Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / IO / Packaging / CompoundFile / FormatVersion.cs / 1 / FormatVersion.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of the FormatVersion class, which describes the versioning // of an individual "format feature" within a compound file. // // History: // 06/20/2002: YoungGK: Created // 05/30/2003: LGolding: Ported to WCP tree. // //----------------------------------------------------------------------------- // Allow use of presharp warning numbers [6506] and [6518] unknown to the compiler #pragma warning disable 1634, 1691 using System; using System.IO; #if PBTCOMPILER using MS.Utility; // For SR.cs #else using System.Windows; using MS.Internal.WindowsBase; // FriendAccessAllowed #endif namespace MS.Internal.IO.Packaging.CompoundFile { ///Class for manipulating version object #if !PBTCOMPILER [FriendAccessAllowed] #endif internal class FormatVersion { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Constructors #if !PBTCOMPILER ////// Constructor for FormatVersion /// private FormatVersion() { } #endif ////// Constructor for FormatVersion with given featureId and version /// /// feature identifier /// version ///reader, updater, and writer versions are set to version public FormatVersion(string featureId, VersionPair version) : this(featureId, version, version, version) { } ////// Constructor for FormatVersion with given featureId and reader, updater, /// and writer version /// /// feature identifier /// Writer Version /// Reader Version /// Updater Version public FormatVersion(String featureId, VersionPair writerVersion, VersionPair readerVersion, VersionPair updaterVersion) { if (featureId == null) throw new ArgumentNullException("featureId"); if (writerVersion == null) throw new ArgumentNullException("writerVersion"); if (readerVersion == null) throw new ArgumentNullException("readerVersion"); if (updaterVersion == null) throw new ArgumentNullException("updaterVersion"); if (featureId.Length == 0) { throw new ArgumentException(SR.Get(SRID.ZeroLengthFeatureID)); } _featureIdentifier = featureId; _reader = readerVersion; _updater = updaterVersion; _writer = writerVersion; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #if !PBTCOMPILER ////// reader version /// public VersionPair ReaderVersion { get { return _reader; } set { if (value == null) { throw new ArgumentNullException("value"); } _reader = value; } } ////// writer version /// public VersionPair WriterVersion { get { return _writer; } set { if (value == null) { throw new ArgumentNullException("value"); } _writer = value; } } ////// updater version /// public VersionPair UpdaterVersion { get { return _updater; } set { if (value == null) { throw new ArgumentNullException("value"); } _updater = value; } } ////// feature identifier /// public String FeatureIdentifier { get { return _featureIdentifier; } } #endif #endregion Public Properties #region Operators #if false ////// == comparison operator /// /// version to be compared /// version to be compared public static bool operator ==(FormatVersion v1, FormatVersion v2) { // We have to cast v1 and v2 to Object // to ensure that the == operator on Ojbect class is used not the == operator on FormatVersion if ((Object) v1 == null || (Object) v2 == null) { return ((Object) v1 == null && (Object) v2 == null); } // Do comparison only if both v1 and v2 are not null return v1.Equals(v2); } ////// != comparison operator /// /// version to be compared /// version to be compared public static bool operator !=(FormatVersion v1, FormatVersion v2) { return !(v1 == v2); } ////// Eaual comparison operator /// /// Object to compare ///ture if the object is equal to this instance public override bool Equals(Object obj) { if (obj == null) { return false; } if (obj.GetType() != typeof(FormatVersion)) { return false; } FormatVersion v = (FormatVersion) obj; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. // Parameter 'v' to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 if (String.CompareOrdinal(_featureIdentifier.ToUpperInvariant(), v.FeatureIdentifier.ToUpperInvariant()) != 0 || _reader != v.ReaderVersion || _writer != v.WriterVersion || _updater != v.UpdaterVersion) { return false; } #pragma warning restore 6506 return true; } ////// Hash code /// public override int GetHashCode() { int hash = _reader.Major & HashMask; hash <<= 5; hash |= (_reader.Minor & HashMask); hash <<= 5; hash |= (_updater.Major & HashMask); hash <<= 5; hash |= (_updater.Minor & HashMask); hash <<= 5; hash |= (_writer.Major & HashMask); hash <<= 5; hash |= (_writer.Minor & HashMask); return hash; } #endif #endregion Operators //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #if !PBTCOMPILER ////// Constructor for FormatVersion with information