Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbLambda.cs / 1305376 / DbLambda.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common.CommandTrees.ExpressionBuilder.Internal; using ReadOnlyVariables = System.Collections.ObjectModel.ReadOnlyCollection; using System.Data.Common.CommandTrees.ExpressionBuilder; using System.Data.Metadata.Edm; using System.Reflection; namespace System.Data.Common.CommandTrees { /// /// Represents a Lambda function that can be invoked to produce a [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbLambda { private readonly ReadOnlyVariables _variables; private readonly DbExpression _body; internal DbLambda(ReadOnlyVariables variables, DbExpression bodyExp) { Debug.Assert(variables != null, "DbLambda.Variables cannot be null"); Debug.Assert(bodyExp != null, "DbLambda.Body cannot be null"); this._variables = variables; this._body = bodyExp; } ///. /// /// Gets the public DbExpression Body { get { return this._body; } } ///that provides the definition of the Lambda function /// /// Gets the public ILists that represent the parameters to the Lambda function and are in scope within . /// Variables { get { return this._variables; } } #if PUBLIC_DBEXPRESSIONBUILDER /// /// Creates a /// An expression that defines the logic of the Lambda function /// /// Awith the specified inline Lambda function implementation and formal parameters. /// collection that represents the formal parameters to the Lambda function. /// These variables are valid for use in the expression. /// /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters ////// . ///is null or contains null, or is null /// /// public static DbLambda Create(DbExpression body, IEnumerablecontains more than one element with the same variable name. /// variables) { return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a /// An expression that defines the logic of the Lambda function /// /// Awith the specified inline Lambda function implementation and formal parameters. /// collection that represents the formal parameters to the Lambda function. /// These variables are valid for use in the expression. /// /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters ////// . ///is null or contains null, or is null /// /// public static DbLambda Create(DbExpression body, params DbVariableReferenceExpression[] variables) { return DbExpressionBuilder.Lambda(body, variables); } ///contains more than one element with the same variable name. /// /// Creates a new /// Awith a single argument of the specified type, as defined by the specified function. /// that defines the EDM type of the argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and single formal parameter. ////// public static DbLambda Create(TypeUsage argument1Type, Funcis null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type); DbExpression body = lambdaFunction(variables[0]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, Funcis null, is null, /// or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type); DbExpression body = lambdaFunction(variables[0], variables[1]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, Funcis null, is null, is null /// or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, Funcis null, is null, is null, /// is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, Funcis null, is null, is null, /// is null, is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, Funcis null, is null, is null, /// is null, is null, is null, /// or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A that defines the EDM type of the tenth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, TypeUsage argument10Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(argument10Type, "argument10Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type, argument10Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8], variables[9]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A that defines the EDM type of the tenth argument to the Lambda function /// A that defines the EDM type of the eleventh argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, TypeUsage argument10Type, TypeUsage argument11Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(argument10Type, "argument10Type"); EntityUtil.CheckArgumentNull(argument11Type, "argument11Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type, argument10Type, argument11Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8], variables[9], variables[10]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A that defines the EDM type of the tenth argument to the Lambda function /// A that defines the EDM type of the eleventh argument to the Lambda function /// A that defines the EDM type of the twelfth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, TypeUsage argument10Type, TypeUsage argument11Type, TypeUsage argument12Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(argument10Type, "argument10Type"); EntityUtil.CheckArgumentNull(argument11Type, "argument11Type"); EntityUtil.CheckArgumentNull(argument12Type, "argument12Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type, argument10Type, argument11Type, argument12Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8], variables[9], variables[10], variables[11]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A