Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / CompiledAction.cs / 1305376 / CompiledAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal abstract class CompiledAction : Action { internal abstract void Compile(Compiler compiler); internal virtual bool CompileAttribute(Compiler compiler) { return false; } public void CompileAttributes(Compiler compiler) { NavigatorInput input = compiler.Input; string element = input.LocalName; if (input.MoveToFirstAttribute()) { do { if (input.NamespaceURI.Length != 0) continue; try { if (CompileAttribute(compiler) == false) { throw XsltException.Create(Res.Xslt_InvalidAttribute, input.LocalName, element); } }catch { if (! compiler.ForwardCompatibility) { throw; } else { // In ForwardCompatibility mode we ignoreing all unknown or incorrect attributes // If it's mandatory attribute we'l notice it absents later. } } } while (input.MoveToNextAttribute()); input.ToParent(); } } // For perf reason we precalculating AVTs at compile time. // If we can do this we set original AVT to null internal static string PrecalculateAvt(ref Avt avt) { string result = null; if(avt != null && avt.IsConstant) { result = avt.Evaluate(null, null); avt = null; } return result; } public void CheckEmpty(Compiler compiler) { // Really EMPTY means no content at all, but the sake of compatibility with MSXML we allow whitespaces string elementName = compiler.Input.Name; if (compiler.Recurse()) { do { // Note: will be reported as XPathNodeType.Text XPathNodeType nodeType = compiler.Input.NodeType; if ( nodeType != XPathNodeType.Whitespace && nodeType != XPathNodeType.Comment && nodeType != XPathNodeType.ProcessingInstruction ) { throw XsltException.Create(Res.Xslt_NotEmptyContents, elementName); } } while (compiler.Advance()); compiler.ToParent(); } } public void CheckRequiredAttribute(Compiler compiler, object attrValue, string attrName) { CheckRequiredAttribute(compiler, attrValue != null, attrName); } public void CheckRequiredAttribute(Compiler compiler, bool attr, string attrName) { if (! attr) { throw XsltException.Create(Res.Xslt_MissingAttribute, attrName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal abstract class CompiledAction : Action { internal abstract void Compile(Compiler compiler); internal virtual bool CompileAttribute(Compiler compiler) { return false; } public void CompileAttributes(Compiler compiler) { NavigatorInput input = compiler.Input; string element = input.LocalName; if (input.MoveToFirstAttribute()) { do { if (input.NamespaceURI.Length != 0) continue; try { if (CompileAttribute(compiler) == false) { throw XsltException.Create(Res.Xslt_InvalidAttribute, input.LocalName, element); } }catch { if (! compiler.ForwardCompatibility) { throw; } else { // In ForwardCompatibility mode we ignoreing all unknown or incorrect attributes // If it's mandatory attribute we'l notice it absents later. } } } while (input.MoveToNextAttribute()); input.ToParent(); } } // For perf reason we precalculating AVTs at compile time. // If we can do this we set original AVT to null internal static string PrecalculateAvt(ref Avt avt) { string result = null; if(avt != null && avt.IsConstant) { result = avt.Evaluate(null, null); avt = null; } return result; } public void CheckEmpty(Compiler compiler) { // Really EMPTY means no content at all, but the sake of compatibility with MSXML we allow whitespaces