Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / SqlClient / SqlException.cs / 1 / SqlException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using System; using System.ComponentModel; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; using System.Text; // StringBuilder [Serializable] #if WINFSInternalOnly internal #else public #endif sealed class SqlException : System.Data.Common.DbException { private SqlErrorCollection _errors; private SqlException(string message, SqlErrorCollection errorCollection) : base(message) { HResult = HResults.SqlException; _errors = errorCollection; } // runtime will call even if private... private SqlException(SerializationInfo si, StreamingContext sc) : base(si, sc) { _errors = (SqlErrorCollection) si.GetValue("Errors", typeof(SqlErrorCollection)); HResult = HResults.SqlException; } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] override public void GetObjectData(SerializationInfo si, StreamingContext context) { if (null == si) { throw new ArgumentNullException("si"); } si.AddValue("Errors", _errors, typeof(SqlErrorCollection)); base.GetObjectData(si, context); } [ DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public SqlErrorCollection Errors { get { if (_errors == null) { _errors = new SqlErrorCollection(); } return _errors; } } /*virtual protected*/private bool ShouldSerializeErrors() { // MDAC 65548 return ((null != _errors) && (0 < _errors.Count)); } public byte Class { get { return this.Errors[0].Class;} } public int LineNumber { get { return this.Errors[0].LineNumber;} } public int Number { get { return this.Errors[0].Number;} } public string Procedure { get { return this.Errors[0].Procedure;} } public string Server { get { return this.Errors[0].Server;} } public byte State { get { return this.Errors[0].State;} } override public string Source { get { return this.Errors[0].Source;} } static internal SqlException CreateException(SqlErrorCollection errorCollection, string serverVersion) { Debug.Assert(null != errorCollection, "no errorCollection?"); // concat all messages together MDAC 65533 StringBuilder message = new StringBuilder(); for (int i = 0; i < errorCollection.Count; i++) { if (i > 0) { message.Append(Environment.NewLine); } message.Append(errorCollection[i].Message); } SqlException exception = new SqlException(message.ToString(), errorCollection); exception.Data.Add("HelpLink.ProdName", "Microsoft SQL Server"); if (!ADP.IsEmpty(serverVersion)) { exception.Data.Add("HelpLink.ProdVer", serverVersion); } exception.Data.Add("HelpLink.EvtSrc", "MSSQLServer"); exception.Data.Add("HelpLink.EvtID", errorCollection[0].Number.ToString(CultureInfo.InvariantCulture)); exception.Data.Add("HelpLink.BaseHelpUrl", "http://go.microsoft.com/fwlink"); exception.Data.Add("HelpLink.LinkId", "20476"); return exception; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using System; using System.ComponentModel; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; using System.Text; // StringBuilder [Serializable] #if WINFSInternalOnly internal #else public #endif sealed class SqlException : System.Data.Common.DbException { private SqlErrorCollection _errors; private SqlException(string message, SqlErrorCollection errorCollection) : base(message) { HResult = HResults.SqlException; _errors = errorCollection; } // runtime will call even if private... private SqlException(SerializationInfo si, StreamingContext sc) : base(si, sc) { _errors = (SqlErrorCollection) si.GetValue("Errors", typeof(SqlErrorCollection)); HResult = HResults.SqlException; } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] override public void GetObjectData(SerializationInfo si, StreamingContext context) { if (null == si) { throw new ArgumentNullException("si"); } si.AddValue("Errors", _errors, typeof(SqlErrorCollection)); base.GetObjectData(si, context); } [ DesignerSerializationVisibility(DesignerSerializationVisibility.Content) ] public SqlErrorCollection Errors { get { if (_errors == null) { _errors = new SqlErrorCollection(); } return _errors; } } /*virtual