Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / RequestTimeoutManager.cs / 3 / RequestTimeoutManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Util; internal class RequestTimeoutManager { private int _requestCount; private DoubleLinkList[] _lists; // partitioned to avoid contention private int _currentList; private int _inProgressLock; // only 1 thread can be cancelling private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 15); // 15 second init precision private Timer _timer; internal RequestTimeoutManager() { // initialize request lists _requestCount = 0; _lists = new DoubleLinkList[13]; for (int i = 0; i < _lists.Length; i++) _lists[i] = new DoubleLinkList(); _currentList = 0; // init lock _inProgressLock = 0; // create the timer #if DBG if (!Debug.IsTagPresent("Timer") || Debug.IsTagEnabled("Timer")) #endif { _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); } } internal void Stop() { // stop the timer if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } while (_inProgressLock != 0) Thread.Sleep(100); // cancel all cancelable requests if (_requestCount > 0) CancelTimedOutRequests(DateTime.UtcNow.AddYears(1)); // } private void TimerCompletionCallback(Object state) { if (_requestCount > 0) CancelTimedOutRequests(DateTime.UtcNow); } private void CancelTimedOutRequests(DateTime now) { // only one thread can be doing it if (Interlocked.CompareExchange(ref _inProgressLock, 1, 0) != 0) return; // collect them all into a separate list with minimal locking ArrayList entries = new ArrayList(_requestCount); // size can change DoubleLinkListEnumerator en; for (int i = 0; i < _lists.Length; i++) { lock (_lists[i]) { en = _lists[i].GetEnumerator(); while (en.MoveNext()) entries.Add(en.GetDoubleLink()); en = null; } } // walk through the collected list to timeout what's needed int n = entries.Count; for (int i = 0; i < n; i++) ((RequestTimeoutEntry)entries[i]).TimeoutIfNeeded(now); // this thread is done -- unlock Interlocked.Exchange(ref _inProgressLock, 0); } internal void Add(HttpContext context) { if (context.TimeoutLink != null) { ((RequestTimeoutEntry)context.TimeoutLink).IncrementCount(); return; } // create new entry RequestTimeoutEntry entry = new RequestTimeoutEntry(context); // add it to the list int i = _currentList++; if (i >= _lists.Length) { i = 0; _currentList = 0; } entry.AddToList(_lists[i]); Interlocked.Increment(ref _requestCount); // update HttpContext context.TimeoutLink = entry; } internal void Remove(HttpContext context) { RequestTimeoutEntry entry = (RequestTimeoutEntry)context.TimeoutLink; // remove from the list if (entry != null) { if( entry.DecrementCount() == 0 ) { entry.RemoveFromList(); Interlocked.Decrement(ref _requestCount); } else { return; } } // update HttpContext context.TimeoutLink = null; } private class RequestTimeoutEntry : DoubleLink { private HttpContext _context; // the request private DoubleLinkList _list; private int _count; internal RequestTimeoutEntry(HttpContext context) { _context = context; _count = 1; } internal void AddToList(DoubleLinkList list) { lock(list) { list.InsertTail(this); _list = list; } } internal void RemoveFromList() { if (_list != null) { lock(_list) { Remove(); _list = null; } } } internal void TimeoutIfNeeded(DateTime now) { Thread thread = _context.MustTimeout(now); if (thread != null) { RemoveFromList(); thread.Abort(new HttpApplication.CancelModuleException(true)); } } internal void IncrementCount() { Interlocked.Increment( ref _count ); } internal int DecrementCount() { return Interlocked.Decrement( ref _count ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Request timeout manager -- implements the request timeout mechanism */ namespace System.Web { using System.Threading; using System.Collections; using System.Web.Util; internal class RequestTimeoutManager { private int _requestCount; private DoubleLinkList[] _lists; // partitioned to avoid contention private int _currentList; private int _inProgressLock; // only 1 thread can be cancelling private readonly TimeSpan _timerPeriod = new TimeSpan(0, 0, 15); // 15 second init precision private Timer _timer; internal RequestTimeoutManager() { // initialize request lists _requestCount = 0; _lists = new DoubleLinkList[13]; for (int i = 0; i < _lists.Length; i++) _lists[i] = new DoubleLinkList(); _currentList = 0; // init lock _inProgressLock = 0; // create the timer #if DBG if (!Debug.IsTagPresent("Timer") || Debug.IsTagEnabled("Timer")) #endif { _timer = new Timer(new TimerCallback(this.TimerCompletionCallback), null, _timerPeriod, _timerPeriod); } } internal void Stop() { // stop the timer if (_timer != null) { ((IDisposable)_timer).Dispose(); _timer = null; } while (_inProgressLock != 0) Thread.Sleep(100); // cancel all cancelable requests if (_requestCount > 0) CancelTimedOutRequests(DateTime.UtcNow.AddYears(1)); // } private void