Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewCellLinkedList.cs / 1305376 / DataGridViewCellLinkedList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Windows.Forms; using System.ComponentModel; ////// /// internal class DataGridViewCellLinkedList : IEnumerable { private DataGridViewCellLinkedListElement lastAccessedElement; private DataGridViewCellLinkedListElement headElement; private int count, lastAccessedIndex; ///Represents a linked list of ///objects IEnumerator IEnumerable.GetEnumerator() { return new DataGridViewCellLinkedListEnumerator(this.headElement); } /// public DataGridViewCellLinkedList() { this.lastAccessedIndex = -1; } /// public DataGridViewCell this[int index] { get { Debug.Assert(index >= 0); Debug.Assert(index < this.count); if (this.lastAccessedIndex == -1 || index < this.lastAccessedIndex) { DataGridViewCellLinkedListElement tmp = this.headElement; int tmpIndex = index; while (tmpIndex > 0) { tmp = tmp.Next; tmpIndex--; } this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return tmp.DataGridViewCell; } else { while (this.lastAccessedIndex < index) { this.lastAccessedElement = this.lastAccessedElement.Next; this.lastAccessedIndex++; } return this.lastAccessedElement.DataGridViewCell; } } } /// public int Count { get { return this.count; } } /// public DataGridViewCell HeadCell { get { Debug.Assert(this.headElement != null); return this.headElement.DataGridViewCell; } } /// public void Add(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); Debug.Assert(dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.CellSelect || dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.ColumnHeaderSelect || dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.RowHeaderSelect); DataGridViewCellLinkedListElement newHead = new DataGridViewCellLinkedListElement(dataGridViewCell); if (this.headElement != null) { newHead.Next = this.headElement; } this.headElement = newHead; this.count++; this.lastAccessedElement = null; this.lastAccessedIndex = -1; } /// public void Clear() { this.lastAccessedElement = null; this.lastAccessedIndex = -1; this.headElement = null; this.count = 0; } /// public bool Contains(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); int index = 0; DataGridViewCellLinkedListElement tmp = this.headElement; while (tmp != null) { if (tmp.DataGridViewCell == dataGridViewCell) { this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return true; } tmp = tmp.Next; index++; } return false; } /// public bool Remove(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); DataGridViewCellLinkedListElement tmp1 = null, tmp2 = this.headElement; while (tmp2 != null) { if (tmp2.DataGridViewCell == dataGridViewCell) { break; } tmp1 = tmp2; tmp2 = tmp2.Next; } if (tmp2.DataGridViewCell == dataGridViewCell) { DataGridViewCellLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; return true; } return false; } /* Unused for now /// public DataGridViewCell RemoveHead() { if (this.headElement == null) { return null; } DataGridViewCellLinkedListElement tmp = this.headElement; this.headElement = tmp.Next; this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; return tmp.DataGridViewCell; } */ /// public int RemoveAllCellsAtBand(bool column, int bandIndex) { int removedCount = 0; DataGridViewCellLinkedListElement tmp1 = null, tmp2 = this.headElement; while (tmp2 != null) { if ((column && tmp2.DataGridViewCell.ColumnIndex == bandIndex) || (!column && tmp2.DataGridViewCell.RowIndex == bandIndex)) { DataGridViewCellLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } tmp2 = tmp3; this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; removedCount++; } else { tmp1 = tmp2; tmp2 = tmp2.Next; } } return removedCount; } } /// /// /// internal class DataGridViewCellLinkedListEnumerator : IEnumerator { private DataGridViewCellLinkedListElement headElement; private DataGridViewCellLinkedListElement current; private bool reset; ///Represents an emunerator of elements in a ///linked list. public DataGridViewCellLinkedListEnumerator(DataGridViewCellLinkedListElement headElement) { this.headElement = headElement; this.reset = true; } /// object IEnumerator.Current { get { Debug.Assert(this.current != null); // Since this is for internal use only. return this.current.DataGridViewCell; } } /// bool