protected*/private bool ShouldSerializeErrors() { // MDAC 65548 return ((null != _errors) && (0 < _errors.Count)); } public byte Class { get { return this.Errors[0].Class;} } public int LineNumber { get { return this.Errors[0].LineNumber;} } public int Number { get { return this.Errors[0].Number;} } public string Procedure { get { return this.Errors[0].Procedure;} } public string Server { get { return this.Errors[0].Server;} } public byte State { get { return this.Errors[0].State;} } override public string Source { get { return this.Errors[0].Source;} } static internal SqlException CreateException(SqlErrorCollection errorCollection, string serverVersion) { Debug.Assert(null != errorCollection, "no errorCollection?"); // concat all messages together MDAC 65533 StringBuilder message = new StringBuilder(); for (int i = 0; i < errorCollection.Count; i++) { if (i > 0) { message.Append(Environment.NewLine); } message.Append(errorCollection[i].Message); } SqlException exception = new SqlException(message.ToString(), errorCollection); exception.Data.Add("HelpLink.ProdName", "Microsoft SQL Server"); if (!ADP.IsEmpty(serverVersion)) { exception.Data.Add("HelpLink.ProdVer", serverVersion); } exception.Data.Add("HelpLink.EvtSrc", "MSSQLServer"); exception.Data.Add("HelpLink.EvtID", errorCollection[0].Number.ToString(CultureInfo.InvariantCulture)); exception.Data.Add("HelpLink.BaseHelpUrl", "http://go.microsoft.com/fwlink"); exception.Data.Add("HelpLink.LinkId", "20476"); return exception; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
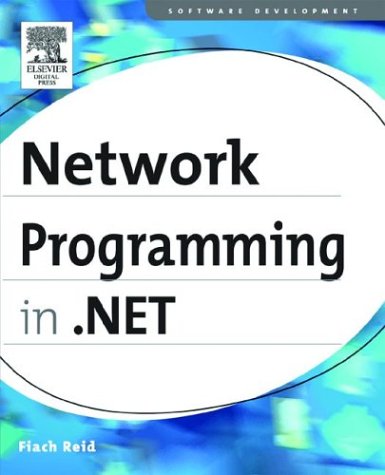
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuntimeHandles.cs
- FrugalMap.cs
- FileLevelControlBuilderAttribute.cs
- CustomErrorsSectionWrapper.cs
- Mappings.cs
- FixUp.cs
- SystemThemeKey.cs
- FileRecordSequenceHelper.cs
- TableHeaderCell.cs
- Polyline.cs
- IISMapPath.cs
- MultiAsyncResult.cs
- SystemIPInterfaceProperties.cs
- CacheMode.cs
- HttpHandlersSection.cs
- FileReservationCollection.cs
- GroupStyle.cs
- CodeCommentStatementCollection.cs
- ExcludeFromCodeCoverageAttribute.cs
- GenericIdentity.cs
- HealthMonitoringSectionHelper.cs
- DesignerActionPropertyItem.cs
- DescendantQuery.cs
- InputManager.cs
- Int64AnimationBase.cs
- oledbconnectionstring.cs
- DllNotFoundException.cs
- TableParaClient.cs
- RawKeyboardInputReport.cs
- AccessorTable.cs
- InternalCompensate.cs
- DataBindingHandlerAttribute.cs
- TextEffectCollection.cs
- ModuleConfigurationInfo.cs
- OrderedDictionary.cs
- Select.cs
- SettingsPropertyValue.cs
- WindowsListViewItemCheckBox.cs
- TdsValueSetter.cs
- DataGridTextBox.cs
- EntityDataReader.cs
- updateconfighost.cs
- ExpressionVisitor.cs
- AsymmetricSignatureFormatter.cs
- FormParameter.cs
- ToolStripSettings.cs
- Effect.cs
- LayoutEngine.cs
- WinInetCache.cs
- ConnectionString.cs
- ToolboxDataAttribute.cs
- XPathAncestorQuery.cs
- PasswordBox.cs
- Vector3D.cs
- QueryAccessibilityHelpEvent.cs
- ManipulationInertiaStartingEventArgs.cs
- WebSysDefaultValueAttribute.cs
- DocumentViewerConstants.cs
- DataGridViewColumn.cs
- RenameRuleObjectDialog.Designer.cs
- CompilerLocalReference.cs
- TreeNodeCollection.cs
- EntityDescriptor.cs
- OdbcFactory.cs
- storepermissionattribute.cs
- ResourceExpression.cs
- IndentedWriter.cs
- ScriptingRoleServiceSection.cs
- OdbcDataAdapter.cs
- RewritingSimplifier.cs
- TextWriterTraceListener.cs
- XmlValidatingReaderImpl.cs
- GroupLabel.cs
- DATA_BLOB.cs
- HandleRef.cs
- ConfigurationStrings.cs
- HtmlMeta.cs
- SettingsPropertyValueCollection.cs
- PixelShader.cs
- HybridObjectCache.cs
- VerbConverter.cs
- WsatConfiguration.cs
- TripleDESCryptoServiceProvider.cs
- ReadOnlyHierarchicalDataSource.cs
- StreamInfo.cs
- ListenDesigner.cs
- xamlnodes.cs
- ConfigXmlSignificantWhitespace.cs
- TextEffectCollection.cs
- _ChunkParse.cs
- StopStoryboard.cs
- FigureParaClient.cs
- FixedLineResult.cs
- TextSelectionHelper.cs
- BitHelper.cs
- ProcessDesigner.cs
- TextRangeEditLists.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- ZipIOCentralDirectoryFileHeader.cs
- MeasurementDCInfo.cs