Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationTypes / System / Windows / Automation / ControlType.cs / 1 / ControlType.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Identifier for Automation ControlTypes // // History: // 02/10/2004 : Srikanth Koneru created // //--------------------------------------------------------------------------- using System; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Identifier for Automation ControlType /// #if (INTERNAL_COMPILE) internal class ControlType : AutomationIdentifier #else public class ControlType : AutomationIdentifier #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal ControlType(int id, Guid guid, string programmaticName) : base(UiaCoreTypesApi.AutomationIdType.ControlType, id, guid, programmaticName) { } ////// internal static ControlType Register(Guid guid, string programmaticName) { return (ControlType)AutomationIdentifier.Register(UiaCoreTypesApi.AutomationIdType.ControlType, guid, programmaticName); } ////// public static ControlType LookupById(int id) { return (ControlType)AutomationIdentifier.LookupById(UiaCoreTypesApi.AutomationIdType.ControlType, id); } //Registers a control type. internal static ControlType Register(Guid guid, string programmaticName, STID stId, AutomationProperty[] requiredProperties, AutomationPattern[] neverSupportedPatterns, AutomationPattern[][] requiredPatternsSets) { ControlType controlType = (ControlType)AutomationIdentifier.Register(UiaCoreTypesApi.AutomationIdType.ControlType, guid, programmaticName); controlType._stId = stId; controlType._requiredPatternsSets = requiredPatternsSets; controlType._neverSupportedPatterns = neverSupportedPatterns; controlType._requiredProperties = requiredProperties; return controlType; } //Never supported patterns and required properties are set to an empty array internal static ControlType Register(Guid guid, string programmaticName, STID stId, AutomationPattern[][] requiredPatternsSets) { return ControlType.Register(guid, programmaticName, stId, new AutomationProperty[0], new AutomationPattern[0], requiredPatternsSets); } //Never supported patterns and required patterns are set to an empty array internal static ControlType Register(Guid guid, string programmaticName, STID stId, AutomationProperty[] requiredProperties) { return ControlType.Register(guid, programmaticName, stId, requiredProperties, new AutomationPattern[0], new AutomationPattern[0][]); } //Required patterns, never supported patterns and required properties are set to an empty array internal static ControlType Register(Guid guid, string programmaticName, STID stId) { return ControlType.Register(guid, programmaticName, stId, new AutomationProperty[0], new AutomationPattern[0], new AutomationPattern[0][]); } #endregion //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Each row of this array contains a set of AutomationPatterns in which /// atleast one set should be supported by the element. /// ///Array of sets of required patterns. public AutomationPattern[][] GetRequiredPatternSets() { int totalRows = this._requiredPatternsSets.Length; AutomationPattern[][] clone = new AutomationPattern[totalRows][]; for (int i = 0; i < totalRows; i++) { clone[i] = (AutomationPattern[])this._requiredPatternsSets[i].Clone(); } return clone; } ////// Gets the array of never supported patterns. /// ///Array of never supported patterns. public AutomationPattern[] GetNeverSupportedPatterns() { return (AutomationPattern[])_neverSupportedPatterns.Clone(); } ////// Gets the array of required properties. /// ///Array of required properties. public AutomationProperty[] GetRequiredProperties() { return (AutomationProperty[])_requiredProperties.Clone(); } #endregion //------------------------------------------------------ // // Public Fields // //------------------------------------------------------ #region Public Fields ///ControlType ID: Button - a clickable button public static readonly ControlType Button = ControlType.Register(AutomationIdentifierGuids.Button_Control, "ControlType.Button", STID.LocalizedControlTypeButton, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern } }); ///ControlType ID: Calendar - A date choosing mechanism public static readonly ControlType Calendar = ControlType.Register(AutomationIdentifierGuids.Calendar_Control, "ControlType.Calendar", STID.LocalizedControlTypeCalendar, new AutomationPattern[][] { new AutomationPattern[] { GridPatternIdentifiers.Pattern, ValuePatternIdentifiers.Pattern, SelectionPatternIdentifiers.Pattern } }); ///ControlType ID: CheckBox - A toggleable checkbox public static readonly ControlType CheckBox = ControlType.Register(AutomationIdentifierGuids.CheckBox_Control, "ControlType.CheckBox", STID.LocalizedControlTypeCheckBox, new AutomationPattern[][] { new AutomationPattern[] { TogglePatternIdentifiers.Pattern } }); ///ControlType ID: ComboBox - An editable dropdown box public static readonly ControlType ComboBox = ControlType.Register(AutomationIdentifierGuids.ComboBox_Control, "ControlType.ComboBox", STID.LocalizedControlTypeComboBox, new AutomationPattern[][] { new AutomationPattern[] { SelectionPatternIdentifiers.Pattern, ExpandCollapsePatternIdentifiers.Pattern } }); ///ControlType ID: Edit - A simple area with a user changeable value public static readonly ControlType Edit = ControlType.Register(AutomationIdentifierGuids.Edit_Control, "ControlType.Edit", STID.LocalizedControlTypeEdit, new AutomationPattern[][] { new AutomationPattern[] { ValuePatternIdentifiers.Pattern } }); ///ControlType ID: Hyperlink - a clickable hyperlink public static readonly ControlType Hyperlink = ControlType.Register(AutomationIdentifierGuids.Hyperlink_Control, "ControlType.Hyperlink", STID.LocalizedControlTypeHyperlink, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern } }); ///ControlType ID: Image - a non-interactive image public static readonly ControlType Image = ControlType.Register(AutomationIdentifierGuids.Image_Control, "ControlType.Image", STID.LocalizedControlTypeImage); ///ControlType ID: ListItem - An Item in a ListView public static readonly ControlType ListItem = ControlType.Register(AutomationIdentifierGuids.ListItem_Control, "ControlType.ListItem", STID.LocalizedControlTypeListItem, new AutomationPattern[][] { new AutomationPattern[] { SelectionItemPatternIdentifiers.Pattern } }); ///ControlType ID: List - a Listview for making a selection public static readonly ControlType List = ControlType.Register(AutomationIdentifierGuids.List_Control, "ControlType.List", STID.LocalizedControlTypeListView, new AutomationPattern[][] { new AutomationPattern[] { SelectionPatternIdentifiers.Pattern, TablePatternIdentifiers.Pattern, GridPatternIdentifiers.Pattern, MultipleViewPatternIdentifiers.Pattern } }); ///ControlType ID: Menu - A menu, usually has menuitems in it public static readonly ControlType Menu = ControlType.Register(AutomationIdentifierGuids.Menu_Control, "ControlType.Menu", STID.LocalizedControlTypeMenu); ///ControlType ID: MenuBar - A menu-bar, usually populated with menubuttons public static readonly ControlType MenuBar = ControlType.Register(AutomationIdentifierGuids.MenuBar_Control, "ControlType.MenuBar", STID.LocalizedControlTypeMenuBar); ///ControlType ID: MenuItem - An item in a menu, usually clickable public static readonly ControlType MenuItem = ControlType.Register(AutomationIdentifierGuids.MenuItem_Control, "ControlType.MenuItem", STID.LocalizedControlTypeMenuItem, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern }, new AutomationPattern[] { ExpandCollapsePatternIdentifiers.Pattern }, new AutomationPattern[] { TogglePatternIdentifiers.Pattern }, }); ///ControlType ID: ProgressBar - Visually indicates the progress of a lengthy operation. public static readonly ControlType ProgressBar = ControlType.Register(AutomationIdentifierGuids.ProgressBar_Control, "ControlType.ProgressBar", STID.LocalizedControlTypeProgressBar, new AutomationPattern[][] { new AutomationPattern[] { ValuePatternIdentifiers.Pattern } }); ///ControlType ID: RadioButton - A selection mechanism allowing exactly 1 selected item in a group public static readonly ControlType RadioButton = ControlType.Register(AutomationIdentifierGuids.RadioButton_Control, "ControlType.RadioButton", STID.LocalizedControlTypeRadioButton); ///ControlType ID: ScrollBar - A Scrollbar, the value is usually used to control scrolling of a window public static readonly ControlType ScrollBar = ControlType.Register(AutomationIdentifierGuids.ScrollBar_Control, "ControlType.ScrollBar", STID.LocalizedControlTypeScrollBar); ///ControlType ID: Slider - A Slider, usually used to set a value public static readonly ControlType Slider = ControlType.Register(AutomationIdentifierGuids.Slider_Control, "ControlType.Slider", STID.LocalizedControlTypeSlider, new AutomationPattern[][] { new AutomationPattern[] { RangeValuePatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern } }); ///ControlType ID: Spinner - A Control that allows you to either enter a numeric value or scroll it up and down public static readonly ControlType Spinner = ControlType.Register(AutomationIdentifierGuids.Spinner_Control, "ControlType.Spinner", STID.LocalizedControlTypeSpinner, new AutomationPattern[][] { new AutomationPattern[] { RangeValuePatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern } }); ///ControlType ID: StatusBar - A bar used to report status of an application public static readonly ControlType StatusBar = ControlType.Register(AutomationIdentifierGuids.StatusBar_Control, "ControlType.StatusBar", STID.LocalizedControlTypeStatusBar); ///ControlType ID: Tab - A Tabbed window public static readonly ControlType Tab = ControlType.Register(AutomationIdentifierGuids.Tab_Control, "ControlType.Tab", STID.LocalizedControlTypeTab); ///ControlType ID: TabItem - An individual tab of a tabbed window public static readonly ControlType TabItem = ControlType.Register(AutomationIdentifierGuids.TabItem_Control, "ControlType.TabItem", STID.LocalizedControlTypeTabItem); ///ControlType ID: Image - a non-interactive text public static readonly ControlType Text = ControlType.Register(AutomationIdentifierGuids.Text_Control, "ControlType.Text", STID.LocalizedControlTypeText); ///ControlType ID: ToolBar - A Bar, usually full of buttons or other controls public static readonly ControlType ToolBar = ControlType.Register(AutomationIdentifierGuids.ToolBar_Control, "ControlType.ToolBar", STID.LocalizedControlTypeToolBar); ///ControlType ID: ToolTip - a small window that pops up containing helpful tips for using a control public static readonly ControlType ToolTip = ControlType.Register(AutomationIdentifierGuids.ToolTip_Control, "ControlType.ToolTip", STID.LocalizedControlTypeToolTip); ///ControlType ID: Tree - A display showing a hierarchy of specific items public static readonly ControlType Tree = ControlType.Register(AutomationIdentifierGuids.Tree_Control, "ControlType.Tree", STID.LocalizedControlTypeTreeView); ///ControlType ID: TreeItem - An individual item in a tree, usually able to be expanded to show its children public static readonly ControlType TreeItem = ControlType.Register(AutomationIdentifierGuids.TreeItem_Control, "ControlType.TreeItem", STID.LocalizedControlTypeTreeViewItem); ///ControlType ID: Custom - Generic ControlType used for anything not covered by another ControlType public static readonly ControlType Custom = ControlType.Register(AutomationIdentifierGuids.Custom_Control, "ControlType.Custom", STID.LocalizedControlTypeCustom); ///ControlType ID: Group - Is a separation in the automation tree so that items that are grouped together have a logical division with the structure. public static readonly ControlType Group = ControlType.Register(AutomationIdentifierGuids.Group_Control, "ControlType.Group", STID.LocalizedControlTypeGroup); ///ControlType ID: Thumb - It provides basic semantic input for dragging/resizing-like input behavior from the user. public static readonly ControlType Thumb = ControlType.Register(AutomationIdentifierGuids.Thumb_Control, "ControlType.Thumb", STID.LocalizedControlTypeThumb); ///ControlType ID: DataGrid - Lets a user easily work with items that contains metadata represented in columns. public static readonly ControlType DataGrid = ControlType.Register(AutomationIdentifierGuids.DataGrid_Control, "ControlType.DataGrid", STID.LocalizedControlTypeDataGrid, new AutomationPattern[][] { new AutomationPattern[] { GridPatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern }, new AutomationPattern[] { TablePatternIdentifiers.Pattern }, }); ///ControlType ID: DataItem - Is more complicated than the simple list item because it has a large amount of information stored within it. public static readonly ControlType DataItem = ControlType.Register(AutomationIdentifierGuids.DataItem_Control, "ControlType.DataItem", STID.LocalizedControlTypeDataItem, new AutomationPattern[][] { new AutomationPattern[] { SelectionItemPatternIdentifiers.Pattern } }); ///ControlType ID: Document - Lets a user view/manipulate multiple pages of text. public static readonly ControlType Document = ControlType.Register(AutomationIdentifierGuids.Document_Control, "ControlType.Document", STID.LocalizedControlTypeDocument, new AutomationProperty[0], new AutomationPattern[] { ValuePatternIdentifiers.Pattern }, new AutomationPattern[][] { new AutomationPattern[] { ScrollPatternIdentifiers.Pattern } , new AutomationPattern[] { TextPatternIdentifiers.Pattern } , }); ///ControlType ID: SplitButton - Enables the ability to both perform an action on the toolbar button and expand the control to a list of other possible actions that can be performed. public static readonly ControlType SplitButton = ControlType.Register(AutomationIdentifierGuids.SplitButton_Control, "ControlType.SplitButton", STID.LocalizedControlTypeSplitButton, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern }, new AutomationPattern[] { ExpandCollapsePatternIdentifiers.Pattern }, }); ///ControlType ID: Window - The object representing the window frame, which contains child objects such as a title bar, client, and other objects contained in a window. public static readonly ControlType Window = ControlType.Register(AutomationIdentifierGuids.Window_Control, "ControlType.Window", STID.LocalizedControlTypeWindow, new AutomationPattern[][] { new AutomationPattern[] { TransformPatternIdentifiers.Pattern }, new AutomationPattern[] { WindowPatternIdentifiers.Pattern }, }); ///ControlType ID: Pane - Simular to Window but does not support WindowPattern. public static readonly ControlType Pane = ControlType.Register(AutomationIdentifierGuids.Pane_Control, "ControlType.Pane", STID.LocalizedControlTypePane, new AutomationPattern[][] { new AutomationPattern[] { TransformPatternIdentifiers.Pattern } }); ///ControlType ID: Header - Provides a visual container for the labels for rows and/or columns of information. public static readonly ControlType Header = ControlType.Register(AutomationIdentifierGuids.Header_Control, "ControlType.Header", STID.LocalizedControlTypeHeader); ///ControlType ID: HeaderItem - Provides a visual label for a row and/or column of information. public static readonly ControlType HeaderItem = ControlType.Register(AutomationIdentifierGuids.HeaderItem_Control, "ControlType.HeaderItem", STID.LocalizedControlTypeHeaderItem); ///ControlType ID: Table - Simular to DataGrid but only contains text elements. public static readonly ControlType Table = ControlType.Register(AutomationIdentifierGuids.Table_Control, "ControlType.Table", STID.LocalizedControlTypeTable, new AutomationPattern[][] { new AutomationPattern[] { GridPatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern }, new AutomationPattern[] { TablePatternIdentifiers.Pattern }, }); ///ControlType ID: TitleBar - The portion of a window that identifies the window. public static readonly ControlType TitleBar = ControlType.Register(AutomationIdentifierGuids.TitleBar_Control, "ControlType.TitleBar", STID.LocalizedControlTypeTitleBar); ///ControlType ID: Separator - An object that creates visual space in controls like menus and toolbars. public static readonly ControlType Separator = ControlType.Register(AutomationIdentifierGuids.Separator_Control, "ControlType.Separator", STID.LocalizedControlTypeSeparator); #endregion //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ///Returns the Localized control type public string LocalizedControlType { get { return ST.Get(_stId); } } #endregion //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPattern[][] _requiredPatternsSets; private AutomationPattern[] _neverSupportedPatterns; private AutomationProperty[] _requiredProperties; private STID _stId; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Identifier for Automation ControlTypes // // History: // 02/10/2004 : Srikanth Koneru created // //--------------------------------------------------------------------------- using System; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Identifier for Automation ControlType /// #if (INTERNAL_COMPILE) internal class ControlType : AutomationIdentifier #else public class ControlType : AutomationIdentifier #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal ControlType(int id, Guid guid, string programmaticName) : base(UiaCoreTypesApi.AutomationIdType.ControlType, id, guid, programmaticName) { } ////// internal static ControlType Register(Guid guid, string programmaticName) { return (ControlType)AutomationIdentifier.Register(UiaCoreTypesApi.AutomationIdType.ControlType, guid, programmaticName); } ////// public static ControlType LookupById(int id) { return (ControlType)AutomationIdentifier.LookupById(UiaCoreTypesApi.AutomationIdType.ControlType, id); } //Registers a control type. internal static ControlType Register(Guid guid, string programmaticName, STID