Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / SlotInfo.cs / 1305376 / SlotInfo.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that keeps track of slot information in a CqlBlock internal class SlotInfo : InternalBase { #region Constructor // effects: Creates a SlotInfo for a CqlBlock X with information // about whether this slot is needed by X's parent // (isRequiredByParent), whether X projects it (isProjected) along with // the slot value (slot) and the output member path (for // regular/non-boolean slots) for the slot internal SlotInfo(bool isRequiredByParent, bool isProjected, ProjectedSlot slot, MemberPath memberPath) : this(isRequiredByParent, isProjected, slot, memberPath, false /* enforceNotNull */) { } // We need to ensure that _from variables are never null since // view generation uses 2-valued boolean logic. // If enforceNotNull=true, the generated CQL adds a condition (AND slot NOT NULL) // This flag is used only for boolean slots. internal SlotInfo(bool isRequiredByParent, bool isProjected, ProjectedSlot slot, MemberPath memberPath, bool enforceNotNull) { m_isRequiredByParent = isRequiredByParent; m_isProjected = isProjected; m_slot = slot; m_memberPath = memberPath; m_enforceNotNull = enforceNotNull; Debug.Assert(false == m_isRequiredByParent || m_slot != null, "Required slots cannot be null"); Debug.Assert(m_slot is AliasedSlot || (m_slot == null && m_memberPath == null) || // unused boolean slot (m_slot is BooleanProjectedSlot) == (m_memberPath == null), "If slot is boolean slot, there is no member path for it and vice-versa"); } #endregion #region Fields private bool m_isRequiredByParent; // If slot is required by the parent private bool m_isProjected; // If the node is capable of projecting this slot private ProjectedSlot m_slot; // The slot represented by this SlotInfo private MemberPath m_memberPath; // The output member path of this slot private bool m_enforceNotNull; // Whether to add AND NOT NULL to CQL #endregion #region Properties // effects: Returns true iff this slot is required by the CqlBlock's parent internal bool IsRequiredByParent { get { return m_isRequiredByParent; } } // effects: Returns true iff this slot is projected by this CqlBlock internal bool IsProjected { get { return m_isProjected; } } // effects: Returns the output memberpath of this slot internal MemberPath MemberPath { get { return m_memberPath; } } // effects: Returns the slot corresponfing to this object internal ProjectedSlot SlotValue { get { return m_slot; } } // effects: Returns the Cql Alias for this slot, e.g., CPerson1_Pid, _from0 internal string CqlFieldAlias { get { return m_slot.CqlFieldAlias(m_memberPath); } } // effects: Returns true if CQL generated for slot needs to have an extra AND IS NOT NULL condition internal bool IsEnforcedNotNull { get { return m_enforceNotNull; } } #endregion #region Methods // effects: Sets the isrequiredbyparent to false. Note we don't have // a setter because we don't want people to set this field to true // after the object has been created internal void ResetIsRequiredByParent() { m_isRequiredByParent = false; } // effects: Modifies builder to contain a Cql representation of this // and returns the modified builder. For different slots, the result // is different, e.g., _from0, CPerson1.pid, TREAT(....) internal StringBuilder AsCql(StringBuilder builder, string blockAlias, int indentLevel) { if (m_enforceNotNull) { builder.Append('('); m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); builder.Append(" AND "); m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); builder.Append(" IS NOT NULL)"); } else { m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); } return builder; } internal override void ToCompactString(StringBuilder builder) { if (m_slot != null) { builder.Append(CqlFieldAlias); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that keeps track of slot information in a CqlBlock internal class SlotInfo : InternalBase { #region Constructor // effects: Creates a SlotInfo for a CqlBlock X with information // about whether this slot is needed by X's parent // (isRequiredByParent), whether X projects it (isProjected) along with // the slot value (slot) and the output member path (for // regular/non-boolean slots) for the slot internal SlotInfo(bool isRequiredByParent, bool isProjected, ProjectedSlot slot, MemberPath memberPath) : this(isRequiredByParent, isProjected, slot, memberPath, false /* enforceNotNull */) { } // We need to ensure that _from variables are never null since // view generation uses 2-valued boolean logic. // If enforceNotNull=true, the generated CQL adds a condition (AND slot NOT NULL) // This flag is used only for boolean slots. internal SlotInfo(bool isRequiredByParent, bool isProjected, ProjectedSlot slot, MemberPath memberPath, bool enforceNotNull) { m_isRequiredByParent = isRequiredByParent; m_isProjected = isProjected; m_slot = slot; m_memberPath = memberPath; m_enforceNotNull = enforceNotNull; Debug.Assert(false == m_isRequiredByParent || m_slot != null, "Required slots cannot be