string elementName = compiler.Input.Name; if (compiler.Recurse()) { do { // Note: will be reported as XPathNodeType.Text XPathNodeType nodeType = compiler.Input.NodeType; if ( nodeType != XPathNodeType.Whitespace && nodeType != XPathNodeType.Comment && nodeType != XPathNodeType.ProcessingInstruction ) { throw XsltException.Create(Res.Xslt_NotEmptyContents, elementName); } } while (compiler.Advance()); compiler.ToParent(); } } public void CheckRequiredAttribute(Compiler compiler, object attrValue, string attrName) { CheckRequiredAttribute(compiler, attrValue != null, attrName); } public void CheckRequiredAttribute(Compiler compiler, bool attr, string attrName) { if (! attr) { throw XsltException.Create(Res.Xslt_MissingAttribute, attrName); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
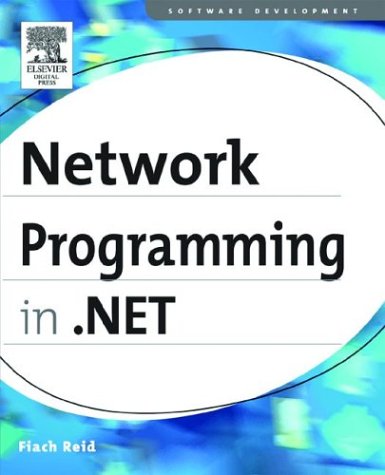
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SatelliteContractVersionAttribute.cs
- WindowCollection.cs
- EnvelopeVersion.cs
- ProcessHostConfigUtils.cs
- Sql8ExpressionRewriter.cs
- XPathSingletonIterator.cs
- CommonObjectSecurity.cs
- CallInfo.cs
- UIAgentAsyncEndRequest.cs
- ComponentConverter.cs
- SystemIPInterfaceStatistics.cs
- SimpleExpression.cs
- HttpStreamFormatter.cs
- DBDataPermissionAttribute.cs
- TreeView.cs
- CheckBoxField.cs
- EdmConstants.cs
- PreservationFileWriter.cs
- TypeRefElement.cs
- Screen.cs
- ResolveResponseInfo.cs
- OracleDataReader.cs
- TypeInitializationException.cs
- DirectoryInfo.cs
- DataFormats.cs
- ResourceFallbackManager.cs
- InputLangChangeRequestEvent.cs
- HashHelper.cs
- CapacityStreamGeometryContext.cs
- dataprotectionpermission.cs
- FrameworkElementFactoryMarkupObject.cs
- WindowsSspiNegotiation.cs
- ConfigurationElement.cs
- EntityProviderServices.cs
- NamedPipeTransportManager.cs
- StorageScalarPropertyMapping.cs
- EditCommandColumn.cs
- baseaxisquery.cs
- InternalsVisibleToAttribute.cs
- DataTableNewRowEvent.cs
- PageEventArgs.cs
- WindowsAuthenticationModule.cs
- ConfigurationLocation.cs
- RegistryPermission.cs
- HtmlInputText.cs
- ColorPalette.cs
- NullableBoolConverter.cs
- MediaCommands.cs
- externdll.cs
- DataRowView.cs
- EdmToObjectNamespaceMap.cs
- DBParameter.cs
- SqlDependencyListener.cs
- TextRange.cs
- HttpRequestWrapper.cs
- LineInfo.cs
- XPathExpr.cs
- EntitySqlQueryBuilder.cs
- DataPagerFieldCollection.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- WindowsScrollBarBits.cs
- XsltArgumentList.cs
- DesignerActionItemCollection.cs
- UpDownBase.cs
- TemplatePagerField.cs
- PermissionAttributes.cs
- ObjRef.cs
- BrowserCapabilitiesCodeGenerator.cs
- SuppressIldasmAttribute.cs
- KeyboardDevice.cs
- CatchDesigner.xaml.cs
- DataObject.cs
- CultureTable.cs
- DataGridRowClipboardEventArgs.cs
- ISFClipboardData.cs
- recordstatescratchpad.cs
- Ops.cs
- FacetValues.cs
- UnauthorizedAccessException.cs
- XmlnsDefinitionAttribute.cs
- PostBackOptions.cs
- DoubleKeyFrameCollection.cs
- QuaternionRotation3D.cs
- DocumentEventArgs.cs
- Configuration.cs
- GridItemCollection.cs
- CommandField.cs
- FileLevelControlBuilderAttribute.cs
- SpellerHighlightLayer.cs
- ExtensionWindowHeader.cs
- SqlDataSourceQuery.cs
- NonBatchDirectoryCompiler.cs
- StoryFragments.cs
- ISFTagAndGuidCache.cs
- CodeAttributeArgumentCollection.cs
- ReverseQueryOperator.cs
- XmlSchemaSimpleTypeList.cs
- SqlMethodAttribute.cs
- BindStream.cs
- InvokeWebService.cs