Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / KnowledgeBase.cs / 2 / KnowledgeBase.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; namespace System.Data.Common.Utils.Boolean { ////// Data structure supporting storage of facts and proof (resolution) of queries given /// those facts. /// /// For instance, we may know the following facts: /// /// A --> B /// A /// /// Given these facts, the knowledge base can prove the query: /// /// B /// /// through resolution. /// ///Type of leaf term identifiers in fact expressions. internal class KnowledgeBase{ private readonly List > _facts; private Vertex _knowledge; private readonly ConversionContext _context; /// /// Initialize a new knowledge base. /// internal KnowledgeBase() { _facts = new List>(); _knowledge = Vertex.One; // we know '1', but nothing else at present _context = IdentifierService .Instance.CreateConversionContext(); } /// /// Adds all facts from another knowledge base /// /// The other knowledge base internal void AddKnowledgeBase(KnowledgeBasekb) { foreach (BoolExpr fact in kb._facts) { AddFact(fact); } } /// /// Adds the given fact to this KB. /// /// Simple fact. internal virtual void AddFact(BoolExprfact) { _facts.Add(fact); Converter converter = new Converter (fact, _context); Vertex factVertex = converter.Vertex; _knowledge = _context.Solver.And(_knowledge, factVertex); } /// /// Adds the given implication to this KB, where implication is of the form: /// /// condition --> implies /// /// Condition /// Entailed expression internal void AddImplication(BoolExprcondition, BoolExpr implies) { AddFact(new Implication(condition, implies)); } /// /// Adds an equivalence to this KB, of the form: /// /// left iff. right /// /// Left operand /// Right operand internal void AddEquivalence(BoolExprleft, BoolExpr right) { AddFact(new Equivalence(left, right)); } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.AppendLine("Facts:"); foreach (BoolExpr fact in _facts) { builder.Append("\t").AppendLine(fact.ToString()); } return builder.ToString(); } // Private class improving debugging output for implication facts // (fact appears as A --> B rather than !A + B) private class Implication : OrExpr { BoolExpr _condition; BoolExpr _implies; // (condition --> implies) iff. (!condition OR implies) internal Implication(BoolExpr condition, BoolExpr implies) : base(condition.MakeNegated(), implies) { _condition = condition; _implies = implies; } public override string ToString() { return StringUtil.FormatInvariant("{0} --> {1}", _condition, _implies); } } // Private class improving debugging output for equivalence facts // (fact appears as A <--> B rather than (!A + B) . (A + !B)) private class Equivalence : AndExpr { BoolExpr _left; BoolExpr _right; // (left iff. right) iff. (left --> right AND right --> left) internal Equivalence(BoolExpr left, BoolExpr right) : base(new Implication(left, right), new Implication(right, left)) { _left = left; _right = right; } public override string ToString() { return StringUtil.FormatInvariant("{0} <--> {1}", _left, _right); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; namespace System.Data.Common.Utils.Boolean { ////// Data structure supporting storage of facts and proof (resolution) of queries given /// those facts. /// /// For instance, we may know the following facts: /// /// A --> B /// A /// /// Given these facts, the knowledge base can prove the query: /// /// B /// /// through resolution. /// ///Type of leaf term identifiers in fact expressions. internal class KnowledgeBase{ private readonly List > _facts; private Vertex _knowledge; private readonly ConversionContext _context; /// /// Initialize a new knowledge base. /// internal KnowledgeBase() { _facts = new List>(); _knowledge = Vertex.One; // we know '1', but nothing else at present _context = IdentifierService .Instance.CreateConversionContext(); } /// /// Adds all facts from another knowledge base /// /// The other knowledge base internal void AddKnowledgeBase(KnowledgeBasekb) { foreach (BoolExpr fact in kb._facts) { AddFact(fact); } } /// /// Adds the given fact to this KB. /// /// Simple fact. internal virtual void AddFact(BoolExprfact) { _facts.Add(fact); Converter converter = new Converter (fact, _context); Vertex factVertex = converter.Vertex; _knowledge = _context.Solver.And(_knowledge, factVertex); } /// /// Adds the given implication to this KB, where implication is of the form: /// /// condition --> implies /// /// Condition /// Entailed expression internal void AddImplication(BoolExprcondition, BoolExpr implies) { AddFact(new Implication(condition, implies)); } /// /// Adds an equivalence to this KB, of the form: /// /// left iff. right /// /// Left operand /// Right operand internal void AddEquivalence(BoolExprleft, BoolExpr right) { AddFact(new Equivalence(left, right)); } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.AppendLine("Facts:"); foreach (BoolExpr fact in _facts) { builder.Append("\t").AppendLine(fact.ToString()); } return builder.ToString(); } // Private class improving debugging output for implication facts // (fact appears as A --> B rather than !A + B) private class Implication : OrExpr { BoolExpr _condition; BoolExpr _implies; // (condition --> implies) iff. (!condition OR implies) internal Implication(BoolExpr condition, BoolExpr implies) : base(condition.MakeNegated(), implies) { _condition = condition; _implies = implies; } public override string ToString() { return StringUtil.FormatInvariant("{0} --> {1}", _condition, _implies); } } // Private class improving debugging output for equivalence facts // (fact appears as A <--> B rather than (!A + B) . (A + !B)) private class Equivalence : AndExpr { BoolExpr _left; BoolExpr _right; // (left iff. right) iff. (left --> right AND right --> left) internal Equivalence(BoolExpr left, BoolExpr right) : base(new Implication(left, right), new Implication(right, left)) { _left = left; _right = right; } public override string ToString() { return StringUtil.FormatInvariant("{0} <--> {1}", _left, _right); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
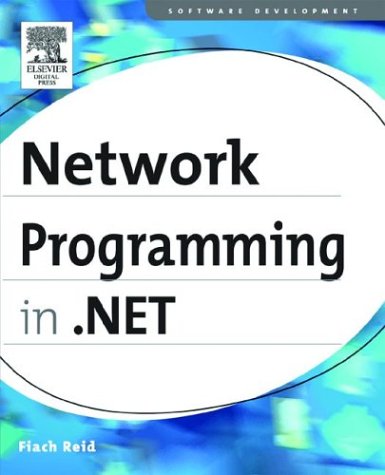
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Graphics.cs
- BatchParser.cs
- ValueChangedEventManager.cs
- ProcessInputEventArgs.cs
- TreeViewTemplateSelector.cs
- UnsafeNativeMethods.cs
- unsafenativemethodsother.cs
- TypeContext.cs
- Point4DValueSerializer.cs
- TextElementEnumerator.cs
- LowerCaseStringConverter.cs
- Int64KeyFrameCollection.cs
- SecurityCriticalDataForSet.cs
- AxHostDesigner.cs
- ClientRuntimeConfig.cs
- ObjectSecurity.cs
- RangeExpression.cs
- Preprocessor.cs
- SafeRegistryHandle.cs
- ConsoleEntryPoint.cs
- selecteditemcollection.cs
- HeaderedContentControl.cs
- DataSourceNameHandler.cs
- RootDesignerSerializerAttribute.cs
- PropertyEntry.cs
- HwndSourceParameters.cs
- NameValueCollection.cs
- EditingCommands.cs
- OrderedHashRepartitionEnumerator.cs
- Color.cs
- EntityClassGenerator.cs
- ThreadExceptionEvent.cs
- GlyphCache.cs
- DesignerDataView.cs
- XmlRawWriter.cs
- ReceiveActivityDesignerTheme.cs
- TranslateTransform.cs
- RowType.cs
- MarkupCompiler.cs
- ZoneMembershipCondition.cs
- CodePageUtils.cs
- _AuthenticationState.cs
- RemotingException.cs
- ListSortDescription.cs
- JsonEnumDataContract.cs
- RecognizeCompletedEventArgs.cs
- MemberDescriptor.cs
- LinqDataSourceStatusEventArgs.cs
- AsyncParams.cs
- InProcStateClientManager.cs
- XmlBoundElement.cs
- ProjectionCamera.cs
- DebugControllerThread.cs
- QuaternionConverter.cs
- ShaderEffect.cs
- UIElement3D.cs
- TextSchema.cs
- FontStyleConverter.cs
- Set.cs
- DesignTimeTemplateParser.cs
- FormViewDeletedEventArgs.cs
- SafeNativeHandle.cs
- StronglyTypedResourceBuilder.cs
- HttpPostedFileBase.cs
- AttachedPropertyMethodSelector.cs
- OutputCacheSettingsSection.cs
- ReadOnlyDataSource.cs
- ImageListDesigner.cs
- ChtmlPhoneCallAdapter.cs
- DropShadowEffect.cs
- PolicyException.cs
- HtmlForm.cs
- Camera.cs
- ScriptControl.cs
- DynamicActivity.cs
- WorkflowServiceHostFactory.cs
- FormsAuthenticationUserCollection.cs
- bidPrivateBase.cs
- WsatTransactionFormatter.cs
- TableHeaderCell.cs
- ListDictionary.cs
- BinaryReader.cs
- AnnotationHelper.cs
- RepeaterItemEventArgs.cs
- PrtCap_Base.cs
- TrustManager.cs
- X509Extension.cs
- RepeaterItem.cs
- FrameworkName.cs
- ActivityDesignerAccessibleObject.cs
- StrokeCollectionConverter.cs
- XmlSchemaElement.cs
- SizeChangedEventArgs.cs
- MachineSettingsSection.cs
- SafeCertificateContext.cs
- OrderedDictionaryStateHelper.cs
- ColumnWidthChangedEvent.cs
- TextParaClient.cs
- RemoteWebConfigurationHostServer.cs
- AssemblyFilter.cs