read from the given stream /// /// Stream where version information is read from ///Before this function call the current position of stream should be /// pointing to the begining of the version structure. After this call the current /// poisition will be pointing immediately after the version structure public static FormatVersion LoadFromStream(Stream stream) { int bytesRead; return LoadFromStream(stream, out bytesRead); } #endif ////// Persist format version to the given stream /// /// the stream to be written ////// This operation will change the stream pointer /// stream can be null and will still return the number of bytes to be written /// public int SaveToStream(Stream stream) { checked { // Suppress 56518 Local IDisposable object not disposed: // Reason: The stream is not owned by the BlockManager, therefore we can // close the BinaryWriter as it will Close the stream underneath. #pragma warning disable 6518 int len = 0; BinaryWriter binarywriter = null; #pragma warning restore 6518 if (stream != null) { binarywriter = new BinaryWriter(stream, System.Text.Encoding.Unicode); } // ************ // feature ID // ************ len += ContainerUtilities.WriteByteLengthPrefixedDWordPaddedUnicodeString(binarywriter, _featureIdentifier); // **************** // Reader Version // **************** if (stream != null) { binarywriter.Write(_reader.Major); // Major number binarywriter.Write(_reader.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; // ***************** // Updater Version // ***************** if (stream != null) { binarywriter.Write(_updater.Major); // Major number binarywriter.Write(_updater.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; // **************** // Writer Version // **************** if (stream != null) { binarywriter.Write(_writer.Major); // Major number binarywriter.Write(_writer.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; return len; } } #if !PBTCOMPILER ////// Check if a component with the given version can read this format version safely /// /// version of a component ///true if this format version can be read safely by the component /// with the given version, otherwise false ////// The given version is checked against ReaderVersion /// public bool IsReadableBy(VersionPair version) { if (version == null) { throw new ArgumentNullException("version"); } return (_reader <= version); } ////// Check if a component with the given version can update this format version safely /// /// version of a component ///true if this format version can be updated safely by the component /// with the given version, otherwise false ////// The given version is checked against UpdaterVersion /// public bool IsUpdatableBy(VersionPair version) { if (version == null) { throw new ArgumentNullException("version"); } return (_updater <= version); } #endif #endregion Public Methods //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #if !PBTCOMPILER ////// Constructor for FormatVersion with information read from the given BinaryReader /// /// BinaryReader where version information is read from /// number of bytes read including padding ///FormatVersion object ////// This operation will change the stream pointer. This function is preferred over the /// LoadFromStream as it doesn't leave around Undisposed BinaryReader, which /// LoadFromStream will /// private static FormatVersion LoadFromBinaryReader(BinaryReader reader, out Int32 bytesRead) { checked { if (reader == null) { throw new ArgumentNullException("reader"); } FormatVersion ver = new FormatVersion(); bytesRead = 0; // Initialize the number of bytes read // ************** // feature ID // ************** Int32 strBytes; ver._featureIdentifier = ContainerUtilities.ReadByteLengthPrefixedDWordPaddedUnicodeString(reader, out strBytes); bytesRead += strBytes; Int16 major; Int16 minor; // **************** // Reader Version // **************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.ReaderVersion = new VersionPair(major, minor); // ***************** // Updater Version // ***************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.UpdaterVersion = new VersionPair(major, minor); // **************** // Writer Version // **************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.WriterVersion = new VersionPair(major, minor); return ver; } } ////// Create FormatVersion object and read version information from the given stream /// /// the stream to read version information from /// number of bytes read including padding ///FormatVersion object ////// This operation will change the stream pointer. This function shouldn't be /// used in the scenarios when LoadFromBinaryReader can do the job. /// LoadFromBinaryReader will not leave around any undisposed objects, /// and LoadFromStream will. /// internal