Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Brushes.cs / 1 / Brushes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Diagnostics; using System; using System.Drawing; ////// /// Brushes for all the standard colors. /// public sealed class Brushes { static readonly object TransparentKey = new object(); static readonly object AliceBlueKey = new object(); static readonly object AntiqueWhiteKey = new object(); static readonly object AquaKey = new object(); static readonly object AquamarineKey = new object(); static readonly object AzureKey = new object(); static readonly object BeigeKey = new object(); static readonly object BisqueKey = new object(); static readonly object BlackKey = new object(); static readonly object BlanchedAlmondKey = new object(); static readonly object BlueKey = new object(); static readonly object BlueVioletKey = new object(); static readonly object BrownKey = new object(); static readonly object BurlyWoodKey = new object(); static readonly object CadetBlueKey = new object(); static readonly object ChartreuseKey = new object(); static readonly object ChocolateKey = new object(); static readonly object ChoralKey = new object(); static readonly object CornflowerBlueKey = new object(); static readonly object CornsilkKey = new object(); static readonly object CrimsonKey = new object(); static readonly object CyanKey = new object(); static readonly object DarkBlueKey = new object(); static readonly object DarkCyanKey = new object(); static readonly object DarkGoldenrodKey = new object(); static readonly object DarkGrayKey = new object(); static readonly object DarkGreenKey = new object(); static readonly object DarkKhakiKey = new object(); static readonly object DarkMagentaKey = new object(); static readonly object DarkOliveGreenKey = new object(); static readonly object DarkOrangeKey = new object(); static readonly object DarkOrchidKey = new object(); static readonly object DarkRedKey = new object(); static readonly object DarkSalmonKey = new object(); static readonly object DarkSeaGreenKey = new object(); static readonly object DarkSlateBlueKey = new object(); static readonly object DarkSlateGrayKey = new object(); static readonly object DarkTurquoiseKey = new object(); static readonly object DarkVioletKey = new object(); static readonly object DeepPinkKey = new object(); static readonly object DeepSkyBlueKey = new object(); static readonly object DimGrayKey = new object(); static readonly object DodgerBlueKey = new object(); static readonly object FirebrickKey = new object(); static readonly object FloralWhiteKey = new object(); static readonly object ForestGreenKey = new object(); static readonly object FuchiaKey = new object(); static readonly object GainsboroKey = new object(); static readonly object GhostWhiteKey = new object(); static readonly object GoldKey = new object(); static readonly object GoldenrodKey = new object(); static readonly object GrayKey = new object(); static readonly object GreenKey = new object(); static readonly object GreenYellowKey = new object(); static readonly object HoneydewKey = new object(); static readonly object HotPinkKey = new object(); static readonly object IndianRedKey = new object(); static readonly object IndigoKey = new object(); static readonly object IvoryKey = new object(); static readonly object KhakiKey = new object(); static readonly object LavenderKey = new object(); static readonly object LavenderBlushKey = new object(); static readonly object LawnGreenKey = new object(); static readonly object LemonChiffonKey = new object(); static readonly object LightBlueKey = new object(); static readonly object LightCoralKey = new object(); static readonly object LightCyanKey = new object(); static readonly object LightGoldenrodYellowKey = new object(); static readonly object LightGreenKey = new object(); static readonly object LightGrayKey = new object(); static readonly object LightPinkKey = new object(); static readonly object LightSalmonKey = new object(); static readonly object LightSeaGreenKey = new object(); static readonly object LightSkyBlueKey = new object(); static readonly object LightSlateGrayKey = new object(); static readonly object LightSteelBlueKey = new object(); static readonly object LightYellowKey = new object(); static readonly object LimeKey = new object(); static readonly object LimeGreenKey = new object(); static readonly object LinenKey = new object(); static readonly object MagentaKey = new object(); static readonly object MaroonKey = new object(); static readonly object MediumAquamarineKey = new object(); static readonly object MediumBlueKey = new object(); static readonly object MediumOrchidKey = new object(); static readonly object MediumPurpleKey = new object(); static readonly object MediumSeaGreenKey = new object(); static readonly object MediumSlateBlueKey = new object(); static readonly object MediumSpringGreenKey = new object(); static readonly object MediumTurquoiseKey = new object(); static readonly object MediumVioletRedKey = new object(); static readonly object MidnightBlueKey = new object(); static readonly object MintCreamKey = new object(); static readonly object MistyRoseKey = new object(); static readonly object MoccasinKey = new object(); static readonly object NavajoWhiteKey = new object(); static readonly object NavyKey = new object(); static readonly object OldLaceKey = new object(); static readonly object OliveKey = new object(); static readonly object OliveDrabKey = new object(); static readonly object OrangeKey = new object(); static readonly object OrangeRedKey = new object(); static readonly object OrchidKey = new object(); static readonly object PaleGoldenrodKey = new object(); static readonly object PaleGreenKey = new object(); static readonly object PaleTurquoiseKey = new object(); static readonly object PaleVioletRedKey = new object(); static readonly object PapayaWhipKey = new object(); static readonly object PeachPuffKey = new object(); static readonly object PeruKey = new object(); static readonly object PinkKey = new object(); static readonly object PlumKey = new object(); static readonly object PowderBlueKey = new object(); static readonly object PurpleKey = new object(); static readonly object RedKey = new object(); static readonly object RosyBrownKey = new object(); static readonly object RoyalBlueKey = new object(); static readonly object SaddleBrownKey = new object(); static readonly object SalmonKey = new object(); static readonly object SandyBrownKey = new object(); static readonly object SeaGreenKey = new object(); static readonly object SeaShellKey = new object(); static readonly object SiennaKey = new object(); static readonly object SilverKey = new object(); static readonly object