Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewCellLinkedList.cs / 1 / DataGridViewCellLinkedList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Windows.Forms; using System.ComponentModel; ////// /// internal class DataGridViewCellLinkedList : IEnumerable { private DataGridViewCellLinkedListElement lastAccessedElement; private DataGridViewCellLinkedListElement headElement; private int count, lastAccessedIndex; ///Represents a linked list of ///objects IEnumerator IEnumerable.GetEnumerator() { return new DataGridViewCellLinkedListEnumerator(this.headElement); } /// public DataGridViewCellLinkedList() { this.lastAccessedIndex = -1; } /// public DataGridViewCell this[int index] { get { Debug.Assert(index >= 0); Debug.Assert(index < this.count); if (this.lastAccessedIndex == -1 || index < this.lastAccessedIndex) { DataGridViewCellLinkedListElement tmp = this.headElement; int tmpIndex = index; while (tmpIndex > 0) { tmp = tmp.Next; tmpIndex--; } this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return tmp.DataGridViewCell; } else { while (this.lastAccessedIndex < index) { this.lastAccessedElement = this.lastAccessedElement.Next; this.lastAccessedIndex++; } return this.lastAccessedElement.DataGridViewCell; } } } /// public int Count { get { return this.count; } } /// public DataGridViewCell HeadCell { get { Debug.Assert(this.headElement != null); return this.headElement.DataGridViewCell; } } /// public void Add(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); Debug.Assert(dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.CellSelect || dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.ColumnHeaderSelect || dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.RowHeaderSelect); DataGridViewCellLinkedListElement newHead = new DataGridViewCellLinkedListElement(dataGridViewCell); if (this.headElement != null) { newHead.Next = this.headElement; } this.headElement = newHead; this.count++; this.lastAccessedElement = null; this.lastAccessedIndex = -1; } /// public void Clear() { this.lastAccessedElement = null; this.lastAccessedIndex = -1; this.headElement = null; this.count = 0; } /// public bool Contains(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); int index = 0; DataGridViewCellLinkedListElement tmp = this.headElement; while (tmp != null) { if (tmp.DataGridViewCell == dataGridViewCell) { this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return true; } tmp = tmp.Next; index++; } return false; } /// public bool Remove(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); DataGridViewCellLinkedListElement tmp1 = null, tmp2 = this.headElement; while (tmp2 != null) { if (tmp2.DataGridViewCell == dataGridViewCell) { break; } tmp1 = tmp2; tmp2 = tmp2.Next; } if (tmp2.DataGridViewCell == dataGridViewCell) { DataGridViewCellLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; return true; } return false; } /* Unused for now /// public DataGridViewCell RemoveHead() { if (this.headElement == null) { return null; } DataGridViewCellLinkedListElement tmp = this.headElement; this.headElement = tmp.Next; this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; return tmp.DataGridViewCell; } */ /// public int RemoveAllCellsAtBand(bool column, int bandIndex) { int removedCount = 0; DataGridViewCellLinkedListElement tmp1 = null, tmp2 = this.headElement; while (tmp2 != null) { if ((column && tmp2.DataGridViewCell.ColumnIndex == bandIndex) || (!column && tmp2.DataGridViewCell.RowIndex == bandIndex)) { DataGridViewCellLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } tmp2 = tmp3; this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; removedCount++; } else { tmp1 = tmp2; tmp2 = tmp2.Next; } } return removedCount; } } /// /// /// internal class DataGridViewCellLinkedListEnumerator : IEnumerator { private DataGridViewCellLinkedListElement headElement; private