Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / DesignTableCollection.cs / 1 / DesignTableCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design{ using System; using System.Collections; using System.Data; using System.Diagnostics; ////// internal class DesignTableCollection : DataSourceCollectionBase { private DesignDataSource dataSource; public DesignTableCollection(DesignDataSource dataSource) : base(dataSource) { this.dataSource = dataSource; } private DataSet DataSet{ get{ if (dataSource != null){ return dataSource.DataSet; } return null; } } protected override Type ItemType { get { return typeof(DesignTable); } } protected override INameService NameService { get { return DataSetNameService.DefaultInstance; } } ////// internal DesignTable this[string name] { get { return (DesignTable)FindObject(name); } } ////// internal DesignTable this[DataTable dataTable] { get { foreach (DesignTable designTable in this) { if (designTable.DataTable == dataTable) { return designTable; } } return null; } } ////// Will throw if name is invalid or a dup /// Add the DataTable to the dataTable if not added yet /// public void Add(DesignTable designTable){ // List.Add(designTable); } public bool Contains( DesignTable table ) { return List.Contains( table ); } public int IndexOf( DesignTable table ) { return List.IndexOf( table ); } public void Remove( DesignTable table ) { List.Remove( table ); } ////// Note: this function need to call base first /// to ensure the undo model work! /// protected override void OnInsert( int index, object value ) { base.OnInsert(index, value); DesignTable designTable = (DesignTable)value; if (designTable.Name == null || designTable.Name.Length == 0) { designTable.Name = CreateUniqueName(designTable); } NameService.ValidateUniqueName(this, designTable.Name); if( (this.dataSource != null) && (designTable.Owner == this.dataSource) ) { Debug.Fail( "Table already belongs to this DataSource" ); return; // no-op } if( (this.dataSource != null) && (designTable.Owner != null) ) { throw new InternalException( VSDExceptions.DataSource.TABLE_BELONGS_TO_OTHER_DATA_SOURCE_MSG, VSDExceptions.DataSource.TABLE_BELONGS_TO_OTHER_DATA_SOURCE_CODE ); } DataSet dataSet = DataSet; if ((dataSet != null) && (!dataSet.Tables.Contains(designTable.DataTable.TableName))) { Debug.Assert( this.dataSource != null, "If we were able to get the DataSet we should have a design time data source as well" ); dataSet.Tables.Add(designTable.DataTable); } designTable.Owner = this.dataSource; } ////// Remove the DataTable in the dataTable if not removed yet /// Note: this function need to call base first /// to ensure the undo model work! /// protected override void OnRemove( int index, object value ) { base.OnRemove(index, value); DesignTable designTable = (DesignTable)value; DataSet dataSet = DataSet; if (dataSet != null && designTable.DataTable != null && dataSet.Tables.Contains(designTable.DataTable.TableName)){ dataSet.Tables.Remove(designTable.DataTable); } designTable.Owner = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
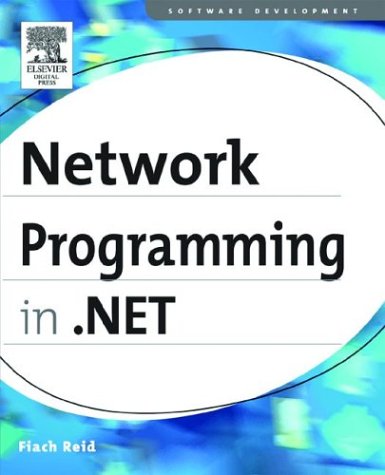
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewHitTestInfo.cs
- SendActivityEventArgs.cs
- SplitContainer.cs
- SqlInfoMessageEvent.cs
- TrackingConditionCollection.cs
- AssemblyInfo.cs
- ContentIterators.cs
- Process.cs
- SafeNativeMethods.cs
- HtmlContainerControl.cs
- ItemsControlAutomationPeer.cs
- UnsafeNativeMethods.cs
- PerfCounters.cs
- util.cs
- PersonalizationDictionary.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- KeyValueConfigurationCollection.cs
- EditCommandColumn.cs
- XslAstAnalyzer.cs
- ObjectHandle.cs
- MimeObjectFactory.cs
- CssStyleCollection.cs
- MimeParameterWriter.cs
- EtwTrace.cs
- WebDisplayNameAttribute.cs
- LocalIdCollection.cs
- RoutedUICommand.cs
- DynamicMetaObjectBinder.cs
- ControlCollection.cs
- RuntimeHelpers.cs
- CodePropertyReferenceExpression.cs
- AnnotationDocumentPaginator.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- PermissionRequestEvidence.cs
- OrderedHashRepartitionEnumerator.cs
- XmlSchemaImport.cs
- MediaElementAutomationPeer.cs
- DataBindEngine.cs
- SamlSubjectStatement.cs
- AppDomainShutdownMonitor.cs
- WinFormsSpinner.cs
- GlyphElement.cs
- CodeDirectoryCompiler.cs
- OdbcDataAdapter.cs
- IChannel.cs
- GroupAggregateExpr.cs
- GenericAuthenticationEventArgs.cs
- VisualTreeUtils.cs
- ApplicationActivator.cs
- ModuleBuilderData.cs
- ObjectSelectorEditor.cs
- PersonalizationEntry.cs
- GeometryGroup.cs
- UpdatePanelControlTrigger.cs
- ICspAsymmetricAlgorithm.cs
- BufferAllocator.cs
- BitmapEffectDrawing.cs
- TraceSection.cs
- EncoderBestFitFallback.cs
- Walker.cs
- ToolStripCodeDomSerializer.cs
- QuaternionAnimation.cs
- RijndaelManaged.cs
- EventWaitHandle.cs
- RoutedPropertyChangedEventArgs.cs
- NominalTypeEliminator.cs
- ListItemConverter.cs
- AddInServer.cs
- HtmlLink.cs
- MDIControlStrip.cs
- AssemblyNameEqualityComparer.cs
- CollectionConverter.cs
- ControlAdapter.cs
- FacetChecker.cs
- DtrList.cs
- OdbcInfoMessageEvent.cs
- MissingManifestResourceException.cs
- ConfigurationStrings.cs
- TrackingProvider.cs
- TransformerTypeCollection.cs
- SpellCheck.cs
- HelpEvent.cs
- XPathCompiler.cs
- MeshGeometry3D.cs
- ToolStripOverflowButton.cs
- SchemaCollectionCompiler.cs
- FileEnumerator.cs
- ArrayList.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- ButtonColumn.cs
- XPathBinder.cs
- TitleStyle.cs
- PagesSection.cs
- DbConnectionInternal.cs
- _SslStream.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- SemanticBasicElement.cs
- ConnectionPoolManager.cs
- NativeBuffer.cs
- Int16.cs