Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / WebControls / TableCellCollection.cs / 1 / TableCellCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.TableCellsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TableCellCollection : IList { ///Encapsulates the collection of ///and objects within a /// /// control. /// private TableRow owner; ////// A protected field of type ///. Represents the /// /// collection internally. /// /// internal TableCellCollection(TableRow owner) { this.owner = owner; } ////// public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///Gets the ////// count in the collection. /// public TableCell this[int index] { get { return(TableCell)owner.Controls[index]; } } ////// Gets a ////// referenced by the specified /// ordinal index value. /// /// public int Add(TableCell cell) { AddAt(-1, cell); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableCell cell) { owner.Controls.AddAt(index, cell); } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableCell[] cells) { if (cells == null) { throw new ArgumentNullException("cells"); } foreach(TableCell cell in cells) { Add(cell); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); } } ///Removes all ///controls /// from the collection. /// public int GetCellIndex(TableCell cell) { if (owner.HasControls()) { return owner.Controls.IndexOf(cell); } return -1; } ///Returns an ordinal index value that represents the position of the /// specified ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the /// collection. In this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableCell cell) { owner.Controls.Remove(cell); } ////// Removes the specified ///from the /// collection. /// /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ////// Removes the ///from the collection at the /// specified index location. /// object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableCell)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableCell) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableCell)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableCell)o); } /// void IList.Insert(int index, object o) { owner.Controls.AddAt(index, (TableCell)o); } /// void IList.Remove(object o) { owner.Controls.Remove((TableCell)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Drawing.Design; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ Editor("System.Web.UI.Design.WebControls.TableCellsCollectionEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TableCellCollection : IList { ///Encapsulates the collection of ///and objects within a /// /// control. /// private TableRow owner; ////// A protected field of type ///. Represents the /// /// collection internally. /// /// internal TableCellCollection(TableRow owner) { this.owner = owner; } ////// public int Count { get { if (owner.HasControls()) { return owner.Controls.Count; } return 0; } } ///Gets the ////// count in the collection. /// public TableCell this[int index] { get { return(TableCell)owner.Controls[index]; } } ////// Gets a ////// referenced by the specified /// ordinal index value. /// /// public int Add(TableCell cell) { AddAt(-1, cell); return owner.Controls.Count - 1; } ////// Adds the specified ///to the end of the collection. /// /// public void AddAt(int index, TableCell cell) { owner.Controls.AddAt(index, cell); } ////// Adds the specified ///to the collection at the specified /// index location. /// /// public void AddRange(TableCell[] cells) { if (cells == null) { throw new ArgumentNullException("cells"); } foreach(TableCell cell in cells) { Add(cell); } } ////// public void Clear() { if (owner.HasControls()) { owner.Controls.Clear(); } } ///Removes all ///controls /// from the collection. /// public int GetCellIndex(TableCell cell) { if (owner.HasControls()) { return owner.Controls.IndexOf(cell); } return -1; } ///Returns an ordinal index value that represents the position of the /// specified ///within the collection. /// public IEnumerator GetEnumerator() { return owner.Controls.GetEnumerator(); } ////// Returns an enumerator of all ///controls within the /// collection. /// /// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies contents from the collection to the specified ///with the /// specified starting index. /// public Object SyncRoot { get { return this;} } ////// Gets the object that can be used to synchronize access to the /// collection. In this case, it is the collection itself. /// ////// public bool IsReadOnly { get { return false;} } ////// Gets a value indicating whether the collection is read-only. /// ////// public bool IsSynchronized { get { return false;} } ////// Gets a value indicating whether access to the collection is synchronized /// (thread-safe). /// ////// public void Remove(TableCell cell) { owner.Controls.Remove(cell); } ////// Removes the specified ///from the /// collection. /// /// public void RemoveAt(int index) { owner.Controls.RemoveAt(index); } // IList implementation, required by collection editor ////// Removes the ///from the collection at the /// specified index location. /// object IList.this[int index] { get { return owner.Controls[index]; } set { RemoveAt(index); AddAt(index, (TableCell)value); } } /// bool IList.IsFixedSize { get { return false; } } /// int IList.Add(object o) { return Add((TableCell) o); } /// bool IList.Contains(object o) { return owner.Controls.Contains((TableCell)o); } /// int IList.IndexOf(object o) { return owner.Controls.IndexOf((TableCell)o); } /// void IList.Insert(int index, object o) { owner.Controls.AddAt(index, (TableCell)o); } /// void IList.Remove(object o) { owner.Controls.Remove((TableCell)o); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
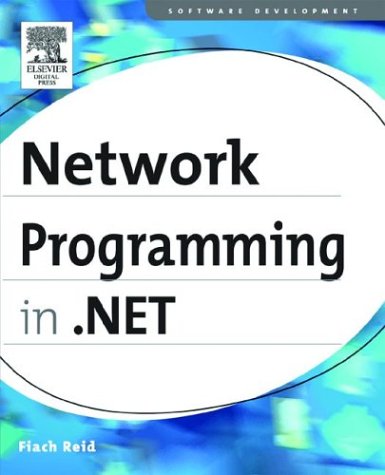
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThousandthOfEmRealDoubles.cs
- XmlSchemaExporter.cs
- PathParser.cs
- _RequestCacheProtocol.cs
- SerialPinChanges.cs
- CaseStatement.cs
- TypeForwardedToAttribute.cs
- MessageDecoder.cs
- RemotingServices.cs
- AccessDataSourceWizardForm.cs
- SelfIssuedAuthProofToken.cs
- BasicBrowserDialog.cs
- SizeFConverter.cs
- MetaColumn.cs
- TextRangeEditLists.cs
- SamlAudienceRestrictionCondition.cs
- EdmComplexTypeAttribute.cs
- DrawListViewSubItemEventArgs.cs
- XmlQueryType.cs
- VSWCFServiceContractGenerator.cs
- ToolboxItemFilterAttribute.cs
- ServicePointManagerElement.cs
- MenuItemBinding.cs
- ResXResourceWriter.cs
- MobileControlBuilder.cs
- XamlContextStack.cs
- IWorkflowDebuggerService.cs
- ProfilePropertySettings.cs
- Win32SafeHandles.cs
- RMPublishingDialog.cs
- ComplexType.cs
- AnnouncementEndpointElement.cs
- BinaryNode.cs
- DescendantOverDescendantQuery.cs
- Policy.cs
- ButtonField.cs
- DataObjectFieldAttribute.cs
- RepeaterItem.cs
- CodeObjectCreateExpression.cs
- MobileErrorInfo.cs
- WebPartDescription.cs
- DateTimeConstantAttribute.cs
- Descriptor.cs
- LabelEditEvent.cs
- IxmlLineInfo.cs
- DSASignatureFormatter.cs
- XmlTypeAttribute.cs
- StickyNoteAnnotations.cs
- XPathNodePointer.cs
- WindowsPen.cs
- SqlCacheDependency.cs
- UniqueSet.cs
- HwndSubclass.cs
- JsonEnumDataContract.cs
- SortFieldComparer.cs
- ObjectDataSourceMethodEditor.cs
- ProfileGroupSettingsCollection.cs
- CodeChecksumPragma.cs
- SpStreamWrapper.cs
- TextAdaptor.cs
- AssemblyInfo.cs
- BufferModeSettings.cs
- ContentHostHelper.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- RotateTransform.cs
- BigInt.cs
- UncommonField.cs
- SQLSingle.cs
- AssociationSetMetadata.cs
- Automation.cs
- BoundField.cs
- Viewport2DVisual3D.cs
- WriteableBitmap.cs
- EndpointConfigContainer.cs
- ScriptResourceInfo.cs
- ReferencedAssembly.cs
- QuaternionIndependentAnimationStorage.cs
- ParserStreamGeometryContext.cs
- CollectionBuilder.cs
- DragEvent.cs
- Mutex.cs
- CollectionMarkupSerializer.cs
- SourceSwitch.cs
- PriorityQueue.cs
- PropertyKey.cs
- CollectionChange.cs
- ColorAnimationBase.cs
- StylusOverProperty.cs
- XmlWhitespace.cs
- CapabilitiesSection.cs
- KerberosTokenFactoryCredential.cs
- ConnectionProviderAttribute.cs
- SmiXetterAccessMap.cs
- LaxModeSecurityHeaderElementInferenceEngine.cs
- InstanceDataCollection.cs
- InputQueue.cs
- RouteUrlExpressionBuilder.cs
- BitmapScalingModeValidation.cs
- UTF8Encoding.cs
- InvalidOperationException.cs