SkyBlueKey = new object(); static readonly object SlateBlueKey = new object(); static readonly object SlateGrayKey = new object(); static readonly object SnowKey = new object(); static readonly object SpringGreenKey = new object(); static readonly object SteelBlueKey = new object(); static readonly object TanKey = new object(); static readonly object TealKey = new object(); static readonly object ThistleKey = new object(); static readonly object TomatoKey = new object(); static readonly object TurquoiseKey = new object(); static readonly object VioletKey = new object(); static readonly object WheatKey = new object(); static readonly object WhiteKey = new object(); static readonly object WhiteSmokeKey = new object(); static readonly object YellowKey = new object(); static readonly object YellowGreenKey = new object(); private Brushes() { } ////// /// A transparent brush. /// public static Brush Transparent { get { Brush transparent = (Brush)SafeNativeMethods.Gdip.ThreadData[TransparentKey]; if (transparent == null) { transparent = new SolidBrush(Color.Transparent); SafeNativeMethods.Gdip.ThreadData[TransparentKey] = transparent; } return transparent; } } ////// /// A brush of the given color. /// public static Brush AliceBlue { get { Brush aliceBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[AliceBlueKey]; if (aliceBlue == null) { aliceBlue = new SolidBrush(Color.AliceBlue); SafeNativeMethods.Gdip.ThreadData[AliceBlueKey] = aliceBlue; } return aliceBlue; } } ////// /// A brush of the given color. /// public static Brush AntiqueWhite { get { Brush antiqueWhite = (Brush)SafeNativeMethods.Gdip.ThreadData[AntiqueWhiteKey]; if (antiqueWhite == null) { antiqueWhite = new SolidBrush(Color.AntiqueWhite); SafeNativeMethods.Gdip.ThreadData[AntiqueWhiteKey] = antiqueWhite; } return antiqueWhite; } } ////// /// A brush of the given color. /// public static Brush Aqua { get { Brush aqua = (Brush)SafeNativeMethods.Gdip.ThreadData[AquaKey]; if (aqua == null) { aqua = new SolidBrush(Color.Aqua); SafeNativeMethods.Gdip.ThreadData[AquaKey] = aqua; } return aqua; } } ////// /// A brush of the given color. /// public static Brush Aquamarine { get { Brush aquamarine = (Brush)SafeNativeMethods.Gdip.ThreadData[AquamarineKey]; if (aquamarine == null) { aquamarine = new SolidBrush(Color.Aquamarine); SafeNativeMethods.Gdip.ThreadData[AquamarineKey] = aquamarine; } return aquamarine; } } ////// /// A brush of the given color. /// public static Brush Azure { get { Brush azure = (Brush)SafeNativeMethods.Gdip.ThreadData[AzureKey]; if (azure == null) { azure = new SolidBrush(Color.Azure); SafeNativeMethods.Gdip.ThreadData[AzureKey] = azure; } return azure; } } ////// /// A brush of the given color. /// public static Brush Beige { get { Brush beige = (Brush)SafeNativeMethods.Gdip.ThreadData[BeigeKey]; if (beige == null) { beige = new SolidBrush(Color.Beige); SafeNativeMethods.Gdip.ThreadData[BeigeKey] = beige; } return beige; } } ////// /// A brush of the given color. /// public static Brush Bisque { get { Brush bisque = (Brush)SafeNativeMethods.Gdip.ThreadData[BisqueKey]; if (bisque == null) { bisque = new SolidBrush(Color.Bisque); SafeNativeMethods.Gdip.ThreadData[BisqueKey] = bisque; } return bisque; } } ////// /// A brush of the given color. /// public static Brush Black { get { Brush black = (Brush)SafeNativeMethods.Gdip.ThreadData[BlackKey]; if (black == null) { black = new SolidBrush(Color.Black); SafeNativeMethods.Gdip.ThreadData[BlackKey] = black; } return black; } } ////// /// A brush of the given color. /// public static Brush BlanchedAlmond { get { Brush blanchedAlmond = (Brush)SafeNativeMethods.Gdip.ThreadData[BlanchedAlmondKey]; if (blanchedAlmond == null) { blanchedAlmond = new SolidBrush(Color.BlanchedAlmond); SafeNativeMethods.Gdip.ThreadData[BlanchedAlmondKey] = blanchedAlmond; } return blanchedAlmond; } } ////// /// A brush of the given color. /// public static Brush Blue { get { Brush blue = (Brush)SafeNativeMethods.Gdip.ThreadData[BlueKey]; if (blue == null) { blue = new SolidBrush(Color.Blue); SafeNativeMethods.Gdip.ThreadData[BlueKey] = blue; } return blue; } } ////// /// A brush of the given color. /// public static Brush BlueViolet { get { Brush blueViolet = (Brush)SafeNativeMethods.Gdip.ThreadData[BlueVioletKey]; if (blueViolet == null) { blueViolet = new SolidBrush(Color.BlueViolet); SafeNativeMethods.Gdip.ThreadData[BlueVioletKey] = blueViolet; } return blueViolet; } } ////// /// A brush of the given color. /// public static Brush Brown { get { Brush brown = (Brush)SafeNativeMethods.Gdip.ThreadData[BrownKey]; if (brown == null) { brown = new SolidBrush(Color.Brown); SafeNativeMethods.Gdip.ThreadData[BrownKey] = brown; } return brown; } } ////// /// A brush of the given color. /// public static Brush BurlyWood { get { Brush burlyWood = (Brush)SafeNativeMethods.Gdip.ThreadData[BurlyWoodKey]; if (burlyWood == null) { burlyWood = new SolidBrush(Color.BurlyWood); SafeNativeMethods.Gdip.ThreadData[BurlyWoodKey] = burlyWood; } return burlyWood; } } ////// /// A brush of the given color. /// public static Brush CadetBlue { get { Brush cadetBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[CadetBlueKey]; if (cadetBlue == null) { cadetBlue = new SolidBrush(Color.CadetBlue); SafeNativeMethods.Gdip.ThreadData[CadetBlueKey] = cadetBlue; } return cadetBlue; } } ////// /// A brush of the given color. /// public static Brush Chartreuse { get { Brush chartreuse = (Brush)SafeNativeMethods.Gdip.ThreadData[ChartreuseKey]; if (chartreuse == null) { chartreuse = new SolidBrush(Color.Chartreuse); SafeNativeMethods.Gdip.ThreadData[ChartreuseKey] = chartreuse; } return chartreuse; } } ////// /// A brush of the given color. /// public static Brush Chocolate { get { Brush chocolate = (Brush)SafeNativeMethods.Gdip.ThreadData[ChocolateKey]; if (chocolate == null) { chocolate = new SolidBrush(Color.Chocolate); SafeNativeMethods.Gdip.ThreadData[ChocolateKey] = chocolate; } return chocolate; } } ////// /// A brush of the given color. /// public static Brush Coral { get { Brush choral = (Brush)SafeNativeMethods.Gdip.ThreadData[ChoralKey]; if (choral == null) { choral = new SolidBrush(Color.Coral); SafeNativeMethods.Gdip.ThreadData[ChoralKey] = choral; } return choral; } } ////// /// A brush of the given color. /// public static Brush CornflowerBlue { get { Brush cornflowerBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[CornflowerBlueKey]; if (cornflowerBlue== null) { cornflowerBlue = new SolidBrush(Color.CornflowerBlue); SafeNativeMethods.Gdip.ThreadData[CornflowerBlueKey] = cornflowerBlue; } return cornflowerBlue; } } ////// /// A brush of the given color. /// public static Brush Cornsilk { get { Brush cornsilk = (Brush)SafeNativeMethods.Gdip.ThreadData[CornsilkKey]; if (cornsilk == null) { cornsilk = new SolidBrush(Color.Cornsilk); SafeNativeMethods.Gdip.ThreadData[CornsilkKey] = cornsilk; } return cornsilk; } } ////// /// A brush of the given color. /// public static Brush Crimson { get { Brush crimson = (Brush)SafeNativeMethods.Gdip.ThreadData[CrimsonKey]; if (crimson == null) { crimson = new SolidBrush(Color.Crimson); SafeNativeMethods.Gdip.ThreadData[CrimsonKey] = crimson; } return crimson; } } ////// /// A brush of the given color. /// public static Brush Cyan { get { Brush cyan = (Brush)SafeNativeMethods.Gdip.ThreadData[CyanKey]; if (cyan == null) { cyan = new SolidBrush(Color.Cyan); SafeNativeMethods.Gdip.ThreadData[CyanKey] = cyan; } return cyan; } } ////// /// A brush of the given color. /// public static Brush DarkBlue { get { Brush darkBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkBlueKey]; if (darkBlue == null) { darkBlue = new SolidBrush(Color.DarkBlue); SafeNativeMethods.Gdip.ThreadData[DarkBlueKey] = darkBlue; } return darkBlue; } } ////// /// A brush of the given color. /// public static Brush DarkCyan { get { Brush darkCyan = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkCyanKey]; if (darkCyan == null) { darkCyan = new SolidBrush(Color.DarkCyan); SafeNativeMethods.Gdip.ThreadData[DarkCyanKey] = darkCyan; } return darkCyan; } } ////// /// A brush of the given color. /// public static Brush DarkGoldenrod { get { Brush darkGoldenrod = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkGoldenrodKey]; if (darkGoldenrod == null) { darkGoldenrod = new SolidBrush(Color.DarkGoldenrod); SafeNativeMethods.Gdip.ThreadData[DarkGoldenrodKey] = darkGoldenrod; } return darkGoldenrod; } } ////// /// A brush of the given color. /// public static Brush DarkGray { get { Brush darkGray = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkGrayKey]; if (darkGray == null) { darkGray = new SolidBrush(Color.DarkGray); SafeNativeMethods.Gdip.ThreadData[DarkGrayKey] = darkGray; } return darkGray; } } ////// /// A brush of the given color. /// public static Brush DarkGreen { get { Brush darkGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkGreenKey]; if (darkGreen == null) { darkGreen = new SolidBrush(Color.DarkGreen); SafeNativeMethods.Gdip.ThreadData[DarkGreenKey] = darkGreen; } return darkGreen; } } ////// /// A brush of the given color. /// public static Brush DarkKhaki { get { Brush darkKhaki = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkKhakiKey]; if (darkKhaki == null) { darkKhaki = new SolidBrush(Color.DarkKhaki); SafeNativeMethods.Gdip.ThreadData[DarkKhakiKey] = darkKhaki; } return darkKhaki; } } ////// /// A brush of the given color. /// public static Brush DarkMagenta { get { Brush darkMagenta = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkMagentaKey]; if (darkMagenta == null) { darkMagenta = new SolidBrush(Color.DarkMagenta); SafeNativeMethods.Gdip.ThreadData[DarkMagentaKey] = darkMagenta; } return darkMagenta; } } ////// /// A brush of the given color. /// public static Brush DarkOliveGreen { get { Brush darkOliveGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkOliveGreenKey]; if (darkOliveGreen == null) { darkOliveGreen = new SolidBrush(Color.DarkOliveGreen); SafeNativeMethods.Gdip.ThreadData[DarkOliveGreenKey] = darkOliveGreen; } return darkOliveGreen; } } ////// /// A brush of the given color. /// public static Brush DarkOrange { get { Brush darkOrange = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkOrangeKey]; if (darkOrange == null) { darkOrange = new SolidBrush(Color.DarkOrange); SafeNativeMethods.Gdip.ThreadData[DarkOrangeKey] = darkOrange; } return darkOrange; } } ////// /// A brush of the given color. /// public static Brush DarkOrchid { get { Brush darkOrchid = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkOrchidKey]; if (darkOrchid == null) { darkOrchid = new SolidBrush(Color.DarkOrchid); SafeNativeMethods.Gdip.ThreadData[DarkOrchidKey] = darkOrchid; } return darkOrchid; } } ////// /// A brush of the given color. /// public static Brush DarkRed { get { Brush darkRed = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkRedKey]; if (darkRed == null) { darkRed = new SolidBrush(Color.DarkRed); SafeNativeMethods.Gdip.ThreadData[DarkRedKey] = darkRed; } return darkRed; } } ////// /// A brush of the given color. /// public static Brush DarkSalmon { get { Brush darkSalmon = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkSalmonKey]; if (darkSalmon == null) { darkSalmon = new SolidBrush(Color.DarkSalmon); SafeNativeMethods.Gdip.ThreadData[DarkSalmonKey] = darkSalmon; } return darkSalmon; } } ////// /// A brush of the given color. /// public static Brush DarkSeaGreen { get { Brush darkSeaGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkSeaGreenKey]; if (darkSeaGreen == null) { darkSeaGreen = new SolidBrush(Color.DarkSeaGreen); SafeNativeMethods.Gdip.ThreadData[DarkSeaGreenKey] = darkSeaGreen; } return darkSeaGreen; } } ////// /// A brush of the given color. /// public static Brush DarkSlateBlue { get { Brush darkSlateBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkSlateBlueKey]; if (darkSlateBlue == null) { darkSlateBlue = new SolidBrush(Color.DarkSlateBlue); SafeNativeMethods.Gdip.ThreadData[DarkSlateBlueKey] = darkSlateBlue; } return darkSlateBlue; } } ////// /// A brush of the given color. /// public static Brush DarkSlateGray { get { Brush darkSlateGray = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkSlateGrayKey]; if (darkSlateGray == null) { darkSlateGray = new SolidBrush(Color.DarkSlateGray); SafeNativeMethods.Gdip.ThreadData[DarkSlateGrayKey] = darkSlateGray; } return darkSlateGray; } } ////// /// A brush of the given color. /// public static Brush DarkTurquoise { get { Brush darkTurquoise = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkTurquoiseKey]; if (darkTurquoise == null) { darkTurquoise = new SolidBrush(Color.DarkTurquoise); SafeNativeMethods.Gdip.ThreadData[DarkTurquoiseKey] = darkTurquoise; } return darkTurquoise; } } ////// /// A brush of the given color. /// public static Brush DarkViolet { get { Brush darkViolet = (Brush)SafeNativeMethods.Gdip.ThreadData[DarkVioletKey]; if (darkViolet == null) { darkViolet = new SolidBrush(Color.DarkViolet); SafeNativeMethods.Gdip.ThreadData[DarkVioletKey] = darkViolet; } return darkViolet; } } ////// /// A brush of the given color. /// public static Brush DeepPink { get { Brush deepPink = (Brush)SafeNativeMethods.Gdip.ThreadData[DeepPinkKey]; if (deepPink == null) { deepPink = new SolidBrush(Color.DeepPink); SafeNativeMethods.Gdip.ThreadData[DeepPinkKey] = deepPink; } return deepPink; } } ////// /// A brush of the given color. /// public static Brush DeepSkyBlue { get { Brush deepSkyBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[DeepSkyBlueKey]; if (deepSkyBlue == null) { deepSkyBlue = new SolidBrush(Color.DeepSkyBlue); SafeNativeMethods.Gdip.ThreadData[DeepSkyBlueKey] = deepSkyBlue; } return deepSkyBlue; } } ////// /// A brush of the given color. /// public static Brush DimGray { get { Brush dimGray = (Brush)SafeNativeMethods.Gdip.ThreadData[DimGrayKey]; if (dimGray == null) { dimGray = new SolidBrush(Color.DimGray); SafeNativeMethods.Gdip.ThreadData[DimGrayKey] = dimGray; } return dimGray; } } ////// /// A brush of the given color. /// public static Brush DodgerBlue { get { Brush dodgerBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[DodgerBlueKey]; if (dodgerBlue == null) { dodgerBlue = new SolidBrush(Color.DodgerBlue); SafeNativeMethods.Gdip.ThreadData[DodgerBlueKey] = dodgerBlue; } return dodgerBlue; } } ////// /// A brush of the given color. /// public static Brush Firebrick { get { Brush firebrick = (Brush)SafeNativeMethods.Gdip.ThreadData[FirebrickKey]; if (firebrick == null) { firebrick = new SolidBrush(Color.Firebrick); SafeNativeMethods.Gdip.ThreadData[FirebrickKey] = firebrick; } return firebrick; } } ////// /// A brush of the given color. /// public static Brush FloralWhite { get { Brush floralWhite = (Brush)SafeNativeMethods.Gdip.ThreadData[FloralWhiteKey]; if (floralWhite == null) { floralWhite = new SolidBrush(Color.FloralWhite); SafeNativeMethods.Gdip.ThreadData[FloralWhiteKey] = floralWhite; } return floralWhite; } } ////// /// A brush of the given color. /// public static Brush ForestGreen { get { Brush forestGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[ForestGreenKey]; if (forestGreen == null) { forestGreen = new SolidBrush(Color.ForestGreen); SafeNativeMethods.Gdip.ThreadData[ForestGreenKey] = forestGreen; } return forestGreen; } } ////// /// A brush of the given color. /// public static Brush Fuchsia { get { Brush fuchia = (Brush)SafeNativeMethods.Gdip.ThreadData[FuchiaKey]; if (fuchia == null) { fuchia = new SolidBrush(Color.Fuchsia); SafeNativeMethods.Gdip.ThreadData[FuchiaKey] = fuchia; } return fuchia; } } ////// /// A brush of the given color. /// public static Brush Gainsboro { get { Brush gainsboro = (Brush)SafeNativeMethods.Gdip.ThreadData[GainsboroKey]; if (gainsboro == null) { gainsboro = new SolidBrush(Color.Gainsboro); SafeNativeMethods.Gdip.ThreadData[GainsboroKey] = gainsboro; } return gainsboro; } } ////// /// A brush of the given color. /// public static Brush GhostWhite { get { Brush ghostWhite = (Brush)SafeNativeMethods.Gdip.ThreadData[GhostWhiteKey]; if (ghostWhite == null) { ghostWhite = new SolidBrush(Color.GhostWhite); SafeNativeMethods.Gdip.ThreadData[GhostWhiteKey] = ghostWhite; } return ghostWhite; } } ////// /// A brush of the given color. /// public static Brush Gold { get { Brush gold = (Brush)SafeNativeMethods.Gdip.ThreadData[GoldKey]; if (gold == null) { gold = new SolidBrush(Color.Gold); SafeNativeMethods.Gdip.ThreadData[GoldKey] = gold; } return gold; } } ////// /// A brush of the given color. /// public static Brush Goldenrod { get { Brush goldenrod = (Brush)SafeNativeMethods.Gdip.ThreadData[GoldenrodKey]; if (goldenrod == null) { goldenrod = new SolidBrush(Color.Goldenrod); SafeNativeMethods.Gdip.ThreadData[GoldenrodKey] = goldenrod; } return goldenrod; } } ////// /// A brush of the given color. /// public static Brush Gray { get { Brush gray = (Brush)SafeNativeMethods.Gdip.ThreadData[GrayKey]; if (gray == null) { gray = new SolidBrush(Color.Gray); SafeNativeMethods.Gdip.ThreadData[GrayKey] = gray; } return gray; } } ////// /// A brush of the given color. /// public static Brush Green { get { Brush green = (Brush)SafeNativeMethods.Gdip.ThreadData[GreenKey]; if (green == null) { green = new SolidBrush(Color.Green); SafeNativeMethods.Gdip.ThreadData[GreenKey] = green; } return green; } } ////// /// A brush of the given color. /// public static Brush GreenYellow { get { Brush greenYellow = (Brush)SafeNativeMethods.Gdip.ThreadData[GreenYellowKey]; if (greenYellow == null) { greenYellow = new SolidBrush(Color.GreenYellow); SafeNativeMethods.Gdip.ThreadData[GreenYellowKey] = greenYellow; } return greenYellow; } } ////// /// A brush of the given color. /// public static Brush Honeydew { get { Brush honeydew = (Brush)SafeNativeMethods.Gdip.ThreadData[HoneydewKey]; if (honeydew == null) { honeydew = new SolidBrush(Color.Honeydew); SafeNativeMethods.Gdip.ThreadData[HoneydewKey] = honeydew; } return honeydew; } } ////// /// A brush of the given color. /// public static Brush HotPink { get { Brush hotPink = (Brush)SafeNativeMethods.Gdip.ThreadData[HotPinkKey]; if (hotPink == null) { hotPink = new SolidBrush(Color.HotPink); SafeNativeMethods.Gdip.ThreadData[HotPinkKey] = hotPink; } return hotPink; } } ////// /// A brush of the given color. /// public static Brush IndianRed { get { Brush indianRed = (Brush)SafeNativeMethods.Gdip.ThreadData[IndianRedKey]; if (indianRed == null) { indianRed = new SolidBrush(Color.IndianRed); SafeNativeMethods.Gdip.ThreadData[IndianRedKey] = indianRed; } return indianRed; } } ////// /// A brush of the given color. /// public static Brush Indigo { get { Brush indigo = (Brush)SafeNativeMethods.Gdip.ThreadData[IndigoKey]; if (indigo == null) { indigo = new SolidBrush(Color.Indigo); SafeNativeMethods.Gdip.ThreadData[IndigoKey] = indigo; } return indigo; } } ////// /// A brush of the given color. /// public static Brush Ivory { get { Brush ivory = (Brush)SafeNativeMethods.Gdip.ThreadData[IvoryKey]; if (ivory == null) { ivory = new SolidBrush(Color.Ivory); SafeNativeMethods.Gdip.ThreadData[IvoryKey] = ivory; } return ivory; } } ////// /// A brush of the given color. /// public static Brush Khaki { get { Brush khaki = (Brush)SafeNativeMethods.Gdip.ThreadData[KhakiKey]; if (khaki == null) { khaki = new SolidBrush(Color.Khaki); SafeNativeMethods.Gdip.ThreadData[KhakiKey] = khaki; } return khaki; } } ////// /// A brush of the given color. /// public static Brush Lavender { get { Brush lavender = (Brush)SafeNativeMethods.Gdip.ThreadData[LavenderKey]; if (lavender == null) { lavender = new SolidBrush(Color.Lavender); SafeNativeMethods.Gdip.ThreadData[LavenderKey] = lavender; } return lavender; } } ////// /// A brush of the given color. /// public static Brush LavenderBlush { get { Brush lavenderBlush = (Brush)SafeNativeMethods.Gdip.ThreadData[LavenderBlushKey]; if (lavenderBlush == null) { lavenderBlush = new SolidBrush(Color.LavenderBlush); SafeNativeMethods.Gdip.ThreadData[LavenderBlushKey] = lavenderBlush; } return lavenderBlush; } } ////// /// A brush of the given color. /// public static Brush LawnGreen { get { Brush lawnGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[LawnGreenKey]; if (lawnGreen == null) { lawnGreen = new