Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / ui / CompositeScriptReference.cs / 1 / CompositeScriptReference.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Drawing.Design; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web; using System.Web.Handlers; using System.Web.Util; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("Path"), TypeConverter(typeof(EmptyStringExpandableObjectConverter)) ] public class CompositeScriptReference : ScriptReferenceBase { private ScriptReferenceCollection _scripts; [ ResourceDescription("CompositeScriptReference_Scripts"), Category("Behavior"), Editor("System.Web.UI.Design.CollectionEditorBase, " + AssemblyRef.SystemWebExtensionsDesign, typeof(UITypeEditor)), DefaultValue(null), PersistenceMode(PersistenceMode.InnerProperty), NotifyParentProperty(true), MergableProperty(false), ] public ScriptReferenceCollection Scripts { get { if (_scripts == null) { _scripts = new ScriptReferenceCollection(); } return _scripts; } } [SuppressMessage("Microsoft.Design", "CA1055", Justification = "Consistent with other URL properties in ASP.NET.")] protected internal override string GetUrl(ScriptManager scriptManager, bool zip) { bool isDebuggingEnabled = !scriptManager.DeploymentSectionRetail && ((ScriptMode == ScriptMode.Debug) || (((ScriptMode == ScriptMode.Inherit) || (ScriptMode == ScriptMode.Auto)) && (scriptManager.IsDebuggingEnabled))); if (!String.IsNullOrEmpty(Path)) { string path = Path; if (isDebuggingEnabled) { path = GetDebugPath(path); } if (scriptManager.EnableScriptLocalization && (ResourceUICultures != null) && (ResourceUICultures.Length != 0)) { CultureInfo currentCulture = CultureInfo.CurrentUICulture; string cultureName = null; bool found = false; while (!currentCulture.Equals(CultureInfo.InvariantCulture)) { cultureName = currentCulture.ToString(); foreach (string uiCulture in ResourceUICultures) { if (String.Equals(cultureName, uiCulture.Trim(), StringComparison.OrdinalIgnoreCase)) { found = true; break; } } if (found) break; currentCulture = currentCulture.Parent; } if (found) { path = (path.Substring(0, path.Length - 2) + cultureName + ".js"); } } // ResolveClientUrl is appropriate here because the path is consumed by the page it was declared within return ClientUrlResolver.ResolveClientUrl(path); } List>>> resources = new List >>>(); Pair >> resourceList = null; foreach (ScriptReference reference in Scripts) { bool hasPath = !String.IsNullOrEmpty(reference.Path); bool isPathBased = hasPath || (!String.IsNullOrEmpty(scriptManager.ScriptPath) && !reference.IgnoreScriptPath); Assembly resourceAssembly = hasPath ? null : (reference.GetAssembly() ?? AssemblyCache.SystemWebExtensions); Assembly cacheAssembly = isPathBased ? null : (reference.GetAssembly() ?? AssemblyCache.SystemWebExtensions); CultureInfo culture = reference.DetermineCulture(); if ((resourceList == null) || (resourceList.First != cacheAssembly)) { resourceList = new Pair >>( cacheAssembly, new List >()); resources.Add(resourceList); } string resourceName = null; ScriptMode effectiveScriptModeForReference = reference.EffectiveScriptMode; bool isDebuggingEnabledForReference = (effectiveScriptModeForReference == ScriptMode.Inherit) ? isDebuggingEnabled : (effectiveScriptModeForReference == ScriptMode.Debug); if (isPathBased) { if (hasPath) { resourceName = reference.GetPath(reference.Path, isDebuggingEnabledForReference); if (scriptManager.EnableScriptLocalization && !culture.Equals(CultureInfo.InvariantCulture)) { resourceName = (resourceName.Substring(0, resourceName.Length - 2) + culture.ToString() + ".js"); } } else { string name = reference.GetResourceName(reference.Name, resourceAssembly, isDebuggingEnabledForReference); resourceName = ScriptReference.GetScriptPath( name, resourceAssembly, culture, scriptManager.ScriptPath); } // ResolveClientUrl not appropriate here because the handler that will serve the response is not // in the same directory as the page that is generating the url. Instead, an absolute url is needed // as with ResolveUrl(). However, ResolveUrl() would prepend the entire application root name. For // example, ~/foo.js would be /TheApplicationRoot/foo.js. If there are many path based scripts the // app root would be repeated many times, which for deep apps or long named apps could cause the url // to reach the maximum 1024 characters very quickly. So, the path