Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / CalendarButton.cs / 1305600 / CalendarButton.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.Windows.Data; namespace System.Windows.Controls.Primitives { ////// Represents a button control used in Calendar Control, which reacts to the Click event. /// public sealed class CalendarButton : Button { ////// Static constructor /// static CalendarButton() { DefaultStyleKeyProperty.OverrideMetadata(typeof(CalendarButton), new FrameworkPropertyMetadata(typeof(CalendarButton))); } ////// Represents the CalendarButton that is used in Calendar Control. /// public CalendarButton() : base() { } #region Public Properties #region HasSelectedDays internal static readonly DependencyPropertyKey HasSelectedDaysPropertyKey = DependencyProperty.RegisterReadOnly( "HasSelectedDays", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for HasSelectedDays property /// public static readonly DependencyProperty HasSelectedDaysProperty = HasSelectedDaysPropertyKey.DependencyProperty; ////// True if the CalendarButton represents a date range containing the display date /// public bool HasSelectedDays { get { return (bool)GetValue(HasSelectedDaysProperty); } internal set { SetValue(HasSelectedDaysPropertyKey, value); } } #endregion HasSelectedDays #region IsInactive internal static readonly DependencyPropertyKey IsInactivePropertyKey = DependencyProperty.RegisterReadOnly( "IsInactive", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsInactive property /// public static readonly DependencyProperty IsInactiveProperty = IsInactivePropertyKey.DependencyProperty; ////// True if the CalendarButton represents /// a month that falls outside the current year /// or /// a year that falls outside the current decade /// public bool IsInactive { get { return (bool)GetValue(IsInactiveProperty); } internal set { SetValue(IsInactivePropertyKey, value); } } #endregion IsInactive #endregion Public Properties #region Internal Properties internal Calendar Owner { get; set; } #endregion Internal Properties #region Public Methods #endregion Public Methods #region Protected Methods ////// Change to the correct visual state for the button. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal override void ChangeVisualState(bool useTransitions) { // Update the SelectionStates group if (HasSelectedDays) { VisualStates.GoToState(this, useTransitions, VisualStates.StateSelected, VisualStates.StateUnselected); } else { VisualStateManager.GoToState(this, VisualStates.StateUnselected, useTransitions); } // Update the ActiveStates group if (!IsInactive) { VisualStates.GoToState(this, useTransitions, VisualStates.StateActive, VisualStates.StateInactive); } else { VisualStateManager.GoToState(this, VisualStates.StateInactive, useTransitions); } // Update the FocusStates group if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateCalendarButtonFocused, VisualStates.StateCalendarButtonUnfocused); } else { VisualStateManager.GoToState(this, VisualStates.StateCalendarButtonUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } ////// Creates the automation peer for the DayButton. /// ///protected override AutomationPeer OnCreateAutomationPeer() { return new CalendarButtonAutomationPeer(this); } #endregion Protected Methods #region Internal Methods internal void SetContentInternal(string value) { SetCurrentValueInternal(ContentControl.ContentProperty, value); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.Windows.Data; namespace System.Windows.Controls.Primitives { /// /// Represents a button control used in Calendar Control, which reacts to the Click event. /// public sealed class CalendarButton : Button { ////// Static constructor /// static CalendarButton() { DefaultStyleKeyProperty.OverrideMetadata(typeof(CalendarButton), new FrameworkPropertyMetadata(typeof(CalendarButton))); } ////// Represents the CalendarButton that is used in Calendar Control. /// public CalendarButton() : base() { } #region Public Properties #region HasSelectedDays internal static readonly DependencyPropertyKey HasSelectedDaysPropertyKey = DependencyProperty.RegisterReadOnly( "HasSelectedDays", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for HasSelectedDays property /// public static readonly DependencyProperty HasSelectedDaysProperty = HasSelectedDaysPropertyKey.DependencyProperty; ////// True if the CalendarButton represents a date range containing the display date /// public bool HasSelectedDays { get { return (bool)GetValue(HasSelectedDaysProperty); } internal set { SetValue(HasSelectedDaysPropertyKey, value); } } #endregion