Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / figurelength.cs / 1305600 / figurelength.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Figure length implementation // // // History: // 06/23/2005 : ghermann - Created - Adapted from GridLength // //--------------------------------------------------------------------------- using MS.Internal; using System.ComponentModel; using System.Globalization; using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows { ////// FigureUnitType enum is used to indicate what kind of value the /// FigureLength is holding. /// public enum FigureUnitType { ////// The value indicates that content should be calculated without constraints. /// Auto = 0, ////// The value is expressed as a pixel. /// Pixel, ////// The value is expressed as fraction of column width. /// Column, ////// The value is expressed as a fraction of content width. /// Content, ////// The value is expressed as a fraction of page width. /// Page, } ////// FigureLength is the type used for height and width on figure element /// [TypeConverter(typeof(FigureLengthConverter))] public struct FigureLength : IEquatable{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Constructor, initializes the FigureLength as absolute value in pixels. /// /// Specifies the number of 'device-independent pixels' /// (96 pixels-per-inch). ////// If public FigureLength(double pixels) : this(pixels, FigureUnitType.Pixel) { } ///pixels parameter isdouble.NaN /// orpixels parameter isdouble.NegativeInfinity /// orpixels parameter isdouble.PositiveInfinity . /// orvalue parameter isnegative . ////// Constructor, initializes the FigureLength and specifies what kind of value /// it will hold. /// /// Value to be stored by this FigureLength /// instance. /// Type of the value to be stored by this FigureLength /// instance. ////// If the ///type parameter isFigureUnitType.Auto , /// then passed in value is ignored and replaced with0 . ////// If public FigureLength(double value, FigureUnitType type) { double maxColumns = PTS.Restrictions.tscColumnRestriction; double maxPixel = Math.Min(1000000, PTS.MaxPageSize); if (DoubleUtil.IsNaN(value)) { throw new ArgumentException(SR.Get(SRID.InvalidCtorParameterNoNaN, "value")); } if (double.IsInfinity(value)) { throw new ArgumentException(SR.Get(SRID.InvalidCtorParameterNoInfinity, "value")); } if (value < 0.0) { throw new ArgumentOutOfRangeException(SR.Get(SRID.InvalidCtorParameterNoNegative, "value")); } if ( type != FigureUnitType.Auto && type != FigureUnitType.Pixel && type != FigureUnitType.Column && type != FigureUnitType.Content && type != FigureUnitType.Page ) { throw new ArgumentException(SR.Get(SRID.InvalidCtorParameterUnknownFigureUnitType, "type")); } if(value > 1.0 && (type == FigureUnitType.Content || type == FigureUnitType.Page)) { throw new ArgumentOutOfRangeException("value"); } if (value > maxColumns && type == FigureUnitType.Column) { throw new ArgumentOutOfRangeException("value"); } if (value > maxPixel && type == FigureUnitType.Pixel) { throw new ArgumentOutOfRangeException("value"); } _unitValue = (type == FigureUnitType.Auto) ? 0.0 : value; _unitType = type; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ///value parameter isdouble.NaN /// orvalue parameter isdouble.NegativeInfinity /// orvalue parameter isdouble.PositiveInfinity . /// orvalue parameter isnegative . ////// Overloaded operator, compares 2 FigureLengths. /// /// first FigureLength to compare. /// second FigureLength to compare. ///true if specified FigureLengths have same value /// and unit type. public static bool operator == (FigureLength fl1, FigureLength fl2) { return ( fl1.FigureUnitType == fl2.FigureUnitType && fl1.Value == fl2.Value ); } ////// Overloaded operator, compares 2 FigureLengths. /// /// first FigureLength to compare. /// second FigureLength to compare. ///true if specified FigureLengths have either different value or /// unit type. public static bool operator != (FigureLength fl1, FigureLength fl2) { return ( fl1.FigureUnitType != fl2.FigureUnitType || fl1.Value != fl2.Value ); } ////// Compares this instance of FigureLength with another object. /// /// Reference to an object for comparison. ///override public bool Equals(object oCompare) { if(oCompare is FigureLength) { FigureLength l = (FigureLength)oCompare; return (this == l); } else return false; } /// true if this FigureLength