Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceQueryOfT.cs / 4 / DataServiceQueryOfT.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// query object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Linq; using System.Linq.Expressions; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif using System.Text; using System.Xml; using System.Reflection; ////// query object /// ///type of object to materialize [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix", Justification = "required for this feature")] public class DataServiceQuery: DataServiceQuery, IQueryable { /// Linq Expression private readonly Expression queryExpression; ///Linq Query Provider private readonly DataServiceQueryProvider queryProvider; ////// query object /// /// expression for query /// query provider for query private DataServiceQuery(Expression expression, DataServiceQueryProvider provider) { Debug.Assert(null != provider.Context, "null context"); Debug.Assert(expression != null, "null expression"); Debug.Assert(provider is DataServiceQueryProvider, "Currently only support Web Query Provider"); this.queryExpression = expression; this.queryProvider = provider; } #region IQueryable implementation ////// Element Type /// public override Type ElementType { get { return typeof(TElement); } } ////// Linq Expression /// public override Expression Expression { get { return this.queryExpression; } } ////// Linq Query Provider /// public override IQueryProvider Provider { get { return this.queryProvider; } } #endregion ////// gets the URI for a the query /// public override Uri RequestUri { get { return this.queryProvider.Translate(this.queryExpression); } } ////// Begins an asynchronous request to an Internet resource. /// /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. public IAsyncResult BeginExecute(AsyncCallback callback, object state) { return base.BeginExecute(this, this.queryProvider.Context, callback, state); } ////// Ends an asynchronous request to an Internet resource. /// /// The pending request for a response. ///An IEnumerable that contains the response from the Internet resource. public IEnumerableEndExecute(IAsyncResult asyncResult) { QueryAsyncResult response = QueryAsyncResult.EndExecute (this, "Execute", asyncResult); IEnumerable result = null; if (HttpStatusCode.NoContent != response.StatusCode) { result = Materialize (this.queryProvider.Context, response.ContentType, response.GetResponseStream); } return (IEnumerable )response.GetResponse(result, typeof(TElement)); } #if !ASTORIA_LIGHT // Synchronous methods not available /// /// Returns an IEnumerable from an Internet resource. /// ///An IEnumerable that contains the response from the Internet resource. public IEnumerableExecute() { return this.Execute (this.queryProvider.Context, this.RequestUri); } #endif /// /// Sets Expand option /// /// Path to expand ///New DataServiceQuery with expand option public DataServiceQueryExpand(string path) { Util.CheckArgumentNull(path, "path"); Util.CheckArgumentNotEmpty(path, "path"); MethodInfo mi = typeof(DataServiceQuery ).GetMethod("Expand"); return (DataServiceQuery )this.Provider.CreateQuery ( Expression.Call( Expression.Convert(this.Expression, typeof(DataServiceQuery )), mi, new Expression[] { Expression.Constant(path) })); } /// /// Sets user option /// /// name of value /// value of option ///New DataServiceQuery with expand option public DataServiceQueryAddQueryOption(string name, object value) { Util.CheckArgumentNull(name, "name"); Util.CheckArgumentNull(value, "value"); MethodInfo mi = typeof(DataServiceQuery ).GetMethod("AddQueryOption"); return (DataServiceQuery )this.Provider.CreateQuery ( Expression.Call( Expression.Convert(this.Expression, typeof(DataServiceQuery )), mi, new Expression[] { Expression.Constant(name), Expression.Constant(value, typeof(object)) })); } /// /// get an enumerator materializes the objects the Uri request /// ///an enumerator #if !ASTORIA_LIGHT // Synchronous methods not available public IEnumeratorGetEnumerator() { return this.Execute().GetEnumerator(); } #else IEnumerator IEnumerable .GetEnumerator() { throw Error.NotSupported(); } #endif /// /// gets the URI for a the query /// ///a string with the URI public override string ToString() { try { return base.ToString(); } catch (NotSupportedException e) { return Strings.ALinq_TranslationError(e.Message); } } ////// get