Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Log / System / IO / Log / LogExtentCollection.cs / 1305376 / LogExtentCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IO.Log { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using Microsoft.Win32.SafeHandles; public sealed class LogExtentCollection : IEnumerable{ LogStore store; int version; internal LogExtentCollection(LogStore store) { this.store = store; } public int Count { get { CLFS_INFORMATION info; this.store.GetLogFileInformation(out info); return (int)info.TotalContainers; } } public int FreeCount { get { CLFS_INFORMATION info; this.store.GetLogFileInformation(out info); return (int)info.FreeContainers; } } int Version { get { return this.version; } } LogStore Store { get { return this.store; } } public void Add(string path) { if(string.IsNullOrEmpty(path)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentNull("path")); } if (this.Count == 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.InvalidOperation(SR.LogStore_SizeRequired)); } UnsafeNativeMethods.AddLogContainerNoSizeSync( this.store.Handle, path); this.version++; } public void Add(string path, long size) { if(string.IsNullOrEmpty(path)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentNull("path")); } if (size <= 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentOutOfRange("size")); } CLFS_INFORMATION info; this.store.GetLogFileInformation(out info); if ((ulong)size < info.ContainerSize) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentInvalid(SR.LogStore_SizeTooSmall)); } ulong ulSize = (ulong)size; UnsafeNativeMethods.AddLogContainerSync( this.store.Handle, ref ulSize, path); this.version++; } IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } public IEnumerator GetEnumerator() { return new LogExtentEnumerator(this); } public void Remove(string path, bool force) { if(string.IsNullOrEmpty(path)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentNull("path")); } UnsafeNativeMethods.RemoveLogContainerSync( this.store.Handle, path, force); this.version++; } public void Remove(LogExtent extent, bool force) { if(extent == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ArgumentNull("extent")); } UnsafeNativeMethods.RemoveLogContainerSync( this.store.Handle, extent.Path, force); this.version++; } class LogExtentEnumerator : IEnumerator { LogExtentCollection collection; List .Enumerator innerEnum; int version; public LogExtentEnumerator(LogExtentCollection collection) { this.collection = collection; this.version = this.collection.Version; SafeFileHandle logHandle = this.collection.Store.Handle; CLFS_SCAN_CONTEXT scanContext = new CLFS_SCAN_CONTEXT(); try { List extents = new List (); CLFS_INFORMATION logInfo; this.collection.Store.GetLogFileInformation(out logInfo); if (logInfo.TotalContainers > 0) { UnsafeNativeMethods.CreateLogContainerScanContextSync( logHandle, 0, logInfo.TotalContainers, CLFS_SCAN_MODE.CLFS_SCAN_FORWARD, ref scanContext); long containerPointer = scanContext.pinfoContainer.ToInt64(); CLFS_CONTAINER_INFORMATION_WRAPPER info; info = new CLFS_CONTAINER_INFORMATION_WRAPPER(); int infoSize; infoSize = Marshal.SizeOf(typeof(CLFS_CONTAINER_INFORMATION_WRAPPER)); for (uint i = 0; i < scanContext.cContainersReturned; i++) { Marshal.PtrToStructure(new