SolidBrush(Color.LawnGreen); SafeNativeMethods.Gdip.ThreadData[LawnGreenKey] = lawnGreen; } return lawnGreen; } } ////// /// A brush of the given color. /// public static Brush LemonChiffon { get { Brush lemonChiffon = (Brush)SafeNativeMethods.Gdip.ThreadData[LemonChiffonKey]; if (lemonChiffon == null) { lemonChiffon = new SolidBrush(Color.LemonChiffon); SafeNativeMethods.Gdip.ThreadData[LemonChiffonKey] = lemonChiffon; } return lemonChiffon; } } ////// /// A brush of the given color. /// public static Brush LightBlue { get { Brush lightBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[LightBlueKey]; if (lightBlue == null) { lightBlue = new SolidBrush(Color.LightBlue); SafeNativeMethods.Gdip.ThreadData[LightBlueKey] = lightBlue; } return lightBlue; } } ////// /// A brush of the given color. /// public static Brush LightCoral { get { Brush lightCoral = (Brush)SafeNativeMethods.Gdip.ThreadData[LightCoralKey]; if (lightCoral == null) { lightCoral = new SolidBrush(Color.LightCoral); SafeNativeMethods.Gdip.ThreadData[LightCoralKey] = lightCoral; } return lightCoral; } } ////// /// A brush of the given color. /// public static Brush LightCyan { get { Brush lightCyan = (Brush)SafeNativeMethods.Gdip.ThreadData[LightCyanKey]; if (lightCyan == null) { lightCyan = new SolidBrush(Color.LightCyan); SafeNativeMethods.Gdip.ThreadData[LightCyanKey] = lightCyan; } return lightCyan; } } ////// /// A brush of the given color. /// public static Brush LightGoldenrodYellow { get { Brush lightGoldenrodYellow = (Brush)SafeNativeMethods.Gdip.ThreadData[LightGoldenrodYellowKey]; if (lightGoldenrodYellow == null) { lightGoldenrodYellow = new SolidBrush(Color.LightGoldenrodYellow); SafeNativeMethods.Gdip.ThreadData[LightGoldenrodYellowKey] = lightGoldenrodYellow; } return lightGoldenrodYellow; } } ////// /// A brush of the given color. /// public static Brush LightGreen { get { Brush lightGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[LightGreenKey]; if (lightGreen == null) { lightGreen = new SolidBrush(Color.LightGreen); SafeNativeMethods.Gdip.ThreadData[LightGreenKey] = lightGreen; } return lightGreen; } } ////// /// A brush of the given color. /// public static Brush LightGray { get { Brush lightGray = (Brush)SafeNativeMethods.Gdip.ThreadData[LightGrayKey]; if (lightGray == null) { lightGray = new SolidBrush(Color.LightGray); SafeNativeMethods.Gdip.ThreadData[LightGrayKey] = lightGray; } return lightGray; } } ////// /// A brush of the given color. /// public static Brush LightPink { get { Brush lightPink = (Brush)SafeNativeMethods.Gdip.ThreadData[LightPinkKey]; if (lightPink == null) { lightPink = new SolidBrush(Color.LightPink); SafeNativeMethods.Gdip.ThreadData[LightPinkKey] = lightPink; } return lightPink; } } ////// /// A brush of the given color. /// public static Brush LightSalmon { get { Brush lightSalmon = (Brush)SafeNativeMethods.Gdip.ThreadData[LightSalmonKey]; if (lightSalmon == null) { lightSalmon = new SolidBrush(Color.LightSalmon); SafeNativeMethods.Gdip.ThreadData[LightSalmonKey] = lightSalmon; } return lightSalmon; } } ////// /// A brush of the given color. /// public static Brush LightSeaGreen { get { Brush lightSeaGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[LightSeaGreenKey]; if (lightSeaGreen == null) { lightSeaGreen = new SolidBrush(Color.LightSeaGreen); SafeNativeMethods.Gdip.ThreadData[LightSeaGreenKey] = lightSeaGreen; } return lightSeaGreen; } } ////// /// A brush of the given color. /// public static Brush LightSkyBlue { get { Brush lightSkyBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[LightSkyBlueKey]; if (lightSkyBlue == null) { lightSkyBlue = new SolidBrush(Color.LightSkyBlue); SafeNativeMethods.Gdip.ThreadData[LightSkyBlueKey] = lightSkyBlue; } return lightSkyBlue; } } ////// /// A brush of the given color. /// public static Brush LightSlateGray { get { Brush lightSlateGray = (Brush)SafeNativeMethods.Gdip.ThreadData[LightSlateGrayKey]; if (lightSlateGray == null) { lightSlateGray = new SolidBrush(Color.LightSlateGray); SafeNativeMethods.Gdip.ThreadData[LightSlateGrayKey] = lightSlateGray; } return lightSlateGray; } } ////// /// A brush of the given color. /// public static Brush LightSteelBlue { get { Brush lightSteelBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[LightSteelBlueKey]; if (lightSteelBlue == null) { lightSteelBlue = new SolidBrush(Color.LightSteelBlue); SafeNativeMethods.Gdip.ThreadData[LightSteelBlueKey] = lightSteelBlue; } return lightSteelBlue; } } ////// /// A brush of the given color. /// public static Brush LightYellow { get { Brush lightYellow = (Brush)SafeNativeMethods.Gdip.ThreadData[LightYellowKey]; if (lightYellow == null) { lightYellow = new SolidBrush(Color.LightYellow); SafeNativeMethods.Gdip.ThreadData[LightYellowKey] = lightYellow; } return lightYellow; } } ////// /// A brush of the given color. /// public static Brush Lime { get { Brush lime = (Brush)SafeNativeMethods.Gdip.ThreadData[LimeKey]; if (lime == null) { lime = new SolidBrush(Color.Lime); SafeNativeMethods.Gdip.ThreadData[LimeKey] = lime; } return lime; } } ////// /// A brush of the given color. /// public static Brush LimeGreen { get { Brush limeGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[LimeGreenKey]; if (limeGreen == null) { limeGreen = new SolidBrush(Color.LimeGreen); SafeNativeMethods.Gdip.ThreadData[LimeGreenKey] = limeGreen; } return limeGreen; } } ////// /// A brush of the given color. /// public static Brush Linen { get { Brush linen = (Brush)SafeNativeMethods.Gdip.ThreadData[LinenKey]; if (linen == null) { linen = new SolidBrush(Color.Linen); SafeNativeMethods.Gdip.ThreadData[LinenKey] = linen; } return linen; } } ////// /// A brush of the given color. /// public static Brush Magenta { get { Brush magenta = (Brush)SafeNativeMethods.Gdip.ThreadData[MagentaKey]; if (magenta == null) { magenta = new SolidBrush(Color.Magenta); SafeNativeMethods.Gdip.ThreadData[MagentaKey] = magenta; } return magenta; } } ////// /// A brush of the given color. /// public static Brush Maroon { get { Brush maroon = (Brush)SafeNativeMethods.Gdip.ThreadData[MaroonKey]; if (maroon == null) { maroon = new SolidBrush(Color.Maroon); SafeNativeMethods.Gdip.ThreadData[MaroonKey] = maroon; } return maroon; } } ////// /// A brush of the given color. /// public static Brush MediumAquamarine { get { Brush mediumAquamarine = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumAquamarineKey]; if (mediumAquamarine == null) { mediumAquamarine = new SolidBrush(Color.MediumAquamarine); SafeNativeMethods.Gdip.ThreadData[MediumAquamarineKey] = mediumAquamarine; } return mediumAquamarine; } } ////// /// A brush of the given color. /// public static Brush MediumBlue { get { Brush mediumBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumBlueKey]; if (mediumBlue == null) { mediumBlue = new SolidBrush(Color.MediumBlue); SafeNativeMethods.Gdip.ThreadData[MediumBlueKey] = mediumBlue; } return mediumBlue; } } ////// /// A brush of the given color. /// public static Brush MediumOrchid { get { Brush mediumOrchid = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumOrchidKey]; if (mediumOrchid == null) { mediumOrchid = new SolidBrush(Color.MediumOrchid); SafeNativeMethods.Gdip.ThreadData[MediumOrchidKey] = mediumOrchid; } return mediumOrchid; } } ////// /// A brush of the given color. /// public static Brush MediumPurple { get { Brush mediumPurple = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumPurpleKey]; if (mediumPurple == null) { mediumPurple = new SolidBrush(Color.MediumPurple); SafeNativeMethods.Gdip.ThreadData[MediumPurpleKey] = mediumPurple; } return mediumPurple; } } ////// /// A brush of the given color. /// public static Brush MediumSeaGreen { get { Brush mediumSeaGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumSeaGreenKey]; if (mediumSeaGreen == null) { mediumSeaGreen = new SolidBrush(Color.MediumSeaGreen); SafeNativeMethods.Gdip.ThreadData[MediumSeaGreenKey] = mediumSeaGreen; } return mediumSeaGreen; } } ////// /// A brush of the given color. /// public static Brush MediumSlateBlue { get { Brush mediumSlateBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumSlateBlueKey]; if (mediumSlateBlue == null) { mediumSlateBlue = new SolidBrush(Color.MediumSlateBlue); SafeNativeMethods.Gdip.ThreadData[MediumSlateBlueKey] = mediumSlateBlue; } return mediumSlateBlue; } } ////// /// A brush of the given color. /// public static Brush MediumSpringGreen { get { Brush mediumSpringGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumSpringGreenKey]; if (mediumSpringGreen == null) { mediumSpringGreen = new SolidBrush(Color.MediumSpringGreen); SafeNativeMethods.Gdip.ThreadData[MediumSpringGreenKey] = mediumSpringGreen; } return mediumSpringGreen; } } ////// /// A brush of the given color. /// public static Brush MediumTurquoise { get { Brush mediumTurquoise = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumTurquoiseKey]; if (mediumTurquoise == null) { mediumTurquoise = new SolidBrush(Color.MediumTurquoise); SafeNativeMethods.Gdip.ThreadData[MediumTurquoiseKey] = mediumTurquoise; } return mediumTurquoise; } } ////// /// A brush of the given color. /// public static Brush MediumVioletRed { get { Brush mediumVioletRed = (Brush)SafeNativeMethods.Gdip.ThreadData[MediumVioletRedKey]; if (mediumVioletRed == null) { mediumVioletRed = new SolidBrush(Color.MediumVioletRed); SafeNativeMethods.Gdip.ThreadData[MediumVioletRedKey] = mediumVioletRed; } return mediumVioletRed; } } ////// /// A brush of the given color. /// public static Brush MidnightBlue { get { Brush midnightBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[MidnightBlueKey]; if (midnightBlue == null) { midnightBlue = new SolidBrush(Color.MidnightBlue); SafeNativeMethods.Gdip.ThreadData[MidnightBlueKey] = midnightBlue; } return midnightBlue; } } ////// /// A brush of the given color. /// public static Brush MintCream { get { Brush mintCream = (Brush)SafeNativeMethods.Gdip.ThreadData[MintCreamKey]; if (mintCream == null) { mintCream = new SolidBrush(Color.MintCream); SafeNativeMethods.Gdip.ThreadData[MintCreamKey] = mintCream; } return mintCream; } } ////// /// A brush of the given color. /// public static Brush MistyRose { get { Brush mistyRose = (Brush)SafeNativeMethods.Gdip.ThreadData[MistyRoseKey]; if (mistyRose == null) { mistyRose = new SolidBrush(Color.MistyRose); SafeNativeMethods.Gdip.ThreadData[MistyRoseKey] = mistyRose; } return mistyRose; } } ////// /// A brush of the given color. /// public static Brush Moccasin { get { Brush moccasin = (Brush)SafeNativeMethods.Gdip.ThreadData[MoccasinKey]; if (moccasin == null) { moccasin = new SolidBrush(Color.Moccasin); SafeNativeMethods.Gdip.ThreadData[MoccasinKey] = moccasin; } return moccasin; } } ////// /// A brush of the given color. /// public static Brush NavajoWhite { get { Brush navajoWhite = (Brush)SafeNativeMethods.Gdip.ThreadData[NavajoWhiteKey]; if (navajoWhite == null) { navajoWhite = new SolidBrush(Color.NavajoWhite); SafeNativeMethods.Gdip.ThreadData[NavajoWhiteKey] = navajoWhite; } return navajoWhite; } } ////// /// A brush of the given color. /// public static Brush Navy { get { Brush navy = (Brush)SafeNativeMethods.Gdip.ThreadData[NavyKey]; if (navy == null) { navy = new SolidBrush(Color.Navy); SafeNativeMethods.Gdip.ThreadData[NavyKey] = navy; } return navy; } } ////// /// A brush of the given color. /// public static Brush OldLace { get { Brush oldLace = (Brush)SafeNativeMethods.Gdip.ThreadData[OldLaceKey]; if (oldLace == null) { oldLace = new SolidBrush(Color.OldLace); SafeNativeMethods.Gdip.ThreadData[OldLaceKey] = oldLace; } return oldLace; } } ////// /// A brush of the given color. /// public static Brush Olive { get { Brush olive = (Brush)SafeNativeMethods.Gdip.ThreadData[OliveKey]; if (olive == null) { olive = new SolidBrush(Color.Olive); SafeNativeMethods.Gdip.ThreadData[OliveKey] = olive; } return olive; } } ////// /// A brush of the given color. /// public static Brush OliveDrab { get { Brush oliveDrab = (Brush)SafeNativeMethods.Gdip.ThreadData[OliveDrabKey]; if (oliveDrab == null) { oliveDrab = new SolidBrush(Color.OliveDrab); SafeNativeMethods.Gdip.ThreadData[OliveDrabKey] = oliveDrab; } return oliveDrab; } } ////// /// A brush of the given color. /// public static Brush Orange { get { Brush orange = (Brush)SafeNativeMethods.Gdip.ThreadData[OrangeKey]; if (orange == null) { orange = new SolidBrush(Color.Orange); SafeNativeMethods.Gdip.ThreadData[OrangeKey] = orange; } return orange; } } ////// /// A brush of the given color. /// public static Brush OrangeRed { get { Brush orangeRed = (Brush)SafeNativeMethods.Gdip.ThreadData[OrangeRedKey]; if (orangeRed == null) { orangeRed = new SolidBrush(Color.OrangeRed); SafeNativeMethods.Gdip.ThreadData[OrangeRedKey] = orangeRed; } return orangeRed; } } ////// /// A brush of the given color. /// public static Brush Orchid { get { Brush orchid = (Brush)SafeNativeMethods.Gdip.ThreadData[OrchidKey]; if (orchid == null) { orchid = new SolidBrush(Color.Orchid); SafeNativeMethods.Gdip.ThreadData[OrchidKey] = orchid; } return orchid; } } ////// /// A brush of the given color. /// public static Brush PaleGoldenrod { get { Brush paleGoldenrod = (Brush)SafeNativeMethods.Gdip.ThreadData[PaleGoldenrodKey]; if (paleGoldenrod == null) { paleGoldenrod = new SolidBrush(Color.PaleGoldenrod); SafeNativeMethods.Gdip.ThreadData[PaleGoldenrodKey] = paleGoldenrod; } return paleGoldenrod; } } ////// /// A brush of the given color. /// public static Brush PaleGreen { get { Brush paleGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[PaleGreenKey]; if (paleGreen == null) { paleGreen = new SolidBrush(Color.PaleGreen); SafeNativeMethods.Gdip.ThreadData[PaleGreenKey] = paleGreen; } return paleGreen; } } ////// /// A brush of the given color. /// public static Brush PaleTurquoise { get { Brush paleTurquoise = (Brush)SafeNativeMethods.Gdip.ThreadData[PaleTurquoiseKey]; if (paleTurquoise == null) { paleTurquoise = new SolidBrush(Color.PaleTurquoise); SafeNativeMethods.Gdip.ThreadData[PaleTurquoiseKey] = paleTurquoise; } return paleTurquoise; } } ////// /// A brush of the given color. /// public static Brush PaleVioletRed { get { Brush paleVioletRed = (Brush)SafeNativeMethods.Gdip.ThreadData[PaleVioletRedKey]; if (paleVioletRed == null) { paleVioletRed = new SolidBrush(Color.PaleVioletRed); SafeNativeMethods.Gdip.ThreadData[PaleVioletRedKey] = paleVioletRed; } return paleVioletRed; } } ////// /// A brush of the given color. /// public static Brush PapayaWhip { get { Brush papayaWhip = (Brush)SafeNativeMethods.Gdip.ThreadData[PapayaWhipKey]; if (papayaWhip == null) { papayaWhip = new SolidBrush(Color.PapayaWhip); SafeNativeMethods.Gdip.ThreadData[PapayaWhipKey] = papayaWhip; } return papayaWhip; } } ////// /// A brush of the given color. /// public static Brush PeachPuff { get { Brush peachPuff = (Brush)SafeNativeMethods.Gdip.ThreadData[PeachPuffKey]; if (peachPuff == null) { peachPuff = new SolidBrush(Color.PeachPuff); SafeNativeMethods.Gdip.ThreadData[PeachPuffKey] = peachPuff; } return peachPuff; } } ////// /// A brush of the given color. /// public static Brush Peru { get { Brush peru = (Brush)SafeNativeMethods.Gdip.ThreadData[PeruKey]; if (peru == null) { peru = new SolidBrush(Color.Peru); SafeNativeMethods.Gdip.ThreadData[PeruKey] = peru; } return peru; } } ////// /// A brush of the given color. /// public static Brush Pink { get { Brush pink = (Brush)SafeNativeMethods.Gdip.ThreadData[PinkKey]; if (pink == null) { pink = new SolidBrush(Color.Pink); SafeNativeMethods.Gdip.ThreadData[PinkKey] = pink; } return pink; } } ////// /// A brush of the given color. /// public static Brush Plum { get { Brush plum = (Brush)SafeNativeMethods.Gdip.ThreadData[PlumKey]; if (plum == null) { plum = new SolidBrush(Color.Plum); SafeNativeMethods.Gdip.ThreadData[PlumKey] = plum; } return plum; } } ////// /// A brush of the given color. /// public static Brush PowderBlue { get { Brush powderBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[PowderBlueKey]; if (powderBlue == null) { powderBlue = new SolidBrush(Color.PowderBlue); SafeNativeMethods.Gdip.ThreadData[PowderBlueKey] = powderBlue; } return powderBlue; } } ////// /// A brush of the given color. /// public static Brush Purple { get { Brush purple = (Brush)SafeNativeMethods.Gdip.ThreadData[PurpleKey]; if (purple == null) { purple = new SolidBrush(Color.Purple); SafeNativeMethods.Gdip.ThreadData[PurpleKey] = purple; } return purple; } } ////// /// A brush of the given color. /// public static Brush Red { get { Brush red = (Brush)SafeNativeMethods.Gdip.ThreadData[RedKey]; if (red == null) { red = new SolidBrush(Color.Red); SafeNativeMethods.Gdip.ThreadData[RedKey] = red; } return red; } } ////// /// A brush of the given color. /// public static Brush RosyBrown { get { Brush rosyBrown = (Brush)SafeNativeMethods.Gdip.ThreadData[RosyBrownKey]; if (rosyBrown == null) { rosyBrown = new SolidBrush(Color.RosyBrown); SafeNativeMethods.Gdip.ThreadData[RosyBrownKey] = rosyBrown; } return rosyBrown; } } ////// /// A brush of the given color. /// public static Brush RoyalBlue { get { Brush royalBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[RoyalBlueKey]; if (royalBlue == null) { royalBlue = new SolidBrush(Color.RoyalBlue); SafeNativeMethods.Gdip.ThreadData[RoyalBlueKey] = royalBlue; } return royalBlue; } } ////// /// A brush of the given color. /// public static Brush SaddleBrown { get { Brush saddleBrown = (Brush)SafeNativeMethods.Gdip.ThreadData[SaddleBrownKey]; if (saddleBrown == null) { saddleBrown = new SolidBrush(Color.SaddleBrown); SafeNativeMethods.Gdip.ThreadData[SaddleBrownKey] = saddleBrown; } return saddleBrown; } } ////// /// A brush of the given color. /// public static Brush Salmon { get { Brush salmon = (Brush)SafeNativeMethods.Gdip.ThreadData[SalmonKey]; if (salmon == null) { salmon = new SolidBrush(Color.Salmon); SafeNativeMethods.Gdip.ThreadData[SalmonKey] = salmon; } return salmon; } } ////// /// A brush of the given color. /// public static Brush SandyBrown { get { Brush sandyBrown = (Brush)SafeNativeMethods.Gdip.ThreadData[SandyBrownKey]; if (sandyBrown == null) { sandyBrown = new SolidBrush(Color.SandyBrown); SafeNativeMethods.Gdip.ThreadData[SandyBrownKey] = sandyBrown; } return sandyBrown; } } ////// /// A brush of the given color. /// public static Brush SeaGreen { get { Brush seaGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[SeaGreenKey]; if (seaGreen == null) { seaGreen = new SolidBrush(Color.SeaGreen); SafeNativeMethods.Gdip.ThreadData[SeaGreenKey] = seaGreen; } return seaGreen; } } ////// /// A brush of the given color. /// public static Brush SeaShell { get { Brush seaShell = (Brush)SafeNativeMethods.Gdip.ThreadData[SeaShellKey]; if (seaShell == null) { seaShell = new SolidBrush(Color.SeaShell); SafeNativeMethods.Gdip.ThreadData[SeaShellKey] = seaShell; } return seaShell; } } ////// /// A brush of the given color. /// public static Brush Sienna { get { Brush sienna = (Brush)SafeNativeMethods.Gdip.ThreadData[SiennaKey]; if (sienna == null) { sienna = new SolidBrush(Color.Sienna); SafeNativeMethods.Gdip.ThreadData[SiennaKey] = sienna; } return sienna; } } ////// /// A brush of the given color. /// public static Brush Silver { get { Brush silver = (Brush)SafeNativeMethods.Gdip.ThreadData[SilverKey]; if (silver == null) { silver = new SolidBrush(Color.Silver); SafeNativeMethods.Gdip.ThreadData[SilverKey] = silver; } return silver; } } ////// /// A brush of the given color. /// public static Brush SkyBlue { get { Brush skyBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[SkyBlueKey]; if (skyBlue == null) { skyBlue = new SolidBrush(Color.SkyBlue); SafeNativeMethods.Gdip.ThreadData[SkyBlueKey] = skyBlue; } return skyBlue; } } ////// /// A brush of the given color. /// public static Brush SlateBlue { get { Brush slateBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[SlateBlueKey]; if (slateBlue == null) { slateBlue = new SolidBrush(Color.SlateBlue); SafeNativeMethods.Gdip.ThreadData[SlateBlueKey] = slateBlue; } return slateBlue; } } ////// /// A brush of the given color. /// public static Brush SlateGray { get { Brush slateGray = (Brush)SafeNativeMethods.Gdip.ThreadData[SlateGrayKey]; if (slateGray == null) { slateGray = new