an enumerator materializes the objects the Uri request /// ///an enumerator System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { #if !ASTORIA_LIGHT // Synchronous methods not available return this.GetEnumerator(); #else throw Error.NotSupported(); #endif } ///enumerable list of materialized objects from stream without having to know T /// context /// contentType /// function to get response stream ///see summary internal override System.Collections.IEnumerable Materialize(DataServiceContext context, string contentType, Funcresponse) { return Materialize (context, contentType, response); } /// /// Ordered DataServiceQuery which implements IOrderedQueryable. /// internal class DataServiceOrderedQuery : DataServiceQuery, IOrderedQueryable , IOrderedQueryable { /// /// constructor /// /// expression for query /// query provider for query internal DataServiceOrderedQuery(Expression expression, DataServiceQueryProvider provider) : base(expression, provider) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// query object // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; using System.Linq; using System.Linq.Expressions; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif using System.Text; using System.Xml; using System.Reflection; ////// query object /// ///type of object to materialize [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix", Justification = "required for this feature")] public class DataServiceQuery: DataServiceQuery, IQueryable { /// Linq Expression private readonly Expression queryExpression; ///Linq Query Provider private readonly DataServiceQueryProvider queryProvider; ////// query object /// /// expression for query /// query provider for query private DataServiceQuery(Expression expression, DataServiceQueryProvider provider) { Debug.Assert(null != provider.Context, "null context"); Debug.Assert(expression != null, "null expression"); Debug.Assert(provider is DataServiceQueryProvider, "Currently only support Web Query Provider"); this.queryExpression = expression; this.queryProvider = provider; } #region IQueryable implementation ////// Element Type /// public override Type ElementType { get { return typeof(TElement); } } ////// Linq Expression /// public override Expression Expression { get { return this.queryExpression; } } ////// Linq Query Provider /// public override IQueryProvider Provider { get { return this.queryProvider; } } #endregion ////// gets the URI for a the query /// public override Uri RequestUri { get { return this.queryProvider.Translate(this.queryExpression); } } ////// Begins an asynchronous request to an Internet resource. /// /// The AsyncCallback delegate. /// The state object for this request. ///An IAsyncResult that references the asynchronous request for a response. public IAsyncResult BeginExecute(AsyncCallback callback, object state) { return base.BeginExecute(this, this.queryProvider.Context, callback, state); } ////// Ends an asynchronous request to an Internet resource. /// /// The pending request for a response. ///An IEnumerable that contains the response from the Internet resource. public IEnumerableEndExecute(IAsyncResult asyncResult) { QueryAsyncResult response = QueryAsyncResult.EndExecute (this, "Execute", asyncResult); IEnumerable result = null; if (HttpStatusCode.NoContent != response.StatusCode) { result = Materialize (this.queryProvider.Context, response.ContentType, response.GetResponseStream); } return (IEnumerable )response.GetResponse(result, typeof(TElement)); } #if !ASTORIA_LIGHT // Synchronous methods not available /// /// Returns an IEnumerable from an Internet resource. /// ///An IEnumerable that contains the response from the Internet resource. public IEnumerableExecute() { return this.Execute (this.queryProvider.Context, this.RequestUri); } #endif /// /// Sets Expand option /// /// Path to expand ///New DataServiceQuery with expand option public DataServiceQueryExpand(string path) { Util.CheckArgumentNull(path, "path"); Util.CheckArgumentNotEmpty(path, "path"); MethodInfo mi = typeof(DataServiceQuery ).GetMethod("Expand"); return (DataServiceQuery )this.Provider.CreateQuery ( Expression.Call( Expression.Convert(this.Expression, typeof(DataServiceQuery )), mi, new Expression[] { Expression.Constant(path) })); } /// /// Sets user option /// /// name of value /// value of option ///New DataServiceQuery with expand option