Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / PartialArray.cs / 1 / PartialArray.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: The PartialArray struct is used when the developer needs to pass a CLR array range to // a function that takes generic IList interface. For cases when the whole array needs to be passed, // CLR array already implements IList. // // // History: // 06/25/2004 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal { ////// The PartialArray struct is used when someone needs to pass a CLR array range to /// a function that takes generic IList interface. For cases when the whole array needs to be passed, /// CLR array already implements IList. /// internal struct PartialArray: IList { private T[] _array; private int _initialIndex; private int _count; public PartialArray(T[] array, int initialIndex, int count) { // make sure early that the caller didn't miscalculate index and count Debug.Assert(initialIndex >= 0 && initialIndex + count <= array.Length); _array = array; _initialIndex = initialIndex; _count = count; } /// /// Convenience helper for passing the whole array. /// /// public PartialArray(T[] array) : this(array, 0, array.Length) {} #region IListMembers public bool IsReadOnly { get { return false; } } public bool Contains(T item) { return IndexOf(item) >= 0; } public bool IsFixedSize { get { return true; } } public bool Remove(T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void RemoveAt(int index) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Clear() { throw new NotSupportedException(); } public void Add(T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Insert(int index, T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public T this[int index] { get { return _array[index + _initialIndex]; } set { _array[index + _initialIndex] = value; } } public int IndexOf(T item) { int index = Array.IndexOf (_array, item, _initialIndex, _count); if (index >= 0) { return index - _initialIndex; } else { return -1; } } #endregion #region ICollection Members public int Count { get { return _count; } } public void CopyTo(T[] array, int arrayIndex) { // parameter validations if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException( SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional), "array"); } if (arrayIndex < 0) { throw new ArgumentOutOfRangeException("arrayIndex"); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array"), "arrayIndex"); } if ((array.Length - Count - arrayIndex) < 0) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); } // do the copying here for (int i = 0; i < Count; i++) { array[arrayIndex + i] = this[i]; } } #endregion #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { for (int i = 0; i < Count; i++) { yield return this[i]; } } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: The PartialArray struct is used when the developer needs to pass a CLR array range to // a function that takes generic IList interface. For cases when the whole array needs to be passed, // CLR array already implements IList. // // // History: // 06/25/2004 : mleonov - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal { /// /// The PartialArray struct is used when someone needs to pass a CLR array range to /// a function that takes generic IList interface. For cases when the whole array needs to be passed, /// CLR array already implements IList. /// internal struct PartialArray: IList { private T[] _array; private int _initialIndex; private int _count; public PartialArray(T[] array, int initialIndex, int count) { // make sure early that the caller didn't miscalculate index and count Debug.Assert(initialIndex >= 0 && initialIndex + count <= array.Length); _array = array; _initialIndex = initialIndex; _count = count; } /// /// Convenience helper for passing the whole array. /// /// public PartialArray(T[] array) : this(array, 0, array.Length) {} #region IListMembers public bool IsReadOnly { get { return false; } } public bool Contains(T item) { return IndexOf(item) >= 0; } public bool IsFixedSize { get { return