Critical for set. // The data itself is not information disclosure but the value controls a critical // operation. // // For example a filepath variable might control what part of the file system the // code gets access to. // // History: // 01/30/05 : [....] Created. // //--------------------------------------------------------------------------- using System ; using System.Security ; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif PRESENTATIONUI using MS.Internal.PresentationUI; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Internal { [FriendAccessAllowed] // Built into Base, also used by Core and Framework. [Serializable] internal struct SecurityCriticalDataForSet{ /// /// Critical - "by definition" - this class is intended only for data that's /// Critical for setting. /// [SecurityCritical] internal SecurityCriticalDataForSet(T value) { _value = value; } ////// Critical - Setter is Critical "by definition" - this class is intended only /// for data that's Critical for setting. /// Safe - get is safe by definition. /// Not Safe - set is not safe by definition. /// internal T Value { #if DEBUG [System.Diagnostics.DebuggerStepThrough] #endif [SecurityCritical, SecurityTreatAsSafe] get { return _value; } #if DEBUG [System.Diagnostics.DebuggerStepThrough] #endif [SecurityCritical] set { _value = value; } } ////// Critical - by definition as this data is Critical for set. /// > [SecurityCritical] private T _value; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
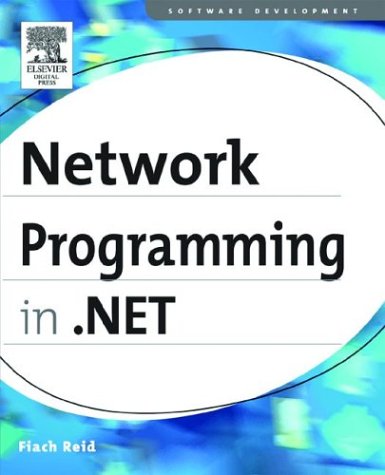
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolboxItemFilterAttribute.cs
- ProjectedSlot.cs
- BatchParser.cs
- RawStylusSystemGestureInputReport.cs
- Vector3DAnimation.cs
- PersonalizationProviderHelper.cs
- AsyncCompletedEventArgs.cs
- WindowsHyperlink.cs
- SerializationSectionGroup.cs
- Mapping.cs
- ConnectionConsumerAttribute.cs
- CriticalFinalizerObject.cs
- InkCanvasInnerCanvas.cs
- EntityViewGenerationAttribute.cs
- MasterPageParser.cs
- InvalidOperationException.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- EntityDataSourceReferenceGroup.cs
- RecognitionResult.cs
- CompressEmulationStream.cs
- TextEditorSelection.cs
- WebException.cs
- AttributeAction.cs
- ListSourceHelper.cs
- PointAnimationClockResource.cs
- TextEditorSpelling.cs
- FileVersionInfo.cs
- ButtonBase.cs
- ConfigXmlAttribute.cs
- Soap12FormatExtensions.cs
- PixelFormat.cs
- WebPartVerbCollection.cs
- ContentControl.cs
- SqlBooleanMismatchVisitor.cs
- FieldToken.cs
- DataException.cs
- SessionSymmetricTransportSecurityProtocolFactory.cs
- ScrollViewer.cs
- CultureTableRecord.cs
- ObjectItemCachedAssemblyLoader.cs
- WsdlHelpGeneratorElement.cs
- ViewCellSlot.cs
- CleanUpVirtualizedItemEventArgs.cs
- Int16KeyFrameCollection.cs
- DescendentsWalkerBase.cs
- CapiNative.cs
- ConfigurationStrings.cs
- KeyGestureValueSerializer.cs
- hwndwrapper.cs
- PrefixQName.cs
- MulticastIPAddressInformationCollection.cs
- XmlTextReaderImplHelpers.cs
- SelectionProviderWrapper.cs
- ParameterCollection.cs
- PartDesigner.cs
- RootContext.cs
- ConfigurationStrings.cs
- WeakReference.cs
- DocumentViewerConstants.cs
- DbParameterHelper.cs
- DataSourceCollectionBase.cs
- SqlDataAdapter.cs
- CustomLineCap.cs
- FilteredXmlReader.cs
- TreeNodeCollection.cs
- WebPartConnectionsConfigureVerb.cs
- NamedObject.cs
- UnsafeNativeMethods.cs
- Type.cs
- Queue.cs
- SessionStateModule.cs
- XmlWellformedWriter.cs
- DataGridViewToolTip.cs
- ProcessInputEventArgs.cs
- HttpCookie.cs
- AssemblyHash.cs
- Typography.cs
- ClientTarget.cs
- SecurityManager.cs
- CompensationToken.cs
- HandlerElementCollection.cs
- TypefaceMap.cs
- WindowsNonControl.cs
- SR.Designer.cs
- GridSplitterAutomationPeer.cs
- KeyNotFoundException.cs
- CombinedGeometry.cs
- SqlUtils.cs
- RawStylusInputCustomData.cs
- RenderOptions.cs
- Point3D.cs
- DatasetMethodGenerator.cs
- ScrollData.cs
- GAC.cs
- RoleManagerModule.cs
- SaveWorkflowAsyncResult.cs
- InternalConfigRoot.cs
- CommandField.cs
- PluggableProtocol.cs
- Properties.cs