Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Compiler / RuntimeVariableList.cs / 1305376 / RuntimeVariableList.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.ComponentModel; using System.Diagnostics; using System.Linq.Expressions.Compiler; namespace System.Runtime.CompilerServices { ////// This API supports the .NET Framework infrastructure and is not intended to be used directly from your code. /// Contains helper methods called from dynamically generated methods. /// [EditorBrowsable(EditorBrowsableState.Never), DebuggerStepThrough] public static partial class RuntimeOps { ////// Creates an interface that can be used to modify closed over variables at runtime. /// /// The closure array. /// An array of indicies into the closure array where variables are found. ///An interface to access variables. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static IRuntimeVariables CreateRuntimeVariables(object[] data, long[] indexes) { return new RuntimeVariableList(data, indexes); } ////// Creates an interface that can be used to modify closed over variables at runtime. /// ///An interface to access variables. [Obsolete("do not use this method", true), EditorBrowsable(EditorBrowsableState.Never)] public static IRuntimeVariables CreateRuntimeVariables() { return new EmptyRuntimeVariables(); } private sealed class EmptyRuntimeVariables : IRuntimeVariables { int IRuntimeVariables.Count { get { return 0; } } object IRuntimeVariables.this[int index] { get { throw new ArgumentOutOfRangeException("index"); } set { throw new ArgumentOutOfRangeException("index"); } } } ////// Provides a list of variables, supporing read/write of the values /// Exposed via RuntimeVariablesExpression /// private sealed class RuntimeVariableList : IRuntimeVariables { // The top level environment. It contains pointers to parent // environments, which are always in the first element private readonly object[] _data; // An array of (int, int) pairs, each representing how to find a // variable in the environment data struction. // // The first integer indicates the number of times to go up in the // closure chain, the second integer indicates the index into that // closure chain. private readonly long[] _indexes; internal RuntimeVariableList(object[] data, long[] indexes) { Debug.Assert(data != null); Debug.Assert(indexes != null); _data = data; _indexes = indexes; } public int Count { get { return _indexes.Length; } } public object this[int index] { get { return GetStrongBox(index).Value; } set { GetStrongBox(index).Value = value; } } private IStrongBox GetStrongBox(int index) { // We lookup the closure using two ints: // 1. The high dword is the number of parents to go up // 2. The low dword is the index into that array long closureKey = _indexes[index]; // walk up the parent chain to find the real environment object[] result = _data; for (int parents = (int)(closureKey >> 32); parents > 0; parents--) { result = HoistedLocals.GetParent(result); } // Return the variable storage return (IStrongBox)result[(int)closureKey]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
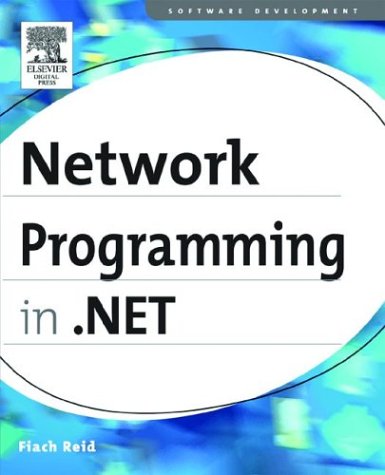
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuaternionConverter.cs
- QilReference.cs
- FileUtil.cs
- EventSinkHelperWriter.cs
- FixedDocumentPaginator.cs
- LineProperties.cs
- QueryCacheManager.cs
- HttpDebugHandler.cs
- Control.cs
- TextPattern.cs
- FusionWrap.cs
- StylusSystemGestureEventArgs.cs
- ToolStripSeparatorRenderEventArgs.cs
- AppLevelCompilationSectionCache.cs
- Point3DCollectionConverter.cs
- RequestStatusBarUpdateEventArgs.cs
- ClientTarget.cs
- DecryptRequest.cs
- ExtensionSimplifierMarkupObject.cs
- RenderingBiasValidation.cs
- CqlLexerHelpers.cs
- ValidatorCollection.cs
- TreeViewItemAutomationPeer.cs
- MarkupWriter.cs
- BindableAttribute.cs
- TreeNodeClickEventArgs.cs
- DataServiceQueryOfT.cs
- CodeEventReferenceExpression.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- sitestring.cs
- ChineseLunisolarCalendar.cs
- DebugView.cs
- ImageClickEventArgs.cs
- hresults.cs
- StringReader.cs
- SelectiveScrollingGrid.cs
- ApplicationFileParser.cs
- GridViewRowPresenter.cs
- ConnectionManagementElement.cs
- CorrelationManager.cs
- DataSourceXmlElementAttribute.cs
- UnauthorizedWebPart.cs
- ControlBuilder.cs
- ToolstripProfessionalRenderer.cs
- SizeIndependentAnimationStorage.cs
- RootBuilder.cs
- DataGridViewColumnEventArgs.cs
- FileDialogCustomPlace.cs
- OleDbPropertySetGuid.cs
- XmlDeclaration.cs
- DocumentApplication.cs
- UserControlCodeDomTreeGenerator.cs
- RijndaelManaged.cs
- SqlClientMetaDataCollectionNames.cs
- SemanticBasicElement.cs
- UserControlBuildProvider.cs
- PasswordTextContainer.cs
- HttpDictionary.cs
- XmlDocumentFragment.cs
- XmlSchemaException.cs
- TreeNodeCollectionEditor.cs
- GeneratedContractType.cs
- TcpTransportManager.cs
- ListItemConverter.cs
- HttpRawResponse.cs
- ConstantSlot.cs
- IdentityElement.cs
- WebBrowser.cs
- XmlSchemaAttributeGroup.cs
- ObjectListComponentEditor.cs
- TextEditorTables.cs
- SoapInteropTypes.cs
- HttpRequestCacheValidator.cs
- ToolStripGripRenderEventArgs.cs
- SerialPinChanges.cs
- _BaseOverlappedAsyncResult.cs
- CanonicalFormWriter.cs
- ArglessEventHandlerProxy.cs
- ServiceProviders.cs
- CounterSet.cs
- WebPartVerbCollection.cs
- RootBrowserWindowProxy.cs
- DecimalConstantAttribute.cs
- RepeatInfo.cs
- NativeMethods.cs
- _ContextAwareResult.cs
- Light.cs
- EventWaitHandle.cs
- CallSiteBinder.cs
- PagesSection.cs
- ReferencedType.cs
- X509Chain.cs
- TargetParameterCountException.cs
- _CacheStreams.cs
- TextBlockAutomationPeer.cs
- EntityParameter.cs
- ListViewDataItem.cs
- Int64AnimationBase.cs
- ImpersonationContext.cs
- XMLSchema.cs