stId, AutomationProperty[] requiredProperties, AutomationPattern[] neverSupportedPatterns, AutomationPattern[][] requiredPatternsSets) { ControlType controlType = (ControlType)AutomationIdentifier.Register(UiaCoreTypesApi.AutomationIdType.ControlType, guid, programmaticName); controlType._stId = stId; controlType._requiredPatternsSets = requiredPatternsSets; controlType._neverSupportedPatterns = neverSupportedPatterns; controlType._requiredProperties = requiredProperties; return controlType; } //Never supported patterns and required properties are set to an empty array internal static ControlType Register(Guid guid, string programmaticName, STID stId, AutomationPattern[][] requiredPatternsSets) { return ControlType.Register(guid, programmaticName, stId, new AutomationProperty[0], new AutomationPattern[0], requiredPatternsSets); } //Never supported patterns and required patterns are set to an empty array internal static ControlType Register(Guid guid, string programmaticName, STID stId, AutomationProperty[] requiredProperties) { return ControlType.Register(guid, programmaticName, stId, requiredProperties, new AutomationPattern[0], new AutomationPattern[0][]); } //Required patterns, never supported patterns and required properties are set to an empty array internal static ControlType Register(Guid guid, string programmaticName, STID stId) { return ControlType.Register(guid, programmaticName, stId, new AutomationProperty[0], new AutomationPattern[0], new AutomationPattern[0][]); } #endregion //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Each row of this array contains a set of AutomationPatterns in which /// atleast one set should be supported by the element. /// ///Array of sets of required patterns. public AutomationPattern[][] GetRequiredPatternSets() { int totalRows = this._requiredPatternsSets.Length; AutomationPattern[][] clone = new AutomationPattern[totalRows][]; for (int i = 0; i < totalRows; i++) { clone[i] = (AutomationPattern[])this._requiredPatternsSets[i].Clone(); } return clone; } ////// Gets the array of never supported patterns. /// ///Array of never supported patterns. public AutomationPattern[] GetNeverSupportedPatterns() { return (AutomationPattern[])_neverSupportedPatterns.Clone(); } ////// Gets the array of required properties. /// ///Array of required properties. public AutomationProperty[] GetRequiredProperties() { return (AutomationProperty[])_requiredProperties.Clone(); } #endregion //------------------------------------------------------ // // Public Fields // //------------------------------------------------------ #region Public Fields ///ControlType ID: Button - a clickable button public static readonly ControlType Button = ControlType.Register(AutomationIdentifierGuids.Button_Control, "ControlType.Button", STID.LocalizedControlTypeButton, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern } }); ///ControlType ID: Calendar - A date choosing mechanism public static readonly ControlType Calendar = ControlType.Register(AutomationIdentifierGuids.Calendar_Control, "ControlType.Calendar", STID.LocalizedControlTypeCalendar, new AutomationPattern[][] { new AutomationPattern[] { GridPatternIdentifiers.Pattern, ValuePatternIdentifiers.Pattern, SelectionPatternIdentifiers.Pattern } }); ///ControlType ID: CheckBox - A toggleable checkbox public static readonly ControlType CheckBox = ControlType.Register(AutomationIdentifierGuids.CheckBox_Control, "ControlType.CheckBox", STID.LocalizedControlTypeCheckBox, new AutomationPattern[][] { new AutomationPattern[] { TogglePatternIdentifiers.Pattern } }); ///ControlType ID: ComboBox - An editable dropdown box public static readonly ControlType ComboBox = ControlType.Register(AutomationIdentifierGuids.ComboBox_Control, "ControlType.ComboBox", STID.LocalizedControlTypeComboBox, new AutomationPattern[][] { new AutomationPattern[] { SelectionPatternIdentifiers.Pattern, ExpandCollapsePatternIdentifiers.Pattern } }); ///ControlType ID: Edit - A simple area with a user changeable value public static readonly ControlType Edit = ControlType.Register(AutomationIdentifierGuids.Edit_Control, "ControlType.Edit", STID.LocalizedControlTypeEdit, new AutomationPattern[][] { new AutomationPattern[] { ValuePatternIdentifiers.Pattern } }); ///ControlType ID: Hyperlink - a clickable hyperlink public static readonly ControlType Hyperlink = ControlType.Register(AutomationIdentifierGuids.Hyperlink_Control, "ControlType.Hyperlink", STID.LocalizedControlTypeHyperlink, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern } }); ///ControlType ID: Image - a non-interactive image public static readonly ControlType Image = ControlType.Register(AutomationIdentifierGuids.Image_Control, "ControlType.Image", STID.LocalizedControlTypeImage); ///ControlType ID: ListItem - An Item in a ListView public static readonly ControlType ListItem = ControlType.Register(AutomationIdentifierGuids.ListItem_Control, "ControlType.ListItem", STID.LocalizedControlTypeListItem, new AutomationPattern[][] { new AutomationPattern[] { SelectionItemPatternIdentifiers.Pattern } }); ///ControlType ID: List - a Listview for making a selection public static readonly ControlType List = ControlType.Register(AutomationIdentifierGuids.List_Control, "ControlType.List", STID.LocalizedControlTypeListView, new AutomationPattern[][] { new AutomationPattern[] { SelectionPatternIdentifiers.Pattern, TablePatternIdentifiers.Pattern, GridPatternIdentifiers.Pattern, MultipleViewPatternIdentifiers.Pattern } }); ///ControlType ID: Menu - A menu, usually has menuitems in it public static readonly ControlType Menu = ControlType.Register(AutomationIdentifierGuids.Menu_Control, "ControlType.Menu", STID.LocalizedControlTypeMenu); ///ControlType ID: MenuBar - A menu-bar, usually populated with menubuttons public static readonly ControlType MenuBar = ControlType.Register(AutomationIdentifierGuids.MenuBar_Control, "ControlType.MenuBar", STID.LocalizedControlTypeMenuBar); ///ControlType ID: MenuItem - An item in a menu, usually clickable public static readonly ControlType MenuItem = ControlType.Register(AutomationIdentifierGuids.MenuItem_Control, "ControlType.MenuItem", STID.LocalizedControlTypeMenuItem, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern }, new AutomationPattern[] { ExpandCollapsePatternIdentifiers.Pattern }, new AutomationPattern[] { TogglePatternIdentifiers.Pattern }, }); ///ControlType ID: ProgressBar - Visually indicates the progress of a lengthy operation. public static readonly ControlType ProgressBar = ControlType.Register(AutomationIdentifierGuids.ProgressBar_Control, "ControlType.ProgressBar", STID.LocalizedControlTypeProgressBar, new AutomationPattern[][] { new AutomationPattern[] { ValuePatternIdentifiers.Pattern } }); ///ControlType ID: RadioButton - A selection mechanism allowing exactly 1 selected item in a group public static readonly ControlType RadioButton = ControlType.Register(AutomationIdentifierGuids.RadioButton_Control, "ControlType.RadioButton", STID.LocalizedControlTypeRadioButton); ///ControlType ID: ScrollBar - A Scrollbar, the value is usually used to control scrolling of a window public static readonly ControlType ScrollBar = ControlType.Register(AutomationIdentifierGuids.ScrollBar_Control, "ControlType.ScrollBar", STID.LocalizedControlTypeScrollBar); ///ControlType ID: Slider - A Slider, usually used to set a value public static readonly ControlType Slider = ControlType.Register(AutomationIdentifierGuids.Slider_Control, "ControlType.Slider", STID.LocalizedControlTypeSlider, new AutomationPattern[][] { new AutomationPattern[] { RangeValuePatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern } }); ///ControlType ID: Spinner - A Control that allows you to either enter a numeric value or scroll it up and down public static readonly ControlType Spinner = ControlType.Register(AutomationIdentifierGuids.Spinner_Control, "ControlType.Spinner", STID.LocalizedControlTypeSpinner, new AutomationPattern[][] { new AutomationPattern[] { RangeValuePatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern } }); ///ControlType ID: StatusBar - A bar used to report status of an application public static readonly ControlType StatusBar = ControlType.Register(AutomationIdentifierGuids.StatusBar_Control, "ControlType.StatusBar", STID.LocalizedControlTypeStatusBar); ///ControlType ID: Tab - A Tabbed window public static readonly ControlType Tab = ControlType.Register(AutomationIdentifierGuids.Tab_Control, "ControlType.Tab", STID.LocalizedControlTypeTab); ///ControlType ID: TabItem - An individual tab of a tabbed window public static readonly ControlType TabItem = ControlType.Register(AutomationIdentifierGuids.TabItem_Control, "ControlType.TabItem", STID.LocalizedControlTypeTabItem); ///ControlType ID: Image - a non-interactive text public static readonly ControlType Text = ControlType.Register(AutomationIdentifierGuids.Text_Control, "ControlType.Text", STID.LocalizedControlTypeText); ///ControlType ID: