Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / SelectedCellsCollection.cs / 1305600 / SelectedCellsCollection.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; namespace System.Windows.Controls { ////// A collection that optimizes the storage of DataGridCellInfo. /// ////// The collection is exposed through the DataGrid.SelectedCells property as /// a generic IList. /// /// The collection maintains a list of DataGridCellInfo so that users of the /// SelectedCells property can interact with it like a normal list. /// /// The collection maintains a dictionary mapping rows to columns and /// a dictionary that maps columns to rows. This allows quick retrieval /// of all selected cells in a particular row or column. These are /// operations that occur when select/deselecting a row or column. /// /// The collection implements all the parts of INotifyCollectionChanged so /// that the DataGrid can be notified of changes, but does not expose the /// interface so that SelectedCells can't be cast to it. This was to /// reduce the test coverage and the undiscoverability of the interface. /// internal sealed class SelectedCellsCollection : VirtualizedCellInfoCollection { #region Construction internal SelectedCellsCollection(DataGrid owner) : base(owner) { } #endregion #region DataGrid API ////// Calculates the bounding box of the cells. /// ///true if not empty, false if empty. internal bool GetSelectionRange(out int minColumnDisplayIndex, out int maxColumnDisplayIndex, out int minRowIndex, out int maxRowIndex) { if (IsEmpty) { minColumnDisplayIndex = -1; maxColumnDisplayIndex = -1; minRowIndex = -1; maxRowIndex = -1; return false; } else { GetBoundingRegion(out minColumnDisplayIndex, out minRowIndex, out maxColumnDisplayIndex, out maxRowIndex); return true; } } #endregion #region Collection Changed Notification ////// Notify the owning DataGrid of changes to this collection. /// protected override void OnCollectionChanged(NotifyCollectionChangedAction action, VirtualizedCellInfoCollection oldItems, VirtualizedCellInfoCollection newItems) { Owner.OnSelectedCellsChanged(action, oldItems, newItems); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
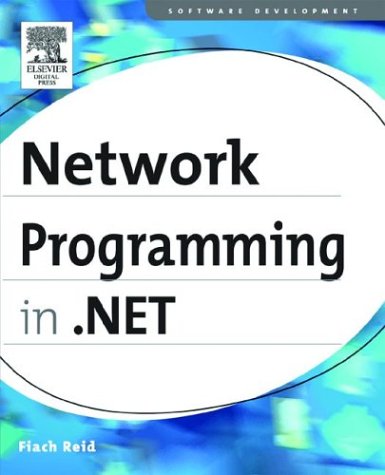
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentViewerBase.cs
- CapabilitiesPattern.cs
- ShaderEffect.cs
- Transform.cs
- ToolStripLabel.cs
- OdbcConnectionStringbuilder.cs
- AxHost.cs
- ListenerAdapterBase.cs
- SQLMoneyStorage.cs
- TreeView.cs
- TextFormatter.cs
- Subset.cs
- ProfileBuildProvider.cs
- RSACryptoServiceProvider.cs
- X509SecurityToken.cs
- Stack.cs
- RecordBuilder.cs
- BaseParagraph.cs
- SAPICategories.cs
- ServiceNotStartedException.cs
- PropertyManager.cs
- Viewport3DVisual.cs
- FacetEnabledSchemaElement.cs
- FirstMatchCodeGroup.cs
- MimeParameterWriter.cs
- XmlQualifiedName.cs
- CapiHashAlgorithm.cs
- ChangePasswordDesigner.cs
- MappingException.cs
- AttributeUsageAttribute.cs
- Menu.cs
- HwndSourceParameters.cs
- X509UI.cs
- EntityModelSchemaGenerator.cs
- CutCopyPasteHelper.cs
- XmlSchemaExternal.cs
- ResourceReferenceKeyNotFoundException.cs
- AspNetSynchronizationContext.cs
- ObjectItemConventionAssemblyLoader.cs
- CharConverter.cs
- SafeViewOfFileHandle.cs
- Knowncolors.cs
- IPPacketInformation.cs
- OleDbDataAdapter.cs
- SqlFunctionAttribute.cs
- ResourceReader.cs
- Pair.cs
- Select.cs
- QilLiteral.cs
- AutomationInteropProvider.cs
- BindingEntityInfo.cs
- XhtmlTextWriter.cs
- CircleHotSpot.cs
- Tuple.cs
- milexports.cs
- TokenFactoryFactory.cs
- UInt32Converter.cs
- RegexCompilationInfo.cs
- DataObjectAttribute.cs
- ACL.cs
- AudioFormatConverter.cs
- ListViewContainer.cs
- FilteredAttributeCollection.cs
- ExpressionBindingCollection.cs
- SoapEnumAttribute.cs
- TcpStreams.cs
- ConsoleKeyInfo.cs
- AppDomainUnloadedException.cs
- IssuedTokensHeader.cs
- EnvironmentPermission.cs
- Convert.cs
- SmtpMail.cs
- FlagsAttribute.cs
- SynchronizationFilter.cs
- DeviceContext2.cs
- VSWCFServiceContractGenerator.cs
- SettingsContext.cs
- MimeWriter.cs
- DependencyObject.cs
- CachedBitmap.cs
- AnimationClock.cs
- ToolBarOverflowPanel.cs
- OpenFileDialog.cs
- DefaultEventAttribute.cs
- arabicshape.cs
- DetailsView.cs
- XmlDeclaration.cs
- StyleTypedPropertyAttribute.cs
- DnsPermission.cs
- Sequence.cs
- SecureConversationVersion.cs
- DoubleIndependentAnimationStorage.cs
- GenericTypeParameterConverter.cs
- ByteStack.cs
- BooleanConverter.cs
- RequestCachePolicy.cs
- PhysicalOps.cs
- WindowsScrollBarBits.cs
- SqlDependencyListener.cs
- ScriptManagerProxy.cs