Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Controls / WebBrowserEvent.cs / 1 / WebBrowserEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // WebBrowserEvent is used to listen to the DWebBrowserEvent2 // of the webbrowser control // // Copied from WebBrowse.cs in winforms // // History // 04/17/05 [....] Created // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using System.Windows; using System.Text; using MS.Win32; //In order to avoid generating warnings about unknown message numbers and //unknown pragmas when compiling your C# source code with the actual C# compiler, //you need to disable warnings 1634 and 1691. (Presharp Documentation) #pragma warning disable 1634, 1691 namespace MS.Internal.Controls { // // Private classes: // [ClassInterface(ClassInterfaceType.None)] internal class WebBrowserEvent : UnsafeNativeMethods.DWebBrowserEvents2 { private WebBrowser parent; public WebBrowserEvent(WebBrowser parent) { this.parent = parent; Debug.Assert(parent != null, "WebBrowser control required for hooking webbrowser events"); } public void CommandStateChange(long command, bool enable) { // // //if (command == NativeMethods.CSC_NAVIGATEBACK) { // this.parent.CanGoBackInternal = enable; //} //else if (command == NativeMethods.CSC_NAVIGATEFORWARD) { // this.parent.CanGoForwardInternal = enable; //} } ////// Critical: This code extracts the IWebBrowser2 interface /// TreatAsSafe: This does not expose the interface, also calling this will not cause a navigation /// it simply enforces a few checks /// [SecurityCritical,SecurityTreatAsSafe] public void BeforeNavigate2(object pDisp, ref object url, ref object flags, ref object targetFrameName, ref object postData, ref object headers, ref bool cancel) { try { Debug.Assert(url == null || url is string, "invalid url type"); Debug.Assert(targetFrameName == null || targetFrameName is string, "invalid targetFrameName type"); Debug.Assert(headers == null || headers is string, "invalid headers type"); // // Due to a bug in the interop code where the variant.bstr value gets set // to -1 on return back to native code, if the original value was null, we // have to set targetFrameName and headers to "". if (targetFrameName == null) { targetFrameName = ""; } if (headers == null) { headers = ""; } UnsafeNativeMethods.IWebBrowser2 axIWebBrowser2 = (UnsafeNativeMethods.IWebBrowser2)pDisp; // This is the condition where we are in a sub window like a frame or iframe or embedded webOC // in that case we do not want to enforce site locking as we want the defualt IE behavior to take // over. if (this.parent.GetAxIWebBrowser2() != axIWebBrowser2) { cancel = false; } else { WebBrowserNavigatingEventArgs e = new WebBrowserNavigatingEventArgs( url == null ? "" : (string)url, targetFrameName == null ? "" : (string)targetFrameName); this.parent.OnNavigating(e); cancel = e.Cancel; } } // We disable this to suppress FXCop warning since in this case we really want to catch all exceptions // please refer to comment below #pragma warning disable 6502 catch { // This is an interesting pattern of putting a try catch block around this that catches everything, // The reason I do this is based on a conversation with [....]. What happens here is if there is // an exception in any of the code above then navigation still continues since COM interop eats up // the exception. But what we want is for this navigation to fail. // There fore I catch all exceptions and cancel navigation cancel = true; } #pragma warning restore 6502 } public void DocumentComplete(object pDisp, ref object url) { //Debug.Assert(url == null || url is string, "invalid url"); //if (this.parent.documentStreamToSetOnLoad != null && (string)url == "about:blank") { //HtmlDocument htmlDocument = this.parent.Document; // The get_Document call does a security check // if (htmlDocument != null) { // UnsafeNativeMethods.IPersistStreamInit psi = htmlDocument.DomDocument as UnsafeNativeMethods.IPersistStreamInit; // Debug.Assert(psi != null, "The Document does not implement IPersistStreamInit"); // UnsafeNativeMethods.IStream iStream = (UnsafeNativeMethods.IStream)new UnsafeNativeMethods.ComStreamFromDataStream( // this.parent.documentStreamToSetOnLoad); // psi.Load(iStream); // } // this.parent.documentStreamToSetOnLoad = null; // this.parent.ShouldSerializeDocumentTextInternal = true; //} //else { // this.parent.ShouldSerializeDocumentTextInternal = false; // WebBrowserDocumentCompletedEventArgs e = new WebBrowserDocumentCompletedEventArgs( // url == null ? "" : (string)url); // this.parent.OnDocumentCompleted(e); //} } public void TitleChange(string text) { //this.parent.OnDocumentTitleChanged(EventArgs.Empty); } public void