Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Objects / objectquery_tresulttype.cs / 1 / objectquery_tresulttype.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- using System; using System.Text.RegularExpressions; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Globalization; using System.Diagnostics; using System.Data; using System.Data.Common; using System.Data.Common.EntitySql; using System.Data.Common.Utils; using System.Data.Mapping; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; using System.Data.Common.CommandTrees.Internal; using System.Data.Objects.DataClasses; using System.Data.Objects.ELinq; using System.Data.Objects.Internal; using System.Data.Common.QueryCache; using System.Data.EntityClient; using System.Linq; using System.Linq.Expressions; namespace System.Data.Objects { ////// This class implements strongly-typed queries at the object-layer through /// Entity SQL text and query-building helper methods. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public partial class ObjectQuery: ObjectQuery, IEnumerable , IQueryable , IOrderedQueryable , IListSource { internal ObjectQuery(ObjectQueryState queryState) : base(queryState) { } #region Public Methods /// /// This method allows explicit query evaluation with a specified merge /// option which will override the merge option property. /// /// /// The MergeOption to use when executing the query. /// ////// An enumerable for the ObjectQuery results. /// public new ObjectResultExecute(MergeOption mergeOption) { EntityUtil.CheckArgumentMergeOption(mergeOption); return this.GetResults(mergeOption); } /// /// Adds a path to the set of navigation property span paths included in the results of this query /// /// The new span path ///A new ObjectQuery that includes the specified span path public ObjectQueryInclude(string path) { EntityUtil.CheckStringArgument(path, "path"); return new ObjectQuery (this.QueryState.Include(this, path)); } #endregion #region IEnumerable implementation /// /// These methods are the "executors" for the query. They can be called /// directly, or indirectly (by foreach'ing through the query, for example). /// IEnumeratorIEnumerable .GetEnumerator() { ObjectResult disposableEnumerable = this.GetResults(null); try { IEnumerator result = disposableEnumerable.GetEnumerator(); return result; } catch { // if there is a problem creating the enumerator, we should dispose // the enumerable (if there is no problem, the enumerator will take // care of the dispose) disposableEnumerable.Dispose(); throw; } } #endregion #region ObjectQuery Overrides internal override IEnumerator GetEnumeratorInternal() { return ((IEnumerable )this).GetEnumerator(); } internal override IList GetIListSourceListInternal() { return ((IListSource)this.GetResults(null)).GetList(); } internal override ObjectResult ExecuteInternal(MergeOption mergeOption) { return this.GetResults(mergeOption); } #endregion #region Private Methods private ObjectResult GetResults(MergeOption? forMergeOption) { this.QueryState.ObjectContext.EnsureConnection(); try { ObjectQueryExecutionPlan execPlan = this.QueryState.GetExecutionPlan(forMergeOption); return execPlan.Execute (this.QueryState.ObjectContext, this.QueryState.Parameters); } catch { this.QueryState.ObjectContext.ReleaseConnection(); throw; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- using System; using System.Text.RegularExpressions; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Globalization; using System.Diagnostics; using System.Data; using System.Data.Common; using System.Data.Common.EntitySql; using System.Data.Common.Utils; using System.Data.Mapping; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; using System.Data.Common.CommandTrees.Internal; using System.Data.Objects.DataClasses; using System.Data.Objects.ELinq; using System.Data.Objects.Internal; using System.Data.Common.QueryCache; using System.Data.EntityClient; using System.Linq; using System.Linq.Expressions; namespace System.Data.Objects { ////// This class implements strongly-typed queries at the object-layer through /// Entity SQL text and query-building helper methods. