Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / QueryRewriting / RoleBoolean.cs / 1 / RoleBoolean.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils.Boolean; using System.Text; using System.Collections.Generic; using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.QueryRewriting { using DomainBoolExpr = BoolExpr>; using DomainNotExpr = NotExpr >; using DomainTermExpr = TermExpr >; /// /// Denotes the fact that the key of the current tuple comes from a specific extent, or association role /// internal class RoleBoolean : TrueFalseLiteral { #region Constructor internal RoleBoolean(EntitySetBase extent) { m_metadataItem = extent; } internal RoleBoolean(AssociationSetEnd end) { m_metadataItem = end; } #endregion #region Fields private MetadataItem m_metadataItem; #endregion #region BoolLiteral members internal override StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { ToCompactString(builder); builder.Append("("); builder.Append(blockAlias); builder.Append(")"); return builder; } internal override StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { AssociationSetEnd end = m_metadataItem as AssociationSetEnd; if (end != null) { builder.Append(Strings.ViewGen_AssociationSet_AsUserString(blockAlias, end.Name, end.ParentAssociationSet)); } else { builder.Append(Strings.ViewGen_EntitySet_AsUserString(blockAlias, m_metadataItem.ToString())); } return builder; } internal override StringBuilder AsNegatedUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { AssociationSetEnd end = m_metadataItem as AssociationSetEnd; if (end != null) { builder.Append(Strings.ViewGen_AssociationSet_AsUserString_Negated(blockAlias, end.Name, end.ParentAssociationSet)); } else { builder.Append(Strings.ViewGen_EntitySet_AsUserString_Negated(blockAlias, m_metadataItem.ToString())); } return builder; } internal override void GetRequiredSlots(MemberPathMapBase projectedSlotMap, bool[] requiredSlots) { throw new NotImplementedException(); } protected override bool IsEqualTo(BoolLiteral right) { RoleBoolean rightBoolean = right as RoleBoolean; if (rightBoolean == null) { return false; } return m_metadataItem == rightBoolean.m_metadataItem; } protected override int GetHash() { return m_metadataItem.GetHashCode(); } // effects: See BoolExpression.RemapBool internal override BoolLiteral RemapBool(Dictionaryremap) { return this; } internal override bool CheckRepInvariant() { return true; } #endregion #region Other Methods internal override void ToCompactString(StringBuilder builder) { AssociationSetEnd end = m_metadataItem as AssociationSetEnd; if (end != null) { builder.Append("InEnd:" + end.ParentAssociationSet + "_" + end.Name); } else { builder.Append("InSet:" + m_metadataItem.ToString()); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils.Boolean; using System.Text; using System.Collections.Generic; using System.Data.Common.Utils; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Mapping.ViewGeneration.Utils; using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.Mapping.ViewGeneration.QueryRewriting { using DomainBoolExpr = BoolExpr>; using DomainNotExpr = NotExpr >; using DomainTermExpr = TermExpr >; /// /// Denotes the fact that the key of the current tuple comes from a specific extent, or association role /// internal class RoleBoolean : TrueFalseLiteral { #region Constructor internal RoleBoolean(EntitySetBase extent) { m_metadataItem = extent; } internal RoleBoolean(AssociationSetEnd end) { m_metadataItem = end; } #endregion #region Fields private MetadataItem m_metadataItem; #endregion #region BoolLiteral members internal override StringBuilder AsCql(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { ToCompactString(builder); builder.Append("("); builder.Append(blockAlias); builder.Append(")"); return builder; } internal override StringBuilder AsUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { AssociationSetEnd end = m_metadataItem as AssociationSetEnd; if (end != null) { builder.Append(Strings.ViewGen_AssociationSet_AsUserString(blockAlias, end.Name, end.ParentAssociationSet)); } else { builder.Append(Strings.ViewGen_EntitySet_AsUserString(blockAlias, m_metadataItem.ToString())); } return builder; } internal override StringBuilder AsNegatedUserString(StringBuilder builder, string blockAlias, bool canSkipIsNotNull) { AssociationSetEnd end = m_metadataItem as AssociationSetEnd; if (end != null) { builder.Append(Strings.ViewGen_AssociationSet_AsUserString_Negated(blockAlias, end.Name, end.ParentAssociationSet)); } else { builder.Append(Strings.ViewGen_EntitySet_AsUserString_Negated(blockAlias, m_metadataItem.ToString())); } return builder; } internal override void GetRequiredSlots(MemberPathMapBase projectedSlotMap, bool[] requiredSlots) { throw new NotImplementedException(); } protected override bool IsEqualTo(BoolLiteral right) { RoleBoolean rightBoolean = right as RoleBoolean; if (rightBoolean == null) { return false; } return m_metadataItem == rightBoolean.m_metadataItem; } protected override int GetHash() { return m_metadataItem.GetHashCode(); } // effects: See BoolExpression.RemapBool internal override BoolLiteral RemapBool(Dictionaryremap) { return this; } internal override bool CheckRepInvariant() { return true; } #endregion #region Other Methods internal override void ToCompactString(StringBuilder builder) { AssociationSetEnd end = m_metadataItem as AssociationSetEnd; if (end != null) { builder.Append("InEnd:" + end.ParentAssociationSet + "_" + end.Name); } else { builder.Append("InSet:" + m_metadataItem.ToString()); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
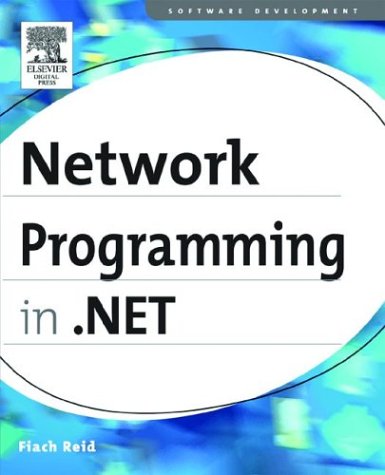
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberPath.cs
- HMACSHA512.cs
- InvalidDataException.cs
- DrawingBrush.cs
- RsaSecurityTokenAuthenticator.cs
- StringValidator.cs
- ProviderMetadata.cs
- _ConnectionGroup.cs
- XPathQueryGenerator.cs
- TakeOrSkipQueryOperator.cs
- CuspData.cs
- TabPanel.cs
- MemberPath.cs
- QilDataSource.cs
- BreakRecordTable.cs
- StreamedFramingRequestChannel.cs
- SqlTriggerContext.cs
- DataContractAttribute.cs
- CodeArrayCreateExpression.cs
- UserControlCodeDomTreeGenerator.cs
- PieceNameHelper.cs
- VariableAction.cs
- VerificationException.cs
- AnnotationComponentChooser.cs
- StylusPointPropertyUnit.cs
- _LoggingObject.cs
- FocusChangedEventArgs.cs
- WpfPayload.cs
- WebPartPersonalization.cs
- Input.cs
- Odbc32.cs
- InnerItemCollectionView.cs
- ProvidersHelper.cs
- UserNameSecurityToken.cs
- Math.cs
- WebPartManager.cs
- SubMenuStyleCollection.cs
- SkewTransform.cs
- TimeSpan.cs
- ApplyTemplatesAction.cs
- MarkupExtensionSerializer.cs
- DataColumnPropertyDescriptor.cs
- DocumentViewerBase.cs
- SizeAnimation.cs
- QueryRewriter.cs
- SamlAuthenticationClaimResource.cs
- WebPartsSection.cs
- XmlSerializerNamespaces.cs
- DecimalConstantAttribute.cs
- storepermissionattribute.cs
- NumericExpr.cs
- FormViewRow.cs
- ProfileService.cs
- PowerModeChangedEventArgs.cs
- CodeDirectiveCollection.cs
- DiffuseMaterial.cs
- DataGridLinkButton.cs
- SecurityDescriptor.cs
- File.cs
- ISAPIApplicationHost.cs
- ColorConvertedBitmap.cs
- OdbcParameterCollection.cs
- AsnEncodedData.cs
- InkCanvasFeedbackAdorner.cs
- XmlSchemaComplexContent.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- XhtmlBasicPageAdapter.cs
- TemplateColumn.cs
- HashSetDebugView.cs
- ConfigDefinitionUpdates.cs
- RealProxy.cs
- DataErrorValidationRule.cs
- AsymmetricSignatureDeformatter.cs
- NullableDoubleMinMaxAggregationOperator.cs
- OdbcUtils.cs
- RSAPKCS1SignatureFormatter.cs
- BulletDecorator.cs
- PenThread.cs
- TreeView.cs
- StyleHelper.cs
- XmlValidatingReader.cs
- EndpointDesigner.cs
- _DisconnectOverlappedAsyncResult.cs
- ResolveNameEventArgs.cs
- Block.cs
- DataSourceXmlElementAttribute.cs
- XmlTypeMapping.cs
- BufferedStream.cs
- ZipPackage.cs
- CodeMemberMethod.cs
- X509ClientCertificateCredentialsElement.cs
- Exception.cs
- DesignerActionMethodItem.cs
- QualifiedCellIdBoolean.cs
- StateMachineSubscriptionManager.cs
- TextFormatterImp.cs
- DescendantBaseQuery.cs
- ConstructorArgumentAttribute.cs
- DataBindingHandlerAttribute.cs
- IPPacketInformation.cs