public DataServiceQueryAddQueryOption(string name, object value) { Util.CheckArgumentNull(name, "name"); Util.CheckArgumentNull(value, "value"); MethodInfo mi = typeof(DataServiceQuery ).GetMethod("AddQueryOption"); return (DataServiceQuery )this.Provider.CreateQuery ( Expression.Call( Expression.Convert(this.Expression, typeof(DataServiceQuery )), mi, new Expression[] { Expression.Constant(name), Expression.Constant(value, typeof(object)) })); } /// /// get an enumerator materializes the objects the Uri request /// ///an enumerator #if !ASTORIA_LIGHT // Synchronous methods not available public IEnumeratorGetEnumerator() { return this.Execute().GetEnumerator(); } #else IEnumerator IEnumerable .GetEnumerator() { throw Error.NotSupported(); } #endif /// /// gets the URI for a the query /// ///a string with the URI public override string ToString() { try { return base.ToString(); } catch (NotSupportedException e) { return Strings.ALinq_TranslationError(e.Message); } } ////// get an enumerator materializes the objects the Uri request /// ///an enumerator System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { #if !ASTORIA_LIGHT // Synchronous methods not available return this.GetEnumerator(); #else throw Error.NotSupported(); #endif } ///enumerable list of materialized objects from stream without having to know T /// context /// contentType /// function to get response stream ///see summary internal override System.Collections.IEnumerable Materialize(DataServiceContext context, string contentType, Funcresponse) { return Materialize (context, contentType, response); } /// /// Ordered DataServiceQuery which implements IOrderedQueryable. /// internal class DataServiceOrderedQuery : DataServiceQuery, IOrderedQueryable , IOrderedQueryable { /// /// constructor /// /// expression for query /// query provider for query internal DataServiceOrderedQuery(Expression expression, DataServiceQueryProvider provider) : base(expression, provider) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
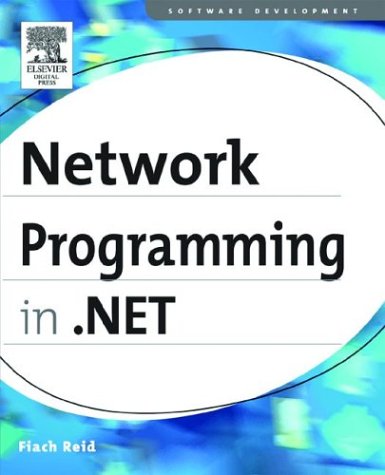
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CanonicalizationDriver.cs
- ModelEditingScope.cs
- PeerCollaborationPermission.cs
- CoTaskMemSafeHandle.cs
- MenuItemStyleCollection.cs
- BitFlagsGenerator.cs
- CharConverter.cs
- QilTargetType.cs
- UrlMappingsSection.cs
- PopupRoot.cs
- CellTreeNodeVisitors.cs
- CallbackTimeoutsElement.cs
- StreamGeometryContext.cs
- SmtpReplyReader.cs
- FolderBrowserDialog.cs
- ExtensionFile.cs
- PackageStore.cs
- XmlChildNodes.cs
- CustomErrorCollection.cs
- Globals.cs
- ListControl.cs
- UserControl.cs
- ProfileServiceManager.cs
- DoubleLinkList.cs
- VirtualizingPanel.cs
- SerialPort.cs
- ToolStrip.cs
- Imaging.cs
- SourceFileBuildProvider.cs
- AbandonedMutexException.cs
- ActivityLocationReferenceEnvironment.cs
- CoreSwitches.cs
- DefaultExpression.cs
- Size.cs
- ExpressionList.cs
- LinearGradientBrush.cs
- EnumerableRowCollectionExtensions.cs
- ContentPresenter.cs
- DispatchWrapper.cs
- CAGDesigner.cs
- LineGeometry.cs
- WorkflowFormatterBehavior.cs
- SqlMultiplexer.cs
- WrappedOptions.cs
- BitmapSourceSafeMILHandle.cs
- GridViewRow.cs
- StateItem.cs
- DataGridViewCellLinkedList.cs
- XPathPatternBuilder.cs
- BasicExpandProvider.cs
- HwndTarget.cs
- ContentType.cs
- WmpBitmapEncoder.cs
- AudioStateChangedEventArgs.cs
- ContentDefinition.cs
- ProtocolViolationException.cs
- SignatureHelper.cs
- DisplayMemberTemplateSelector.cs
- ByteStorage.cs
- DateTimeConverter.cs
- Attributes.cs
- DataGridViewIntLinkedList.cs
- PasswordRecovery.cs
- Unit.cs
- XmlSiteMapProvider.cs
- CompiledIdentityConstraint.cs
- SByte.cs
- RuntimeVariablesExpression.cs
- Int32Rect.cs
- FilteredAttributeCollection.cs
- Opcode.cs
- DescendentsWalker.cs
- CallContext.cs
- CustomAttribute.cs
- FileLevelControlBuilderAttribute.cs
- LabelDesigner.cs
- SR.cs
- SrgsToken.cs
- WebPartCatalogAddVerb.cs
- Journal.cs
- TextElementEditingBehaviorAttribute.cs
- BridgeDataReader.cs
- SimpleTextLine.cs
- CompilerState.cs
- ReadOnlyObservableCollection.cs
- AnnotationResourceCollection.cs
- XmlnsCache.cs
- TemplateControlParser.cs
- HtmlInputSubmit.cs
- basecomparevalidator.cs
- FixedPageProcessor.cs
- TreeSet.cs
- HandleExceptionArgs.cs
- HttpCacheParams.cs
- SolidColorBrush.cs
- PropertyMappingExceptionEventArgs.cs
- ZipPackage.cs
- ImageCodecInfo.cs
- XmlNamespaceMapping.cs
- XmlUtf8RawTextWriter.cs