Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / PtsHost / ParagraphVisual.cs / 1305600 / ParagraphVisual.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ParagraphVisaul.cs // // Description: Visual representing a paragraph. // // History: // 05/20/2003 : [....] - created. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; namespace MS.Internal.PtsHost { ////// Visual representing a paragraph. /// internal class ParagraphVisual : DrawingVisual { ////// Constructor. /// internal ParagraphVisual() { _renderBounds = Rect.Empty; } ////// Draw background and border. /// /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorder(Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { if (_backgroundBrush != backgroundBrush || _renderBounds != renderBounds || _borderBrush != borderBrush || !Thickness.AreClose(_borderThickness, borderThickness)) { // Open DrawingContext and draw background. using (DrawingContext dc = RenderOpen()) { DrawBackgroundAndBorderIntoContext(dc, backgroundBrush, borderBrush, borderThickness, renderBounds, isFirstChunk, isLastChunk); } } } ////// Draw background and border. /// /// Drawing context. /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorderIntoContext(DrawingContext dc, Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { // We do not want to cause the user's Brushes to become frozen when we // freeze the Pen in OnRender, therefore we make our own copy of the // Brushes if they are not already frozen. _backgroundBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(backgroundBrush); _renderBounds = renderBounds; _borderBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(borderBrush); _borderThickness = borderThickness; // Exclude top/bottom border if this is not the first/last chunk of the paragraph. if (!isFirstChunk) { _borderThickness.Top = 0.0; } if (!isLastChunk) { _borderThickness.Bottom = 0.0; } // If we have a brush with which to draw the border, do so. // NB: We double draw corners right now. Corner handling is tricky (bevelling, &c...) and // we need a firm spec before doing "the right thing." (greglett, ffortes) if (_borderBrush != null) { // Initialize the first pen. Note that each pen is created via new() // and frozen if possible. Doing this avoids the overhead of // maintaining changed handlers. Pen pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Left; if (pen.CanFreeze) { pen.Freeze(); } if (_borderThickness.IsUniform) { // Uniform border; stroke a rectangle. dc.DrawRectangle(null, pen, new Rect( new Point(_renderBounds.Left + pen.Thickness * 0.5, _renderBounds.Bottom - pen.Thickness * 0.5), new Point(_renderBounds.Right - pen.Thickness * 0.5, _renderBounds.Top + pen.Thickness * 0.5))); } else { if (DoubleUtil.GreaterThan(_borderThickness.Left, 0)) { dc.DrawLine(pen, new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Right, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Right; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Top, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Top; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Top + pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Top + pen.Thickness / 2)); } if (DoubleUtil.GreaterThan(_borderThickness.Bottom, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Bottom; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Bottom - pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Bottom - pen.Thickness / 2)); } } } // Draw background in rectangle inside border. if (_backgroundBrush != null) { dc.DrawRectangle(_backgroundBrush, null, new Rect( new Point(_renderBounds.Left + _borderThickness.Left, _renderBounds.Top + _borderThickness.Top), new Point(_renderBounds.Right - _borderThickness.Right, _renderBounds.Bottom - _borderThickness.Bottom))); } } private Brush _backgroundBrush; // Background brush private Brush _borderBrush; // Border brush private Thickness _borderThickness; // Border thickness private Rect _renderBounds; // Render bounds of the visual } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ParagraphVisaul.cs // // Description: Visual representing a paragraph. // // History: // 05/20/2003 : [....] - created. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; namespace MS.Internal.PtsHost { ////// Visual representing a paragraph. /// internal class ParagraphVisual : DrawingVisual { ////// Constructor. /// internal ParagraphVisual() { _renderBounds = Rect.Empty; } ////// Draw background and border. /// /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorder(Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { if (_backgroundBrush != backgroundBrush || _renderBounds != renderBounds || _borderBrush != borderBrush || !Thickness.AreClose(_borderThickness, borderThickness)) { // Open DrawingContext and draw background. using (DrawingContext dc = RenderOpen()) { DrawBackgroundAndBorderIntoContext(dc, backgroundBrush, borderBrush, borderThickness, renderBounds, isFirstChunk, isLastChunk); } } } ////// Draw