Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / Util / OrderedDictionary.cs / 1 / OrderedDictionary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Util { using System; using System.Collections; using System.Collections.Generic; internal class OrderedDictionary: IDictionary { private Dictionary _dictionary; private List _keys; private List _values; // Cannot easily support ctor that takes IEqualityComparer, since List doesn't have an easy // way to use the IEqualityComparer. public OrderedDictionary() : this(0) { } public OrderedDictionary(int capacity) { _dictionary = new Dictionary (capacity); _keys = new List (capacity); _values = new List (capacity); } public int Count { get { return _dictionary.Count; } } public ICollection Keys { get { return _keys.AsReadOnly(); } } public TValue this[TKey key] { get { return _dictionary[key]; } set { // If key has already been added, we must first remove it from the lists so it is not // in the lists multiple times. RemoveFromLists(key); _dictionary[key] = value; _keys.Add(key); _values.Add(value); } } public ICollection Values { get { return _values.AsReadOnly(); } } public void Add(TKey key, TValue value) { // Dictionary.Add() will throw if it already contains key _dictionary.Add(key, value); _keys.Add(key); _values.Add(value); } public void Clear() { _dictionary.Clear(); _keys.Clear(); _values.Clear(); } public bool ContainsKey(TKey key) { return _dictionary.ContainsKey(key); } public bool ContainsValue(TValue value) { return _dictionary.ContainsValue(value); } public IEnumerator > GetEnumerator() { int i = 0; // Must use foreach instead of a for loop, since we want the underlying List enumerator to // throw an exception if the list is modified during enumeration. foreach (TKey key in _keys) { yield return new KeyValuePair (key, _values[i]); i++; } } private void RemoveFromLists(TKey key) { int index = _keys.IndexOf(key); if (index != -1) { _keys.RemoveAt(index); _values.RemoveAt(index); } } public bool Remove(TKey key) { RemoveFromLists(key); return _dictionary.Remove(key); } public bool TryGetValue(TKey key, out TValue value) { return _dictionary.TryGetValue(key, out value); } #region ICollection > Members bool ICollection >.IsReadOnly { get { return ((ICollection >)_dictionary).IsReadOnly; } } void ICollection >.Add(KeyValuePair item) { Add(item.Key, item.Value); } bool ICollection >.Contains(KeyValuePair item) { return ((ICollection >)_dictionary).Contains(item); } void ICollection >.CopyTo(KeyValuePair [] array, int arrayIndex) { ((ICollection >)_dictionary).CopyTo(array, arrayIndex); } bool ICollection >.Remove(KeyValuePair item) { bool removed = ((ICollection >)_dictionary).Remove(item); // Only remove from lists if it was removed from the dictionary, since the dictionary may contain // the key but not the value. if (removed) { RemoveFromLists(item.Key); } return removed; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Util { using System; using System.Collections; using System.Collections.Generic; internal class OrderedDictionary: IDictionary { private Dictionary _dictionary; private List _keys; private List _values; // Cannot easily support ctor that takes IEqualityComparer, since List doesn't have an easy // way to use the IEqualityComparer. public OrderedDictionary() : this(0) { } public OrderedDictionary(int capacity) { _dictionary = new Dictionary (capacity); _keys = new List (capacity); _values = new List (capacity); } public int Count { get { return _dictionary.Count; } } public ICollection Keys { get { return _keys.AsReadOnly(); } } public TValue this[TKey key] { get { return _dictionary[key]; } set { // If key has already been added, we must first remove it from the lists so it is not // in the lists multiple times. RemoveFromLists(key); _dictionary[key] = value; _keys.Add(key); _values.Add(value); } } public ICollection Values { get { return _values.AsReadOnly(); } } public void Add(TKey key, TValue value) { // Dictionary.Add() will throw if it already contains key _dictionary.Add(key, value); _keys.Add(key); _values.Add(value); } public void Clear() { _dictionary.Clear(); _keys.Clear(); _values.Clear(); } public bool ContainsKey(TKey key) { return _dictionary.ContainsKey(key); } public bool ContainsValue(TValue value) { return _dictionary.ContainsValue(value); } public IEnumerator > GetEnumerator() { int i = 0; // Must use foreach instead of a for loop, since we want the underlying List enumerator to // throw an exception if the list is modified during enumeration. foreach (TKey key in _keys) { yield return new KeyValuePair (key, _values[i]); i++; } } private void RemoveFromLists(TKey key) { int index = _keys.IndexOf(key); if (index != -1) { _keys.RemoveAt(index); _values.RemoveAt(index); } } public bool Remove(TKey key) { RemoveFromLists(key); return _dictionary.Remove(key); } public bool TryGetValue(TKey key, out TValue value) { return _dictionary.TryGetValue(key, out value); } #region ICollection > Members bool ICollection >.IsReadOnly { get { return ((ICollection >)_dictionary).IsReadOnly; } } void ICollection >.Add(KeyValuePair item) { Add(item.Key, item.Value); } bool ICollection >.Contains(KeyValuePair item) { return ((ICollection >)_dictionary).Contains(item); } void ICollection >.CopyTo(KeyValuePair [] array, int arrayIndex) { ((ICollection >)_dictionary).CopyTo(array, arrayIndex); } bool ICollection >.Remove(KeyValuePair item) { bool removed = ((ICollection >)_dictionary).Remove(item); // Only remove from lists if it was removed from the dictionary, since the dictionary may contain // the key but not the value. if (removed) { RemoveFromLists(item.Key); } return removed; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
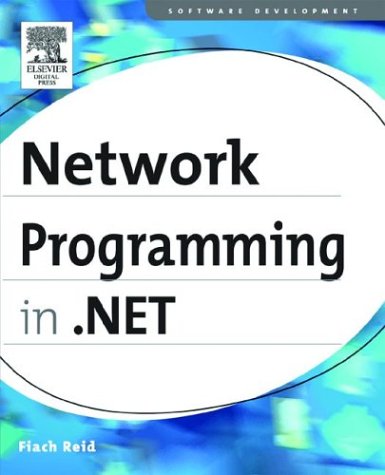
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommonProperties.cs
- DataGridViewToolTip.cs
- SRef.cs
- BaseValidatorDesigner.cs
- initElementDictionary.cs
- Win32KeyboardDevice.cs
- TextPointerBase.cs
- ComPlusThreadInitializer.cs
- Registry.cs
- WpfKnownMemberInvoker.cs
- datacache.cs
- TextEditorCharacters.cs
- HyperLink.cs
- NamedPipeAppDomainProtocolHandler.cs
- HebrewCalendar.cs
- IPCCacheManager.cs
- dsa.cs
- EdmComplexPropertyAttribute.cs
- SynchronizationScope.cs
- Timer.cs
- DoubleCollectionValueSerializer.cs
- TypeSystemHelpers.cs
- DbExpressionBuilder.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- CellQuery.cs
- PieceDirectory.cs
- VersionConverter.cs
- IList.cs
- MemoryResponseElement.cs
- SiteMapNodeItem.cs
- DbDataRecord.cs
- TreeView.cs
- ConvertTextFrag.cs
- SudsCommon.cs
- CodeCatchClause.cs
- OdbcDataReader.cs
- RepeatBehaviorConverter.cs
- DataGridViewCellCollection.cs
- IncrementalHitTester.cs
- DeferredTextReference.cs
- CodeIdentifiers.cs
- DataGridViewRowPrePaintEventArgs.cs
- XmlMtomWriter.cs
- MemoryMappedFileSecurity.cs
- XmlDocument.cs
- Matrix3D.cs
- PlaceHolder.cs
- ReferencedAssembly.cs
- HtmlControl.cs
- StorageEntityTypeMapping.cs
- ClosableStream.cs
- MsmqBindingBase.cs
- FloatUtil.cs
- PartialClassGenerationTask.cs
- ToolStripSystemRenderer.cs
- TemplateComponentConnector.cs
- ErrorReporting.cs
- Drawing.cs
- SafeReversePInvokeHandle.cs
- SelectQueryOperator.cs
- RegisteredHiddenField.cs
- SqlCachedBuffer.cs
- Context.cs
- WindowsGraphicsWrapper.cs
- ToolStripPanel.cs
- ToolStripItemRenderEventArgs.cs
- CryptoStream.cs
- StoryFragments.cs
- GlobalDataBindingHandler.cs
- LogicalExpressionEditor.cs
- Touch.cs
- DropSource.cs
- XPathParser.cs
- BuildProviderCollection.cs
- ConfigurationValues.cs
- DataControlImageButton.cs
- NotConverter.cs
- Compiler.cs
- Propagator.JoinPropagator.cs
- ProbeMatchesMessageCD1.cs
- KeyInstance.cs
- SaveCardRequest.cs
- AnimationLayer.cs
- BinaryConverter.cs
- HttpRuntime.cs
- WebPartCatalogAddVerb.cs
- XmlNullResolver.cs
- UseLicense.cs
- CacheSection.cs
- MasterPageBuildProvider.cs
- ControlTemplate.cs
- EventWaitHandle.cs
- TextFormatterHost.cs
- MetafileHeader.cs
- InboundActivityHelper.cs
- StrokeIntersection.cs
- EtwTrace.cs
- AppSettingsReader.cs
- PolyQuadraticBezierSegment.cs
- AttributeCollection.cs