Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Input / InputLanguageSource.cs / 1 / InputLanguageSource.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The source of the input language of the thread. // // History: // 07/30/2003 : yutakas - ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Security; using System.Security.Permissions; using System.Collections; using System.Globalization; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Media; using System.Runtime.InteropServices; using System.Diagnostics; using MS.Win32; using MS.Utility; namespace System.Windows.Input { //----------------------------------------------------- // // InputLanguageSource class // //----------------------------------------------------- ////// This is an internal. The source for input languages. /// internal sealed class InputLanguageSource : IInputLanguageSource, IDisposable { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// This is an internal. The source for input languages. /// internal InputLanguageSource(InputLanguageManager inputlanguagemanager) { _inputlanguagemanager = inputlanguagemanager; // initialize the current input language. _langid = (short)NativeMethods.IntPtrToInt32(SafeNativeMethods.GetKeyboardLayout(0)); // store the dispatcher thread id. This will be used to call GetKeyboardLayout() from // other thread. _dispatcherThreadId = SafeNativeMethods.GetCurrentThreadId(); // Register source _inputlanguagemanager.RegisterInputLanguageSource(this); } //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Dispose method. /// public void Dispose() { if (_ipp != null) Uninitialize(); } ////// IIInputLanguageSource.Initialize() /// This creates ITfInputProcessorProfile object and advice sink. /// public void Initialize() { EnsureInputProcessorProfile(); } ////// IIInputLanguageSource.Uninitialize() /// This releases ITfInputProcessorProfile object and unadvice sink. /// public void Uninitialize() { if (_ipp != null) { _ipp.Uninitialize(); _ipp = null; } } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ ////// returns the current input language of this win32 thread. /// public CultureInfo CurrentInputLanguage { get { return new CultureInfo(_CurrentInputLanguage); } set { _CurrentInputLanguage = (short)value.LCID; } } ////// returns the list of the available input languages of this win32 thread. /// public IEnumerable InputLanguageList { get { EnsureInputProcessorProfile(); if (_ipp == null) { ArrayList al = new ArrayList(); al.Add(CurrentInputLanguage); return al; } return _ipp.InputLanguageList; } } //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- ////// The input language change call back from the sink. /// internal bool OnLanguageChange(short langid) { if (_langid != langid) { // Call InputLanguageManager if its current source is this. if (InputLanguageManager.Current.Source == this) { return InputLanguageManager.Current.ReportInputLanguageChanging(new CultureInfo(langid), new CultureInfo(_langid)); } } return true; } ////// The input language changed call back from the sink. /// internal void OnLanguageChanged() { short langid = _CurrentInputLanguage; if (_langid != langid) { short prevlangid = _langid; _langid = langid; // Call InputLanguageManager if its current source is this. if (InputLanguageManager.Current.Source == this) { InputLanguageManager.Current.ReportInputLanguageChanged(new CultureInfo(langid), new CultureInfo(prevlangid)); } } } //----------------------------------------------------- // // Private Method // //------------------------------------------------------ ////// This creates ITfInputProcessorProfile object and advice sink. /// ////// Critical - calls unmanaged code (initializing input) /// TreatAsSafe - ok to call any number of times /// [SecurityCritical, SecurityTreatAsSafe] private void EnsureInputProcessorProfile() { // _ipp has been initialzied. Don't do this again. if (_ipp != null) return; // We don't need to initialize _ipp if there is onlyone keyboard layout. // Only one input language is available. if (SafeNativeMethods.GetKeyboardLayoutList(0, null) <= 1) return; Debug.Assert(_ipp == null, "_EnsureInputProcesoorProfile has been called."); InputLanguageProfileNotifySink lpns; lpns = new InputLanguageProfileNotifySink(this); _ipp= new InputProcessorProfiles(); if (!