TimerCompletionCallback(Object state) { if (_requestCount > 0) CancelTimedOutRequests(DateTime.UtcNow); } private void CancelTimedOutRequests(DateTime now) { // only one thread can be doing it if (Interlocked.CompareExchange(ref _inProgressLock, 1, 0) != 0) return; // collect them all into a separate list with minimal locking ArrayList entries = new ArrayList(_requestCount); // size can change DoubleLinkListEnumerator en; for (int i = 0; i < _lists.Length; i++) { lock (_lists[i]) { en = _lists[i].GetEnumerator(); while (en.MoveNext()) entries.Add(en.GetDoubleLink()); en = null; } } // walk through the collected list to timeout what's needed int n = entries.Count; for (int i = 0; i < n; i++) ((RequestTimeoutEntry)entries[i]).TimeoutIfNeeded(now); // this thread is done -- unlock Interlocked.Exchange(ref _inProgressLock, 0); } internal void Add(HttpContext context) { if (context.TimeoutLink != null) { ((RequestTimeoutEntry)context.TimeoutLink).IncrementCount(); return; } // create new entry RequestTimeoutEntry entry = new RequestTimeoutEntry(context); // add it to the list int i = _currentList++; if (i >= _lists.Length) { i = 0; _currentList = 0; } entry.AddToList(_lists[i]); Interlocked.Increment(ref _requestCount); // update HttpContext context.TimeoutLink = entry; } internal void Remove(HttpContext context) { RequestTimeoutEntry entry = (RequestTimeoutEntry)context.TimeoutLink; // remove from the list if (entry != null) { if( entry.DecrementCount() == 0 ) { entry.RemoveFromList(); Interlocked.Decrement(ref _requestCount); } else { return; } } // update HttpContext context.TimeoutLink = null; } private class RequestTimeoutEntry : DoubleLink { private HttpContext _context; // the request private DoubleLinkList _list; private int _count; internal RequestTimeoutEntry(HttpContext context) { _context = context; _count = 1; } internal void AddToList(DoubleLinkList list) { lock(list) { list.InsertTail(this); _list = list; } } internal void RemoveFromList() { if (_list != null) { lock(_list) { Remove(); _list = null; } } } internal void TimeoutIfNeeded(DateTime now) { Thread thread = _context.MustTimeout(now); if (thread != null) { RemoveFromList(); thread.Abort(new HttpApplication.CancelModuleException(true)); } } internal void IncrementCount() { Interlocked.Increment( ref _count ); } internal int DecrementCount() { return Interlocked.Decrement( ref _count ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
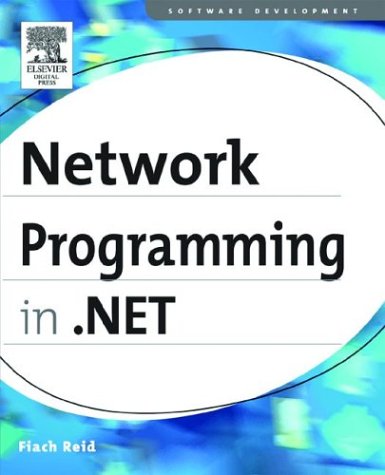
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UInt16Converter.cs
- TransactedBatchingElement.cs
- StructuralType.cs
- ReadOnlyPropertyMetadata.cs
- ConversionContext.cs
- RectConverter.cs
- CallInfo.cs
- FormatConvertedBitmap.cs
- OdbcConnectionPoolProviderInfo.cs
- DetailsViewRowCollection.cs
- DataBindingHandlerAttribute.cs
- PointLightBase.cs
- figurelengthconverter.cs
- TextEditorCopyPaste.cs
- FixedSOMTextRun.cs
- SqlFileStream.cs
- UrlPropertyAttribute.cs
- CodeDirectiveCollection.cs
- Composition.cs
- GridViewCommandEventArgs.cs
- Preprocessor.cs
- SmiEventSink_DeferedProcessing.cs
- CriticalExceptions.cs
- RayMeshGeometry3DHitTestResult.cs
- Comparer.cs
- SendKeys.cs
- UnmanagedMemoryStreamWrapper.cs
- DefaultValueAttribute.cs
- WindowsGraphicsCacheManager.cs
- EntityChangedParams.cs
- httpapplicationstate.cs
- OrderedDictionary.cs
- SqlDataSourceCache.cs
- CacheModeConverter.cs
- ComponentEditorPage.cs
- TextEndOfLine.cs
- StylusButtonCollection.cs
- Contracts.cs
- MasterPageParser.cs
- Fonts.cs
- DataGridRelationshipRow.cs
- ScaleTransform3D.cs
- ProtocolsConfigurationEntry.cs
- ValidationErrorCollection.cs
- CompositeKey.cs
- ProtocolsConfigurationEntry.cs
- Membership.cs
- MultiView.cs
- InkSerializer.cs
- XmlDictionaryReaderQuotas.cs
- BitmapEffectInputData.cs
- MaxValueConverter.cs
- Policy.cs
- SystemIcmpV4Statistics.cs
- ScriptRegistrationManager.cs
- UIntPtr.cs
- SapiGrammar.cs
- ProfileGroupSettings.cs
- PrintPreviewControl.cs
- QueryPageSettingsEventArgs.cs
- OracleCommand.cs
- DataGridAutomationPeer.cs
- bindurihelper.cs
- Models.cs
- EdmValidator.cs
- X509CertificateCollection.cs
- AutomationPatternInfo.cs
- KnownTypesHelper.cs
- TextServicesDisplayAttribute.cs
- FontUnitConverter.cs
- ListViewItem.cs
- Missing.cs
- KeyedQueue.cs
- UrlMapping.cs
- DataKey.cs
- DecoderReplacementFallback.cs
- MissingMemberException.cs
- LayoutManager.cs
- SQLResource.cs
- XmlSecureResolver.cs
- TemplateControl.cs
- Crypto.cs
- UnsafeNativeMethods.cs
- FacetDescription.cs
- HMACMD5.cs
- Renderer.cs
- TcpWorkerProcess.cs
- Soap12ServerProtocol.cs
- MenuEventArgs.cs
- ContainerParagraph.cs
- XamlNamespaceHelper.cs
- LightweightCodeGenerator.cs
- CharacterBuffer.cs
- FixedSOMLineRanges.cs
- SafeFileMapViewHandle.cs
- IsolatedStorageFilePermission.cs
- BuilderInfo.cs
- DisplayClaim.cs
- SmiEventSink_Default.cs
- ColumnReorderedEventArgs.cs