Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / ImportRequest.cs / 1 / ImportRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Security.Principal; using System.Diagnostics; using System.Threading; //ManualResetEvent using System.ComponentModel; //Win32Exception using System.IO; //Stream using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using Microsoft.InfoCards.Diagnostics; // // This class is an instance of a request to import a card from a file. // It starts up the UI in the 'Import' mode. // class ImportRequest : ClientUIRequest { FileStream m_importFile; //keep a read pointer to the file string m_filename; public ImportRequest( Process callingProcess, WindowsIdentity callingIdentity, InfoCardUIAgent uiAgent, IntPtr rpcHandle, Stream inArgs, Stream outArgs ) : base( callingProcess, callingIdentity, uiAgent, rpcHandle, inArgs, outArgs, InfoCardUIAgent.CallMode.Import, ExceptionList.AllNonFatal ) { } public string ImportedFile { get{ return m_filename; } } // // No input args // protected override void OnMarshalInArgs() { BinaryReader breader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); string filename = Utility.DeserializeString( breader ); if (String.IsNullOrEmpty(filename) || filename.Length > Constants.Maxima.FileNameLength) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFileName ) ) ); } if( !Path.IsPathRooted( filename ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFileName) ) ); } try { m_filename = filename; m_importFile = new FileStream( m_filename, FileMode.Open, FileAccess.Read, FileShare.Read ); } catch( ArgumentException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( IOException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( NotSupportedException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( UnauthorizedAccessException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } } protected override void OnProcess() { // // ClientUiRequest's member function // StartAndWaitForUIAgent(); } // // No Output args. // protected override void OnMarshalOutArgs() { } protected override void OnDisposeAsUser() { base.OnDisposeAsUser(); if( null != m_importFile ) { m_importFile.Dispose(); m_importFile = null; } } // // Summary // Gets called a) if there was an exception in client processing // or b) if there was an exception in the child's request processing // protected override bool OnHandleException( Exception e, out int errorCode ) { errorCode = 0; bool handled = false; if( e is UserCancelledException ) { errorCode = (e as UserCancelledException).NativeHResult; handled = true; } return handled; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
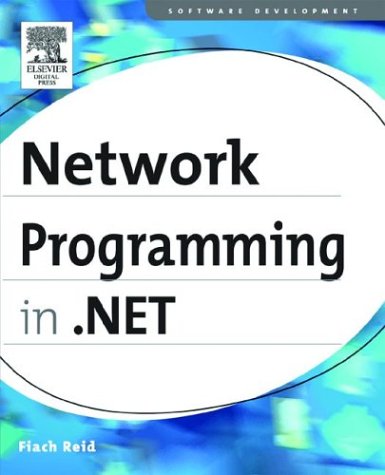
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionsCollectionEditor.cs
- OleServicesContext.cs
- Schema.cs
- MaskedTextProvider.cs
- WindowsComboBox.cs
- InheritanceContextHelper.cs
- StrongNameMembershipCondition.cs
- ObjectContextServiceProvider.cs
- UnknownWrapper.cs
- CacheDependency.cs
- QueryTask.cs
- MetadataSource.cs
- RuntimeWrappedException.cs
- ZoomPercentageConverter.cs
- listitem.cs
- MethodAccessException.cs
- SnapLine.cs
- EncodingStreamWrapper.cs
- IxmlLineInfo.cs
- SplitterDesigner.cs
- HttpModuleAction.cs
- StsCommunicationException.cs
- TriState.cs
- validationstate.cs
- TypeDescriptionProvider.cs
- RuntimeEnvironment.cs
- ExpressionBuilder.cs
- ELinqQueryState.cs
- ToolZone.cs
- DataFieldCollectionEditor.cs
- FamilyTypefaceCollection.cs
- CommandEventArgs.cs
- SpnEndpointIdentity.cs
- Constants.cs
- RegexReplacement.cs
- AutomationPatternInfo.cs
- MetafileHeader.cs
- EditorZoneBase.cs
- GAC.cs
- PenCursorManager.cs
- BitmapSizeOptions.cs
- SafeUserTokenHandle.cs
- ViewLoader.cs
- DeadLetterQueue.cs
- TraceHandlerErrorFormatter.cs
- TypefaceCollection.cs
- Ops.cs
- DataRelation.cs
- SchemeSettingElement.cs
- ActivityDesignerLayoutSerializers.cs
- initElementDictionary.cs
- PDBReader.cs
- AssertUtility.cs
- FixedSOMContainer.cs
- TimerEventSubscriptionCollection.cs
- AdornerLayer.cs
- Base64Stream.cs
- TypeLoader.cs
- ProviderUtil.cs
- CheckBox.cs
- TableAdapterManagerGenerator.cs
- XmlSiteMapProvider.cs
- ParameterBuilder.cs
- ViewValidator.cs
- ListParagraph.cs
- AnnouncementInnerClient11.cs
- CredentialCache.cs
- AsyncOperation.cs
- UnsafeNativeMethods.cs
- ObjectTag.cs
- InputProcessorProfilesLoader.cs
- EventDescriptor.cs
- Size.cs
- SplitterPanel.cs
- Pointer.cs
- ListBindableAttribute.cs
- X509CertificateCollection.cs
- EventInfo.cs
- PartialArray.cs
- UriExt.cs
- TextProperties.cs
- TransactionCache.cs
- DesignerWithHeader.cs
- NameValueSectionHandler.cs
- StructuredCompositeActivityDesigner.cs
- BrowserCapabilitiesCompiler.cs
- UnsafeMethods.cs
- ObjectDataSourceFilteringEventArgs.cs
- Environment.cs
- ApplicationId.cs
- MetadataItemCollectionFactory.cs
- SimpleHandlerFactory.cs
- SqlPersonalizationProvider.cs
- Component.cs
- RealProxy.cs
- NumberAction.cs
- KoreanLunisolarCalendar.cs
- ActivityValidator.cs
- mediaeventargs.cs
- UnsafeNativeMethods.cs