static FormatVersion LoadFromStream(Stream stream, out Int32 bytesRead) { if (stream == null) { throw new ArgumentNullException("stream"); } // Suppress 56518 Local IDisposable object not disposed: // Reason: The stream is not owned by the BlockManager, therefore we can // close the BinaryWriter as it will Close the stream underneath. #pragma warning disable 6518 BinaryReader streamReader = new BinaryReader(stream, System.Text.Encoding.Unicode); #pragma warning restore 6518 return LoadFromBinaryReader(streamReader, out bytesRead); } #endif //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Member Variables private VersionPair _reader; private VersionPair _updater; private VersionPair _writer; private String _featureIdentifier; #if false static private readonly int HashMask = 0x1f; #endif #endregion Member Variables } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implementation of the FormatVersion class, which describes the versioning // of an individual "format feature" within a compound file. // // History: // 06/20/2002: YoungGK: Created // 05/30/2003: LGolding: Ported to WCP tree. // //----------------------------------------------------------------------------- // Allow use of presharp warning numbers [6506] and [6518] unknown to the compiler #pragma warning disable 1634, 1691 using System; using System.IO; #if PBTCOMPILER using MS.Utility; // For SR.cs #else using System.Windows; using MS.Internal.WindowsBase; // FriendAccessAllowed #endif namespace MS.Internal.IO.Packaging.CompoundFile { ///Class for manipulating version object #if !PBTCOMPILER [FriendAccessAllowed] #endif internal class FormatVersion { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Constructors #if !PBTCOMPILER ////// Constructor for FormatVersion /// private FormatVersion() { } #endif ////// Constructor for FormatVersion with given featureId and version /// /// feature identifier /// version ///reader, updater, and writer versions are set to version public FormatVersion(string featureId, VersionPair version) : this(featureId, version, version, version) { } ////// Constructor for FormatVersion with given featureId and reader, updater, /// and writer version /// /// feature identifier /// Writer Version /// Reader Version /// Updater Version public FormatVersion(String featureId, VersionPair writerVersion, VersionPair readerVersion, VersionPair updaterVersion) { if (featureId == null) throw new ArgumentNullException("featureId"); if (writerVersion == null) throw new ArgumentNullException("writerVersion"); if (readerVersion == null) throw new ArgumentNullException("readerVersion"); if (updaterVersion == null) throw new ArgumentNullException("updaterVersion"); if (featureId.Length == 0) { throw new ArgumentException(SR.Get(SRID.ZeroLengthFeatureID)); } _featureIdentifier = featureId; _reader = readerVersion; _updater = updaterVersion; _writer = writerVersion; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #if !PBTCOMPILER ////// reader version /// public VersionPair ReaderVersion { get { return _reader; } set { if (value == null) { throw new ArgumentNullException("value"); } _reader = value; } } ////// writer version /// public VersionPair WriterVersion { get { return _writer; } set { if (value == null) { throw new ArgumentNullException("value"); } _writer = value; } } ////// updater version /// public VersionPair UpdaterVersion { get { return _updater; } set { if (value == null) { throw new ArgumentNullException("value"); } _updater = value; } } ////// feature identifier /// public String FeatureIdentifier { get { return _featureIdentifier; } } #endif #endregion Public Properties #region Operators #if false ////// == comparison operator /// /// version to be compared /// version to be compared public static bool operator ==(FormatVersion v1, FormatVersion v2) { // We have to cast v1 and v2 to Object // to ensure that the == operator on Ojbect class is used not the == operator on FormatVersion if ((Object) v1 == null || (Object) v2 == null) { return ((Object) v1 == null && (Object) v2 == null); } // Do comparison only if both v1 and v2 are not null return v1.Equals(v2); } ////// != comparison operator /// /// version to be compared /// version to be compared public static bool operator !=(FormatVersion v1, FormatVersion v2) { return !