Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / TabRenderer.cs / 1 / TabRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TabRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; //cannot instantiate private TabRenderer() { } ////// This is a rendering class for the Tab control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabItem(Graphics g, Rectangle bounds, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, false, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageRectangle, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabPage(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.Tab.Pane.Normal, 0); visualStyleRenderer.DrawBackground(g, bounds); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Renders a TabPage. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TabRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; //cannot instantiate private TabRenderer() { } ////// This is a rendering class for the Tab control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabItem(Graphics g, Rectangle bounds, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, false, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { DrawTabItem(g, bounds, tabItemText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageRectangle, focused, state); } ////// Renders a Tab item. /// ////// /// public static void DrawTabItem(Graphics g, Rectangle bounds, string tabItemText, Font font, TextFormatFlags flags, Image image, Rectangle imageRectangle, bool focused, TabItemState state) { InitializeRenderer(VisualStyleElement.Tab.TabItem.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); // I need this hack since GetBackgroundContentRectangle() returns same rectangle // as bounds for this control! Rectangle contentBounds = Rectangle.Inflate(bounds, -3, -3); visualStyleRenderer.DrawImage(g, imageRectangle, image); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, tabItemText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Tab item. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTabPage(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.Tab.Pane.Normal, 0); visualStyleRenderer.DrawBackground(g, bounds); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Renders a TabPage. /// ///
Link Menu
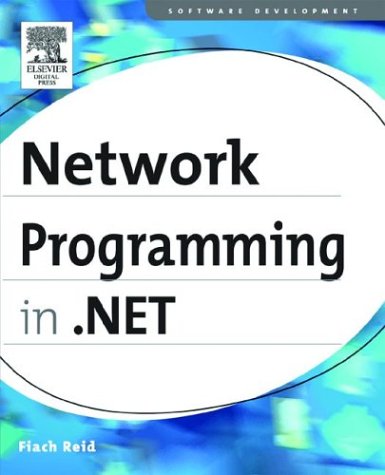
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinaryNode.cs
- PaperSource.cs
- DragEventArgs.cs
- SafeProcessHandle.cs
- ObjectParameter.cs
- WindowsUserNameCachingSecurityTokenAuthenticator.cs
- TemplateXamlParser.cs
- Normalization.cs
- NumberFunctions.cs
- PrtTicket_Editor.cs
- AccessorTable.cs
- MediaContextNotificationWindow.cs
- PlanCompilerUtil.cs
- MenuAutoFormat.cs
- Rotation3DKeyFrameCollection.cs
- ListBindingHelper.cs
- TaskHelper.cs
- EntryIndex.cs
- TargetParameterCountException.cs
- Attachment.cs
- RequestCachePolicy.cs
- MultipartIdentifier.cs
- SystemIPAddressInformation.cs
- DiscoveryEndpoint.cs
- SHA512Cng.cs
- HtmlWindow.cs
- DataGridBoolColumn.cs
- ReaderWriterLockWrapper.cs
- AutomationElementIdentifiers.cs
- smtpconnection.cs
- _OverlappedAsyncResult.cs
- DataGridTable.cs
- SqlExpander.cs
- EntityDataSourceContextCreatingEventArgs.cs
- versioninfo.cs
- UnsafeNativeMethods.cs
- ToolBarButton.cs
- DataRelationPropertyDescriptor.cs
- ListParagraph.cs
- MarginsConverter.cs
- ConfigurationElementProperty.cs
- SecureStringHasher.cs
- Enum.cs
- QueryPageSettingsEventArgs.cs
- Vector3D.cs
- TemplateControl.cs
- CollectionView.cs
- PageTheme.cs
- NameSpaceEvent.cs
- UniqueID.cs
- Debugger.cs
- TextFormatterImp.cs
- TypefaceCollection.cs
- Int16.cs
- StatusBarPanelClickEvent.cs
- ScriptControl.cs
- XmlIncludeAttribute.cs
- ListChangedEventArgs.cs
- CssClassPropertyAttribute.cs
- CompilerErrorCollection.cs
- ShaderEffect.cs
- ToolBarButton.cs
- ConnectionOrientedTransportManager.cs
- TypedServiceOperationListItem.cs
- SeverityFilter.cs
- RenderContext.cs
- HwndSource.cs
- ErrorWebPart.cs
- PanelDesigner.cs
- StickyNote.cs
- PersonalizationEntry.cs
- SQLInt16Storage.cs
- RectangleHotSpot.cs
- DataGridViewCellCollection.cs
- ControlPropertyNameConverter.cs
- CalendarDesigner.cs
- MetadataArtifactLoaderFile.cs
- querybuilder.cs
- SoundPlayerAction.cs
- MD5Cng.cs
- ObsoleteAttribute.cs
- EntityConnection.cs
- MailMessage.cs
- TextBlock.cs
- TextEditorDragDrop.cs
- OutputCacheProfileCollection.cs
- DataSysAttribute.cs
- TypeInformation.cs
- DataColumnMapping.cs
- FunctionQuery.cs
- Types.cs
- BindingGroup.cs
- AsyncStreamReader.cs
- filewebrequest.cs
- EffectiveValueEntry.cs
- Win32KeyboardDevice.cs
- ProviderUtil.cs
- DynamicResourceExtensionConverter.cs
- StringFreezingAttribute.cs
- ProjectionCamera.cs