Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / HtmlHistory.cs / 1 / HtmlHistory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Drawing; using System.Drawing.Printing; using System.Windows.Forms; using System.Security.Permissions; using System.Security; using System.Runtime.InteropServices; using System.Net; using System.Globalization; namespace System.Windows.Forms { ////// /// [PermissionSetAttribute(SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class HtmlHistory : IDisposable { private UnsafeNativeMethods.IOmHistory htmlHistory; private bool disposed; [PermissionSet(SecurityAction.Demand, Name = "FullTrust")] internal HtmlHistory(UnsafeNativeMethods.IOmHistory history) { this.htmlHistory = history; Debug.Assert(this.NativeOmHistory != null, "The history object should implement IOmHistory"); } private UnsafeNativeMethods.IOmHistory NativeOmHistory { get { if (this.disposed) { throw new System.ObjectDisposedException(GetType().Name); } return this.htmlHistory; } } ///[To be supplied.] ///public void Dispose() { this.htmlHistory = null; this.disposed = true; GC.SuppressFinalize(this); } /// /// /// public int Length { get { return (int)this.NativeOmHistory.GetLength(); } } ///[To be supplied.] ////// /// public void Back(int numberBack) { if (numberBack < 0) { throw new ArgumentOutOfRangeException("numberBack", SR.GetString(SR.InvalidLowBoundArgumentEx, "numberBack", numberBack.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } else if (numberBack > 0) { object oNumForward = (object)(-numberBack); this.NativeOmHistory.Go(ref oNumForward); } } ///[To be supplied.] ////// /// public void Forward(int numberForward) { if (numberForward < 0) { throw new ArgumentOutOfRangeException("numberForward", SR.GetString(SR.InvalidLowBoundArgumentEx, "numberForward", numberForward.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } else if (numberForward > 0) { object oNumForward = (object)numberForward; this.NativeOmHistory.Go(ref oNumForward); } } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Usage", "CA2234:PassSystemUriObjectsInsteadOfStrings")] public void Go(Uri url) { Go(url.ToString()); } ///Go to a specific Uri in the history ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public void Go(string urlString) { object loc = (object)urlString; this.NativeOmHistory.Go(ref loc); } ///Go to a specific url(string) in the history ////// /// public void Go(int relativePosition) { object loc = (object)relativePosition; this.NativeOmHistory.Go(ref loc); } ///Go to the specified position in the history list ////// /// public object DomHistory { get { return this.NativeOmHistory; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.IO; using System.Drawing; using System.Drawing.Printing; using System.Windows.Forms; using System.Security.Permissions; using System.Security; using System.Runtime.InteropServices; using System.Net; using System.Globalization; namespace System.Windows.Forms { ////// /// [PermissionSetAttribute(SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class HtmlHistory : IDisposable { private UnsafeNativeMethods.IOmHistory htmlHistory; private bool disposed; [PermissionSet(SecurityAction.Demand, Name = "FullTrust")] internal HtmlHistory(UnsafeNativeMethods.IOmHistory history) { this.htmlHistory = history; Debug.Assert(this.NativeOmHistory != null, "The history object should implement IOmHistory"); } private UnsafeNativeMethods.IOmHistory NativeOmHistory { get { if (this.disposed) { throw new System.ObjectDisposedException(GetType().Name); } return this.htmlHistory; } } ///[To be supplied.] ///public void Dispose() { this.htmlHistory = null; this.disposed = true; GC.SuppressFinalize(this); } /// /// /// public int Length { get { return (int)this.NativeOmHistory.GetLength(); } } ///[To be supplied.] ////// /// public void Back(int numberBack) { if (numberBack < 0) { throw new ArgumentOutOfRangeException("numberBack", SR.GetString(SR.InvalidLowBoundArgumentEx, "numberBack", numberBack.