ToolBar - A Bar, usually full of buttons or other controls public static readonly ControlType ToolBar = ControlType.Register(AutomationIdentifierGuids.ToolBar_Control, "ControlType.ToolBar", STID.LocalizedControlTypeToolBar); ///ControlType ID: ToolTip - a small window that pops up containing helpful tips for using a control public static readonly ControlType ToolTip = ControlType.Register(AutomationIdentifierGuids.ToolTip_Control, "ControlType.ToolTip", STID.LocalizedControlTypeToolTip); ///ControlType ID: Tree - A display showing a hierarchy of specific items public static readonly ControlType Tree = ControlType.Register(AutomationIdentifierGuids.Tree_Control, "ControlType.Tree", STID.LocalizedControlTypeTreeView); ///ControlType ID: TreeItem - An individual item in a tree, usually able to be expanded to show its children public static readonly ControlType TreeItem = ControlType.Register(AutomationIdentifierGuids.TreeItem_Control, "ControlType.TreeItem", STID.LocalizedControlTypeTreeViewItem); ///ControlType ID: Custom - Generic ControlType used for anything not covered by another ControlType public static readonly ControlType Custom = ControlType.Register(AutomationIdentifierGuids.Custom_Control, "ControlType.Custom", STID.LocalizedControlTypeCustom); ///ControlType ID: Group - Is a separation in the automation tree so that items that are grouped together have a logical division with the structure. public static readonly ControlType Group = ControlType.Register(AutomationIdentifierGuids.Group_Control, "ControlType.Group", STID.LocalizedControlTypeGroup); ///ControlType ID: Thumb - It provides basic semantic input for dragging/resizing-like input behavior from the user. public static readonly ControlType Thumb = ControlType.Register(AutomationIdentifierGuids.Thumb_Control, "ControlType.Thumb", STID.LocalizedControlTypeThumb); ///ControlType ID: DataGrid - Lets a user easily work with items that contains metadata represented in columns. public static readonly ControlType DataGrid = ControlType.Register(AutomationIdentifierGuids.DataGrid_Control, "ControlType.DataGrid", STID.LocalizedControlTypeDataGrid, new AutomationPattern[][] { new AutomationPattern[] { GridPatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern }, new AutomationPattern[] { TablePatternIdentifiers.Pattern }, }); ///ControlType ID: DataItem - Is more complicated than the simple list item because it has a large amount of information stored within it. public static readonly ControlType DataItem = ControlType.Register(AutomationIdentifierGuids.DataItem_Control, "ControlType.DataItem", STID.LocalizedControlTypeDataItem, new AutomationPattern[][] { new AutomationPattern[] { SelectionItemPatternIdentifiers.Pattern } }); ///ControlType ID: Document - Lets a user view/manipulate multiple pages of text. public static readonly ControlType Document = ControlType.Register(AutomationIdentifierGuids.Document_Control, "ControlType.Document", STID.LocalizedControlTypeDocument, new AutomationProperty[0], new AutomationPattern[] { ValuePatternIdentifiers.Pattern }, new AutomationPattern[][] { new AutomationPattern[] { ScrollPatternIdentifiers.Pattern } , new AutomationPattern[] { TextPatternIdentifiers.Pattern } , }); ///ControlType ID: SplitButton - Enables the ability to both perform an action on the toolbar button and expand the control to a list of other possible actions that can be performed. public static readonly ControlType SplitButton = ControlType.Register(AutomationIdentifierGuids.SplitButton_Control, "ControlType.SplitButton", STID.LocalizedControlTypeSplitButton, new AutomationPattern[][] { new AutomationPattern[] { InvokePatternIdentifiers.Pattern }, new AutomationPattern[] { ExpandCollapsePatternIdentifiers.Pattern }, }); ///ControlType ID: Window - The object representing the window frame, which contains child objects such as a title bar, client, and other objects contained in a window. public static readonly ControlType Window = ControlType.Register(AutomationIdentifierGuids.Window_Control, "ControlType.Window", STID.LocalizedControlTypeWindow, new AutomationPattern[][] { new AutomationPattern[] { TransformPatternIdentifiers.Pattern }, new AutomationPattern[] { WindowPatternIdentifiers.Pattern }, }); ///ControlType ID: Pane - Simular to Window but does not support WindowPattern. public static readonly ControlType Pane = ControlType.Register(AutomationIdentifierGuids.Pane_Control, "ControlType.Pane", STID.LocalizedControlTypePane, new