Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / LocalIdCollection.cs / 1 / LocalIdCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; // // Summary: // The collection of LocalIds used during search operations. // // Remarks: // This is for internal store use only. // internal class LocalIdCollection { SortedListm_inner; public LocalIdCollection() { m_inner = new SortedList (); } // // Summary: // Adds an item to the list to the correct spot in the list. // Parameters: // item: The value to add to the list // public void Add( int item ) { if( !m_inner.ContainsKey( item ) ) { m_inner.Add( item, item ); } } public void Clear() { m_inner.Clear(); } public int Count { get { return m_inner.Count; } } // // Summary: // Filters the current list of localIds, // // Remarks: // Opearation is O(n) // // Parameters: // itemsToKeep: the list of items not to remove from the list. // // public void Filter( LocalIdCollection itemsToKeep ) { int count = m_inner.Count; for( int i = count - 1; i >=0; i-- ) { if( !itemsToKeep.m_inner.ContainsKey( m_inner.Keys[i] ) ) { m_inner.RemoveAt( i ); } } } public IList Keys { get { return m_inner.Keys; } } // } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
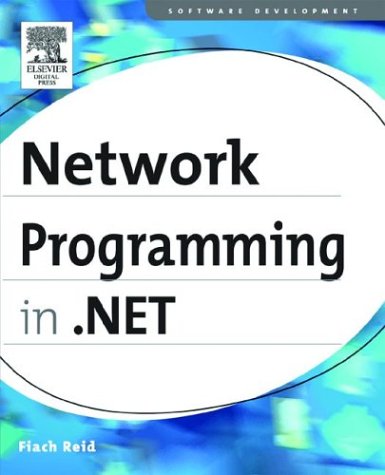
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventWaitHandleSecurity.cs
- ConfigXmlText.cs
- DataGridCell.cs
- ScrollContentPresenter.cs
- MobileErrorInfo.cs
- ACE.cs
- AutomationProperty.cs
- XPathNodeInfoAtom.cs
- NativeMethods.cs
- SoapFaultCodes.cs
- CompositeControlDesigner.cs
- RemoteCryptoSignHashRequest.cs
- ExpressionBuilder.cs
- SmtpFailedRecipientsException.cs
- VideoDrawing.cs
- XmlWhitespace.cs
- PassportAuthentication.cs
- DiscriminatorMap.cs
- HwndHost.cs
- PropertyGridEditorPart.cs
- SqlNode.cs
- Configuration.cs
- PolicyException.cs
- MILUtilities.cs
- WindowsListViewSubItem.cs
- Stopwatch.cs
- JsonDataContract.cs
- IOException.cs
- EventLog.cs
- XPathNodeInfoAtom.cs
- DayRenderEvent.cs
- FunctionDetailsReader.cs
- WebPartEventArgs.cs
- Transform.cs
- TableStyle.cs
- QueryOpeningEnumerator.cs
- FontCollection.cs
- Empty.cs
- DocumentEventArgs.cs
- DomNameTable.cs
- COMException.cs
- mansign.cs
- SByte.cs
- ComponentManagerBroker.cs
- SerialPinChanges.cs
- ProcessModelInfo.cs
- FactoryGenerator.cs
- Block.cs
- XmlObjectSerializerReadContextComplex.cs
- DocumentSequenceHighlightLayer.cs
- DynamicPropertyReader.cs
- DbProviderManifest.cs
- MethodToken.cs
- SoapSchemaImporter.cs
- ProfileGroupSettings.cs
- Form.cs
- Context.cs
- URL.cs
- ADMembershipUser.cs
- MetaTable.cs
- SerializationObjectManager.cs
- Literal.cs
- X509Extension.cs
- PkcsUtils.cs
- ActivationServices.cs
- SizeAnimation.cs
- ActivationServices.cs
- SystemIPAddressInformation.cs
- ErrorEventArgs.cs
- ExeConfigurationFileMap.cs
- TreeViewHitTestInfo.cs
- PinnedBufferMemoryStream.cs
- RenderDataDrawingContext.cs
- TraceLevelStore.cs
- IsolatedStorageException.cs
- WeakReference.cs
- MobileCapabilities.cs
- ResourceSetExpression.cs
- ValuePattern.cs
- GroupQuery.cs
- SpellerHighlightLayer.cs
- BaseTemplateCodeDomTreeGenerator.cs
- PagedDataSource.cs
- CqlBlock.cs
- PropertyValueUIItem.cs
- Wizard.cs
- EntityKey.cs
- processwaithandle.cs
- CmsUtils.cs
- XmlnsCache.cs
- COM2Properties.cs
- AnnotationService.cs
- ScriptModule.cs
- WindowHideOrCloseTracker.cs
- ResXFileRef.cs
- StringFreezingAttribute.cs
- QilBinary.cs
- WebConvert.cs
- DbProviderSpecificTypePropertyAttribute.cs
- ExpandCollapseProviderWrapper.cs