HasSelectedDays #region IsInactive internal static readonly DependencyPropertyKey IsInactivePropertyKey = DependencyProperty.RegisterReadOnly( "IsInactive", typeof(bool), typeof(CalendarButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsInactive property /// public static readonly DependencyProperty IsInactiveProperty = IsInactivePropertyKey.DependencyProperty; ////// True if the CalendarButton represents /// a month that falls outside the current year /// or /// a year that falls outside the current decade /// public bool IsInactive { get { return (bool)GetValue(IsInactiveProperty); } internal set { SetValue(IsInactivePropertyKey, value); } } #endregion IsInactive #endregion Public Properties #region Internal Properties internal Calendar Owner { get; set; } #endregion Internal Properties #region Public Methods #endregion Public Methods #region Protected Methods ////// Change to the correct visual state for the button. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal override void ChangeVisualState(bool useTransitions) { // Update the SelectionStates group if (HasSelectedDays) { VisualStates.GoToState(this, useTransitions, VisualStates.StateSelected, VisualStates.StateUnselected); } else { VisualStateManager.GoToState(this, VisualStates.StateUnselected, useTransitions); } // Update the ActiveStates group if (!IsInactive) { VisualStates.GoToState(this, useTransitions, VisualStates.StateActive, VisualStates.StateInactive); } else { VisualStateManager.GoToState(this, VisualStates.StateInactive, useTransitions); } // Update the FocusStates group if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateCalendarButtonFocused, VisualStates.StateCalendarButtonUnfocused); } else { VisualStateManager.GoToState(this, VisualStates.StateCalendarButtonUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } ////// Creates the automation peer for the DayButton. /// ///protected override AutomationPeer OnCreateAutomationPeer() { return new CalendarButtonAutomationPeer(this); } #endregion Protected Methods #region Internal Methods internal void SetContentInternal(string value) { SetCurrentValueInternal(ContentControl.ContentProperty, value); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
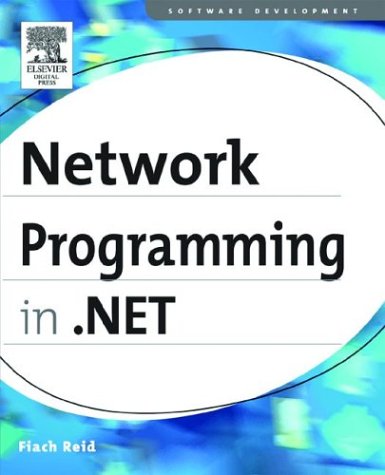
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientApiGenerator.cs
- Vector3DKeyFrameCollection.cs
- GlyphRunDrawing.cs
- ApplicationSecurityManager.cs
- ContextMenuAutomationPeer.cs
- IRCollection.cs
- MappingException.cs
- rsa.cs
- CategoryAttribute.cs
- FormViewPagerRow.cs
- Logging.cs
- ParameterRetriever.cs
- Point3DAnimationBase.cs
- HitTestFilterBehavior.cs
- RegexGroupCollection.cs
- SystemIPInterfaceStatistics.cs
- Nodes.cs
- dtdvalidator.cs
- StrongNameKeyPair.cs
- DirectoryNotFoundException.cs
- ErrorStyle.cs
- ResXBuildProvider.cs
- SamlAuthenticationClaimResource.cs
- PathGeometry.cs
- UshortList2.cs
- Adorner.cs
- RoutedEventArgs.cs
- ToolStripArrowRenderEventArgs.cs
- FieldNameLookup.cs
- BindableTemplateBuilder.cs
- Win32PrintDialog.cs
- EventPrivateKey.cs
- SafeEventHandle.cs
- InputReport.cs
- ConnectionStringsExpressionBuilder.cs
- Int32AnimationUsingKeyFrames.cs
- UnsafeNativeMethodsPenimc.cs
- WindowsAuthenticationModule.cs
- ProfileEventArgs.cs
- VisualBrush.cs
- PropertyEntry.cs
- GridLength.cs
- RIPEMD160.cs
- Highlights.cs
- BaseAppDomainProtocolHandler.cs
- ClientBuildManagerCallback.cs
- ImportCatalogPart.cs
- XmlValidatingReaderImpl.cs
- XmlSerializer.cs
- AppDomainFactory.cs
- ValidatingPropertiesEventArgs.cs
- _UncName.cs
- TypedDatasetGenerator.cs
- PlaceHolder.cs
- Listbox.cs
- XmlBoundElement.cs
- XmlSchema.cs
- PartialCachingAttribute.cs
- DataBindingCollection.cs
- ThrowHelper.cs
- PropertyPushdownHelper.cs
- SessionPageStatePersister.cs
- UpdatePanelTriggerCollection.cs
- ApplicationHost.cs
- SqlFunctionAttribute.cs
- OptionalMessageQuery.cs
- SiteMapNodeItemEventArgs.cs
- Visual3D.cs
- ParameterToken.cs
- Task.cs
- ExceptionUtil.cs
- DesignerTextWriter.cs
- DataSourceCache.cs
- PrinterResolution.cs
- FileSystemEnumerable.cs
- BuildDependencySet.cs
- COM2ComponentEditor.cs
- DiscoveryDocumentSearchPattern.cs
- WinFormsUtils.cs
- LocalizationComments.cs
- LocatorManager.cs
- ConnectorSelectionGlyph.cs
- Localizer.cs
- UndirectedGraph.cs
- XmlSubtreeReader.cs
- DbConnectionOptions.cs
- MatchAttribute.cs
- SqlDependencyListener.cs
- StateWorkerRequest.cs
- xmlglyphRunInfo.cs
- ErrorWebPart.cs
- DataFormat.cs
- Tuple.cs
- smtppermission.cs
- PolyBezierSegment.cs
- AttachedAnnotation.cs
- sqlmetadatafactory.cs
- BooleanFunctions.cs
- DataControlField.cs
- TemplateParser.cs