Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / VirtualizingPanel.cs / 1 / VirtualizingPanel.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Windows.Media; using System.Windows.Controls.Primitives; // IItemContainerGenerator namespace System.Windows.Controls { ////// A base class that provides access to information that is useful for panels that with to implement virtualization. /// public abstract class VirtualizingPanel : Panel { ////// The default constructor. /// protected VirtualizingPanel() : base(true) { } ////// The generator associated with this panel. /// public IItemContainerGenerator ItemContainerGenerator { get { return Generator; } } ////// Adds a child to the InternalChildren collection. /// This method is meant to be used when a virtualizing panel /// generates a new child. This method circumvents some validation /// that occurs in UIElementCollection.Add. /// /// Child to add. protected void AddInternalChild(UIElement child) { AddInternalChild(InternalChildren, child); } ////// Inserts a child into the InternalChildren collection. /// This method is meant to be used when a virtualizing panel /// generates a new child. This method circumvents some validation /// that occurs in UIElementCollection.Insert. /// /// The index at which to insert the child. /// Child to insert. protected void InsertInternalChild(int index, UIElement child) { InsertInternalChild(InternalChildren, index, child); } ////// Removes a child from the InternalChildren collection. /// This method is meant to be used when a virtualizing panel /// re-virtualizes a new child. This method circumvents some validation /// that occurs in UIElementCollection.RemoveRange. /// /// /// protected void RemoveInternalChildRange(int index, int range) { RemoveInternalChildRange(InternalChildren, index, range); } // This is internal as an optimization for VirtualizingStackPanel (so it doesn't need to re-query InternalChildren repeatedly) internal static void AddInternalChild(UIElementCollection children, UIElement child) { children.AddInternal(child); } // This is internal as an optimization for VirtualizingStackPanel (so it doesn't need to re-query InternalChildren repeatedly) internal static void InsertInternalChild(UIElementCollection children, int index, UIElement child) { children.InsertInternal(index, child); } // This is internal as an optimization for VirtualizingStackPanel (so it doesn't need to re-query InternalChildren repeatedly) internal static void RemoveInternalChildRange(UIElementCollection children, int index, int range) { children.RemoveRangeInternal(index, range); } ////// Called when the Items collection associated with the containing ItemsControl changes. /// /// sender /// Event arguments protected virtual void OnItemsChanged(object sender, ItemsChangedEventArgs args) { } ////// Called when the UI collection of children is cleared by the base Panel class. /// protected virtual void OnClearChildren() { } ////// Generates the item at the specified index and calls BringIntoView on it. /// /// Specify the item index that should become visible protected internal virtual void BringIndexIntoView(int index) { } internal override void OnItemsChangedInternal(object sender, ItemsChangedEventArgs args) { switch (args.Action) { case NotifyCollectionChangedAction.Add: case NotifyCollectionChangedAction.Remove: case NotifyCollectionChangedAction.Replace: case NotifyCollectionChangedAction.Move: // Don't allow Panel's code to run for add/remove/replace/move break; default: base.OnItemsChangedInternal(sender, args); break; } OnItemsChanged(sender, args); } internal override void OnClearChildrenInternal() { OnClearChildren(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Windows.Media; using System.Windows.Controls.Primitives; // IItemContainerGenerator namespace System.Windows.Controls { ////// A base class that provides access to information that is useful for panels that with to implement virtualization. /// public abstract class VirtualizingPanel : Panel { ////// The default constructor. /// protected VirtualizingPanel() : base(true) { } ////// The generator associated with this panel. /// public IItemContainerGenerator ItemContainerGenerator { get { return Generator; } } ////// Adds a child to the InternalChildren collection. /// This method is meant to be used when a virtualizing panel /// generates a new child. This method circumvents some validation /// that occurs in UIElementCollection.Add. /// /// Child to add. protected void AddInternalChild(UIElement child) { AddInternalChild(InternalChildren, child); } ////// Inserts a child into the InternalChildren collection. /// This method is meant to be used when a virtualizing panel /// generates a new child. This method circumvents some validation /// that occurs in UIElementCollection.Insert. /// /// The index at which to insert the