Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / InternalTrees / Nodes.cs / 1305376 / Nodes.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Globalization; using System.Diagnostics; namespace System.Data.Query.InternalTrees { ////// A Node describes a node in a query tree. Each node has an operator, and /// a list of zero or more children of that operator. /// internal class Node { #region private state private int m_id; private Listm_children; private Op m_op; private NodeInfo m_nodeInfo; #endregion #region constructors /// /// Basic constructor. /// /// NEVER call this routine directly - you should always use the Command.CreateNode /// factory methods. /// /// id for the node /// The operator /// List of child nodes internal Node(int nodeId, Op op, Listchildren) { m_id = nodeId; m_op = op; m_children = children; } /// /// This routine is only used for building up rule patterns. /// NEVER use this routine for building up nodes in a user command tree. /// /// /// internal Node(Op op, params Node[] children) : this(-1, op, new List(children)) { } #endregion #region public properties and methods #if DEBUG internal int Id { get { return m_id; } } #endif /// /// Get the list of children /// internal ListChildren { get { return m_children; } } /// /// Gets or sets the node's operator /// internal Op Op { get { return m_op; } set { m_op = value; } } ////// Simpler (?) getter/setter routines /// internal Node Child0 { get { return m_children[0]; } set { m_children[0] = value; } } ////// Do I have a zeroth child? /// internal bool HasChild0 { get { return m_children.Count > 0; } } ////// Get/set first child /// internal Node Child1 { get { return m_children[1]; } set { m_children[1] = value; } } ////// Do I have a child1? /// internal bool HasChild1 { get { return m_children.Count > 1; } } ////// get/set second child /// internal Node Child2 { get { return m_children[2]; } set { m_children[2] = value; } } ////// get/set second child /// internal Node Child3 { get { return m_children[3]; } /* commented out because of fxcop - there are no upstream callers -- set { m_children[3] = value; }*/ } ////// Do I have a child2 (third child really) /// internal bool HasChild2 { get { return m_children.Count > 2; } } ////// Do I have a child3 (fourth child really) /// internal bool HasChild3 { get { return m_children.Count > 3; } } #region equivalence functions ////// Is this subtree equivalent to another subtree /// /// ///internal bool IsEquivalent(Node other) { if (this.Children.Count != other.Children.Count) { return false; } bool? opEquivalent = this.Op.IsEquivalent(other.Op); if (opEquivalent != true) { return false; } for (int i = 0; i < this.Children.Count; i++) { if (!this.Children[i].IsEquivalent(other.Children[i])) { return false; } } return true; } #endregion #region NodeInfo methods and properties /// /// Has the node info been initialized, i.e. previously computed /// internal bool IsNodeInfoInitialized { get { return (m_nodeInfo != null); } } ////// Get the nodeInfo for a node. Initializes it, if it has not yet been initialized /// /// Current command object ///NodeInfo for this node internal NodeInfo GetNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } return m_nodeInfo; } ////// Gets the "extended" nodeinfo for a node; if it has not yet been initialized, then it will be /// /// current command object ///extended nodeinfo for this node internal ExtendedNodeInfo GetExtendedNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } ExtendedNodeInfo extendedNodeInfo = m_nodeInfo as ExtendedNodeInfo; Debug.Assert(extendedNodeInfo != null); return extendedNodeInfo; } private void InitializeNodeInfo(Command command) { if (this.Op.IsRelOp || this.Op.IsPhysicalOp) { m_nodeInfo = new ExtendedNodeInfo(command); } else { m_nodeInfo = new NodeInfo(command); } command.RecomputeNodeInfo(this); } #endregion #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Globalization; using System.Diagnostics; namespace System.Data.Query.InternalTrees { ////// A Node describes a node in a query tree. Each node has an operator, and /// a list of zero or more children of that operator. /// internal class Node { #region private state private int m_id; private Listm_children; private Op m_op; private NodeInfo m_nodeInfo; #endregion #region constructors /// /// Basic constructor. /// /// NEVER call this routine directly - you should always use the Command.CreateNode /// factory methods. /// /// id for the node /// The operator /// List of child nodes internal Node(int nodeId, Op op, Listchildren) { m_id = nodeId; m_op = op; m_children = children; } /// /// This routine is only used for building up rule patterns. /// NEVER use this routine for building up nodes in a user command tree. /// /// /// internal Node(Op op, params Node[] children) : this(-1, op, new List(children)) { } #endregion #region public properties and methods #if DEBUG internal int Id { get { return m_id; } } #endif /// /// Get the list of children /// internal ListChildren { get { return m_children; } } /// /// Gets or sets the node's