SetSecureLockIcon(int secureLockIcon) { //this.parent.encryptionLevel = (WebBrowserEncryptionLevel)secureLockIcon; //this.parent.OnEncryptionLevelChanged(EventArgs.Empty); } public void NavigateComplete2(object pDisp, ref object url) { Debug.Assert(url == null || url is string, "invalid url type"); //WebBrowserNavigatedEventArgs e = new WebBrowserNavigatedEventArgs( //url == null ? "" : (string)url); //this.parent.OnNavigated(e); } public void NewWindow2(ref object ppDisp, ref bool cancel) { //CancelEventArgs e = new CancelEventArgs(); //this.parent.OnNewWindow(e); //cancel = e.Cancel; } public void ProgressChange(int progress, int progressMax) { //WebBrowserProgressChangedEventArgs e = new WebBrowserProgressChangedEventArgs(progress, progressMax); //this.parent.OnProgressChanged(e); } public void StatusTextChange(string text) { //this.parent.statusText = (text == null) ? "" : text; //this.parent.OnStatusTextChanged(EventArgs.Empty); } public void DownloadBegin() { //this.parent.OnFileDownload(EventArgs.Empty); } public void FileDownload(ref bool cancel) { } public void PrivacyImpactedStateChange(bool bImpacted) { } public void UpdatePageStatus(object pDisp, ref object nPage, ref object fDone) { } public void PrintTemplateTeardown(object pDisp) { } public void PrintTemplateInstantiation(object pDisp) { } public void NavigateError(object pDisp, ref object url, ref object frame, ref object statusCode, ref bool cancel) { } public void ClientToHostWindow(ref long cX, ref long cY) { } public void WindowClosing(bool isChildWindow, ref bool cancel) { } public void WindowSetHeight(int height) { } public void WindowSetWidth(int width) { } public void WindowSetTop(int top) { } public void WindowSetLeft(int left) { } public void WindowSetResizable(bool resizable) { } public void OnTheaterMode(bool theaterMode) { } public void OnFullScreen(bool fullScreen) { } public void OnStatusBar(bool statusBar) { } public void OnMenuBar(bool menuBar) { } public void OnToolBar(bool toolBar) { } public void OnVisible(bool visible) { } public void OnQuit() { } public void PropertyChange(string szProperty) { } public void DownloadComplete() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
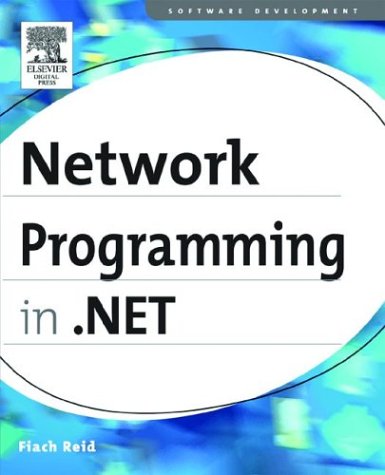
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ASCIIEncoding.cs
- DesignerActionItem.cs
- CodeComment.cs
- Schema.cs
- MobileControlPersister.cs
- SchemaExporter.cs
- DeviceSpecificChoice.cs
- CapabilitiesPattern.cs
- EncoderExceptionFallback.cs
- TextContainerChangedEventArgs.cs
- ScrollItemPatternIdentifiers.cs
- DesignerUtility.cs
- CssTextWriter.cs
- TextSimpleMarkerProperties.cs
- TdsParameterSetter.cs
- CodeDirectoryCompiler.cs
- XamlSerializerUtil.cs
- ConfigErrorGlyph.cs
- EventNotify.cs
- Sentence.cs
- TimeZone.cs
- MailDefinition.cs
- PageClientProxyGenerator.cs
- SecurityResources.cs
- TextTreeExtractElementUndoUnit.cs
- OracleRowUpdatingEventArgs.cs
- SeparatorAutomationPeer.cs
- X509CertificateCollection.cs
- SqlUserDefinedTypeAttribute.cs
- ComplexObject.cs
- NumberFormatInfo.cs
- HashHelper.cs
- ExtenderControl.cs
- NamedPipeAppDomainProtocolHandler.cs
- FontFamilyValueSerializer.cs
- CodeTypeReferenceCollection.cs
- TextLine.cs
- UriSection.cs
- cryptoapiTransform.cs
- HttpException.cs
- UnionQueryOperator.cs
- ThreadStateException.cs
- BatchParser.cs
- IsolatedStorageFile.cs
- Adorner.cs
- XmlILCommand.cs
- ToolStripLocationCancelEventArgs.cs
- CqlGenerator.cs
- PersonalizationStateQuery.cs
- WindowsFormsHostAutomationPeer.cs
- XmlSchemaObjectTable.cs
- ExtensionQuery.cs
- EDesignUtil.cs
- WizardPanelChangingEventArgs.cs
- DataColumnCollection.cs
- ConnectionStringsExpressionBuilder.cs
- ClientRoleProvider.cs
- EncryptedKeyIdentifierClause.cs
- SQLSingleStorage.cs
- UserMapPath.cs
- HelpEvent.cs
- PropertyItemInternal.cs
- Attributes.cs
- TextContainerHelper.cs
- DllNotFoundException.cs
- ToolBar.cs
- TypeDescriptorContext.cs
- SoapParser.cs
- ObjectDataSourceDisposingEventArgs.cs
- GACMembershipCondition.cs
- ListBoxDesigner.cs
- ICspAsymmetricAlgorithm.cs
- IIS7UserPrincipal.cs
- SourceFileBuildProvider.cs
- FileSecurity.cs
- WebBrowserSiteBase.cs
- VisualStyleRenderer.cs
- SSmlParser.cs
- EntitySqlQueryCacheKey.cs
- PathFigureCollectionValueSerializer.cs
- ProgramNode.cs
- SamlAttribute.cs
- TypeContext.cs
- PeerResolver.cs
- PrivilegeNotHeldException.cs
- SelectedCellsCollection.cs
- TemplateField.cs
- ToolStripRenderer.cs
- CompiledQueryCacheEntry.cs
- KeyValueSerializer.cs
- WsdlBuildProvider.cs
- GlyphRunDrawing.cs
- SHA512CryptoServiceProvider.cs
- WebBrowserEvent.cs
- UnmanagedMarshal.cs
- NotFiniteNumberException.cs
- DateTimeValueSerializer.cs
- DetailsView.cs
- DesignerLoader.cs
- Publisher.cs