is combined with the control's // AppRelativeTemplateSourceDirectory manually, so that ~/foo.js remains ~/foo.js, and foo/bar.js // becomes ~/templatesource/foo/bar.js. Absolute paths can remain as is. The ScriptResourceHandler will // resolve the ~/ with the app root using VirtualPathUtility.ToAbsolute(). if (UrlPath.IsRelativeUrl(resourceName) && !UrlPath.IsAppRelativePath(resourceName)) { resourceName = UrlPath.Combine(ClientUrlResolver.AppRelativeTemplateSourceDirectory, resourceName); } } else { resourceName = reference.GetResourceName(reference.Name, resourceAssembly, isDebuggingEnabledForReference); } resourceList.Second.Add(new Pair (resourceName, culture)); } return ScriptResourceHandler.GetScriptResourceUrl(resources, zip, NotifyScriptLoaded); } protected internal override bool IsFromSystemWebExtensions() { foreach (ScriptReference script in Scripts) { if (script.GetAssembly() == AssemblyCache.SystemWebExtensions) { return true; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Drawing.Design; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web; using System.Web.Handlers; using System.Web.Util; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("Path"), TypeConverter(typeof(EmptyStringExpandableObjectConverter)) ] public class CompositeScriptReference : ScriptReferenceBase { private ScriptReferenceCollection _scripts; [ ResourceDescription("CompositeScriptReference_Scripts"), Category("Behavior"), Editor("System.Web.UI.Design.CollectionEditorBase, " + AssemblyRef.SystemWebExtensionsDesign, typeof(UITypeEditor)), DefaultValue(null), PersistenceMode(PersistenceMode.InnerProperty), NotifyParentProperty(true), MergableProperty(false), ] public ScriptReferenceCollection Scripts { get { if (_scripts == null) { _scripts = new ScriptReferenceCollection(); } return _scripts; } } [SuppressMessage("Microsoft.Design", "CA1055", Justification = "Consistent with other URL properties in ASP.NET.")] protected internal override string GetUrl(ScriptManager scriptManager, bool zip) { bool isDebuggingEnabled = !scriptManager.DeploymentSectionRetail && ((ScriptMode == ScriptMode.Debug) || (((ScriptMode == ScriptMode.Inherit) || (ScriptMode == ScriptMode.Auto)) && (scriptManager.IsDebuggingEnabled))); if (!String.IsNullOrEmpty(Path)) { string path = Path; if (isDebuggingEnabled) { path = GetDebugPath(path); } if (scriptManager.EnableScriptLocalization && (ResourceUICultures != null) && (ResourceUICultures.Length != 0)) { CultureInfo currentCulture = CultureInfo.CurrentUICulture; string cultureName = null; bool found = false; while (!currentCulture.Equals(CultureInfo.InvariantCulture)) { cultureName = currentCulture.ToString(); foreach (string uiCulture in ResourceUICultures) { if (String.Equals(cultureName, uiCulture.Trim(), StringComparison.OrdinalIgnoreCase)) { found = true; break; } } if (found) break; currentCulture = currentCulture.Parent; } if (found) { path = (path.Substring(0, path.Length - 2) + cultureName + ".js"); } } // ResolveClientUrl is appropriate here because the path is consumed by the page it was declared within return ClientUrlResolver.ResolveClientUrl(path); } List>>> resources = new List >>>(); Pair >> resourceList = null; foreach (ScriptReference reference in Scripts) { bool hasPath = !String.IsNullOrEmpty(reference.Path); bool isPathBased = hasPath || (!String.IsNullOrEmpty(scriptManager.ScriptPath) && !reference.IgnoreScriptPath); Assembly resourceAssembly = hasPath ? null : (reference.GetAssembly() ?? AssemblyCache.SystemWebExtensions); Assembly cacheAssembly = isPathBased ? null : (reference.GetAssembly() ?? AssemblyCache.SystemWebExtensions); CultureInfo culture = reference.DetermineCulture(); if ((resourceList == null) || (resourceList.First != cacheAssembly)) { resourceList = new Pair >>( cacheAssembly, new List >()); resources.Add(resourceList); } string resourceName = null; ScriptMode effectiveScriptModeForReference = reference.EffectiveScriptMode; bool isDebuggingEnabledForReference = (effectiveScriptModeForReference == ScriptMode.Inherit) ? isDebuggingEnabled : (effectiveScriptModeForReference == ScriptMode.Debug); if (isPathBased) { if (hasPath) { resourceName = reference.GetPath(reference.Path, isDebuggingEnabledForReference); if (scriptManager.EnableScriptLocalization && !culture.Equals(CultureInfo.InvariantCulture)) { resourceName = (resourceName.Substring(0, resourceName.Length - 2) + culture.ToString() + ".js"); } } else { string name = reference.GetResourceName(reference.Name, resourceAssembly, isDebuggingEnabledForReference); resourceName = ScriptReference.GetScriptPath( name, resourceAssembly, culture, scriptManager.ScriptPath); } // ResolveClientUrl not appropriate here because the handler that will serve the response is not // in the same directory as the page that is generating the url. Instead, an absolute url is needed // as with ResolveUrl(). However, ResolveUrl() would prepend the entire application root name. For // example, ~/foo.js would be /TheApplicationRoot/foo.js. If there are many path based scripts the // app root would be repeated many times, which for deep apps or long named apps could cause the url // to reach the maximum 1024 characters very quickly. So, the path is combined with the control's // AppRelativeTemplateSourceDirectory manually, so that ~/foo.js remains ~/foo.js, and foo/bar.js // becomes ~/templatesource/foo/bar.js. Absolute paths can remain as is. The ScriptResourceHandler will // resolve the ~/ with the app root using VirtualPathUtility.ToAbsolute(). if (UrlPath.IsRelativeUrl(resourceName) && !UrlPath.IsAppRelativePath(resourceName)) { resourceName = UrlPath.Combine(ClientUrlResolver.AppRelativeTemplateSourceDirectory, resourceName); } } else { resourceName = reference.GetResourceName(reference.Name, resourceAssembly, isDebuggingEnabledForReference); } resourceList.Second.Add(new Pair (resourceName, culture)); } return ScriptResourceHandler.GetScriptResourceUrl(resources, zip, NotifyScriptLoaded); } protected internal override bool IsFromSystemWebExtensions() { foreach (ScriptReference script in Scripts) { if (script.GetAssembly() == AssemblyCache.SystemWebExtensions) { return true; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
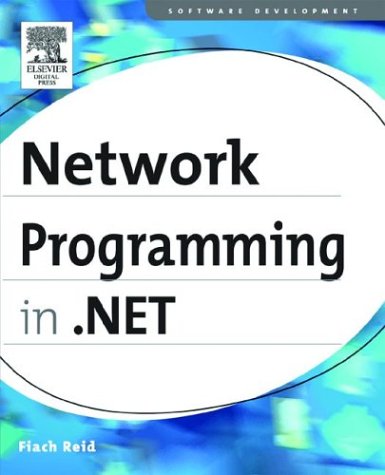
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebBrowserEvent.cs
- RoutedEventConverter.cs
- HandlerFactoryWrapper.cs
- ScrollItemPatternIdentifiers.cs
- selecteditemcollection.cs
- EntityDataSourceContextCreatedEventArgs.cs
- ServiceOperationWrapper.cs
- DiscoveryReference.cs
- PtsCache.cs
- CqlParser.cs
- InternalsVisibleToAttribute.cs
- CreateUserWizardStep.cs
- FontFaceLayoutInfo.cs
- DeflateStreamAsyncResult.cs
- HwndHostAutomationPeer.cs
- SourceFileBuildProvider.cs
- EventLogEntry.cs
- LeaseManager.cs
- SamlAdvice.cs
- LowerCaseStringConverter.cs
- CommonRemoteMemoryBlock.cs
- NavigationWindowAutomationPeer.cs
- CodeExporter.cs
- CodeIdentifiers.cs
- CustomCategoryAttribute.cs
- SHA256.cs
- EffectiveValueEntry.cs
- MetadataCacheItem.cs
- DBAsyncResult.cs
- DataServiceOperationContext.cs
- x509store.cs
- OleServicesContext.cs
- RootDesignerSerializerAttribute.cs
- ContextDataSourceContextData.cs
- COM2ColorConverter.cs
- SmtpMail.cs
- BitmapEffectDrawingContent.cs
- HttpCapabilitiesSectionHandler.cs
- SessionParameter.cs
- NotifyIcon.cs
- DefaultObjectSerializer.cs
- WebResourceUtil.cs
- StopStoryboard.cs
- SoapRpcMethodAttribute.cs
- ArrayTypeMismatchException.cs
- MimeTypePropertyAttribute.cs
- ChtmlTextWriter.cs
- SqlRowUpdatingEvent.cs
- DataGridViewRowsRemovedEventArgs.cs
- SystemIPv6InterfaceProperties.cs
- SynchronizationFilter.cs
- UDPClient.cs
- PerfService.cs
- XmlSchemaObjectTable.cs
- WebPartEditorCancelVerb.cs
- RelationshipDetailsRow.cs
- DynamicDiscoSearcher.cs
- CachedTypeface.cs
- ResourceDefaultValueAttribute.cs
- SortQuery.cs
- DataGridViewTopRowAccessibleObject.cs
- BindingWorker.cs
- Compress.cs
- EntitySet.cs
- RegexEditorDialog.cs
- ColorPalette.cs
- StyleTypedPropertyAttribute.cs
- ScriptReferenceEventArgs.cs
- DataSourceHelper.cs
- ItemsControlAutomationPeer.cs
- VisualBrush.cs
- WindowsButton.cs
- XMLUtil.cs
- DecoderFallback.cs
- Codec.cs
- IteratorFilter.cs
- WpfWebRequestHelper.cs
- ConstructorBuilder.cs
- QilVisitor.cs
- UIElementHelper.cs
- BaseCodePageEncoding.cs
- DataControlFieldCollection.cs
- XmlTextWriter.cs
- MethodRental.cs
- DictionarySurrogate.cs
- ParserHooks.cs
- CopyNamespacesAction.cs
- SocketAddress.cs
- TagPrefixAttribute.cs
- AuthStoreRoleProvider.cs
- SymLanguageType.cs
- SoapSchemaMember.cs
- WorkflowServiceNamespace.cs
- ElementAction.cs
- SqlFunctionAttribute.cs
- SecurityTokenSpecification.cs
- DataGridViewRowHeaderCell.cs
- AssemblyAttributes.cs
- PageAsyncTask.cs
- HttpCapabilitiesEvaluator.cs