Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Sys / System / Configuration / NameValueSectionHandler.cs / 1 / NameValueSectionHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Xml; using System.Globalization; ////// Simple dictionary config factory /// public class NameValueSectionHandler : IConfigurationSectionHandler { const string defaultKeyAttribute = "key"; const string defaultValueAttribute = "value"; ////// public object Create(object parent, object context, XmlNode section) { return CreateStatic(parent, section, KeyAttributeName, ValueAttributeName); } internal static object CreateStatic(object parent, XmlNode section) { return CreateStatic(parent, section, defaultKeyAttribute, defaultValueAttribute); } internal static object CreateStatic(object parent, XmlNode section, string keyAttriuteName, string valueAttributeName) { ReadOnlyNameValueCollection result; // start result off as a shallow clone of the parent if (parent == null) result = new ReadOnlyNameValueCollection(StringComparer.OrdinalIgnoreCase); else { ReadOnlyNameValueCollection parentCollection = (ReadOnlyNameValueCollection)parent; result = new ReadOnlyNameValueCollection(parentCollection); } // process XML HandlerBase.CheckForUnrecognizedAttributes(section); foreach (XmlNode child in section.ChildNodes) { // skip whitespace and comments if (HandlerBase.IsIgnorableAlsoCheckForNonElement(child)) continue; // handle[To be supplied.] ///, , tags if (child.Name == "add") { String key = HandlerBase.RemoveRequiredAttribute(child, keyAttriuteName); String value = HandlerBase.RemoveRequiredAttribute(child, valueAttributeName, true/*allowEmptyString*/); HandlerBase.CheckForUnrecognizedAttributes(child); result[key] = value; } else if (child.Name == "remove") { String key = HandlerBase.RemoveRequiredAttribute(child, keyAttriuteName); HandlerBase.CheckForUnrecognizedAttributes(child); result.Remove(key); } else if (child.Name.Equals("clear")) { HandlerBase.CheckForUnrecognizedAttributes(child); result.Clear(); } else { HandlerBase.ThrowUnrecognizedElement(child); } } result.SetReadOnly(); return result; } /// /// protected virtual string KeyAttributeName { get { return defaultKeyAttribute;} } ///[To be supplied.] ////// protected virtual string ValueAttributeName { get { return defaultValueAttribute;} } } }[To be supplied.] ///
Link Menu
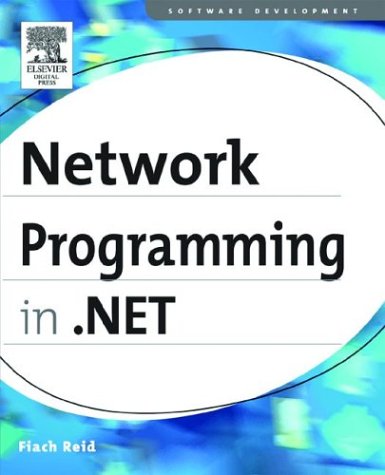
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataFile.cs
- SQLMembershipProvider.cs
- Vector3DIndependentAnimationStorage.cs
- ErrorHandler.cs
- AsyncOperation.cs
- UnaryNode.cs
- FigureParaClient.cs
- KeyValuePair.cs
- LicenseException.cs
- ToolstripProfessionalRenderer.cs
- translator.cs
- BitmapEffectInputConnector.cs
- EventDescriptor.cs
- TableMethodGenerator.cs
- HtmlInputSubmit.cs
- FileReservationCollection.cs
- ListView.cs
- OperationResponse.cs
- TextRangeProviderWrapper.cs
- Crc32.cs
- Profiler.cs
- FontDriver.cs
- DataGridViewTextBoxColumn.cs
- CustomDictionarySources.cs
- TextHintingModeValidation.cs
- XmlQueryContext.cs
- AutomationProperty.cs
- WizardStepBase.cs
- HttpCachePolicy.cs
- Span.cs
- HebrewNumber.cs
- SamlAuthenticationStatement.cs
- AddInPipelineAttributes.cs
- ExecutionContext.cs
- DBCommand.cs
- AnnotationComponentChooser.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- BatchParser.cs
- HtmlTextArea.cs
- HandleTable.cs
- TdsParserStateObject.cs
- XsltLibrary.cs
- WebBrowsableAttribute.cs
- LeftCellWrapper.cs
- DodSequenceMerge.cs
- StylusTip.cs
- ToolBarButton.cs
- _HeaderInfoTable.cs
- NodeInfo.cs
- TextServicesHost.cs
- ClientBuildManager.cs
- WorkflowRequestContext.cs
- ServiceNotStartedException.cs
- SafeThemeHandle.cs
- RSACryptoServiceProvider.cs
- WorkflowElementDialog.cs
- InstanceNotReadyException.cs
- StrokeNode.cs
- DependencyPropertyKey.cs
- CaseStatementProjectedSlot.cs
- DesignerView.Commands.cs
- Psha1DerivedKeyGeneratorHelper.cs
- ToolboxComponentsCreatedEventArgs.cs
- WebPartConnectionsEventArgs.cs
- ErasingStroke.cs
- KeyGestureConverter.cs
- SerializationTrace.cs
- HostingPreferredMapPath.cs
- SelectingProviderEventArgs.cs
- ValidatedMobileControlConverter.cs
- MenuScrollingVisibilityConverter.cs
- XmlSchemaCompilationSettings.cs
- ZipIORawDataFileBlock.cs
- InfoCardProofToken.cs
- DoWorkEventArgs.cs
- HttpInputStream.cs
- OneOfConst.cs
- CqlErrorHelper.cs
- WorkflowMarkupSerializationException.cs
- DecoderNLS.cs
- DiscoveryDocumentLinksPattern.cs
- ConfigurationManagerHelper.cs
- HttpHandlersSection.cs
- UnicodeEncoding.cs
- XhtmlCssHandler.cs
- ImageBrush.cs
- WebPartUtil.cs
- QilTernary.cs
- EdmSchemaAttribute.cs
- EventHandlersDesigner.cs
- HttpPostedFileWrapper.cs
- Calendar.cs
- InternalConfigEventArgs.cs
- ShapingWorkspace.cs
- SQLDecimalStorage.cs
- TargetFrameworkAttribute.cs
- GridViewColumnCollectionChangedEventArgs.cs
- FastEncoderWindow.cs
- SystemResources.cs
- DocumentViewerConstants.cs