AutomationPattern[][] { new AutomationPattern[] { TransformPatternIdentifiers.Pattern } }); ///ControlType ID: Header - Provides a visual container for the labels for rows and/or columns of information. public static readonly ControlType Header = ControlType.Register(AutomationIdentifierGuids.Header_Control, "ControlType.Header", STID.LocalizedControlTypeHeader); ///ControlType ID: HeaderItem - Provides a visual label for a row and/or column of information. public static readonly ControlType HeaderItem = ControlType.Register(AutomationIdentifierGuids.HeaderItem_Control, "ControlType.HeaderItem", STID.LocalizedControlTypeHeaderItem); ///ControlType ID: Table - Simular to DataGrid but only contains text elements. public static readonly ControlType Table = ControlType.Register(AutomationIdentifierGuids.Table_Control, "ControlType.Table", STID.LocalizedControlTypeTable, new AutomationPattern[][] { new AutomationPattern[] { GridPatternIdentifiers.Pattern }, new AutomationPattern[] { SelectionPatternIdentifiers.Pattern }, new AutomationPattern[] { TablePatternIdentifiers.Pattern }, }); ///ControlType ID: TitleBar - The portion of a window that identifies the window. public static readonly ControlType TitleBar = ControlType.Register(AutomationIdentifierGuids.TitleBar_Control, "ControlType.TitleBar", STID.LocalizedControlTypeTitleBar); ///ControlType ID: Separator - An object that creates visual space in controls like menus and toolbars. public static readonly ControlType Separator = ControlType.Register(AutomationIdentifierGuids.Separator_Control, "ControlType.Separator", STID.LocalizedControlTypeSeparator); #endregion //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ///Returns the Localized control type public string LocalizedControlType { get { return ST.Get(_stId); } } #endregion //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPattern[][] _requiredPatternsSets; private AutomationPattern[] _neverSupportedPatterns; private AutomationProperty[] _requiredProperties; private STID _stId; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
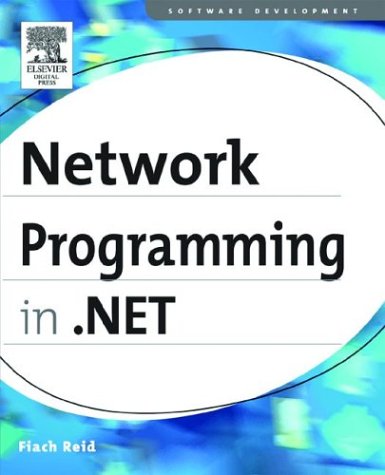
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CachedPathData.cs
- RadioButton.cs
- ProcessThreadCollection.cs
- externdll.cs
- util.cs
- KeyPressEvent.cs
- QueueProcessor.cs
- AnnouncementDispatcherAsyncResult.cs
- MTConfigUtil.cs
- AttachmentCollection.cs
- DataControlFieldCollection.cs
- PeerNameResolver.cs
- NavigationPropertySingletonExpression.cs
- TextElement.cs
- BitVector32.cs
- DoWorkEventArgs.cs
- RuleSetReference.cs
- TableLayoutPanel.cs
- InteropAutomationProvider.cs
- TemplateBindingExtensionConverter.cs
- FilteredSchemaElementLookUpTable.cs
- SqlXmlStorage.cs
- StreamHelper.cs
- Utils.cs
- recordstate.cs
- ClaimSet.cs
- TraceSection.cs
- InputDevice.cs
- querybuilder.cs
- SelectionEditingBehavior.cs
- DependencyObjectProvider.cs
- Code.cs
- MailHeaderInfo.cs
- ObjectQueryExecutionPlan.cs
- SpeakProgressEventArgs.cs
- Image.cs
- SpeechAudioFormatInfo.cs
- WinFormsSecurity.cs
- MouseEventArgs.cs
- StringBuilder.cs
- Tile.cs
- StringAnimationBase.cs
- WebServiceReceiveDesigner.cs
- ExpandSegment.cs
- PackagingUtilities.cs
- MailWebEventProvider.cs
- ClientSettingsProvider.cs
- TraceSwitch.cs
- Invariant.cs
- _TransmitFileOverlappedAsyncResult.cs
- MimeObjectFactory.cs
- FamilyMap.cs
- DetailsViewRow.cs
- VolatileEnlistmentMultiplexing.cs
- ManagedFilter.cs
- ContainerFilterService.cs
- IteratorDescriptor.cs
- nulltextcontainer.cs
- FlagsAttribute.cs
- CookieHandler.cs
- TextServicesCompartmentEventSink.cs
- HttpRawResponse.cs
- WorkflowInlining.cs
- QueryHandler.cs
- CodeComment.cs
- ImageFormatConverter.cs
- Polygon.cs
- SecurityTokenAuthenticator.cs
- control.ime.cs
- ContentDisposition.cs
- ProfileProvider.cs
- RecognizerInfo.cs
- ComponentEvent.cs
- SqlException.cs
- TraceContextRecord.cs
- Canvas.cs
- BitmapVisualManager.cs
- _ConnectionGroup.cs
- PrtTicket_Editor.cs
- Color.cs
- UrlRoutingHandler.cs
- ExtentCqlBlock.cs
- InkCanvasFeedbackAdorner.cs
- MetadataUtilsSmi.cs
- ScrollData.cs
- XmlDocumentFieldSchema.cs
- AssemblyAssociatedContentFileAttribute.cs
- DocumentPaginator.cs
- FragmentNavigationEventArgs.cs
- ClockController.cs
- ActivityExecutionContextCollection.cs
- ConfigXmlElement.cs
- MenuBase.cs
- WebBrowserDocumentCompletedEventHandler.cs
- ReadOnlyDictionary.cs
- Attributes.cs
- COM2ComponentEditor.cs
- HandlerBase.cs
- CancelEventArgs.cs
- TrustLevel.cs