Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / Odbc / DbDataRecord.cs / 1 / DbDataRecord.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.ComponentModel; //Component using System.Data; using System.Runtime.InteropServices; //Marshal using System.Reflection; //Missing namespace System.Data.Odbc { sealed internal class DbSchemaInfo { internal DbSchemaInfo() { } internal string _name; internal string _typename; internal Type _type; internal ODBC32.SQL_TYPE? _dbtype; internal object _scale; internal object _precision; // extension to allow BindCol // internal int _columnlength; // internal int _valueOffset; // offset to the data in the row buffer internal int _lengthOffset; // offset to the length in the row buffer internal ODBC32.SQL_C _sqlctype; // need this to bind the value internal ODBC32.SQL_TYPE _sql_type; // need that to properly marshal the value } ///////////////////////////////////////////////////////////////////////////// // Cache // // This is a on-demand cache, only caching what the user requests. // The reational is that for ForwardOnly access (the default and LCD of drivers) // we cannot obtain the data more than once, and even GetData(0) (to determine is-null) // still obtains data for fixed lenght types. // So simple code like: // if(!rReader.IsDBNull(i)) // rReader.GetInt32(i) // // Would fail, unless we cache on the IsDBNull call, and return the cached // item for GetInt32. This actually improves perf anyway, (even if the driver could // support it), since we are not making a seperate interop call... // We do not cache all columns, so reading out of order is still not // ///////////////////////////////////////////////////////////////////////////// sealed internal class DbCache { //Data private bool[] _isBadValue; private DbSchemaInfo[] _schema; private object[] _values; private OdbcDataReader _record; internal int _count; internal bool _randomaccess = true; //Constructor internal DbCache(OdbcDataReader record, int count) { _count = count; _record = record; _randomaccess = (!record.IsBehavior(CommandBehavior.SequentialAccess)); _values = new object[count]; _isBadValue = new bool[count]; } //Accessor internal object this[int i] { get { if(_isBadValue[i]) { OverflowException innerException = (OverflowException)Values[i]; throw new OverflowException(innerException.Message, innerException); } return Values[i]; } set { Values[i] = value; _isBadValue[i] = false; } } internal int Count { get { return _count; } } internal void InvalidateValue(int i) { _isBadValue[i] = true; } internal object[] Values { get { return _values; } } internal object AccessIndex(int i) { //Note: We could put this directly in this[i], instead of having an explicit overload. //However that means that EVERY access into the cache takes the hit of checking, so //something as simple as the following code would take two hits. It's nice not to //have to take the hit when you know what your doing. // // if(cache[i] == null) // .... // return cache[i]; object[] values = this.Values; if(_randomaccess) { //Random //Means that the user can ask for the values int any order (ie: out of order). // In order to acheive this on a forward only stream, we need to actually // retreive all the value in between so they can go back to values they've skipped for(int c = 0; c < i; c++) { if(values[c] == null) { values[c] = _record.GetValue(c); } } } return values[i]; } internal DbSchemaInfo GetSchema(int i) { if(_schema == null) { _schema = new DbSchemaInfo[Count]; } if(_schema[i] == null) { _schema[i] = new DbSchemaInfo(); } return _schema[i]; } internal void FlushValues() { //Set all objects to null (to explcitly release them) //Note: SchemaInfo remains the same for all rows - no need to reget those... int count = _values.Length; for(int i = 0; i < count; ++i) { _values[i] = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
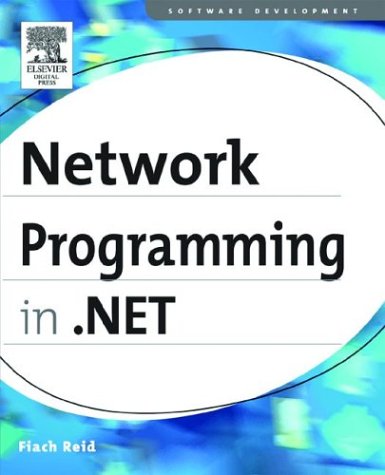
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlPointCollectionSerializer.cs
- Nullable.cs
- FunctionQuery.cs
- RadioButtonFlatAdapter.cs
- ScriptRegistrationManager.cs
- HttpHandlersSection.cs
- UpdateManifestForBrowserApplication.cs
- SHA384Cng.cs
- XamlClipboardData.cs
- Listbox.cs
- RenderData.cs
- Line.cs
- DataViewListener.cs
- ExtendedTransformFactory.cs
- ProxyWebPartConnectionCollection.cs
- EmptyImpersonationContext.cs
- TextAutomationPeer.cs
- ISFClipboardData.cs
- TransportDefaults.cs
- StateMachineHelpers.cs
- ConfigXmlDocument.cs
- EntityTypeBase.cs
- Int32Rect.cs
- StateManagedCollection.cs
- WindowsRegion.cs
- SqlUtils.cs
- LinqDataSourceValidationException.cs
- XmlDictionaryReader.cs
- _TransmitFileOverlappedAsyncResult.cs
- FormViewActionList.cs
- CodeValidator.cs
- followingquery.cs
- ResourceProperty.cs
- FixedNode.cs
- BaseCodePageEncoding.cs
- BadImageFormatException.cs
- VariableDesigner.xaml.cs
- ProviderSettings.cs
- ManagementEventWatcher.cs
- BitmapData.cs
- TypeSystemProvider.cs
- StrokeRenderer.cs
- CopyAction.cs
- FigureParaClient.cs
- XmlNullResolver.cs
- SmiEventSink_DeferedProcessing.cs
- ExpressionConverter.cs
- SqlClientWrapperSmiStream.cs
- RuleProcessor.cs
- LayoutManager.cs
- GeometryModel3D.cs
- AsymmetricCryptoHandle.cs
- Comparer.cs
- LineBreakRecord.cs
- MaterialCollection.cs
- EndpointConfigContainer.cs
- MessageBox.cs
- HatchBrush.cs
- XsdDateTime.cs
- AgileSafeNativeMemoryHandle.cs
- LocalizationComments.cs
- FormatterConverter.cs
- DataGridViewCellParsingEventArgs.cs
- SafeThreadHandle.cs
- ByteAnimation.cs
- HtmlSelect.cs
- TextRenderer.cs
- SortExpressionBuilder.cs
- ObjectSpanRewriter.cs
- Guid.cs
- WindowsClaimSet.cs
- handlecollector.cs
- XmlSchemaAnyAttribute.cs
- PageStatePersister.cs
- KeyedHashAlgorithm.cs
- WindowsImpersonationContext.cs
- GridViewColumnHeader.cs
- WrappedIUnknown.cs
- RemotingAttributes.cs
- EntityProviderFactory.cs
- SharedDp.cs
- UserControl.cs
- MimePart.cs
- UnsafeMethods.cs
- CollaborationHelperFunctions.cs
- AddInServer.cs
- ToolStripItemClickedEventArgs.cs
- HtmlShimManager.cs
- ServiceInstanceProvider.cs
- FontDifferentiator.cs
- UrlMappingsSection.cs
- ConnectionInterfaceCollection.cs
- FolderBrowserDialog.cs
- NaturalLanguageHyphenator.cs
- HelloMessageCD1.cs
- CustomCategoryAttribute.cs
- securitycriticaldataformultiplegetandset.cs
- SchemaCollectionCompiler.cs
- DataServiceStreamResponse.cs
- AccessedThroughPropertyAttribute.cs