Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / XpsTokenContext.cs / 1 / XpsTokenContext.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: XpsTokenContext.cs Abstract: Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; namespace System.Windows.Xps.Serialization { internal class XpsTokenContext : System.ComponentModel.ITypeDescriptorContext { ////// Constructor for XpsTokenContext /// public XpsTokenContext( PackageSerializationManager serializationManager, SerializablePropertyContext propertyContext ) { // // Make necessary checks and throw necessary exceptions // this.serializationManager = serializationManager; this.targetObject = propertyContext.TargetObject; this.objectValue = propertyContext.Value; this.propertyInfo = propertyContext.PropertyInfo; this.dependencyProperty = (propertyContext is SerializableDependencyPropertyContext) ? (DependencyProperty)((SerializableDependencyPropertyContext)propertyContext).DependencyProperty : null; } ////// Constructor for XpsTokenContext /// public XpsTokenContext( PackageSerializationManager serializationManager, Object targetObject, Object objectValue ) { // // Make necessary checks and throw necessary exceptions // this.serializationManager = serializationManager; this.targetObject = targetObject; this.objectValue = objectValue; this.propertyInfo = null; this.dependencyProperty = null; } ////// /// public void OnComponentChanged() { } //// // public bool OnComponentChanging() { return false; } //// // public object GetService( Type serviceType ) { Object serviceObject = null; if (serviceType == typeof(XpsSerializationManager) || serviceType == typeof(XpsSerializationManagerAsync) || serviceType == typeof(ServiceProviders)) { serviceObject = serializationManager; } return serviceObject; } //// // public System.ComponentModel.IContainer Container { get { return null; } } //// // public object Instance { get { return objectValue; } } //// // public PropertyInfo PropertyInfo { get { return propertyInfo; } } //// // public DependencyProperty DependencyProperty { get { return dependencyProperty; } } //// // public object TargetObject { get { return targetObject; } } //// // public PropertyDescriptor PropertyDescriptor { get { return null; } } private PackageSerializationManager serializationManager; private Object targetObject; private Object objectValue; private PropertyInfo propertyInfo; private DependencyProperty dependencyProperty; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
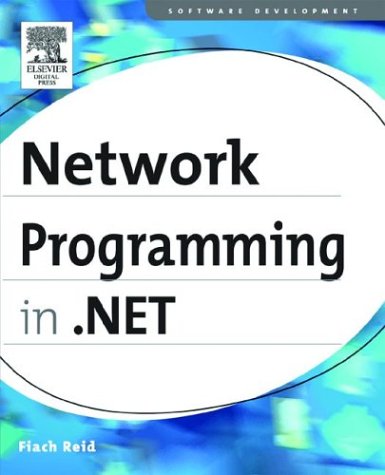
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WasHostedComPlusFactory.cs
- ISAPIApplicationHost.cs
- ReflectionTypeLoadException.cs
- ExtensionWindowHeader.cs
- DebugController.cs
- SiteMapNodeCollection.cs
- HttpPostedFileWrapper.cs
- cache.cs
- WebPartCatalogAddVerb.cs
- BamlMapTable.cs
- DataControlImageButton.cs
- ObjectPropertyMapping.cs
- ImportCatalogPart.cs
- ProxyElement.cs
- GridViewRow.cs
- HttpRequest.cs
- SecurityException.cs
- TrustLevel.cs
- SizeAnimationBase.cs
- PersistChildrenAttribute.cs
- AutomationInteropProvider.cs
- PocoEntityKeyStrategy.cs
- XmlSchemaDocumentation.cs
- FixedSOMImage.cs
- InstancePersistenceCommand.cs
- UIElementHelper.cs
- ClientRuntime.cs
- MimeParameterWriter.cs
- ResourceReferenceExpression.cs
- HtmlMeta.cs
- GridViewUpdateEventArgs.cs
- XPathDocumentBuilder.cs
- ObjectNotFoundException.cs
- HostedTransportConfigurationBase.cs
- XslCompiledTransform.cs
- NameTable.cs
- DataDocumentXPathNavigator.cs
- EntityParameter.cs
- ClipboardProcessor.cs
- SecurityHeaderLayout.cs
- WebBrowserContainer.cs
- SmtpNegotiateAuthenticationModule.cs
- ParentQuery.cs
- FloaterParagraph.cs
- MobileTextWriter.cs
- ElementHostAutomationPeer.cs
- StrongNameMembershipCondition.cs
- SoapCommonClasses.cs
- IODescriptionAttribute.cs
- DataRow.cs
- EdmTypeAttribute.cs
- ComplexLine.cs
- RepeatInfo.cs
- RowUpdatedEventArgs.cs
- DesignerOptionService.cs
- TextBoxBase.cs
- XmlMtomReader.cs
- SqlEnums.cs
- WebPartCancelEventArgs.cs
- BaseTransportHeaders.cs
- _NetworkingPerfCounters.cs
- MsmqIntegrationSecurityMode.cs
- SmtpFailedRecipientException.cs
- DataGridViewCellLinkedList.cs
- EntitySetDataBindingList.cs
- ProcessHostFactoryHelper.cs
- XPathDocumentIterator.cs
- ProfileGroupSettingsCollection.cs
- FormViewInsertedEventArgs.cs
- TrackingStringDictionary.cs
- VScrollProperties.cs
- Viewport3DAutomationPeer.cs
- KnownIds.cs
- ProfilePropertyMetadata.cs
- StringCollectionMarkupSerializer.cs
- SimplePropertyEntry.cs
- AsyncDataRequest.cs
- IIS7UserPrincipal.cs
- SvcMapFileSerializer.cs
- QuadraticBezierSegment.cs
- FixedTextPointer.cs
- Calendar.cs
- X509SecurityTokenProvider.cs
- ImageListStreamer.cs
- M3DUtil.cs
- DataSourceControl.cs
- PathTooLongException.cs
- URLString.cs
- BuiltInPermissionSets.cs
- WFItemsToSpacerVisibility.cs
- COM2ExtendedUITypeEditor.cs
- BrowserCapabilitiesFactoryBase.cs
- RewritingValidator.cs
- DiscoveryRequestHandler.cs
- SmtpClient.cs
- NotifyParentPropertyAttribute.cs
- RectValueSerializer.cs
- XmlQueryCardinality.cs
- Compiler.cs
- SmtpNetworkElement.cs