_ipp.Initialize(lpns)) { _ipp = null; } } //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ ////// The current input language in LANGID of this win32 thread. /// private short _CurrentInputLanguage { get { // Return input language of the dispatcher thread. return (short)NativeMethods.IntPtrToInt32(SafeNativeMethods.GetKeyboardLayout(_dispatcherThreadId)); } set { EnsureInputProcessorProfile(); if (_ipp != null) { _ipp.CurrentInputLanguage = value; } } } //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // the current input language in LANGID. private short _langid; // The dispatcher thread id. private int _dispatcherThreadId; // the connected input language manager. InputLanguageManager _inputlanguagemanager; // the reference to ITfInputProcessorProfile. InputProcessorProfiles _ipp; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The source of the input language of the thread. // // History: // 07/30/2003 : yutakas - ported from dotnet tree. // //--------------------------------------------------------------------------- using System.Security; using System.Security.Permissions; using System.Collections; using System.Globalization; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Media; using System.Runtime.InteropServices; using System.Diagnostics; using MS.Win32; using MS.Utility; namespace System.Windows.Input { //----------------------------------------------------- // // InputLanguageSource class // //----------------------------------------------------- ////// This is an internal. The source for input languages. /// internal sealed class InputLanguageSource : IInputLanguageSource, IDisposable { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// This is an internal. The source for input languages. /// internal InputLanguageSource(InputLanguageManager inputlanguagemanager) { _inputlanguagemanager = inputlanguagemanager; // initialize the current input language. _langid = (short)NativeMethods.IntPtrToInt32(SafeNativeMethods.GetKeyboardLayout(0)); // store the dispatcher thread id. This will be used to call GetKeyboardLayout() from // other thread. _dispatcherThreadId = SafeNativeMethods.GetCurrentThreadId(); // Register source _inputlanguagemanager.RegisterInputLanguageSource(this); } //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Dispose method. /// public void Dispose() { if (_ipp != null) Uninitialize(); } ////// IIInputLanguageSource.Initialize() /// This creates ITfInputProcessorProfile object and advice sink. /// public void Initialize() { EnsureInputProcessorProfile(); } ////// IIInputLanguageSource.Uninitialize() /// This releases ITfInputProcessorProfile object and unadvice sink. /// public void Uninitialize() { if (_ipp != null) { _ipp.Uninitialize(); _ipp = null; } } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ ////// returns the current input language of this win32 thread. /// public CultureInfo CurrentInputLanguage { get { return new CultureInfo(_CurrentInputLanguage); } set { _CurrentInputLanguage = (short)value.LCID; } } ////// returns the list of the available input languages of this win32 thread. /// public IEnumerable InputLanguageList { get { EnsureInputProcessorProfile(); if (_ipp == null) { ArrayList al = new ArrayList(); al.Add(CurrentInputLanguage); return al; } return _ipp.InputLanguageList; } } //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- ////// The input language change call back from the sink. /// internal bool OnLanguageChange(short langid) { if (_langid != langid) { // Call InputLanguageManager if its current source is this. if (InputLanguageManager.Current.Source == this) { return InputLanguageManager.Current.ReportInputLanguageChanging(new CultureInfo(langid), new CultureInfo(_langid)); } } return true; } ////// The input language changed call back from the sink. /// internal void OnLanguageChanged() { short langid = _CurrentInputLanguage; if (_langid != langid) { short prevlangid = _langid; _langid = langid; // Call InputLanguageManager if its current source is this. if (InputLanguageManager.Current.Source == this) { InputLanguageManager.Current.ReportInputLanguageChanged(new CultureInfo(langid), new