child. /// Child to insert. protected void InsertInternalChild(int index, UIElement child) { InsertInternalChild(InternalChildren, index, child); } ////// Removes a child from the InternalChildren collection. /// This method is meant to be used when a virtualizing panel /// re-virtualizes a new child. This method circumvents some validation /// that occurs in UIElementCollection.RemoveRange. /// /// /// protected void RemoveInternalChildRange(int index, int range) { RemoveInternalChildRange(InternalChildren, index, range); } // This is internal as an optimization for VirtualizingStackPanel (so it doesn't need to re-query InternalChildren repeatedly) internal static void AddInternalChild(UIElementCollection children, UIElement child) { children.AddInternal(child); } // This is internal as an optimization for VirtualizingStackPanel (so it doesn't need to re-query InternalChildren repeatedly) internal static void InsertInternalChild(UIElementCollection children, int index, UIElement child) { children.InsertInternal(index, child); } // This is internal as an optimization for VirtualizingStackPanel (so it doesn't need to re-query InternalChildren repeatedly) internal static void RemoveInternalChildRange(UIElementCollection children, int index, int range) { children.RemoveRangeInternal(index, range); } ////// Called when the Items collection associated with the containing ItemsControl changes. /// /// sender /// Event arguments protected virtual void OnItemsChanged(object sender, ItemsChangedEventArgs args) { } ////// Called when the UI collection of children is cleared by the base Panel class. /// protected virtual void OnClearChildren() { } ////// Generates the item at the specified index and calls BringIntoView on it. /// /// Specify the item index that should become visible protected internal virtual void BringIndexIntoView(int index) { } internal override void OnItemsChangedInternal(object sender, ItemsChangedEventArgs args) { switch (args.Action) { case NotifyCollectionChangedAction.Add: case NotifyCollectionChangedAction.Remove: case NotifyCollectionChangedAction.Replace: case NotifyCollectionChangedAction.Move: // Don't allow Panel's code to run for add/remove/replace/move break; default: base.OnItemsChangedInternal(sender, args); break; } OnItemsChanged(sender, args); } internal override void OnClearChildrenInternal() { OnClearChildren(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
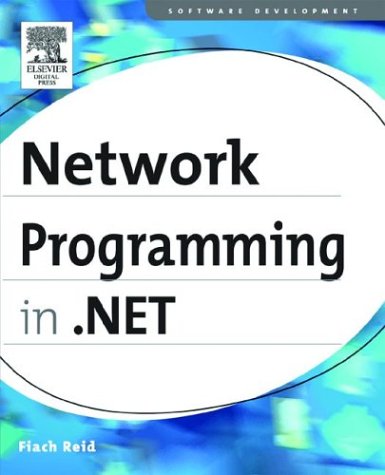
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ElementProxy.cs
- RowParagraph.cs
- ToolStripContentPanelRenderEventArgs.cs
- HtmlHistory.cs
- CodeEntryPointMethod.cs
- CfgRule.cs
- ParseHttpDate.cs
- Registry.cs
- ErrorRuntimeConfig.cs
- MetabaseReader.cs
- Vector3DCollection.cs
- BufferedGraphicsManager.cs
- ActivityXRefPropertyEditor.cs
- WebPartZoneBase.cs
- HyperLinkField.cs
- BaseCodePageEncoding.cs
- DiagnosticSection.cs
- WebPartDisplayModeEventArgs.cs
- AlphabetConverter.cs
- DesignerSerializerAttribute.cs
- DoubleLinkListEnumerator.cs
- SqlDataSourceQueryEditor.cs
- HttpCookiesSection.cs
- SeverityFilter.cs
- OperatorExpressions.cs
- Int64Storage.cs
- Int32EqualityComparer.cs
- CodeCastExpression.cs
- OrderByBuilder.cs
- MexBindingBindingCollectionElement.cs
- EventPrivateKey.cs
- ServiceOperationUIEditor.cs
- RetrieveVirtualItemEventArgs.cs
- ConfigPathUtility.cs
- AtlasWeb.Designer.cs
- MatrixKeyFrameCollection.cs
- codemethodreferenceexpression.cs
- HybridDictionary.cs
- WebConfigurationManager.cs
- Rectangle.cs
- MetadataResolver.cs
- ClientConvert.cs
- JsonMessageEncoderFactory.cs
- ListViewItem.cs
- XmlILConstructAnalyzer.cs
- formatter.cs
- _FixedSizeReader.cs
- ScriptControl.cs
- DefaultParameterValueAttribute.cs
- Journaling.cs
- Currency.cs
- ThicknessKeyFrameCollection.cs
- MethodImplAttribute.cs
- StickyNoteAnnotations.cs
- RectAnimationBase.cs
- NativeMethodsCLR.cs
- ApplicationManager.cs
- NetDispatcherFaultException.cs
- DataFormats.cs
- HandoffBehavior.cs
- GraphicsPathIterator.cs
- MD5.cs
- FormParameter.cs
- SettingsPropertyValueCollection.cs
- Queue.cs
- WindowsListViewScroll.cs
- CopyNamespacesAction.cs
- _IPv6Address.cs
- MexHttpBindingElement.cs
- XmlnsDefinitionAttribute.cs
- PanelStyle.cs
- EnvelopedPkcs7.cs
- Floater.cs
- WorkBatch.cs
- input.cs
- SafeBuffer.cs
- MultiViewDesigner.cs
- ListChangedEventArgs.cs
- MetadataItem_Static.cs
- SeekStoryboard.cs
- MediaCommands.cs
- OdbcConnectionString.cs
- ResourceCategoryAttribute.cs
- XmlNamespaceMappingCollection.cs
- SessionIDManager.cs
- formatter.cs
- _NetRes.cs
- TrackingMemoryStream.cs
- RestHandler.cs
- BinaryCommonClasses.cs
- BypassElementCollection.cs
- TemplateKeyConverter.cs
- ArrangedElementCollection.cs
- ToolStripPanelRenderEventArgs.cs
- Drawing.cs
- AudioBase.cs
- ZipPackage.cs
- DataSysAttribute.cs
- WebConfigurationHostFileChange.cs
- ValidateNames.cs