Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Expander.cs / 1305600 / Expander.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.ComponentModel; using MS.Internal.KnownBoxes; namespace System.Windows.Controls { ////// Specifies the expanding direction of a expansion. /// public enum ExpandDirection { ////// Expander will expand to the down direction. /// Down = 0, ////// Expander will expand to the up direction. /// Up = 1, ////// Expander will expand to the left direction. /// Left = 2, ////// Expander will expand to the right direction. /// Right = 3, } ////// An Expander control allows a user to view a header and expand that /// header to see further details of the content, or to collapse the section /// up to the header to save space. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // cannot be read & localized as string public class Expander : HeaderedContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors static Expander() { DefaultStyleKeyProperty.OverrideMetadata(typeof(Expander), new FrameworkPropertyMetadata(typeof(Expander))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(Expander)); IsTabStopProperty.OverrideMetadata(typeof(Expander), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); IsMouseOverPropertyKey.OverrideMetadata(typeof(Expander), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); IsEnabledProperty.OverrideMetadata(typeof(Expander), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); } #endregion //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// ExpandDirection specifies to which direction the content will expand /// [Bindable(true), Category("Behavior")] public ExpandDirection ExpandDirection { get { return (ExpandDirection) GetValue(ExpandDirectionProperty); } set { SetValue(ExpandDirectionProperty, value); } } ////// The DependencyProperty for the ExpandDirection property. /// Default Value: ExpandDirection.Down /// public static readonly DependencyProperty ExpandDirectionProperty = DependencyProperty.Register( "ExpandDirection", typeof(ExpandDirection), typeof(Expander), new FrameworkPropertyMetadata( ExpandDirection.Down /* default value */, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault, new PropertyChangedCallback(OnVisualStatePropertyChanged)), new ValidateValueCallback(IsValidExpandDirection)); private static bool IsValidExpandDirection(object o) { ExpandDirection value = (ExpandDirection)o; return (value == ExpandDirection.Down || value == ExpandDirection.Left || value == ExpandDirection.Right || value == ExpandDirection.Up); } ////// The DependencyProperty for the IsExpanded property. /// Default Value: false /// public static readonly DependencyProperty IsExpandedProperty = DependencyProperty.Register( "IsExpanded", typeof(bool), typeof(Expander), new FrameworkPropertyMetadata( BooleanBoxes.FalseBox /* default value */, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault | FrameworkPropertyMetadataOptions.Journal, new PropertyChangedCallback(OnIsExpandedChanged))); private static void OnIsExpandedChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Expander ep = (Expander) d; bool newValue = (bool) e.NewValue; // Fire accessibility event ExpanderAutomationPeer peer = UIElementAutomationPeer.FromElement(ep) as ExpanderAutomationPeer; if(peer != null) { peer.RaiseExpandCollapseAutomationEvent(!newValue, newValue); } if (newValue) { ep.OnExpanded(); } else { ep.OnCollapsed(); } ep.UpdateVisualState(); } ////// IsExpanded indicates whether the expander is currently expanded. /// [Bindable(true), Category("Appearance")] public bool IsExpanded { get { return (bool) GetValue(IsExpandedProperty); } set { SetValue(IsExpandedProperty, BooleanBoxes.Box(value)); } } ////// Expanded event. /// public static readonly RoutedEvent ExpandedEvent = EventManager.RegisterRoutedEvent("Expanded", RoutingStrategy.Bubble, typeof(RoutedEventHandler), typeof(Expander) ); ////// Expanded