Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Annotations / Storage / StoreAnnotationsMap.cs / 1 / StoreAnnotationsMap.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Map of annotation instances handed out by a particular store. // Used to guarantee only one instance of an annotation is ever // produced. Also register for notifications on the instances // and passes change notifications on to the store. // // Spec: [....]/sites/ag/Specifications/CAF%20Storage%20Spec.doc // // History: // 07/15/2004: [....]: Added new class. // 08/18/2004: [....]: Added querying capabilities to the map to improve performance // 10/20/2004: [....]: Moved class to MS.Internal. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.IO; using System.Windows; using System.Windows.Annotations; using MS.Utility; namespace MS.Internal.Annotations.Storage { ////// A map the store uses to maintain having one instance /// of the object model /// internal class StoreAnnotationsMap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor for StoreAnnotationsMap /// It takes instances of the AnnotationAuthorChangedEventHandler, AnnotationResourceChangedEventHandler, AnnotationResourceChangedEventHandler delegates /// /// delegate used to register for AuthorChanged events on all annotations /// delegate used to register for AnchorChanged events on all annotations /// delegate used to register for CargoChanged events on all annotations internal StoreAnnotationsMap(AnnotationAuthorChangedEventHandler authorChanged, AnnotationResourceChangedEventHandler anchorChanged, AnnotationResourceChangedEventHandler cargoChanged) { Debug.Assert(authorChanged != null && anchorChanged != null && cargoChanged != null, "Author and Anchor and Cargo must not be null"); _authorChanged = authorChanged; _anchorChanged = anchorChanged; _cargoChanged = cargoChanged; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Add an annotation to the map /// throws an exception if the annotation already exists /// /// instance of annotation to add to the map /// the initial dirty flag of the annotation in the map public void AddAnnotation(Annotation annotation, bool dirty) { Debug.Assert(FindAnnotation(annotation.Id) == null, "annotation not found"); annotation.AuthorChanged += OnAuthorChanged; annotation.AnchorChanged += OnAnchorChanged; annotation.CargoChanged += OnCargoChanged; _currentAnnotations.Add(annotation.Id, new CachedAnnotation(annotation, dirty)); } ////// remove an annotation from the map if it exists /// otherwise return /// /// id of the annotation to remove public void RemoveAnnotation(Guid id) { CachedAnnotation existingCachedAnnot = null; if (_currentAnnotations.TryGetValue(id, out existingCachedAnnot)) { existingCachedAnnot.Annotation.AuthorChanged -= OnAuthorChanged; existingCachedAnnot.Annotation.AnchorChanged -= OnAnchorChanged; existingCachedAnnot.Annotation.CargoChanged -= OnCargoChanged; _currentAnnotations.Remove(id); } } ////// Queries the store map for annotations that have an anchor /// that contains a locator that begins with the locator parts /// in anchorLocator. /// /// the locator we are looking for ////// A list of annotations that have locators in their anchors /// starting with the same locator parts list as of the input locator /// If no such annotations an empty list will be returned. The method /// never returns null. /// public DictionaryFindAnnotations(ContentLocator anchorLocator) { if (anchorLocator == null) throw new ArgumentNullException("locator"); Dictionary annotations = new Dictionary (); // Dictionary .ValueCollection.Enumerator annotationsEnumerator = _currentAnnotations.Values.GetEnumerator(); while (annotationsEnumerator.MoveNext()) { Annotation annotation = annotationsEnumerator.Current.Annotation; bool matchesQuery = false; foreach (AnnotationResource resource in annotation.Anchors) { foreach (ContentLocatorBase locator in resource.ContentLocators) { ContentLocator ContentLocator = locator as ContentLocator; if (ContentLocator != null) { if (ContentLocator.StartsWith(anchorLocator)) { matchesQuery = true; } } else { ContentLocatorGroup ContentLocatorGroup = locator as ContentLocatorGroup; if (ContentLocatorGroup != null) { foreach(ContentLocator list in ContentLocatorGroup.Locators) { if (list.StartsWith(anchorLocator)) { matchesQuery = true; break; } } } } if (matchesQuery) { annotations.Add(annotation.Id, annotation); break; } } if (matchesQuery) break; } } return annotations; } /// /// Returns a dictionary of all annotations in the map /// ///annotations list. Can return an empty list, but never null. public DictionaryFindAnnotations() { Dictionary annotations = new Dictionary (); foreach (KeyValuePair annotKV in _currentAnnotations) { annotations.Add(annotKV.Key, annotKV.Value.Annotation); } return annotations; } /// /// Find an annottaion that has a specific id in the map /// returns null if not found /// /// id of the annotation to find public Annotation FindAnnotation(Guid id) { CachedAnnotation cachedAnnotation = null; if (_currentAnnotations.TryGetValue(id, out cachedAnnotation)) { return cachedAnnotation.Annotation; } return null; } ////// Returns a list of all dirty annotations in the map /// ///annotations list. Can return an empty list, but never null. public ListFindDirtyAnnotations() { List annotations = new List (); foreach (KeyValuePair annotKV in _currentAnnotations) { if (annotKV.Value.Dirty) { annotations.Add(annotKV.Value.Annotation); } } return annotations; } /// /// Sets all the dirty annotations in the map to clean /// public void ValidateDirtyAnnotations() { foreach (KeyValuePairannotKV in _currentAnnotations) { if (annotKV.Value.Dirty) { annotKV.Value.Dirty = false; } } } #endregion Public Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// the event handler for AnchorChanged Annotation event /// internally it calls the store AnnotationModified delegate /// /// annotation that changed /// args describing the change private void OnAnchorChanged(object sender, AnnotationResourceChangedEventArgs args) { _currentAnnotations[args.Annotation.Id].Dirty = true; _anchorChanged(sender, args); } ////// the event handler for CargoChanged Annotation event /// internally it calls the store AnnotationModified delegate /// /// annotation that changed /// args describing the change private void OnCargoChanged(object sender, AnnotationResourceChangedEventArgs args) { _currentAnnotations[args.Annotation.Id].Dirty = true; _cargoChanged(sender, args); } ////// the event handler for AuthorChanged Annotation event /// internally it calls the store AnnotationModified delegate /// /// annotation that changed /// args describing the change private void OnAuthorChanged(object sender, AnnotationAuthorChangedEventArgs args) { _currentAnnotations[args.Annotation.Id].Dirty = true; _authorChanged(sender, args); } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields // a dictionary that holds an annotation id to an annotation instance private Dictionary_currentAnnotations = new Dictionary (); // The delegates to be notified for Annotation changes private AnnotationAuthorChangedEventHandler _authorChanged; private AnnotationResourceChangedEventHandler _anchorChanged; private AnnotationResourceChangedEventHandler _cargoChanged; #endregion Private Fields //----------------------------------------------------- // // Private Classes // //----------------------------------------------------- #region Private Classes /// /// This class contains an annotation and a dirty flag /// It is needed because when it is time to flush /// the store - we should write only dirty annotations /// private class CachedAnnotation { ////// Construct a CachedAnnotation /// /// The annotation instance to be cached /// A flag to indicate if the annotation is dirty public CachedAnnotation(Annotation annotation, bool dirty) { Annotation = annotation; Dirty = dirty; } ////// The cached Annotation instance /// ///public Annotation Annotation { get { return _annotation; } set { _annotation = value; } } /// /// A flag to indicate if the cached annotation is dirty or not /// ///public bool Dirty { get { return _dirty; } set { _dirty = value; } } private Annotation _annotation; private bool _dirty; } #endregion Private Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
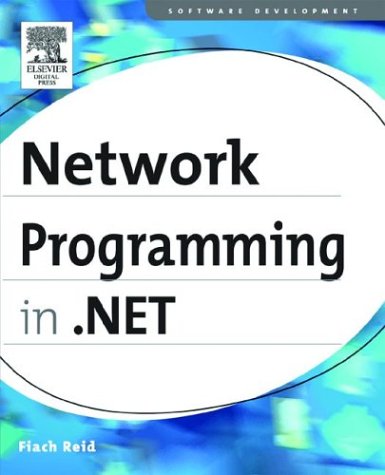
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MessageQueueTransaction.cs
- TableItemPattern.cs
- EFDataModelProvider.cs
- ExtensionQuery.cs
- XamlVector3DCollectionSerializer.cs
- CompilerGlobalScopeAttribute.cs
- dataSvcMapFileLoader.cs
- DateTimeFormat.cs
- NavigationWindowAutomationPeer.cs
- SessionEndedEventArgs.cs
- PersonalizationProvider.cs
- XmlSchemaChoice.cs
- SocketElement.cs
- BooleanStorage.cs
- WebPageTraceListener.cs
- FaultDescription.cs
- EffectiveValueEntry.cs
- Substitution.cs
- ObjectView.cs
- CfgSemanticTag.cs
- DecimalSumAggregationOperator.cs
- ApplicationServiceHelper.cs
- ContextDataSourceView.cs
- TextWriterTraceListener.cs
- AttributeEmitter.cs
- XpsSerializationManagerAsync.cs
- ArrayEditor.cs
- FrameworkElement.cs
- DateTimeSerializationSection.cs
- EndOfStreamException.cs
- MemberHolder.cs
- ScrollItemPatternIdentifiers.cs
- TextContainerHelper.cs
- Walker.cs
- HtmlTable.cs
- Directory.cs
- XmlWriterSettings.cs
- DesignTimeTemplateParser.cs
- ConfigurationValues.cs
- WebPartChrome.cs
- SafeTimerHandle.cs
- TaskHelper.cs
- FixedSOMGroup.cs
- TreeBuilderBamlTranslator.cs
- TagPrefixInfo.cs
- HtmlInputReset.cs
- AccessibilityApplicationManager.cs
- FormsAuthenticationTicket.cs
- CatalogZone.cs
- PathSegmentCollection.cs
- WindowsIdentity.cs
- LOSFormatter.cs
- ContractMapping.cs
- ProfileGroupSettingsCollection.cs
- SqlBuffer.cs
- PreviewKeyDownEventArgs.cs
- BaseDataBoundControlDesigner.cs
- TextBlock.cs
- DataGridToolTip.cs
- SiteMapNodeItem.cs
- HttpCapabilitiesEvaluator.cs
- FontConverter.cs
- FormsAuthenticationEventArgs.cs
- OutputScopeManager.cs
- ValidationRule.cs
- SqlDataSourceFilteringEventArgs.cs
- IsolatedStorageFilePermission.cs
- OleCmdHelper.cs
- GenericWebPart.cs
- RuntimeHelpers.cs
- ScriptingRoleServiceSection.cs
- HttpPostedFile.cs
- CompilerGlobalScopeAttribute.cs
- AddressHeader.cs
- TextServicesDisplayAttributePropertyRanges.cs
- BrowserCapabilitiesCodeGenerator.cs
- ApplySecurityAndSendAsyncResult.cs
- Rect.cs
- FormsAuthenticationCredentials.cs
- Canvas.cs
- BamlRecordHelper.cs
- ToolBarDesigner.cs
- ProvidersHelper.cs
- IntMinMaxAggregationOperator.cs
- ViewStateException.cs
- LogicalExpr.cs
- securestring.cs
- exports.cs
- _HeaderInfoTable.cs
- UserControlBuildProvider.cs
- PropertyChangeTracker.cs
- OdbcConnectionStringbuilder.cs
- InkCanvasFeedbackAdorner.cs
- EventProvider.cs
- ChannelAcceptor.cs
- PropertyTab.cs
- CroppedBitmap.cs
- ObjectNavigationPropertyMapping.cs
- Clipboard.cs
- ControlCachePolicy.cs