IntPtr(containerPointer), info); LogExtent extent = new LogExtent( info.info.GetActualFileName(logHandle), info.info.FileSize, (LogExtentState)info.info.State); extents.Add(extent); containerPointer += infoSize; } } this.innerEnum = extents.GetEnumerator(); } finally { if ((scanContext.eScanMode & CLFS_SCAN_MODE.CLFS_SCAN_INITIALIZED) != 0) { UnsafeNativeMethods.ScanLogContainersSyncClose( ref scanContext); } } } object IEnumerator.Current { get { return this.Current; } } public LogExtent Current { get { return this.innerEnum.Current; } } public bool MoveNext() { if (this.version != this.collection.Version) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( Error.InvalidOperation(SR.InvalidOperation_EnumFailedVersion)); } return this.innerEnum.MoveNext(); } public void Dispose() { this.innerEnum.Dispose(); } public void Reset() { ((IEnumerator)this.innerEnum).Reset(); this.version = this.collection.Version; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
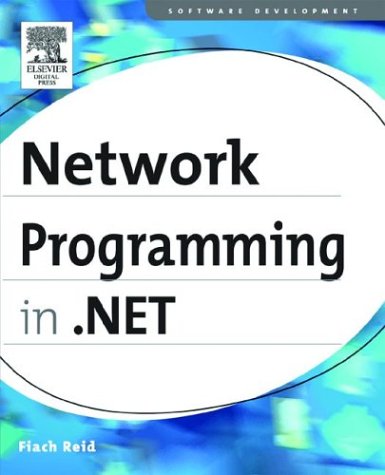
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusTip.cs
- Parallel.cs
- cookiecontainer.cs
- ToolStripTemplateNode.cs
- MultiTrigger.cs
- CodePageEncoding.cs
- Timer.cs
- tibetanshape.cs
- SimpleTypeResolver.cs
- DispatchChannelSink.cs
- StateValidator.cs
- PriorityChain.cs
- unsafeIndexingFilterStream.cs
- PtsHelper.cs
- CaseInsensitiveOrdinalStringComparer.cs
- RangeBase.cs
- Expander.cs
- EntityObject.cs
- EditModeSwitchButton.cs
- ResourceSetExpression.cs
- DesignerVerbCollection.cs
- GroupBoxRenderer.cs
- DocumentSchemaValidator.cs
- QueryConverter.cs
- SplitterDesigner.cs
- TemplateManager.cs
- ProjectedSlot.cs
- IPeerNeighbor.cs
- ColorConverter.cs
- RelatedPropertyManager.cs
- DataGridViewTextBoxCell.cs
- StorageAssociationTypeMapping.cs
- AssemblySettingAttributes.cs
- WebPartUserCapability.cs
- ErrorWrapper.cs
- HyperLink.cs
- ListBoxItem.cs
- TextStore.cs
- PassportPrincipal.cs
- Menu.cs
- ViewEvent.cs
- ToolStripProgressBar.cs
- PseudoWebRequest.cs
- ExcludeFromCodeCoverageAttribute.cs
- WebRequest.cs
- RegexWorker.cs
- CssStyleCollection.cs
- Fx.cs
- OperationExecutionFault.cs
- DataGridViewTextBoxCell.cs
- GC.cs
- TrackPoint.cs
- Automation.cs
- storepermissionattribute.cs
- XhtmlBasicTextViewAdapter.cs
- ScrollChrome.cs
- WebRequestModuleElementCollection.cs
- Convert.cs
- WebEncodingValidatorAttribute.cs
- BaseDataListDesigner.cs
- NameValueConfigurationCollection.cs
- EntityDataSourceStatementEditorForm.cs
- BackgroundWorker.cs
- WizardPanel.cs
- ReadOnlyDictionary.cs
- BooleanStorage.cs
- InvalidAsynchronousStateException.cs
- BitmapEffectInput.cs
- ItemsPanelTemplate.cs
- TransformerConfigurationWizardBase.cs
- MetadataItemCollectionFactory.cs
- AssociationTypeEmitter.cs
- ColorConvertedBitmap.cs
- TrackBar.cs
- TransformCryptoHandle.cs
- ReferencedCollectionType.cs
- StandardCommands.cs
- NumberFormatter.cs
- GeneratedContractType.cs
- PropertyChangedEventManager.cs
- XmlNamedNodeMap.cs
- ReturnType.cs
- NodeLabelEditEvent.cs
- Stroke.cs
- DataGridViewCell.cs
- ErrorStyle.cs
- NameScope.cs
- Type.cs
- ScaleTransform.cs
- ReflectPropertyDescriptor.cs
- ApplicationInfo.cs
- LinkClickEvent.cs
- Table.cs
- Condition.cs
- MediaTimeline.cs
- Property.cs
- LogEntry.cs
- TextBoxView.cs
- SamlSerializer.cs
- ArrayHelper.cs