that defines the EDM type of the tenth argument to the Lambda function /// A that defines the EDM type of the eleventh argument to the Lambda function /// A that defines the EDM type of the twelfth argument to the Lambda function /// A that defines the EDM type of the thirteenth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, TypeUsage argument10Type, TypeUsage argument11Type, TypeUsage argument12Type, TypeUsage argument13Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(argument10Type, "argument10Type"); EntityUtil.CheckArgumentNull(argument11Type, "argument11Type"); EntityUtil.CheckArgumentNull(argument12Type, "argument12Type"); EntityUtil.CheckArgumentNull(argument13Type, "argument13Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type, argument10Type, argument11Type, argument12Type, argument13Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8], variables[9], variables[10], variables[11], variables[12]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A that defines the EDM type of the tenth argument to the Lambda function /// A that defines the EDM type of the eleventh argument to the Lambda function /// A that defines the EDM type of the twelfth argument to the Lambda function /// A that defines the EDM type of the thirteenth argument to the Lambda function /// A that defines the EDM type of the fourteenth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, TypeUsage argument10Type, TypeUsage argument11Type, TypeUsage argument12Type, TypeUsage argument13Type, TypeUsage argument14Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(argument10Type, "argument10Type"); EntityUtil.CheckArgumentNull(argument11Type, "argument11Type"); EntityUtil.CheckArgumentNull(argument12Type, "argument12Type"); EntityUtil.CheckArgumentNull(argument13Type, "argument13Type"); EntityUtil.CheckArgumentNull(argument14Type, "argument14Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type, argument10Type, argument11Type, argument12Type, argument13Type, argument14Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8], variables[9], variables[10], variables[11], variables[12], variables[13]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A that defines the EDM type of the tenth argument to the Lambda function /// A that defines the EDM type of the eleventh argument to the Lambda function /// A that defines the EDM type of the twelfth argument to the Lambda function /// A that defines the EDM type of the thirteenth argument to the Lambda function /// A that defines the EDM type of the fourteenth argument to the Lambda function /// A that defines the EDM type of the fifteenth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, TypeUsage argument10Type, TypeUsage argument11Type, TypeUsage argument12Type, TypeUsage argument13Type, TypeUsage argument14Type, TypeUsage argument15Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(argument10Type, "argument10Type"); EntityUtil.CheckArgumentNull(argument11Type, "argument11Type"); EntityUtil.CheckArgumentNull(argument12Type, "argument12Type"); EntityUtil.CheckArgumentNull(argument13Type, "argument13Type"); EntityUtil.CheckArgumentNull(argument14Type, "argument14Type"); EntityUtil.CheckArgumentNull(argument15Type, "argument15Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type, argument10Type, argument11Type, argument12Type, argument13Type, argument14Type, argument15Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8], variables[9], variables[10], variables[11], variables[12], variables[13], variables[14]); return DbExpressionBuilder.Lambda(body, variables); } /// /// Creates a new /// Awith arguments of the specified types, as defined by the specified function. /// that defines the EDM type of the first argument to the Lambda function /// A that defines the EDM type of the second argument to the Lambda function /// A that defines the EDM type of the third argument to the Lambda function /// A that defines the EDM type of the fourth argument to the Lambda function /// A that defines the EDM type of the fifth argument to the Lambda function /// A that defines the EDM type of the sixth argument to the Lambda function /// A that defines the EDM type of the seventh argument to the Lambda function /// A that defines the EDM type of the eighth argument to the Lambda function /// A that defines the EDM type of the ninth argument to the Lambda function /// A that defines the EDM type of the tenth argument to the Lambda function /// A that defines the EDM type of the eleventh argument to the Lambda function /// A that defines the EDM type of the twelfth argument to the Lambda function /// A that defines the EDM type of the thirteenth argument to the Lambda function /// A that defines the EDM type of the fourteenth argument to the Lambda function /// A that defines the EDM type of the fifteenth argument to the Lambda function /// A that defines the EDM type of the sixteenth argument to the Lambda function /// A function that defines the logic of the Lambda function as a /// A new DbLambda that describes an inline Lambda function with the specified body and formal parameters. ////// public static DbLambda Create(TypeUsage argument1Type, TypeUsage argument2Type, TypeUsage argument3Type, TypeUsage argument4Type, TypeUsage argument5Type, TypeUsage argument6Type, TypeUsage