true; } } public bool Remove(T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void RemoveAt(int index) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Clear() { throw new NotSupportedException(); } public void Add(T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Insert(int index, T item) { throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public T this[int index] { get { return _array[index + _initialIndex]; } set { _array[index + _initialIndex] = value; } } public int IndexOf(T item) { int index = Array.IndexOf (_array, item, _initialIndex, _count); if (index >= 0) { return index - _initialIndex; } else { return -1; } } #endregion #region ICollection Members public int Count { get { return _count; } } public void CopyTo(T[] array, int arrayIndex) { // parameter validations if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException( SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional), "array"); } if (arrayIndex < 0) { throw new ArgumentOutOfRangeException("arrayIndex"); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array"), "arrayIndex"); } if ((array.Length - Count - arrayIndex) < 0) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); } // do the copying here for (int i = 0; i < Count; i++) { array[arrayIndex + i] = this[i]; } } #endregion #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { for (int i = 0; i < Count; i++) { yield return this[i]; } } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
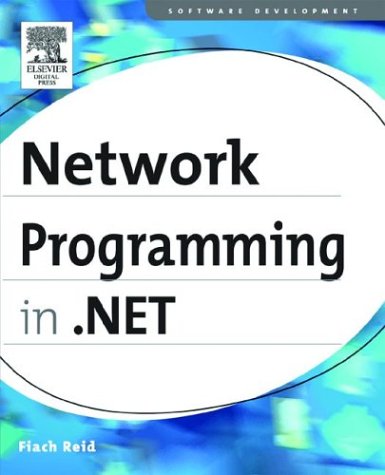
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NotSupportedException.cs
- SqlCacheDependencyDatabase.cs
- TemplateControl.cs
- FacetChecker.cs
- SoapSchemaImporter.cs
- AutomationPatternInfo.cs
- TreeWalkHelper.cs
- MetadataPropertyAttribute.cs
- URIFormatException.cs
- DeclaredTypeValidatorAttribute.cs
- RegexCharClass.cs
- SplashScreenNativeMethods.cs
- HtmlGenericControl.cs
- SmtpSection.cs
- PointHitTestResult.cs
- PathStreamGeometryContext.cs
- ListViewGroup.cs
- PreviewControlDesigner.cs
- HttpPostedFile.cs
- TextChangedEventArgs.cs
- LineServicesRun.cs
- PathGeometry.cs
- DependencyObjectProvider.cs
- LinkedList.cs
- TypeReference.cs
- ChannelDemuxer.cs
- ComPlusSynchronizationContext.cs
- DiffuseMaterial.cs
- TextFindEngine.cs
- XmlDictionaryString.cs
- ResizeGrip.cs
- DataGridViewComboBoxCell.cs
- ZipPackage.cs
- TemplateKeyConverter.cs
- RightsManagementErrorHandler.cs
- MissingMemberException.cs
- CompModSwitches.cs
- SelectorAutomationPeer.cs
- ListViewUpdatedEventArgs.cs
- UserNameSecurityTokenAuthenticator.cs
- DataGridViewCellLinkedList.cs
- TemplateBindingExtension.cs
- ResolveNameEventArgs.cs
- WhitespaceRuleReader.cs
- TypeUsageBuilder.cs
- StringReader.cs
- StringStorage.cs
- PropertyGeneratedEventArgs.cs
- CodeBlockBuilder.cs
- TemplateLookupAction.cs
- TabletDevice.cs
- RegistryPermission.cs
- MultiPropertyDescriptorGridEntry.cs
- PagePropertiesChangingEventArgs.cs
- SystemIPv6InterfaceProperties.cs
- Rect.cs
- SimpleHandlerFactory.cs
- Page.cs
- _SslSessionsCache.cs
- ConfigXmlWhitespace.cs
- IPipelineRuntime.cs
- ScriptReferenceBase.cs
- LabelDesigner.cs
- DataSourceIDConverter.cs
- _AutoWebProxyScriptWrapper.cs
- Point3DAnimationBase.cs
- CountdownEvent.cs
- MatrixConverter.cs
- XmlByteStreamWriter.cs
- StubHelpers.cs
- DataServiceHostWrapper.cs
- ConsoleTraceListener.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- COAUTHIDENTITY.cs
- SymmetricKeyWrap.cs
- DocumentViewerConstants.cs
- StorageModelBuildProvider.cs
- RequestResizeEvent.cs
- XamlToRtfParser.cs
- MaskedTextProvider.cs
- PersianCalendar.cs
- XmlILTrace.cs
- HttpProfileGroupBase.cs
- CustomAssemblyResolver.cs
- Viewport2DVisual3D.cs
- SupportsEventValidationAttribute.cs
- StyleCollection.cs
- ScriptReferenceBase.cs
- CellIdBoolean.cs
- XmlCountingReader.cs
- RuntimeArgumentHandle.cs
- SmiMetaDataProperty.cs
- SmiContext.cs
- SchemaCompiler.cs
- ListBase.cs
- GenericQueueSurrogate.cs
- UpdateManifestForBrowserApplication.cs
- StylusPointPropertyUnit.cs
- ApplicationDirectoryMembershipCondition.cs
- ContextInformation.cs