IEnumerator.MoveNext() { if (this.reset) { Debug.Assert(this.current == null); this.current = this.headElement; this.reset = false; } else { Debug.Assert(this.current != null); // Since this is for internal use only. this.current = this.current.Next; } return (this.current != null); } /// void IEnumerator.Reset() { this.reset = true; this.current = null; } } /// /// /// internal class DataGridViewCellLinkedListElement { private DataGridViewCell dataGridViewCell; private DataGridViewCellLinkedListElement next; ///Represents an element in a ///linked list. public DataGridViewCellLinkedListElement(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); this.dataGridViewCell = dataGridViewCell; } /// public DataGridViewCell DataGridViewCell { get { return this.dataGridViewCell; } } /// public DataGridViewCellLinkedListElement Next { get { return this.next; } set { this.next = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Windows.Forms; using System.ComponentModel; ////// /// internal class DataGridViewCellLinkedList : IEnumerable { private DataGridViewCellLinkedListElement lastAccessedElement; private DataGridViewCellLinkedListElement headElement; private int count, lastAccessedIndex; ///Represents a linked list of ///objects IEnumerator IEnumerable.GetEnumerator() { return new DataGridViewCellLinkedListEnumerator(this.headElement); } /// public DataGridViewCellLinkedList() { this.lastAccessedIndex = -1; } /// public DataGridViewCell this[int index] { get { Debug.Assert(index >= 0); Debug.Assert(index < this.count); if (this.lastAccessedIndex == -1 || index < this.lastAccessedIndex) { DataGridViewCellLinkedListElement tmp = this.headElement; int tmpIndex = index; while (tmpIndex > 0) { tmp = tmp.Next; tmpIndex--; } this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return tmp.DataGridViewCell; } else { while (this.lastAccessedIndex < index) { this.lastAccessedElement = this.lastAccessedElement.Next; this.lastAccessedIndex++; } return this.lastAccessedElement.DataGridViewCell; } } } /// public int Count { get { return this.count; } } /// public DataGridViewCell HeadCell { get { Debug.Assert(this.headElement != null); return this.headElement.DataGridViewCell; } } /// public void Add(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); Debug.Assert(dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.CellSelect || dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.ColumnHeaderSelect || dataGridViewCell.DataGridView.SelectionMode == DataGridViewSelectionMode.RowHeaderSelect); DataGridViewCellLinkedListElement newHead = new DataGridViewCellLinkedListElement(dataGridViewCell); if (this.headElement != null) { newHead.Next = this.headElement; } this.headElement = newHead; this.count++; this.lastAccessedElement = null; this.lastAccessedIndex = -1; } /// public void Clear() { this.lastAccessedElement = null; this.lastAccessedIndex = -1; this.headElement = null; this.count = 0; } /// public bool Contains(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); int index = 0; DataGridViewCellLinkedListElement tmp = this.headElement; while (tmp != null) { if (tmp.DataGridViewCell == dataGridViewCell) { this.lastAccessedElement = tmp; this.lastAccessedIndex = index; return true; } tmp = tmp.Next; index++; } return false; } /// public bool Remove(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); DataGridViewCellLinkedListElement tmp1 = null, tmp2 = this.headElement; while (tmp2 != null) { if (tmp2.DataGridViewCell == dataGridViewCell) { break; } tmp1 = tmp2; tmp2 = tmp2.Next; } if (tmp2.DataGridViewCell == dataGridViewCell) { DataGridViewCellLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; return true; } return false; } /* Unused for now /// public DataGridViewCell RemoveHead() { if (this.headElement == null) { return null; } DataGridViewCellLinkedListElement tmp = this.headElement; this.headElement = tmp.Next; this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; return tmp.DataGridViewCell; } */ /// public int RemoveAllCellsAtBand(bool column, int bandIndex) { int removedCount = 0; DataGridViewCellLinkedListElement tmp1 = null, tmp2 = this.headElement; while (tmp2 != null) { if ((column && tmp2.DataGridViewCell.ColumnIndex == bandIndex) || (!column && tmp2.DataGridViewCell.RowIndex == bandIndex)) { DataGridViewCellLinkedListElement