(v1 == v2); } ////// Eaual comparison operator /// /// Object to compare ///ture if the object is equal to this instance public override bool Equals(Object obj) { if (obj == null) { return false; } if (obj.GetType() != typeof(FormatVersion)) { return false; } FormatVersion v = (FormatVersion) obj; //PRESHARP:Parameter to this public method must be validated: A null-dereference can occur here. // Parameter 'v' to this public method must be validated: A null-dereference can occur here. //This is a false positive as the checks above can gurantee no null dereference will occur #pragma warning disable 6506 if (String.CompareOrdinal(_featureIdentifier.ToUpperInvariant(), v.FeatureIdentifier.ToUpperInvariant()) != 0 || _reader != v.ReaderVersion || _writer != v.WriterVersion || _updater != v.UpdaterVersion) { return false; } #pragma warning restore 6506 return true; } ////// Hash code /// public override int GetHashCode() { int hash = _reader.Major & HashMask; hash <<= 5; hash |= (_reader.Minor & HashMask); hash <<= 5; hash |= (_updater.Major & HashMask); hash <<= 5; hash |= (_updater.Minor & HashMask); hash <<= 5; hash |= (_writer.Major & HashMask); hash <<= 5; hash |= (_writer.Minor & HashMask); return hash; } #endif #endregion Operators //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #if !PBTCOMPILER ////// Constructor for FormatVersion with information read from the given stream /// /// Stream where version information is read from ///Before this function call the current position of stream should be /// pointing to the begining of the version structure. After this call the current /// poisition will be pointing immediately after the version structure public static FormatVersion LoadFromStream(Stream stream) { int bytesRead; return LoadFromStream(stream, out bytesRead); } #endif ////// Persist format version to the given stream /// /// the stream to be written ////// This operation will change the stream pointer /// stream can be null and will still return the number of bytes to be written /// public int SaveToStream(Stream stream) { checked { // Suppress 56518 Local IDisposable object not disposed: // Reason: The stream is not owned by the BlockManager, therefore we can // close the BinaryWriter as it will Close the stream underneath. #pragma warning disable 6518 int len = 0; BinaryWriter binarywriter = null; #pragma warning restore 6518 if (stream != null) { binarywriter = new BinaryWriter(stream, System.Text.Encoding.Unicode); } // ************ // feature ID // ************ len += ContainerUtilities.WriteByteLengthPrefixedDWordPaddedUnicodeString(binarywriter, _featureIdentifier); // **************** // Reader Version // **************** if (stream != null) { binarywriter.Write(_reader.Major); // Major number binarywriter.Write(_reader.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; // ***************** // Updater Version // ***************** if (stream != null) { binarywriter.Write(_updater.Major); // Major number binarywriter.Write(_updater.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; // **************** // Writer Version // **************** if (stream != null) { binarywriter.Write(_writer.Major); // Major number binarywriter.Write(_writer.Minor); // Minor number } len += ContainerUtilities.Int16Size; len += ContainerUtilities.Int16Size; return len; } } #if !PBTCOMPILER ////// Check if a component with the given version can read this format version safely /// /// version of a component ///true if this format version can be read safely by the component /// with the given version, otherwise false ////// The given version is checked against ReaderVersion /// public bool IsReadableBy(VersionPair version) { if (version == null) { throw new ArgumentNullException("version"); } return (_reader <= version); } ////// Check if a component with the given version can update this format version safely /// /// version of a component ///true if this format version can be updated safely by the component /// with the given version, otherwise false ////// The given version is checked against UpdaterVersion /// public bool IsUpdatableBy(VersionPair version) { if (version == null) { throw new ArgumentNullException("version"); } return (_updater <= version); } #endif #endregion Public Methods //----------------------------------------------------- // // Public Events // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Constructors // //----------------------------------------------------- // None //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ // None //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #if !PBTCOMPILER ////// Constructor for FormatVersion with information read from the given BinaryReader /// /// BinaryReader where version information is read from /// number of bytes read including padding ///FormatVersion object ////// This operation will change the stream pointer. This function is preferred over the /// LoadFromStream as it doesn't leave around Undisposed BinaryReader, which /// LoadFromStream will /// private static FormatVersion LoadFromBinaryReader(BinaryReader reader, out Int32 bytesRead) { checked { if (reader == null) { throw new ArgumentNullException("reader"); } FormatVersion ver = new FormatVersion(); bytesRead = 0; // Initialize the number of bytes read // ************** // feature ID // ************** Int32 strBytes; ver._featureIdentifier = ContainerUtilities.ReadByteLengthPrefixedDWordPaddedUnicodeString(reader, out strBytes); bytesRead += strBytes; Int16 major; Int16 minor; // **************** // Reader Version // **************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.ReaderVersion = new VersionPair(major, minor); // ***************** // Updater Version // ***************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.UpdaterVersion = new VersionPair(major, minor); // **************** // Writer Version // **************** major = reader.ReadInt16(); // Major number bytesRead += ContainerUtilities.Int16Size; minor = reader.ReadInt16(); // Minor number bytesRead += ContainerUtilities.Int16Size; ver.WriterVersion = new VersionPair(major, minor); return ver; } } ////// Create FormatVersion object and read version information from the given stream /// /// the stream to read version information from /// number of bytes read including padding ///FormatVersion object ////// This operation will change the stream pointer. This function shouldn't be /// used in the scenarios when LoadFromBinaryReader can do the job. /// LoadFromBinaryReader will not leave around any undisposed objects, /// and LoadFromStream will. /// internal static FormatVersion LoadFromStream(Stream stream, out Int32 bytesRead) { if (stream == null) { throw new ArgumentNullException("stream"); } // Suppress 56518 Local IDisposable object not disposed: // Reason: The stream is not owned by the BlockManager, therefore we can // close the BinaryWriter as it will Close the stream underneath. #pragma warning disable 6518 BinaryReader streamReader = new BinaryReader(stream, System.Text.Encoding.Unicode); #pragma warning restore 6518 return LoadFromBinaryReader(streamReader, out bytesRead); } #endif //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- // None //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- // None //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Member Variables private VersionPair _reader; private VersionPair _updater; private VersionPair _writer; private String _featureIdentifier; #if false static private readonly int HashMask = 0x1f; #endif #endregion Member Variables } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
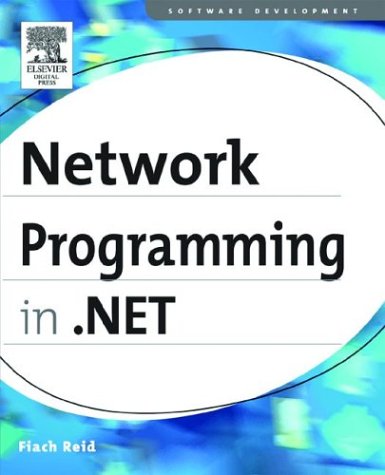
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Dump.cs
- Utils.cs
- XmlBinaryWriter.cs
- WindowsIdentity.cs
- DataGridViewSortCompareEventArgs.cs
- Pkcs7Recipient.cs
- ListControlBoundActionList.cs
- IDQuery.cs
- MultiAsyncResult.cs
- SqlConnectionStringBuilder.cs
- WindowsListViewItemStartMenu.cs
- MessageFormatterConverter.cs
- DeviceFilterDictionary.cs
- PropertyItem.cs
- HttpWebRequestElement.cs
- SetterBaseCollection.cs
- XPathNodeInfoAtom.cs
- ExceptionHandlerDesigner.cs
- WorkflowRuntime.cs
- PanelDesigner.cs
- DocumentPageHost.cs
- XmlDataProvider.cs
- MailWriter.cs
- BuildManager.cs
- PeerNameResolver.cs
- MaterialGroup.cs
- FontStyles.cs
- IndentedTextWriter.cs
- Baml2006ReaderSettings.cs
- PageThemeParser.cs
- RadioButton.cs
- TypeResolver.cs
- PenThreadWorker.cs
- BrushValueSerializer.cs
- NullableIntAverageAggregationOperator.cs
- BaseTemplateBuildProvider.cs
- DependsOnAttribute.cs
- SynchronizedCollection.cs
- ValidationPropertyAttribute.cs
- ErrorEventArgs.cs
- Control.cs
- SecurityResources.cs
- ImageConverter.cs
- PassportAuthentication.cs
- EventLogEntry.cs
- ExpressionBindings.cs
- XslAst.cs
- BooleanExpr.cs
- ItemsPanelTemplate.cs
- ToolStripArrowRenderEventArgs.cs
- AlgoModule.cs
- FontStretch.cs
- Site.cs
- DataConnectionHelper.cs
- DesignConnection.cs
- DispatchWrapper.cs
- TextSearch.cs
- Relationship.cs
- BulletedListEventArgs.cs
- HtmlWindow.cs
- ResXResourceSet.cs
- Tile.cs
- ObjectSelectorEditor.cs
- FormatConvertedBitmap.cs
- ModelItemDictionaryImpl.cs
- ObjectNavigationPropertyMapping.cs
- SQLDateTime.cs
- AutomationFocusChangedEventArgs.cs
- TagMapInfo.cs
- ZipIOCentralDirectoryFileHeader.cs
- ContentValidator.cs
- BinaryNode.cs
- CursorConverter.cs
- SqlBulkCopyColumnMappingCollection.cs
- Config.cs
- ImageListImageEditor.cs
- TraceContext.cs
- Span.cs
- ClientBuildManager.cs
- MsmqInputMessage.cs
- NavigationService.cs
- DbConnectionPoolOptions.cs
- SymbolEqualComparer.cs
- ExpressionLexer.cs
- BamlLocalizableResourceKey.cs
- ZipArchive.cs
- XmlEncoding.cs
- BindingCollection.cs
- DataGridViewMethods.cs
- CustomAttribute.cs
- StylusPointCollection.cs
- XamlToRtfWriter.cs
- WindowsImpersonationContext.cs
- BamlLocalizabilityResolver.cs
- SerializableAttribute.cs
- NotificationContext.cs
- BitmapEffectState.cs
- Point3DCollectionConverter.cs
- PolicyDesigner.cs
- MeasurementDCInfo.cs