instance has the same value /// and unit type as oCompare./// Compares this instance of FigureLength with another object. /// /// FigureLength to compare. ///public bool Equals(FigureLength figureLength) { return (this == figureLength); } /// true if this FigureLength instance has the same value /// and unit type as figureLength./// ////// public override int GetHashCode() { return ((int)_unitValue + (int)_unitType); } /// /// Returns public bool IsAbsolute { get { return (_unitType == FigureUnitType.Pixel); } } ///true if this FigureLength instance holds /// an absolute (pixel) value. ////// Returns public bool IsAuto { get { return (_unitType == FigureUnitType.Auto); } } ///true if this FigureLength instance is /// automatic (not specified). ////// Returns public bool IsColumn { get { return (_unitType == FigureUnitType.Column); } } ///true if this FigureLength instance is column relative. ////// Returns public bool IsContent { get { return (_unitType == FigureUnitType.Content); } } ///true if this FigureLength instance is content relative. ////// Returns public bool IsPage { get { return (_unitType == FigureUnitType.Page); } } ///true if this FigureLength instance is page relative. ////// Returns value part of this FigureLength instance. /// public double Value { get { return ((_unitType == FigureUnitType.Auto) ? 1.0 : _unitValue); } } ////// Returns unit type of this FigureLength instance. /// public FigureUnitType FigureUnitType { get { return (_unitType); } } ////// Returns the string representation of this object. /// public override string ToString() { return FigureLengthConverter.ToString(this, CultureInfo.InvariantCulture); } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private double _unitValue; // unit value storage private FigureUnitType _unitType; // unit type storage #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
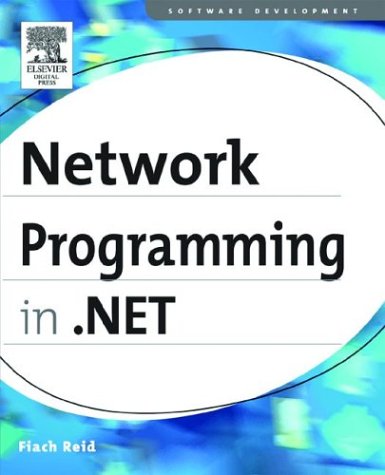
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServerReliableChannelBinder.cs
- HttpRequest.cs
- Message.cs
- HostingEnvironment.cs
- CompiledIdentityConstraint.cs
- PropertyRecord.cs
- SqlException.cs
- SystemThemeKey.cs
- ParameterCollection.cs
- PrimitiveXmlSerializers.cs
- ScaleTransform.cs
- Assert.cs
- TimelineCollection.cs
- GridViewDeletedEventArgs.cs
- InstancePersistence.cs
- FtpWebRequest.cs
- ValueOfAction.cs
- LockedAssemblyCache.cs
- XPathEmptyIterator.cs
- AccessViolationException.cs
- SystemIPInterfaceProperties.cs
- CustomErrorsSection.cs
- SelectionItemPattern.cs
- ConfigXmlElement.cs
- DependencyStoreSurrogate.cs
- CounterSetInstance.cs
- TrackingMemoryStreamFactory.cs
- WorkflowApplicationEventArgs.cs
- CommandConverter.cs
- BitmapCodecInfoInternal.cs
- VectorCollection.cs
- CodeTypeDeclaration.cs
- ZoneButton.cs
- StrokeNode.cs
- ToolboxComponentsCreatedEventArgs.cs
- InstancePersistenceEvent.cs
- UnsafePeerToPeerMethods.cs
- RegexCapture.cs
- SslStream.cs
- FixedPageAutomationPeer.cs
- DesignerVerbToolStripMenuItem.cs
- ComponentCommands.cs
- CryptoApi.cs
- CellParagraph.cs
- MenuEventArgs.cs
- LinqTreeNodeEvaluator.cs
- TimelineClockCollection.cs
- Ray3DHitTestResult.cs
- StylusButtonEventArgs.cs
- ZoneIdentityPermission.cs
- EventSourceCreationData.cs
- TrackingMemoryStreamFactory.cs
- DbDataAdapter.cs
- ExpressionBuilderCollection.cs
- PersonalizablePropertyEntry.cs
- DetailsViewInsertEventArgs.cs
- SymbolType.cs
- WorkflowWebHostingModule.cs
- ProxyDataContractResolver.cs
- ISFTagAndGuidCache.cs
- SingleAnimationUsingKeyFrames.cs
- Typography.cs
- TimeSpan.cs
- WebPartTracker.cs
- UInt64Converter.cs
- XhtmlBasicTextBoxAdapter.cs
- ValidationSummaryDesigner.cs
- XmlName.cs
- SymLanguageVendor.cs
- SessionPageStatePersister.cs
- PathSegment.cs
- TokenBasedSetEnumerator.cs
- ButtonPopupAdapter.cs
- RefreshEventArgs.cs
- ICollection.cs
- TagMapCollection.cs
- Query.cs
- PeerSecurityHelpers.cs
- XamlDesignerSerializationManager.cs
- ManipulationStartingEventArgs.cs
- Slider.cs
- EntityDataSourceViewSchema.cs
- StyleHelper.cs
- Group.cs
- DecoderReplacementFallback.cs
- PeerIPHelper.cs
- BlockCollection.cs
- XmlAttributeProperties.cs
- CreatingCookieEventArgs.cs
- ApplicationDirectoryMembershipCondition.cs
- CompoundFileIOPermission.cs
- GraphicsPathIterator.cs
- EncryptedXml.cs
- Point.cs
- EntityTypeEmitter.cs
- LocalizationCodeDomSerializer.cs
- SQLByteStorage.cs
- mediaclock.cs
- RelationHandler.cs
- TripleDES.cs