operator /// internal Op Op { get { return m_op; } set { m_op = value; } } ////// Simpler (?) getter/setter routines /// internal Node Child0 { get { return m_children[0]; } set { m_children[0] = value; } } ////// Do I have a zeroth child? /// internal bool HasChild0 { get { return m_children.Count > 0; } } ////// Get/set first child /// internal Node Child1 { get { return m_children[1]; } set { m_children[1] = value; } } ////// Do I have a child1? /// internal bool HasChild1 { get { return m_children.Count > 1; } } ////// get/set second child /// internal Node Child2 { get { return m_children[2]; } set { m_children[2] = value; } } ////// get/set second child /// internal Node Child3 { get { return m_children[3]; } /* commented out because of fxcop - there are no upstream callers -- set { m_children[3] = value; }*/ } ////// Do I have a child2 (third child really) /// internal bool HasChild2 { get { return m_children.Count > 2; } } ////// Do I have a child3 (fourth child really) /// internal bool HasChild3 { get { return m_children.Count > 3; } } #region equivalence functions ////// Is this subtree equivalent to another subtree /// /// ///internal bool IsEquivalent(Node other) { if (this.Children.Count != other.Children.Count) { return false; } bool? opEquivalent = this.Op.IsEquivalent(other.Op); if (opEquivalent != true) { return false; } for (int i = 0; i < this.Children.Count; i++) { if (!this.Children[i].IsEquivalent(other.Children[i])) { return false; } } return true; } #endregion #region NodeInfo methods and properties /// /// Has the node info been initialized, i.e. previously computed /// internal bool IsNodeInfoInitialized { get { return (m_nodeInfo != null); } } ////// Get the nodeInfo for a node. Initializes it, if it has not yet been initialized /// /// Current command object ///NodeInfo for this node internal NodeInfo GetNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } return m_nodeInfo; } ////// Gets the "extended" nodeinfo for a node; if it has not yet been initialized, then it will be /// /// current command object ///extended nodeinfo for this node internal ExtendedNodeInfo GetExtendedNodeInfo(Command command) { if (m_nodeInfo == null) { InitializeNodeInfo(command); } ExtendedNodeInfo extendedNodeInfo = m_nodeInfo as ExtendedNodeInfo; Debug.Assert(extendedNodeInfo != null); return extendedNodeInfo; } private void InitializeNodeInfo(Command command) { if (this.Op.IsRelOp || this.Op.IsPhysicalOp) { m_nodeInfo = new ExtendedNodeInfo(command); } else { m_nodeInfo = new NodeInfo(command); } command.RecomputeNodeInfo(this); } #endregion #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
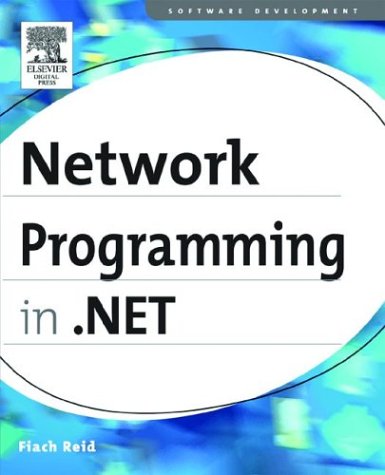
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinkButton.cs
- TextReader.cs
- ProxyElement.cs
- EntityModelBuildProvider.cs
- CodeTryCatchFinallyStatement.cs
- CorrelationManager.cs
- PageEventArgs.cs
- ActivityDesignerAccessibleObject.cs
- CheckBox.cs
- DecryptedHeader.cs
- Filter.cs
- FileCodeGroup.cs
- BaseCollection.cs
- BuildManager.cs
- WebPartPersonalization.cs
- util.cs
- SqlClientMetaDataCollectionNames.cs
- DirectoryNotFoundException.cs
- GroupBoxRenderer.cs
- SharedPersonalizationStateInfo.cs
- VectorAnimation.cs
- NativeObjectSecurity.cs
- TagMapInfo.cs
- XPathNode.cs
- WebConfigurationManager.cs
- GenerateTemporaryTargetAssembly.cs
- ImpersonateTokenRef.cs
- TemplateInstanceAttribute.cs
- processwaithandle.cs
- VisualStyleTypesAndProperties.cs
- SqlDataAdapter.cs
- TriggerBase.cs
- LineGeometry.cs
- DetailsViewDeletedEventArgs.cs
- BooleanProjectedSlot.cs
- VisualBrush.cs
- RMPublishingDialog.cs
- ServiceDurableInstanceContextProvider.cs
- BlobPersonalizationState.cs
- NativeCompoundFileAPIs.cs
- OdbcConnection.cs
- FacetEnabledSchemaElement.cs
- NativeMethodsCLR.cs
- NaturalLanguageHyphenator.cs
- BitmapEffectInput.cs
- ToolBar.cs
- ComplexBindingPropertiesAttribute.cs
- UInt64Storage.cs
- UInt32Converter.cs
- XamlToRtfWriter.cs
- SchemaObjectWriter.cs
- Transform.cs
- SafeArrayTypeMismatchException.cs
- MultipartIdentifier.cs
- RawMouseInputReport.cs
- AssociationType.cs
- ImmutableObjectAttribute.cs
- CompilerState.cs
- MSG.cs
- HttpListenerElement.cs
- OrderedHashRepartitionEnumerator.cs
- Misc.cs
- URIFormatException.cs
- ClipboardData.cs
- AdjustableArrowCap.cs
- Handle.cs
- MissingSatelliteAssemblyException.cs
- ImageListUtils.cs
- SafeNativeMethodsMilCoreApi.cs
- PackWebRequest.cs
- EasingKeyFrames.cs
- UnsafeNativeMethods.cs
- ExternalException.cs
- ModulesEntry.cs
- TransformConverter.cs
- MembershipSection.cs
- ProtocolsSection.cs
- ContentTextAutomationPeer.cs
- QilGeneratorEnv.cs
- SoapIgnoreAttribute.cs
- Constants.cs
- XmlNamespaceManager.cs
- WsdlBuildProvider.cs
- ToolZone.cs
- ProcessHostFactoryHelper.cs
- RowCache.cs
- ADMembershipProvider.cs
- QuaternionRotation3D.cs
- KeysConverter.cs
- WebPart.cs
- ResourceAssociationTypeEnd.cs
- UInt16.cs
- RetrieveVirtualItemEventArgs.cs
- ChangeTracker.cs
- ScalarType.cs
- CompiledXpathExpr.cs
- XmlName.cs
- SingleAnimationBase.cs
- QilVisitor.cs
- GZipStream.cs