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public partial class ObjectQuery: ObjectQuery, IEnumerable , IQueryable , IOrderedQueryable , IListSource { internal ObjectQuery(ObjectQueryState queryState) : base(queryState) { } #region Public Methods /// /// This method allows explicit query evaluation with a specified merge /// option which will override the merge option property. /// /// /// The MergeOption to use when executing the query. /// ////// An enumerable for the ObjectQuery results. /// public new ObjectResultExecute(MergeOption mergeOption) { EntityUtil.CheckArgumentMergeOption(mergeOption); return this.GetResults(mergeOption); } /// /// Adds a path to the set of navigation property span paths included in the results of this query /// /// The new span path ///A new ObjectQuery that includes the specified span path public ObjectQueryInclude(string path) { EntityUtil.CheckStringArgument(path, "path"); return new ObjectQuery (this.QueryState.Include(this, path)); } #endregion #region IEnumerable implementation /// /// These methods are the "executors" for the query. They can be called /// directly, or indirectly (by foreach'ing through the query, for example). /// IEnumeratorIEnumerable .GetEnumerator() { ObjectResult disposableEnumerable = this.GetResults(null); try { IEnumerator result = disposableEnumerable.GetEnumerator(); return result; } catch { // if there is a problem creating the enumerator, we should dispose // the enumerable (if there is no problem, the enumerator will take // care of the dispose) disposableEnumerable.Dispose(); throw; } } #endregion #region ObjectQuery Overrides internal override IEnumerator GetEnumeratorInternal() { return ((IEnumerable )this).GetEnumerator(); } internal override IList GetIListSourceListInternal() { return ((IListSource)this.GetResults(null)).GetList(); } internal override ObjectResult ExecuteInternal(MergeOption mergeOption) { return this.GetResults(mergeOption); } #endregion #region Private Methods private ObjectResult GetResults(MergeOption? forMergeOption) { this.QueryState.ObjectContext.EnsureConnection(); try { ObjectQueryExecutionPlan execPlan = this.QueryState.GetExecutionPlan(forMergeOption); return execPlan.Execute (this.QueryState.ObjectContext, this.QueryState.Parameters); } catch { this.QueryState.ObjectContext.ReleaseConnection(); throw; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
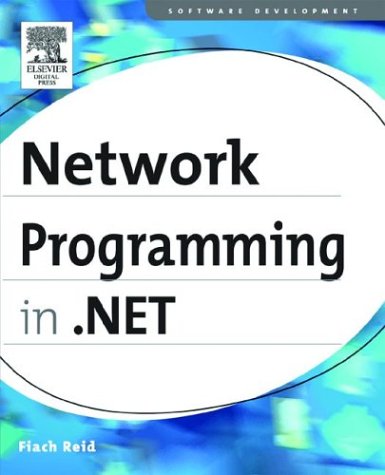
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- basecomparevalidator.cs
- UnsafeNativeMethodsTablet.cs
- LazyInitializer.cs
- GroupItemAutomationPeer.cs
- UInt64Storage.cs
- PopupControlService.cs
- NetWebProxyFinder.cs
- ConfigurationProperty.cs
- ConfigurationValue.cs
- ParameterModifier.cs
- UpdatePanel.cs
- DataGridViewRowEventArgs.cs
- DbTypeMap.cs
- SqlRowUpdatedEvent.cs
- CodeMethodMap.cs
- PlatformNotSupportedException.cs
- DataRowCollection.cs
- EntitySqlQueryBuilder.cs
- CodeNamespaceImport.cs
- DefaultShape.cs
- WindowsProgressbar.cs
- DesignTimeTemplateParser.cs
- CatalogZoneBase.cs
- PageAdapter.cs
- ApplicationSecurityInfo.cs
- SingleAnimationUsingKeyFrames.cs
- ClientOptions.cs
- ConditionCollection.cs
- StringConcat.cs
- PowerModeChangedEventArgs.cs
- SEHException.cs
- ChtmlLinkAdapter.cs
- TextReturnReader.cs
- XmlStreamStore.cs
- XmlAttributeCollection.cs
- HTMLTextWriter.cs
- CacheForPrimitiveTypes.cs
- Types.cs
- EntityDataSourceMemberPath.cs
- SourceFileBuildProvider.cs
- StructuredTypeEmitter.cs
- Trace.cs
- SafeProcessHandle.cs
- StyleCollectionEditor.cs
- SessionStateModule.cs
- GetWinFXPath.cs
- DateTimeValueSerializer.cs
- WindowsAuthenticationEventArgs.cs
- BooleanSwitch.cs
- FragmentQueryProcessor.cs
- ResourceExpressionEditorSheet.cs
- SqlUdtInfo.cs
- XPathPatternBuilder.cs
- PackageRelationship.cs
- PersonalizationAdministration.cs
- DaylightTime.cs
- Selection.cs
- RoutedEvent.cs
- X509Certificate2.cs
- RayHitTestParameters.cs
- ChangePassword.cs
- DataBindingCollection.cs
- MetadataItem_Static.cs
- AssociationSet.cs
- IntegerFacetDescriptionElement.cs
- SchemaAttDef.cs
- unsafenativemethodsother.cs
- XmlSchemaSimpleContent.cs
- UnionCqlBlock.cs
- PriorityQueue.cs
- ExternalCalls.cs
- SchemaImporter.cs
- StoreContentChangedEventArgs.cs
- WebPartPersonalization.cs
- PeerNeighborManager.cs
- SessionEndingEventArgs.cs
- ServicePointManagerElement.cs
- _FtpDataStream.cs
- WmlCalendarAdapter.cs
- DbConnectionPoolOptions.cs
- GenerateTemporaryTargetAssembly.cs
- UrlMappingsModule.cs
- SocketElement.cs
- BufferModeSettings.cs
- EntityDataSourceEntitySetNameItem.cs
- MDIControlStrip.cs
- ComboBox.cs
- XmlQualifiedNameTest.cs
- LateBoundBitmapDecoder.cs
- SingleTagSectionHandler.cs
- WebPartEditorOkVerb.cs
- GZipStream.cs
- DataGridViewRowCancelEventArgs.cs
- XmlParserContext.cs
- IndexedString.cs
- CodeChecksumPragma.cs
- DataGridCommandEventArgs.cs
- EFColumnProvider.cs
- ErrorStyle.cs
- Base64Encoding.cs