DataGridViewCellLinkedListElement current; private bool reset; ///Represents an emunerator of elements in a ///linked list. public DataGridViewCellLinkedListEnumerator(DataGridViewCellLinkedListElement headElement) { this.headElement = headElement; this.reset = true; } /// object IEnumerator.Current { get { Debug.Assert(this.current != null); // Since this is for internal use only. return this.current.DataGridViewCell; } } /// bool IEnumerator.MoveNext() { if (this.reset) { Debug.Assert(this.current == null); this.current = this.headElement; this.reset = false; } else { Debug.Assert(this.current != null); // Since this is for internal use only. this.current = this.current.Next; } return (this.current != null); } /// void IEnumerator.Reset() { this.reset = true; this.current = null; } } /// /// /// internal class DataGridViewCellLinkedListElement { private DataGridViewCell dataGridViewCell; private DataGridViewCellLinkedListElement next; ///Represents an element in a ///linked list. public DataGridViewCellLinkedListElement(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); this.dataGridViewCell = dataGridViewCell; } /// public DataGridViewCell DataGridViewCell { get { return this.dataGridViewCell; } } /// public DataGridViewCellLinkedListElement Next { get { return this.next; } set { this.next = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
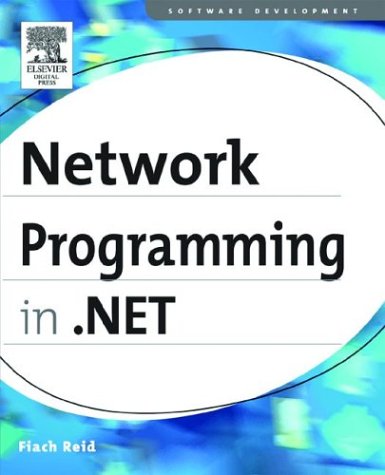
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JsonReaderDelegator.cs
- FilePrompt.cs
- SingleAnimationBase.cs
- PinnedBufferMemoryStream.cs
- InvalidOleVariantTypeException.cs
- bidPrivateBase.cs
- DynamicILGenerator.cs
- ProviderIncompatibleException.cs
- ControlIdConverter.cs
- SqlCaseSimplifier.cs
- SimpleTypeResolver.cs
- StaticDataManager.cs
- GeneralTransform3DGroup.cs
- HtmlImage.cs
- ArrayEditor.cs
- EllipseGeometry.cs
- TextTrailingCharacterEllipsis.cs
- StyleSelector.cs
- DefaultAsyncDataDispatcher.cs
- keycontainerpermission.cs
- WindowsUpDown.cs
- BamlRecordWriter.cs
- Solver.cs
- PromptStyle.cs
- GenericIdentity.cs
- SlotInfo.cs
- TdsParameterSetter.cs
- OutputCacheSection.cs
- ProcessHostMapPath.cs
- VectorCollectionConverter.cs
- Random.cs
- TrackingProfileDeserializationException.cs
- FlowLayoutSettings.cs
- PerfService.cs
- RelatedImageListAttribute.cs
- UserNamePasswordValidationMode.cs
- NetworkInformationPermission.cs
- DayRenderEvent.cs
- SqlFunctionAttribute.cs
- Transform3D.cs
- WindowsUpDown.cs
- IChannel.cs
- PropertyCondition.cs
- _AutoWebProxyScriptHelper.cs
- XsdDataContractImporter.cs
- WorkflowRuntimeServiceElementCollection.cs
- ActivityPreviewDesigner.cs
- CompressedStack.cs
- InvokeGenerator.cs
- XmlLanguage.cs
- DataGridViewCellStyleBuilderDialog.cs
- DrawingAttributeSerializer.cs
- ListInitExpression.cs
- PagesSection.cs
- wmiutil.cs
- AppModelKnownContentFactory.cs
- ExtentJoinTreeNode.cs
- DefaultEventAttribute.cs
- ServiceNameElement.cs
- NamespaceCollection.cs
- ResXResourceReader.cs
- HostProtectionException.cs
- IxmlLineInfo.cs
- Point3DAnimationBase.cs
- RoleExceptions.cs
- WebRequest.cs
- UserValidatedEventArgs.cs
- DataGridViewCellFormattingEventArgs.cs
- XPathMultyIterator.cs
- Part.cs
- DataServiceContext.cs
- XmlArrayItemAttributes.cs
- SpecularMaterial.cs
- XPathParser.cs
- Switch.cs
- SettingsBase.cs
- HttpDictionary.cs
- WebPartHeaderCloseVerb.cs
- SimpleType.cs
- ScriptMethodAttribute.cs
- ReadOnlyObservableCollection.cs
- TogglePattern.cs
- DiagnosticsConfigurationHandler.cs
- SqlUtils.cs
- RemotingConfigParser.cs
- OleDbTransaction.cs
- ToolStripContentPanelRenderEventArgs.cs
- HScrollProperties.cs
- Graphics.cs
- NgenServicingAttributes.cs
- SessionIDManager.cs
- AlternationConverter.cs
- TemplateBuilder.cs
- Lasso.cs
- QueryRewriter.cs
- TableCellCollection.cs
- PrintControllerWithStatusDialog.cs
- NullReferenceException.cs
- TextBoxBase.cs
- MappingMetadataHelper.cs