null"); Debug.Assert(m_slot is AliasedSlot || (m_slot == null && m_memberPath == null) || // unused boolean slot (m_slot is BooleanProjectedSlot) == (m_memberPath == null), "If slot is boolean slot, there is no member path for it and vice-versa"); } #endregion #region Fields private bool m_isRequiredByParent; // If slot is required by the parent private bool m_isProjected; // If the node is capable of projecting this slot private ProjectedSlot m_slot; // The slot represented by this SlotInfo private MemberPath m_memberPath; // The output member path of this slot private bool m_enforceNotNull; // Whether to add AND NOT NULL to CQL #endregion #region Properties // effects: Returns true iff this slot is required by the CqlBlock's parent internal bool IsRequiredByParent { get { return m_isRequiredByParent; } } // effects: Returns true iff this slot is projected by this CqlBlock internal bool IsProjected { get { return m_isProjected; } } // effects: Returns the output memberpath of this slot internal MemberPath MemberPath { get { return m_memberPath; } } // effects: Returns the slot corresponfing to this object internal ProjectedSlot SlotValue { get { return m_slot; } } // effects: Returns the Cql Alias for this slot, e.g., CPerson1_Pid, _from0 internal string CqlFieldAlias { get { return m_slot.CqlFieldAlias(m_memberPath); } } // effects: Returns true if CQL generated for slot needs to have an extra AND IS NOT NULL condition internal bool IsEnforcedNotNull { get { return m_enforceNotNull; } } #endregion #region Methods // effects: Sets the isrequiredbyparent to false. Note we don't have // a setter because we don't want people to set this field to true // after the object has been created internal void ResetIsRequiredByParent() { m_isRequiredByParent = false; } // effects: Modifies builder to contain a Cql representation of this // and returns the modified builder. For different slots, the result // is different, e.g., _from0, CPerson1.pid, TREAT(....) internal StringBuilder AsCql(StringBuilder builder, string blockAlias, int indentLevel) { if (m_enforceNotNull) { builder.Append('('); m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); builder.Append(" AND "); m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); builder.Append(" IS NOT NULL)"); } else { m_slot.AsCql(builder, m_memberPath, blockAlias, indentLevel); } return builder; } internal override void ToCompactString(StringBuilder builder) { if (m_slot != null) { builder.Append(CqlFieldAlias); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
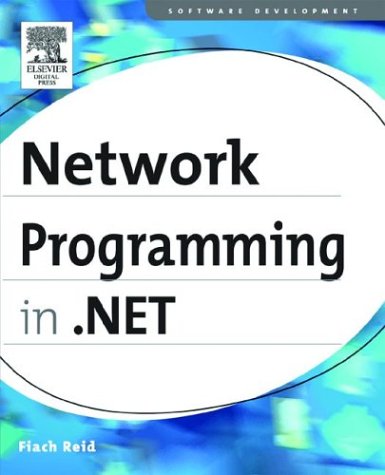
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExtendedPropertiesHandler.cs
- ThemeableAttribute.cs
- DataDocumentXPathNavigator.cs
- MeshGeometry3D.cs
- ConfigXmlReader.cs
- ConnectionPoint.cs
- SerialReceived.cs
- Label.cs
- BindableAttribute.cs
- DynamicEndpoint.cs
- WorkflowElementDialog.cs
- BrowserCapabilitiesFactory.cs
- XamlSerializerUtil.cs
- CommentAction.cs
- RecognizedAudio.cs
- TimeStampChecker.cs
- DesignerLoader.cs
- SerialErrors.cs
- InputLanguageEventArgs.cs
- IndexerReference.cs
- storepermission.cs
- FocusWithinProperty.cs
- ViewManager.cs
- VisualBrush.cs
- ListChangedEventArgs.cs
- MemoryPressure.cs
- FontResourceCache.cs
- WizardDesigner.cs
- WebBrowserDocumentCompletedEventHandler.cs
- GeometryModel3D.cs
- SecurityElement.cs
- HtmlInputFile.cs
- NamedPipeHostedTransportConfiguration.cs
- GridViewRowPresenterBase.cs
- XPathCompileException.cs
- XmlBaseWriter.cs
- ConnectionStringSettings.cs
- SecurityCriticalDataForSet.cs
- TemplatedMailWebEventProvider.cs
- WindowHideOrCloseTracker.cs
- Message.cs
- SimpleApplicationHost.cs
- ComponentResourceKeyConverter.cs
- HttpInputStream.cs
- CursorInteropHelper.cs
- Binding.cs
- EncodedStreamFactory.cs
- DecoderNLS.cs
- RecordsAffectedEventArgs.cs
- ToolboxItem.cs
- ProgramPublisher.cs
- PenCursorManager.cs
- SmtpMail.cs
- DateTimeOffsetConverter.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- DelegateBodyWriter.cs
- SwitchAttribute.cs
- PageContentCollection.cs
- ConfigurationValidatorAttribute.cs
- PathFigureCollection.cs
- PostBackOptions.cs
- AddressAlreadyInUseException.cs
- SoapInteropTypes.cs
- DependentList.cs
- ProfileManager.cs
- ToolStripScrollButton.cs
- RegionData.cs
- PersonalizationStateInfo.cs
- WebMessageEncodingBindingElement.cs
- DesigntimeLicenseContext.cs
- EventProxy.cs
- PrivilegeNotHeldException.cs
- XmlSchemaExternal.cs
- RelationshipConverter.cs
- HtmlImage.cs
- OpCopier.cs
- QilInvoke.cs
- FrameworkElementFactoryMarkupObject.cs
- IndentedWriter.cs
- IteratorFilter.cs
- ToolStripPanelSelectionGlyph.cs
- ToolboxItemFilterAttribute.cs
- WpfXamlType.cs
- OpenFileDialog.cs
- DatagridviewDisplayedBandsData.cs
- SectionRecord.cs
- ImageClickEventArgs.cs
- DNS.cs
- QueryStringParameter.cs
- DropSource.cs
- DiscriminatorMap.cs
- ControlSerializer.cs
- DataGridViewCellValidatingEventArgs.cs
- AuthenticationSection.cs
- NativeRecognizer.cs
- XmlWriterTraceListener.cs
- ParameterReplacerVisitor.cs
- MSAANativeProvider.cs
- ConnectionConsumerAttribute.cs
- bindurihelper.cs