tmp3 = tmp2.Next; if (tmp1 == null) { this.headElement = tmp3; } else { tmp1.Next = tmp3; } tmp2 = tmp3; this.count--; this.lastAccessedElement = null; this.lastAccessedIndex = -1; removedCount++; } else { tmp1 = tmp2; tmp2 = tmp2.Next; } } return removedCount; } } /// /// /// internal class DataGridViewCellLinkedListEnumerator : IEnumerator { private DataGridViewCellLinkedListElement headElement; private DataGridViewCellLinkedListElement current; private bool reset; ///Represents an emunerator of elements in a ///linked list. public DataGridViewCellLinkedListEnumerator(DataGridViewCellLinkedListElement headElement) { this.headElement = headElement; this.reset = true; } /// object IEnumerator.Current { get { Debug.Assert(this.current != null); // Since this is for internal use only. return this.current.DataGridViewCell; } } /// bool IEnumerator.MoveNext() { if (this.reset) { Debug.Assert(this.current == null); this.current = this.headElement; this.reset = false; } else { Debug.Assert(this.current != null); // Since this is for internal use only. this.current = this.current.Next; } return (this.current != null); } /// void IEnumerator.Reset() { this.reset = true; this.current = null; } } /// /// /// internal class DataGridViewCellLinkedListElement { private DataGridViewCell dataGridViewCell; private DataGridViewCellLinkedListElement next; ///Represents an element in a ///linked list. public DataGridViewCellLinkedListElement(DataGridViewCell dataGridViewCell) { Debug.Assert(dataGridViewCell != null); this.dataGridViewCell = dataGridViewCell; } /// public DataGridViewCell DataGridViewCell { get { return this.dataGridViewCell; } } /// public DataGridViewCellLinkedListElement Next { get { return this.next; } set { this.next = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
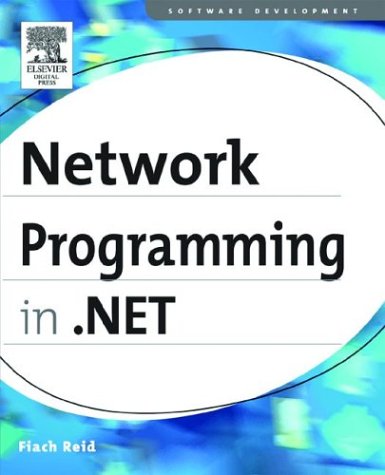
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymbolDocumentInfo.cs
- MaterialGroup.cs
- RealProxy.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- ResourcePart.cs
- LineProperties.cs
- SemanticAnalyzer.cs
- SetStoryboardSpeedRatio.cs
- ListViewGroupConverter.cs
- SmtpSection.cs
- CacheChildrenQuery.cs
- UTF7Encoding.cs
- ExceptionWrapper.cs
- AutomationTextAttribute.cs
- SqlPersistenceProviderFactory.cs
- SwitchLevelAttribute.cs
- ReflectionHelper.cs
- ConnectionManagementElementCollection.cs
- documentsequencetextcontainer.cs
- WorkflowTraceTransfer.cs
- ADMembershipUser.cs
- SelfIssuedSamlTokenFactory.cs
- figurelength.cs
- WithStatement.cs
- TypeHelper.cs
- FrameworkElementAutomationPeer.cs
- DefaultEventAttribute.cs
- AffineTransform3D.cs
- Random.cs
- UnsafeNativeMethodsTablet.cs
- Merger.cs
- ColorBlend.cs
- ValidationService.cs
- TextServicesCompartmentEventSink.cs
- CollectionViewGroup.cs
- Win32.cs
- ErrorHandler.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- Random.cs
- ZipIOBlockManager.cs
- BufferedGraphicsContext.cs
- ArrayTypeMismatchException.cs
- RtfControlWordInfo.cs
- PropertyTabChangedEvent.cs
- CommandLineParser.cs
- XmlDesignerDataSourceView.cs
- PtsContext.cs
- MobileFormsAuthentication.cs
- NoneExcludedImageIndexConverter.cs
- altserialization.cs
- AnonymousIdentificationModule.cs
- DeploymentSectionCache.cs
- IHttpResponseInternal.cs
- ToolBarButton.cs
- COM2Properties.cs
- CodePropertyReferenceExpression.cs
- CollectionChangedEventManager.cs
- DataGridViewCellCancelEventArgs.cs
- ObfuscateAssemblyAttribute.cs
- SR.cs
- ObjectTypeMapping.cs
- EncodingNLS.cs
- PageAction.cs
- CombinedGeometry.cs
- Cell.cs
- BroadcastEventHelper.cs
- BounceEase.cs
- ForwardPositionQuery.cs
- Size3DValueSerializer.cs
- ListViewInsertedEventArgs.cs
- NamespaceCollection.cs
- StyleXamlParser.cs
- WorkflowEnvironment.cs
- RuleInfoComparer.cs
- TransformedBitmap.cs
- XmlNamespaceManager.cs
- AspCompat.cs
- AddInSegmentDirectoryNotFoundException.cs
- SuppressMergeCheckAttribute.cs
- MenuItemStyleCollection.cs
- Single.cs
- DesignerForm.cs
- XmlParser.cs
- ParameterBinding.cs
- FileIOPermission.cs
- documentation.cs
- DataGridColumn.cs
- DesignerRegionMouseEventArgs.cs
- QueryConverter.cs
- PolyLineSegment.cs
- CultureMapper.cs
- CodeComment.cs
- HitTestParameters3D.cs
- Rights.cs
- AddInBase.cs
- PeerApplication.cs
- _ChunkParse.cs
- ComPlusSynchronizationContext.cs
- Table.cs
- TextEndOfParagraph.cs