event. It is fired when IsExpanded changed from false to true. /// public event RoutedEventHandler Expanded { add { AddHandler(ExpandedEvent, value); } remove { RemoveHandler(ExpandedEvent, value); } } ////// Collapsed event. /// public static readonly RoutedEvent CollapsedEvent = EventManager.RegisterRoutedEvent("Collapsed", RoutingStrategy.Bubble, typeof(RoutedEventHandler), typeof(Expander) ); ////// Collapsed event. It is fired when IsExpanded changed from true to false. /// public event RoutedEventHandler Collapsed { add { AddHandler(CollapsedEvent, value); } remove { RemoveHandler(CollapsedEvent, value); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods internal override void ChangeVisualState(bool useTransitions) { // Handle the Common states if (!IsEnabled) { VisualStates.GoToState(this, useTransitions, VisualStates.StateDisabled, VisualStates.StateNormal); } else if (IsMouseOver) { VisualStates.GoToState(this, useTransitions, VisualStates.StateMouseOver, VisualStates.StateNormal); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateNormal); } // Handle the Focused states if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateFocused, VisualStates.StateUnfocused); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateUnfocused); } if (IsExpanded) { VisualStates.GoToState(this, useTransitions, VisualStates.StateExpanded); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateCollapsed); } switch (ExpandDirection) { case ExpandDirection.Down: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandDown); break; case ExpandDirection.Up: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandUp); break; case ExpandDirection.Left: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandLeft); break; default: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandRight); break; } base.ChangeVisualState(useTransitions); } ////// A virtual function that is called when the IsExpanded property is changed to true. /// Default behavior is to raise an ExpandedEvent. /// protected virtual void OnExpanded() { RoutedEventArgs args = new RoutedEventArgs(); args.RoutedEvent = Expander.ExpandedEvent; args.Source = this; RaiseEvent(args); } ////// A virtual function that is called when the IsExpanded property is changed to false. /// Default behavior is to raise a CollapsedEvent. /// protected virtual void OnCollapsed() { RaiseEvent(new RoutedEventArgs(Expander.CollapsedEvent, this)); } #endregion #region Accessibility ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ExpanderAutomationPeer(this); } #endregion //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Automation; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.ComponentModel; using MS.Internal.KnownBoxes; namespace System.Windows.Controls { ///) /// /// Specifies the expanding direction of a expansion. /// public enum ExpandDirection { ////// Expander will expand to the down direction. /// Down = 0, ////// Expander will expand to the up direction. /// Up = 1, ////// Expander will expand to the left direction. /// Left = 2, ////// Expander will expand to the right direction. /// Right = 3, } ////// An Expander control allows a user to view a header and expand that /// header to see further details of the content, or to collapse the section /// up to the header to save space. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // cannot be read & localized as string public class Expander : HeaderedContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors static Expander() { DefaultStyleKeyProperty.OverrideMetadata(typeof(Expander), new FrameworkPropertyMetadata(typeof(Expander))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(Expander)); IsTabStopProperty.OverrideMetadata(typeof(Expander), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); IsMouseOverPropertyKey.OverrideMetadata(typeof(Expander), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); IsEnabledProperty.OverrideMetadata(typeof(Expander), new UIPropertyMetadata(new PropertyChangedCallback(OnVisualStatePropertyChanged))); } #endregion //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// ExpandDirection specifies to which direction the content will expand /// [Bindable(true), Category("Behavior")] public ExpandDirection ExpandDirection { get { return (ExpandDirection) GetValue(ExpandDirectionProperty); } set { SetValue(ExpandDirectionProperty, value); } } ////// The DependencyProperty for the ExpandDirection property. /// Default Value: ExpandDirection.Down /// public static readonly DependencyProperty ExpandDirectionProperty = DependencyProperty.Register( "ExpandDirection", typeof(ExpandDirection), typeof(Expander), new FrameworkPropertyMetadata( ExpandDirection.Down /* default value */, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault, new PropertyChangedCallback(OnVisualStatePropertyChanged)), new ValidateValueCallback(IsValidExpandDirection)); private static bool IsValidExpandDirection(object o) { ExpandDirection value = (ExpandDirection)o; return (value == ExpandDirection.Down || value == ExpandDirection.Left || value == ExpandDirection.Right || value == ExpandDirection.Up); } ////// The DependencyProperty for the IsExpanded property. /// Default Value: false /// public static readonly DependencyProperty IsExpandedProperty = DependencyProperty.Register( "IsExpanded", typeof(bool), typeof(Expander), new FrameworkPropertyMetadata( BooleanBoxes.FalseBox /* default value */, FrameworkPropertyMetadataOptions.BindsTwoWayByDefault | FrameworkPropertyMetadataOptions.Journal, new PropertyChangedCallback(OnIsExpandedChanged))); private static void OnIsExpandedChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { Expander ep = (Expander) d; bool newValue = (bool) e.NewValue; // Fire accessibility event ExpanderAutomationPeer peer = UIElementAutomationPeer.FromElement(ep) as ExpanderAutomationPeer; if(peer != null) { peer.RaiseExpandCollapseAutomationEvent(!newValue, newValue); } if (newValue) { ep.OnExpanded(); } else { ep.OnCollapsed(); } ep.UpdateVisualState(); } ////// IsExpanded indicates whether the expander is currently expanded. /// [Bindable(true), Category("Appearance")] public bool IsExpanded { get { return (bool) GetValue(IsExpandedProperty); } set { SetValue(IsExpandedProperty, BooleanBoxes.Box(value)); } } ////// Expanded event. /// public static readonly RoutedEvent ExpandedEvent = EventManager.RegisterRoutedEvent("Expanded", RoutingStrategy.Bubble, typeof(RoutedEventHandler), typeof(Expander) ); ////// Expanded event. It is fired when IsExpanded changed from false to true. /// public event RoutedEventHandler Expanded { add { AddHandler(ExpandedEvent, value); } remove { RemoveHandler(ExpandedEvent, value); } } ////// Collapsed event. /// public static readonly RoutedEvent CollapsedEvent = EventManager.RegisterRoutedEvent("Collapsed", RoutingStrategy.Bubble, typeof(RoutedEventHandler), typeof(Expander) ); ////// Collapsed event. It is fired when IsExpanded changed from true to false. /// public event RoutedEventHandler Collapsed { add { AddHandler(CollapsedEvent, value); } remove { RemoveHandler(CollapsedEvent, value); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods internal override void ChangeVisualState(bool useTransitions) { // Handle the Common states if (!IsEnabled) { VisualStates.GoToState(this, useTransitions, VisualStates.StateDisabled, VisualStates.StateNormal); } else if (IsMouseOver) { VisualStates.GoToState(this, useTransitions, VisualStates.StateMouseOver, VisualStates.StateNormal); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateNormal); } // Handle the Focused states if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateFocused, VisualStates.StateUnfocused); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateUnfocused); } if (IsExpanded) { VisualStates.GoToState(this, useTransitions, VisualStates.StateExpanded); } else { VisualStates.GoToState(this, useTransitions, VisualStates.StateCollapsed); } switch (ExpandDirection) { case ExpandDirection.Down: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandDown); break; case ExpandDirection.Up: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandUp); break; case ExpandDirection.Left: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandLeft); break; default: VisualStates.GoToState(this, useTransitions, VisualStates.StateExpandRight); break; } base.ChangeVisualState(useTransitions); } ////// A virtual function that is called when the IsExpanded property is changed to true. /// Default behavior is to raise an ExpandedEvent. /// protected virtual void OnExpanded() { RoutedEventArgs args = new RoutedEventArgs(); args.RoutedEvent = Expander.ExpandedEvent; args.Source = this; RaiseEvent(args); } ////// A virtual function that is called when the IsExpanded property is changed to false. /// Default behavior is to raise a CollapsedEvent. /// protected virtual void OnCollapsed() { RaiseEvent(new RoutedEventArgs(Expander.CollapsedEvent, this)); } #endregion #region Accessibility ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new ExpanderAutomationPeer(this); } #endregion //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.) ///
Link Menu
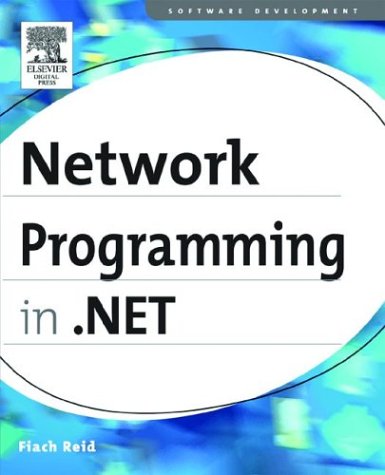
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ModifyActivitiesPropertyDescriptor.cs
- XmlTextReaderImpl.cs
- MeasureData.cs
- PenLineCapValidation.cs
- RightsManagementPermission.cs
- CodeMemberMethod.cs
- XomlCompilerHelpers.cs
- Assert.cs
- ObjectConverter.cs
- ViewService.cs
- SecurityProtocolFactory.cs
- TextBox.cs
- MeshGeometry3D.cs
- Inflater.cs
- ADMembershipUser.cs
- serverconfig.cs
- DateTimeFormatInfoScanner.cs
- SelectedGridItemChangedEvent.cs
- XmlSchemaParticle.cs
- Timer.cs
- CodeNamespaceImport.cs
- MethodBody.cs
- BackStopAuthenticationModule.cs
- SelectedDatesCollection.cs
- XPathDocumentNavigator.cs
- FileVersionInfo.cs
- EnvironmentPermission.cs
- SqlProfileProvider.cs
- CodeParameterDeclarationExpressionCollection.cs
- DelegateSerializationHolder.cs
- SqlDataSourceWizardForm.cs
- PictureBox.cs
- FormatterConverter.cs
- XmlReflectionImporter.cs
- IntegerCollectionEditor.cs
- PrintEvent.cs
- RestHandler.cs
- DialogResultConverter.cs
- Size.cs
- SystemDropShadowChrome.cs
- IDispatchConstantAttribute.cs
- SmiEventStream.cs
- GeneralTransform.cs
- ProfileService.cs
- Blend.cs
- WrappingXamlSchemaContext.cs
- SqlDataSourceFilteringEventArgs.cs
- DesignerForm.cs
- Selector.cs
- _RequestLifetimeSetter.cs
- ToolStripDropTargetManager.cs
- ClientSponsor.cs
- BitmapPalette.cs
- TextParagraphProperties.cs
- DrawingGroup.cs
- AccessDataSourceView.cs
- SafeViewOfFileHandle.cs
- FixedSOMSemanticBox.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- ParserContext.cs
- Inline.cs
- Stack.cs
- ScrollBar.cs
- MaterialCollection.cs
- DataGridViewRowPrePaintEventArgs.cs
- AutoCompleteStringCollection.cs
- SafeLocalMemHandle.cs
- GridLengthConverter.cs
- XmlTextReaderImplHelpers.cs
- HandlerBase.cs
- CopyOnWriteList.cs
- InternalDuplexChannelListener.cs
- Point4D.cs
- ImageResources.Designer.cs
- NativeMethodsCLR.cs
- WebPartsPersonalizationAuthorization.cs
- FunctionDetailsReader.cs
- ToolStripSystemRenderer.cs
- XMLSchema.cs
- Configuration.cs
- TypeUsage.cs
- XmlResolver.cs
- DataGridDetailsPresenter.cs
- OleDbException.cs
- filewebrequest.cs
- remotingproxy.cs
- ClientSponsor.cs
- SpellCheck.cs
- MenuItemStyle.cs
- EventLog.cs
- SqlNotificationEventArgs.cs
- ImageCodecInfo.cs
- RectangleConverter.cs
- CodeSubDirectoriesCollection.cs
- ComplexBindingPropertiesAttribute.cs
- WindowsProgressbar.cs
- KeyInterop.cs
- ReferentialConstraintRoleElement.cs
- OleDbConnectionFactory.cs
- FilteredDataSetHelper.cs