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } else if (numberBack > 0) { object oNumForward = (object)(-numberBack); this.NativeOmHistory.Go(ref oNumForward); } } ///[To be supplied.] ////// /// public void Forward(int numberForward) { if (numberForward < 0) { throw new ArgumentOutOfRangeException("numberForward", SR.GetString(SR.InvalidLowBoundArgumentEx, "numberForward", numberForward.ToString(CultureInfo.CurrentCulture), (0).ToString(CultureInfo.CurrentCulture))); } else if (numberForward > 0) { object oNumForward = (object)numberForward; this.NativeOmHistory.Go(ref oNumForward); } } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Usage", "CA2234:PassSystemUriObjectsInsteadOfStrings")] public void Go(Uri url) { Go(url.ToString()); } ///Go to a specific Uri in the history ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public void Go(string urlString) { object loc = (object)urlString; this.NativeOmHistory.Go(ref loc); } ///Go to a specific url(string) in the history ////// /// public void Go(int relativePosition) { object loc = (object)relativePosition; this.NativeOmHistory.Go(ref loc); } ///Go to the specified position in the history list ////// /// public object DomHistory { get { return this.NativeOmHistory; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
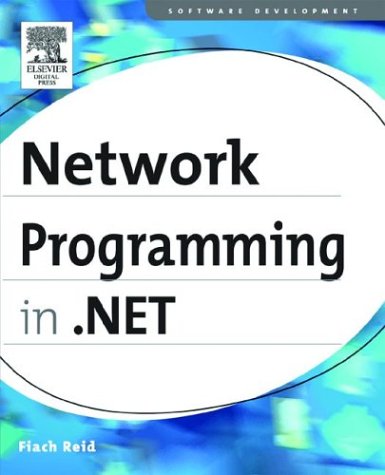
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DeviceContext2.cs
- WorkflowQueuingService.cs
- CodePrimitiveExpression.cs
- SelectionService.cs
- TypeDelegator.cs
- PersistenceException.cs
- TextRangeBase.cs
- GenericWebPart.cs
- DataGridAutoFormatDialog.cs
- TableChangeProcessor.cs
- JsonDeserializer.cs
- ServiceControllerDesigner.cs
- dataprotectionpermissionattribute.cs
- ImpersonationContext.cs
- SchemaDeclBase.cs
- TabPage.cs
- PaperSize.cs
- MarshalDirectiveException.cs
- RemoteWebConfigurationHost.cs
- EventItfInfo.cs
- XhtmlConformanceSection.cs
- ProofTokenCryptoHandle.cs
- Matrix3D.cs
- InputProcessorProfiles.cs
- StringCollection.cs
- Padding.cs
- WebConfigurationHost.cs
- DateTimeUtil.cs
- FileDataSourceCache.cs
- ParagraphVisual.cs
- DataRelation.cs
- ToggleButton.cs
- SmiConnection.cs
- OracleNumber.cs
- Wildcard.cs
- TaiwanCalendar.cs
- MethodExpr.cs
- ScriptingWebServicesSectionGroup.cs
- SqlTypeConverter.cs
- WSFederationHttpBindingCollectionElement.cs
- LingerOption.cs
- SqlUnionizer.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- OptimizerPatterns.cs
- HistoryEventArgs.cs
- CompositeCollectionView.cs
- WebDisplayNameAttribute.cs
- AnonymousIdentificationModule.cs
- VerificationException.cs
- OrderPreservingPipeliningMergeHelper.cs
- SizeConverter.cs
- CompilerParameters.cs
- MouseGesture.cs
- X509ClientCertificateCredentialsElement.cs
- SafeCertificateContext.cs
- ExpressionParser.cs
- ICollection.cs
- PenThreadWorker.cs
- SendActivityEventArgs.cs
- XsltException.cs
- ItemsChangedEventArgs.cs
- GeometryDrawing.cs
- ListCardsInFileRequest.cs
- WorkBatch.cs
- QilBinary.cs
- DataSourceView.cs
- PropertyChangedEventArgs.cs
- XhtmlBasicValidationSummaryAdapter.cs
- HotSpot.cs
- FormViewActionList.cs
- FileLogRecordStream.cs
- StrongNameUtility.cs
- NodeInfo.cs
- XmlSerializationReader.cs
- TypeSemantics.cs
- TypeContext.cs
- DomNameTable.cs
- XmlStreamNodeWriter.cs
- ConfigXmlText.cs
- LogEntryUtils.cs
- TransactionException.cs
- WebPart.cs
- KeyValueInternalCollection.cs
- DropShadowBitmapEffect.cs
- TemporaryBitmapFile.cs
- SecureEnvironment.cs
- SecurityElement.cs
- DebugView.cs
- IFlowDocumentViewer.cs
- PtsCache.cs
- AssociationSet.cs
- PageTheme.cs
- MimeImporter.cs
- GlyphsSerializer.cs
- HttpContext.cs
- ContextMenuStrip.cs
- ObjectView.cs
- _LazyAsyncResult.cs
- PropertyMapper.cs
- EventSetter.cs