Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / StreamSecurityUpgradeAcceptorBase.cs / 1 / StreamSecurityUpgradeAcceptorBase.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.IO; using System.ServiceModel.Security; abstract class StreamSecurityUpgradeAcceptorBase : StreamSecurityUpgradeAcceptor { SecurityMessageProperty remoteSecurity; bool securityUpgraded; string upgradeString; protected StreamSecurityUpgradeAcceptorBase(string upgradeString) { this.upgradeString = upgradeString; } public override Stream AcceptUpgrade(Stream stream) { if (stream == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("stream"); } Stream result = this.OnAcceptUpgrade(stream, out this.remoteSecurity); this.securityUpgraded = true; return result; } public override IAsyncResult BeginAcceptUpgrade(Stream stream, AsyncCallback callback, object state) { if (stream == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("stream"); } return this.OnBeginAcceptUpgrade(stream, callback, state); } public override bool CanUpgrade(string contentType) { if (this.securityUpgraded) { return false; } return (contentType == this.upgradeString); } public override Stream EndAcceptUpgrade(IAsyncResult result) { if (result == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("result"); } Stream retValue = this.OnEndAcceptUpgrade(result, out this.remoteSecurity); this.securityUpgraded = true; return retValue; } public override SecurityMessageProperty GetRemoteSecurity() { // this could be null if upgrade not completed. return this.remoteSecurity; } protected abstract Stream OnAcceptUpgrade(Stream stream, out SecurityMessageProperty remoteSecurity); protected abstract IAsyncResult OnBeginAcceptUpgrade(Stream stream, AsyncCallback callback, object state); protected abstract Stream OnEndAcceptUpgrade(IAsyncResult result, out SecurityMessageProperty remoteSecurity); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
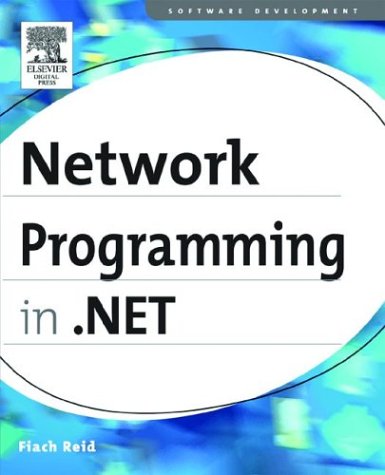
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlCacheDependencyDatabaseCollection.cs
- EncoderExceptionFallback.cs
- HeaderFilter.cs
- OleDbConnectionFactory.cs
- CompilationSection.cs
- TargetParameterCountException.cs
- LocalizationComments.cs
- XslNumber.cs
- ModelFunctionTypeElement.cs
- ZipIOLocalFileHeader.cs
- LoginAutoFormat.cs
- DataViewSettingCollection.cs
- StrongNameIdentityPermission.cs
- ListBox.cs
- StyleHelper.cs
- PageThemeCodeDomTreeGenerator.cs
- DataTableReaderListener.cs
- HebrewCalendar.cs
- WebBrowser.cs
- PropertyChangedEventArgs.cs
- BStrWrapper.cs
- XPathException.cs
- IfAction.cs
- ReadOnlyCollection.cs
- RegexGroupCollection.cs
- PkcsUtils.cs
- OracleLob.cs
- EventEntry.cs
- CompensationExtension.cs
- Internal.cs
- JumpPath.cs
- MDIClient.cs
- RequestTimeoutManager.cs
- WebBrowserContainer.cs
- AppDomainManager.cs
- FontStyle.cs
- DataSourceProvider.cs
- GZipStream.cs
- VoiceSynthesis.cs
- SqlParameterCollection.cs
- DeflateStream.cs
- CheckBoxBaseAdapter.cs
- ContainerFilterService.cs
- RawStylusInputCustomDataList.cs
- DataGridClipboardCellContent.cs
- Window.cs
- Parameter.cs
- AttributeEmitter.cs
- CssStyleCollection.cs
- SafeRightsManagementSessionHandle.cs
- DefaultMemberAttribute.cs
- DecimalConstantAttribute.cs
- ValidationManager.cs
- ArrayWithOffset.cs
- TimerTable.cs
- SiteMapNodeItem.cs
- MutexSecurity.cs
- HierarchicalDataBoundControl.cs
- HtmlTitle.cs
- ExtendedPropertyCollection.cs
- XmlDocumentSerializer.cs
- TextRangeEditLists.cs
- BypassElementCollection.cs
- WorkflowCommandExtensionItem.cs
- GB18030Encoding.cs
- HtmlInputImage.cs
- SerialReceived.cs
- CompressionTransform.cs
- OneOf.cs
- GridEntryCollection.cs
- SizeFConverter.cs
- DesignerCommandAdapter.cs
- RelationshipManager.cs
- _AutoWebProxyScriptWrapper.cs
- safelinkcollection.cs
- EntityAdapter.cs
- DrawingGroup.cs
- AsynchronousChannelMergeEnumerator.cs
- XPathNavigatorReader.cs
- Point3DConverter.cs
- EventNotify.cs
- PerfCounters.cs
- BitmapEffectInputData.cs
- ConditionalBranch.cs
- FrameworkElement.cs
- DataControlReferenceCollection.cs
- FixedHighlight.cs
- TextRangeEditLists.cs
- LifetimeServices.cs
- EdmFunction.cs
- DesignerDataColumn.cs
- ValuePatternIdentifiers.cs
- VisualStyleTypesAndProperties.cs
- SchemaImporterExtensionElement.cs
- WindowsIPAddress.cs
- TitleStyle.cs
- XsltInput.cs
- DeploymentExceptionMapper.cs
- HtmlTextArea.cs
- XamlToRtfParser.cs