CultureInfo(prevlangid)); } } } //----------------------------------------------------- // // Private Method // //------------------------------------------------------ ////// This creates ITfInputProcessorProfile object and advice sink. /// ////// Critical - calls unmanaged code (initializing input) /// TreatAsSafe - ok to call any number of times /// [SecurityCritical, SecurityTreatAsSafe] private void EnsureInputProcessorProfile() { // _ipp has been initialzied. Don't do this again. if (_ipp != null) return; // We don't need to initialize _ipp if there is onlyone keyboard layout. // Only one input language is available. if (SafeNativeMethods.GetKeyboardLayoutList(0, null) <= 1) return; Debug.Assert(_ipp == null, "_EnsureInputProcesoorProfile has been called."); InputLanguageProfileNotifySink lpns; lpns = new InputLanguageProfileNotifySink(this); _ipp= new InputProcessorProfiles(); if (!_ipp.Initialize(lpns)) { _ipp = null; } } //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ ////// The current input language in LANGID of this win32 thread. /// private short _CurrentInputLanguage { get { // Return input language of the dispatcher thread. return (short)NativeMethods.IntPtrToInt32(SafeNativeMethods.GetKeyboardLayout(_dispatcherThreadId)); } set { EnsureInputProcessorProfile(); if (_ipp != null) { _ipp.CurrentInputLanguage = value; } } } //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields // the current input language in LANGID. private short _langid; // The dispatcher thread id. private int _dispatcherThreadId; // the connected input language manager. InputLanguageManager _inputlanguagemanager; // the reference to ITfInputProcessorProfile. InputProcessorProfiles _ipp; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
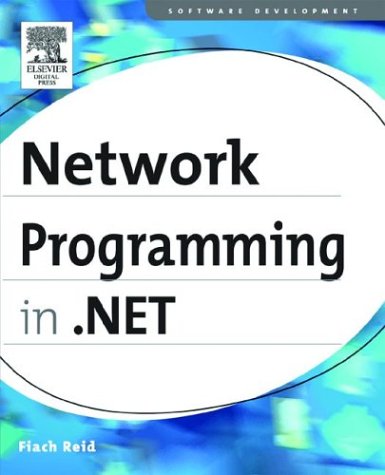
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BCryptHashAlgorithm.cs
- X509AsymmetricSecurityKey.cs
- SetterBase.cs
- DbConnectionStringCommon.cs
- ContentElement.cs
- XmlNotation.cs
- NativeConfigurationLoader.cs
- CodeFieldReferenceExpression.cs
- RelationshipNavigation.cs
- DetailsView.cs
- odbcmetadatacollectionnames.cs
- ProviderMetadataCachedInformation.cs
- GeometryDrawing.cs
- Module.cs
- WmlTextViewAdapter.cs
- ConstructorExpr.cs
- OutputWindow.cs
- Int64.cs
- InstanceStore.cs
- RegexCapture.cs
- SiteMapNodeItemEventArgs.cs
- WrappedIUnknown.cs
- ItemCollection.cs
- CodeMethodReturnStatement.cs
- WS2007HttpBinding.cs
- OdbcDataReader.cs
- UndoManager.cs
- NativeMethods.cs
- DbProviderSpecificTypePropertyAttribute.cs
- TreeViewImageKeyConverter.cs
- XmlBinaryReaderSession.cs
- SafeProcessHandle.cs
- FixedHyperLink.cs
- NetCodeGroup.cs
- ColorKeyFrameCollection.cs
- SubMenuStyle.cs
- LinkedDataMemberFieldEditor.cs
- SiteMapNodeCollection.cs
- NumberFunctions.cs
- PriorityQueue.cs
- SessionPageStateSection.cs
- PageAsyncTaskManager.cs
- SettingsProperty.cs
- DateTimeValueSerializer.cs
- SamlAuthenticationClaimResource.cs
- ConfigurationStrings.cs
- ColumnMapProcessor.cs
- NetworkInterface.cs
- BaseProcessor.cs
- OdbcUtils.cs
- HandlerMappingMemo.cs
- UriExt.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- SwitchElementsCollection.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- PrintDialogException.cs
- EdmProperty.cs
- PrivateFontCollection.cs
- FileLevelControlBuilderAttribute.cs
- ReadOnlyCollectionBuilder.cs
- Helper.cs
- SmiMetaData.cs
- FileDialog.cs
- XmlDesigner.cs
- WebPartConnectionsDisconnectVerb.cs
- BitmapVisualManager.cs
- DesignerVerbCollection.cs
- KoreanCalendar.cs
- CachedCompositeFamily.cs
- HtmlInputRadioButton.cs
- WebPartMovingEventArgs.cs
- MouseActionValueSerializer.cs
- HandlerBase.cs
- Documentation.cs
- Span.cs
- InfoCardProofToken.cs
- AssemblyAttributes.cs
- DrawingGroup.cs
- SendMessageRecord.cs
- _NetworkingPerfCounters.cs
- SymLanguageVendor.cs
- ListDictionary.cs
- Ipv6Element.cs
- IOException.cs
- DefaultValueConverter.cs
- METAHEADER.cs
- CellQuery.cs
- odbcmetadatacollectionnames.cs
- IResourceProvider.cs
- ControlBindingsCollection.cs
- ReceiveActivity.cs
- DateTimeOffset.cs
- XmlDataLoader.cs
- KeySpline.cs
- XmlSchemaNotation.cs
- EncoderBestFitFallback.cs
- FixedLineResult.cs
- DivideByZeroException.cs
- HandleExceptionArgs.cs
- Missing.cs