Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / BufferedGraphics.cs / 1 / BufferedGraphics.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System; using System.ComponentModel; using System.Collections; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Text; using System.Diagnostics; using System.Runtime.InteropServices; using System.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; ////// /// The BufferedGraphics class can be thought of as a "Token" or "Reference" to the /// buffer that a BufferedGraphicsContext creates. While a BufferedGraphics is /// outstanding, the memory associated with the buffer is locked. The general design /// is such that under normal conditions a single BufferedGraphics will be in use at /// one time for a given BufferedGraphicsContext. /// [SuppressMessage("Microsoft.Usage", "CA2216:DisposableTypesShouldDeclareFinalizer")] public sealed class BufferedGraphics : IDisposable { private Graphics bufferedGraphicsSurface; private Graphics targetGraphics; private BufferedGraphicsContext context; private IntPtr targetDC; private Point targetLoc; private Size virtualSize; private bool disposeContext; private static int rop = 0xcc0020; // RasterOp.SOURCE.GetRop(); ////// /// Internal constructor, this class is created by the BufferedGraphicsContext. /// internal BufferedGraphics(Graphics bufferedGraphicsSurface, BufferedGraphicsContext context, Graphics targetGraphics, IntPtr targetDC, Point targetLoc, Size virtualSize) { this.context = context; this.bufferedGraphicsSurface = bufferedGraphicsSurface; this.targetDC = targetDC; this.targetGraphics = targetGraphics; this.targetLoc = targetLoc; this.virtualSize = virtualSize; } ~BufferedGraphics() { Dispose(false); } ////// /// Disposes the object and releases the lock on the memory. /// public void Dispose() { Dispose(true); } private void Dispose(bool disposing) { if (disposing) { if (context != null) { context.ReleaseBuffer(this); if (DisposeContext) { context.Dispose(); context = null; } } if (bufferedGraphicsSurface != null) { bufferedGraphicsSurface.Dispose(); bufferedGraphicsSurface = null; } } } ////// /// Internal property - determines if we need to dispose of the Context when this is disposed /// internal bool DisposeContext { get { return disposeContext; } set { disposeContext = value; } } ////// /// Allows access to the Graphics wrapper for the buffer. /// public Graphics Graphics { get { Debug.Assert(bufferedGraphicsSurface != null, "The BufferedGraphicsSurface is null!"); return bufferedGraphicsSurface; } } ////// /// Renders the buffer to the original graphics used to allocate the buffer. /// public void Render() { if (targetGraphics != null) { Render(targetGraphics); } else { RenderInternal(new HandleRef(Graphics, targetDC), this); } } ////// /// Renders the buffer to the specified target graphics. /// public void Render(Graphics target) { if (target != null) { IntPtr targetDC = target.GetHdc(); try { RenderInternal(new HandleRef(target, targetDC), this); } finally { target.ReleaseHdcInternal(targetDC); } } } ////// /// Renders the buffer to the specified target HDC. /// public void Render(IntPtr targetDC) { IntSecurity.UnmanagedCode.Demand(); RenderInternal(new HandleRef(null, targetDC), this); } ////// /// Internal method that renders the specified buffer into the target. /// private void RenderInternal(HandleRef refTargetDC, BufferedGraphics buffer) { IntPtr sourceDC = buffer.Graphics.GetHdc(); try { SafeNativeMethods.BitBlt(refTargetDC, targetLoc.X, targetLoc.Y, virtualSize.Width, virtualSize.Height, new HandleRef(buffer.Graphics, sourceDC), 0, 0, rop); } finally { buffer.Graphics.ReleaseHdcInternal(sourceDC); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
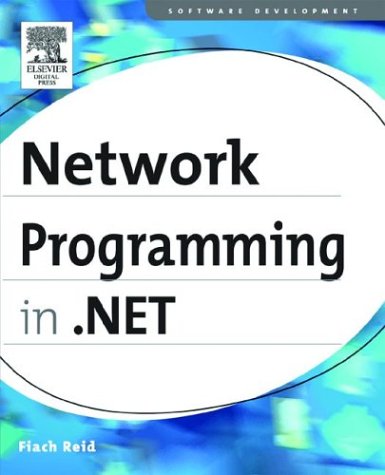
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogicalExpr.cs
- HtmlHistory.cs
- CodeGotoStatement.cs
- HttpCacheVary.cs
- FacetValues.cs
- TrackingMemoryStreamFactory.cs
- StyleSelector.cs
- SessionStateSection.cs
- EventRouteFactory.cs
- VScrollBar.cs
- SHA1CryptoServiceProvider.cs
- TagMapInfo.cs
- TrackingCondition.cs
- ErrorTableItemStyle.cs
- MachinePropertyVariants.cs
- DetailsView.cs
- TreeView.cs
- NullableLongAverageAggregationOperator.cs
- CompilerTypeWithParams.cs
- AnonymousIdentificationModule.cs
- InternalsVisibleToAttribute.cs
- EntityExpressionVisitor.cs
- SqlDelegatedTransaction.cs
- LogArchiveSnapshot.cs
- fixedPageContentExtractor.cs
- XslException.cs
- DBSchemaRow.cs
- propertytag.cs
- CodeSnippetCompileUnit.cs
- XmlUrlResolver.cs
- LogSwitch.cs
- ObservableCollection.cs
- _IPv4Address.cs
- XmlNodeReader.cs
- StyleBamlRecordReader.cs
- SimpleType.cs
- ListViewItemCollectionEditor.cs
- VariableQuery.cs
- XmlStringTable.cs
- HierarchicalDataSourceConverter.cs
- Line.cs
- EntityClassGenerator.cs
- CompModSwitches.cs
- RoleGroupCollectionEditor.cs
- AuthenticationSection.cs
- Transform3DGroup.cs
- UnsafeNativeMethods.cs
- TraceUtility.cs
- DocobjHost.cs
- ChannelTracker.cs
- TextFormatter.cs
- ComponentCollection.cs
- RedBlackList.cs
- PageContentAsyncResult.cs
- ObjectRef.cs
- Symbol.cs
- RuntimeVariableList.cs
- mediaclock.cs
- XamlFigureLengthSerializer.cs
- IndicShape.cs
- ContentControl.cs
- XPathPatternParser.cs
- HashStream.cs
- TreeNodeClickEventArgs.cs
- BamlBinaryWriter.cs
- EmbeddedMailObjectsCollection.cs
- ControlPaint.cs
- ThemeInfoAttribute.cs
- clipboard.cs
- WizardStepBase.cs
- MarginCollapsingState.cs
- WindowsRegion.cs
- WindowsTokenRoleProvider.cs
- LogConverter.cs
- DataSourceView.cs
- CompleteWizardStep.cs
- ObjectHelper.cs
- XmlnsCache.cs
- LabelExpression.cs
- ListViewInsertEventArgs.cs
- TreeNodeConverter.cs
- ApplicationServicesHostFactory.cs
- Main.cs
- TextWriter.cs
- ReachSerializationUtils.cs
- KeySplineConverter.cs
- TagPrefixCollection.cs
- EditorPartChrome.cs
- VisualStates.cs
- linebase.cs
- HatchBrush.cs
- PreservationFileReader.cs
- KeyedCollection.cs
- LoginName.cs
- StaticResourceExtension.cs
- FrameDimension.cs
- Timeline.cs
- ObjectItemCachedAssemblyLoader.cs
- smtpconnection.cs
- NavigationPropertyAccessor.cs