argument7Type, TypeUsage argument8Type, TypeUsage argument9Type, TypeUsage argument10Type, TypeUsage argument11Type, TypeUsage argument12Type, TypeUsage argument13Type, TypeUsage argument14Type, TypeUsage argument15Type, TypeUsage argument16Type, Funcis null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, is null, is null, /// is null, or is null or produces a result of null. /// lambdaFunction) { EntityUtil.CheckArgumentNull(argument1Type, "argument1Type"); EntityUtil.CheckArgumentNull(argument2Type, "argument2Type"); EntityUtil.CheckArgumentNull(argument3Type, "argument3Type"); EntityUtil.CheckArgumentNull(argument4Type, "argument4Type"); EntityUtil.CheckArgumentNull(argument5Type, "argument5Type"); EntityUtil.CheckArgumentNull(argument6Type, "argument6Type"); EntityUtil.CheckArgumentNull(argument7Type, "argument7Type"); EntityUtil.CheckArgumentNull(argument8Type, "argument8Type"); EntityUtil.CheckArgumentNull(argument9Type, "argument9Type"); EntityUtil.CheckArgumentNull(argument10Type, "argument10Type"); EntityUtil.CheckArgumentNull(argument11Type, "argument11Type"); EntityUtil.CheckArgumentNull(argument12Type, "argument12Type"); EntityUtil.CheckArgumentNull(argument13Type, "argument13Type"); EntityUtil.CheckArgumentNull(argument14Type, "argument14Type"); EntityUtil.CheckArgumentNull(argument15Type, "argument15Type"); EntityUtil.CheckArgumentNull(argument16Type, "argument16Type"); EntityUtil.CheckArgumentNull(lambdaFunction, "lambdaFunction"); DbVariableReferenceExpression[] variables = CreateVariables(lambdaFunction.Method, argument1Type, argument2Type, argument3Type, argument4Type, argument5Type, argument6Type, argument7Type, argument8Type, argument9Type, argument10Type, argument11Type, argument12Type, argument13Type, argument14Type, argument15Type, argument16Type); DbExpression body = lambdaFunction(variables[0], variables[1], variables[2], variables[3], variables[4], variables[5], variables[6], variables[7], variables[8], variables[9], variables[10], variables[11], variables[12], variables[13], variables[14], variables[15]); return DbExpressionBuilder.Lambda(body, variables); } private static DbVariableReferenceExpression[] CreateVariables(MethodInfo lambdaMethod, params TypeUsage[] argumentTypes) { Debug.Assert(lambdaMethod != null, "Lambda function method must not be null"); string[] paramNames = DbExpressionBuilder.ExtractAliases(lambdaMethod); Debug.Assert(paramNames.Length == argumentTypes.Length, "Lambda function method parameter count does not match argument count"); DbVariableReferenceExpression[] result = new DbVariableReferenceExpression[argumentTypes.Length]; for (int idx = 0; idx < paramNames.Length; idx++) { Debug.Assert(argumentTypes[idx] != null, "DbLambda.Create allowed null type argument"); result[idx] = argumentTypes[idx].Variable(paramNames[idx]); } return result; } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
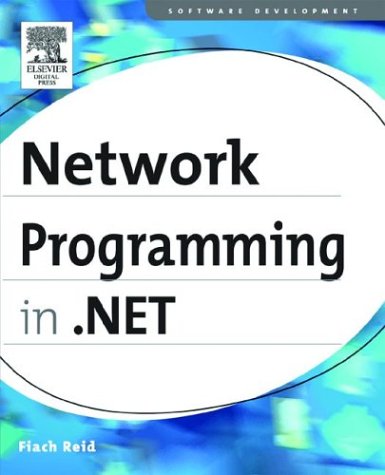
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SemanticResultKey.cs
- WmfPlaceableFileHeader.cs
- Qualifier.cs
- DataGridHeaderBorder.cs
- ProxyGenerationError.cs
- DocumentReference.cs
- LinqDataSourceDeleteEventArgs.cs
- SymbolMethod.cs
- FixedStringLookup.cs
- XPathNodeInfoAtom.cs
- DefaultEventAttribute.cs
- Pen.cs
- OrderByBuilder.cs
- SrgsElementFactory.cs
- TextBoxAutoCompleteSourceConverter.cs
- DataServiceRequestArgs.cs
- ListDictionaryInternal.cs
- MulticastNotSupportedException.cs
- ServiceProviders.cs
- ObjectSet.cs
- ToolStripDropDownItemDesigner.cs
- ContentValidator.cs
- XAMLParseException.cs
- ResourceExpressionBuilder.cs
- HotSpot.cs
- Point4D.cs
- HttpSocketManager.cs
- TransportReplyChannelAcceptor.cs
- MsmqIntegrationElement.cs
- ClientRoleProvider.cs
- Size.cs
- StylusLogic.cs
- RealProxy.cs
- GenericUriParser.cs
- WSDualHttpBindingElement.cs
- ButtonFlatAdapter.cs
- Baml2006KnownTypes.cs
- CompoundFileIOPermission.cs
- FlowDocumentScrollViewer.cs
- StyleHelper.cs
- TypeUtil.cs
- SqlNodeTypeOperators.cs
- ZipIOCentralDirectoryFileHeader.cs
- SplitterPanel.cs
- ZoneLinkButton.cs
- MeasurementDCInfo.cs
- PropertiesTab.cs
- SemanticResultKey.cs
- XomlDesignerLoader.cs
- IssuedTokenParametersEndpointAddressElement.cs
- SyndicationDeserializer.cs
- XmlSchemaObjectTable.cs
- SafeEventLogReadHandle.cs
- SystemIcons.cs
- AddIn.cs
- DelimitedListTraceListener.cs
- Marshal.cs
- AssemblyCollection.cs
- Shape.cs
- Buffer.cs
- SignedXml.cs
- OSFeature.cs
- RepeatButtonAutomationPeer.cs
- AppDomainAttributes.cs
- ContentFilePart.cs
- DependencyStoreSurrogate.cs
- DateTimeFormatInfoScanner.cs
- HostingEnvironmentException.cs
- XmlILModule.cs
- ResourceSetExpression.cs
- DrawingBrush.cs
- SamlDelegatingWriter.cs
- PowerEase.cs
- PenCursorManager.cs
- DataTemplate.cs
- StylusLogic.cs
- PasswordDeriveBytes.cs
- _NetworkingPerfCounters.cs
- Queue.cs
- SkewTransform.cs
- SpeechSeg.cs
- NullExtension.cs
- X509ChainPolicy.cs
- XmlObjectSerializerReadContextComplexJson.cs
- ReadOnlyPropertyMetadata.cs
- AdapterDictionary.cs
- ClientConvert.cs
- OrthographicCamera.cs
- QuinticEase.cs
- IpcClientManager.cs
- sqlcontext.cs
- ValidationHelper.cs
- DbMetaDataFactory.cs
- WebControlsSection.cs
- NamespaceDecl.cs
- CodeTypeMember.cs
- DataGridViewCell.cs
- LogicalExpressionEditor.cs
- TrackingMemoryStreamFactory.cs
- Message.cs