SolidBrush(Color.SlateGray); SafeNativeMethods.Gdip.ThreadData[SlateGrayKey] = slateGray; } return slateGray; } } ////// /// A brush of the given color. /// public static Brush Snow { get { Brush snow = (Brush)SafeNativeMethods.Gdip.ThreadData[SnowKey]; if (snow == null) { snow = new SolidBrush(Color.Snow); SafeNativeMethods.Gdip.ThreadData[SnowKey] = snow; } return snow; } } ////// /// A brush of the given color. /// public static Brush SpringGreen { get { Brush springGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[SpringGreenKey]; if (springGreen == null) { springGreen = new SolidBrush(Color.SpringGreen); SafeNativeMethods.Gdip.ThreadData[SpringGreenKey] = springGreen; } return springGreen; } } ////// /// A brush of the given color. /// public static Brush SteelBlue { get { Brush steelBlue = (Brush)SafeNativeMethods.Gdip.ThreadData[SteelBlueKey]; if (steelBlue == null) { steelBlue = new SolidBrush(Color.SteelBlue); SafeNativeMethods.Gdip.ThreadData[SteelBlueKey] = steelBlue; } return steelBlue; } } ////// /// A brush of the given color. /// public static Brush Tan { get { Brush tan = (Brush)SafeNativeMethods.Gdip.ThreadData[TanKey]; if (tan == null) { tan = new SolidBrush(Color.Tan); SafeNativeMethods.Gdip.ThreadData[TanKey] = tan; } return tan; } } ////// /// A brush of the given color. /// public static Brush Teal { get { Brush teal = (Brush)SafeNativeMethods.Gdip.ThreadData[TealKey]; if (teal == null) { teal = new SolidBrush(Color.Teal); SafeNativeMethods.Gdip.ThreadData[TealKey] = teal; } return teal; } } ////// /// A brush of the given color. /// public static Brush Thistle { get { Brush thistle = (Brush)SafeNativeMethods.Gdip.ThreadData[ThistleKey]; if (thistle == null) { thistle = new SolidBrush(Color.Thistle); SafeNativeMethods.Gdip.ThreadData[ThistleKey] = thistle; } return thistle; } } ////// /// A brush of the given color. /// public static Brush Tomato { get { Brush tomato = (Brush)SafeNativeMethods.Gdip.ThreadData[TomatoKey]; if (tomato == null) { tomato = new SolidBrush(Color.Tomato); SafeNativeMethods.Gdip.ThreadData[TomatoKey] = tomato; } return tomato; } } ////// /// A brush of the given color. /// public static Brush Turquoise { get { Brush turquoise = (Brush)SafeNativeMethods.Gdip.ThreadData[TurquoiseKey]; if (turquoise == null) { turquoise = new SolidBrush(Color.Turquoise); SafeNativeMethods.Gdip.ThreadData[TurquoiseKey] = turquoise; } return turquoise; } } ////// /// A brush of the given color. /// public static Brush Violet { get { Brush violet = (Brush)SafeNativeMethods.Gdip.ThreadData[VioletKey]; if (violet == null) { violet = new SolidBrush(Color.Violet); SafeNativeMethods.Gdip.ThreadData[VioletKey] = violet; } return violet; } } ////// /// A brush of the given color. /// public static Brush Wheat { get { Brush wheat = (Brush)SafeNativeMethods.Gdip.ThreadData[WheatKey]; if (wheat == null) { wheat = new SolidBrush(Color.Wheat); SafeNativeMethods.Gdip.ThreadData[WheatKey] = wheat; } return wheat; } } ////// /// A brush of the given color. /// public static Brush White { get { Brush white = (Brush)SafeNativeMethods.Gdip.ThreadData[WhiteKey]; if (white == null) { white = new SolidBrush(Color.White); SafeNativeMethods.Gdip.ThreadData[WhiteKey] = white; } return white; } } ////// /// A brush of the given color. /// public static Brush WhiteSmoke { get { Brush whiteSmoke = (Brush)SafeNativeMethods.Gdip.ThreadData[WhiteSmokeKey]; if (whiteSmoke == null) { whiteSmoke = new SolidBrush(Color.WhiteSmoke); SafeNativeMethods.Gdip.ThreadData[WhiteSmokeKey] = whiteSmoke; } return whiteSmoke; } } ////// /// A brush of the given color. /// public static Brush Yellow { get { Brush yellow = (Brush)SafeNativeMethods.Gdip.ThreadData[YellowKey]; if (yellow == null) { yellow = new SolidBrush(Color.Yellow); SafeNativeMethods.Gdip.ThreadData[YellowKey] = yellow; } return yellow; } } ////// /// A brush of the given color. /// public static Brush YellowGreen { get { Brush yellowGreen = (Brush)SafeNativeMethods.Gdip.ThreadData[YellowGreenKey]; if (yellowGreen == null) { yellowGreen = new SolidBrush(Color.YellowGreen); SafeNativeMethods.Gdip.ThreadData[YellowGreenKey] = yellowGreen; } return yellowGreen; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
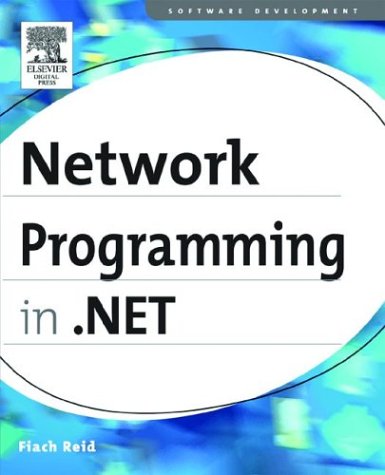
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectDataSourceDisposingEventArgs.cs
- UpdatePanelTrigger.cs
- ContentPresenter.cs
- EntityDataSourceViewSchema.cs
- SoundPlayerAction.cs
- CompilerError.cs
- EntitySetBaseCollection.cs
- HtmlControlPersistable.cs
- XhtmlStyleClass.cs
- XmlILModule.cs
- ToolStripLocationCancelEventArgs.cs
- PathGradientBrush.cs
- BinaryObjectReader.cs
- CompiledQuery.cs
- BooleanExpr.cs
- SkinIDTypeConverter.cs
- EdmScalarPropertyAttribute.cs
- TemplateInstanceAttribute.cs
- FrameworkName.cs
- SmtpFailedRecipientsException.cs
- KeyedHashAlgorithm.cs
- TextEffect.cs
- OutputCacheProfileCollection.cs
- DataGridViewBand.cs
- HttpCachePolicyWrapper.cs
- WindowHelperService.cs
- xsdvalidator.cs
- StorageConditionPropertyMapping.cs
- PersonalizationDictionary.cs
- SecurityDocument.cs
- WriteableBitmap.cs
- ObjectListComponentEditor.cs
- ZipArchive.cs
- ProtectedConfiguration.cs
- ClientBuildManagerCallback.cs
- SafeRegistryKey.cs
- XXXInfos.cs
- IBuiltInEvidence.cs
- Ops.cs
- ExceptionValidationRule.cs
- ConfigurationValidatorBase.cs
- ErrorProvider.cs
- DataView.cs
- TextRenderer.cs
- Empty.cs
- DocComment.cs
- X509PeerCertificateAuthentication.cs
- WebServiceData.cs
- XmlSchemaNotation.cs
- EditorResources.cs
- EventRecordWrittenEventArgs.cs
- SupportingTokenBindingElement.cs
- DeploymentSection.cs
- WindowInteractionStateTracker.cs
- PrintDocument.cs
- SmtpNetworkElement.cs
- DrawingDrawingContext.cs
- __Error.cs
- KoreanLunisolarCalendar.cs
- ToolboxItemFilterAttribute.cs
- ProfilePropertySettingsCollection.cs
- DecoderBestFitFallback.cs
- SerializationEventsCache.cs
- JsonWriterDelegator.cs
- ComponentEvent.cs
- ConnectionProviderAttribute.cs
- SslStream.cs
- PriorityBinding.cs
- BitmapPalette.cs
- PermissionToken.cs
- DoubleCollection.cs
- LocatorGroup.cs
- DateTimeFormatInfo.cs
- NavigationExpr.cs
- TextFormattingConverter.cs
- SqlFileStream.cs
- WarningException.cs
- SoapInteropTypes.cs
- SQLInt32.cs
- ProgressBar.cs
- Condition.cs
- DesignerProperties.cs
- QueryException.cs
- OraclePermissionAttribute.cs
- RootAction.cs
- NavigationExpr.cs
- TrackingMemoryStreamFactory.cs
- LingerOption.cs
- ControllableStoryboardAction.cs
- IndicFontClient.cs
- dataSvcMapFileLoader.cs
- TextElementEnumerator.cs
- NonVisualControlAttribute.cs
- AsymmetricKeyExchangeDeformatter.cs
- MsmqProcessProtocolHandler.cs
- XAMLParseException.cs
- SerializableAttribute.cs
- CompilerCollection.cs
- ToolStripSplitButton.cs
- Block.cs