background and border. /// /// Drawing context. /// The brush used for background. /// The brush used for border. /// Border thickness. /// Render bounds of the visual. /// Whether this is paragraph's first chunk. /// Whether this is paragraph's last chunk. internal void DrawBackgroundAndBorderIntoContext(DrawingContext dc, Brush backgroundBrush, Brush borderBrush, Thickness borderThickness, Rect renderBounds, bool isFirstChunk, bool isLastChunk) { // We do not want to cause the user's Brushes to become frozen when we // freeze the Pen in OnRender, therefore we make our own copy of the // Brushes if they are not already frozen. _backgroundBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(backgroundBrush); _renderBounds = renderBounds; _borderBrush = (Brush)FreezableOperations.GetAsFrozenIfPossible(borderBrush); _borderThickness = borderThickness; // Exclude top/bottom border if this is not the first/last chunk of the paragraph. if (!isFirstChunk) { _borderThickness.Top = 0.0; } if (!isLastChunk) { _borderThickness.Bottom = 0.0; } // If we have a brush with which to draw the border, do so. // NB: We double draw corners right now. Corner handling is tricky (bevelling, &c...) and // we need a firm spec before doing "the right thing." (greglett, ffortes) if (_borderBrush != null) { // Initialize the first pen. Note that each pen is created via new() // and frozen if possible. Doing this avoids the overhead of // maintaining changed handlers. Pen pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Left; if (pen.CanFreeze) { pen.Freeze(); } if (_borderThickness.IsUniform) { // Uniform border; stroke a rectangle. dc.DrawRectangle(null, pen, new Rect( new Point(_renderBounds.Left + pen.Thickness * 0.5, _renderBounds.Bottom - pen.Thickness * 0.5), new Point(_renderBounds.Right - pen.Thickness * 0.5, _renderBounds.Top + pen.Thickness * 0.5))); } else { if (DoubleUtil.GreaterThan(_borderThickness.Left, 0)) { dc.DrawLine(pen, new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Left + pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Right, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Right; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Top), new Point(_renderBounds.Right - pen.Thickness / 2, _renderBounds.Bottom)); } if (DoubleUtil.GreaterThan(_borderThickness.Top, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Top; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Top + pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Top + pen.Thickness / 2)); } if (DoubleUtil.GreaterThan(_borderThickness.Bottom, 0)) { pen = new Pen(); pen.Brush = _borderBrush; pen.Thickness = _borderThickness.Bottom; if (pen.CanFreeze) { pen.Freeze(); } dc.DrawLine(pen, new Point(_renderBounds.Left, _renderBounds.Bottom - pen.Thickness / 2), new Point(_renderBounds.Right, _renderBounds.Bottom - pen.Thickness / 2)); } } } // Draw background in rectangle inside border. if (_backgroundBrush != null) { dc.DrawRectangle(_backgroundBrush, null, new Rect( new Point(_renderBounds.Left + _borderThickness.Left, _renderBounds.Top + _borderThickness.Top), new Point(_renderBounds.Right - _borderThickness.Right, _renderBounds.Bottom - _borderThickness.Bottom))); } } private Brush _backgroundBrush; // Background brush private Brush _borderBrush; // Border brush private Thickness _borderThickness; // Border thickness private Rect _renderBounds; // Render bounds of the visual } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
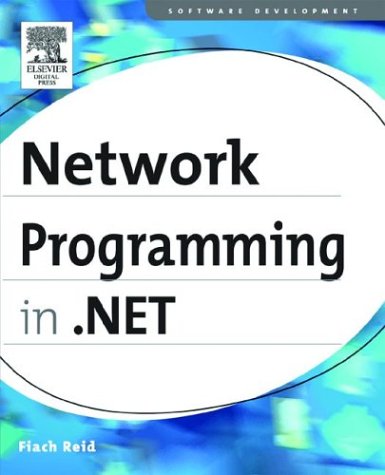
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- validationstate.cs
- SendSecurityHeaderElement.cs
- OleDbConnection.cs
- GroupQuery.cs
- precedingquery.cs
- EnumDataContract.cs
- ComboBox.cs
- RPIdentityRequirement.cs
- FixedSOMLineRanges.cs
- VersionConverter.cs
- COM2ComponentEditor.cs
- coordinatorscratchpad.cs
- ColorAnimationBase.cs
- LayoutEditorPart.cs
- Stylesheet.cs
- LoginDesigner.cs
- MultiSelector.cs
- HostProtectionException.cs
- StylusTip.cs
- PropertyPathConverter.cs
- ActivityDesignerHelper.cs
- QilPatternFactory.cs
- XmlDataCollection.cs
- AsymmetricSignatureFormatter.cs
- X509Chain.cs
- BitmapImage.cs
- OleDbConnectionFactory.cs
- SqlColumnizer.cs
- ImageMapEventArgs.cs
- WSSecurityPolicy12.cs
- GlyphRun.cs
- X509SecurityToken.cs
- TextLineBreak.cs
- BuildProvider.cs
- Clock.cs
- UriParserTemplates.cs
- Int32RectConverter.cs
- DataServiceResponse.cs
- ValidatingCollection.cs
- Attributes.cs
- ScaleTransform3D.cs
- InvariantComparer.cs
- ResourceWriter.cs
- InvokeHandlers.cs
- SqlMetaData.cs
- AppDomainInfo.cs
- XsltQilFactory.cs
- TraceSection.cs
- Int32AnimationBase.cs
- AlphabeticalEnumConverter.cs
- SQLSingleStorage.cs
- SettingsAttributeDictionary.cs
- RemotingException.cs
- SineEase.cs
- GeometryModel3D.cs
- RangeValidator.cs
- PasswordBoxAutomationPeer.cs
- Calendar.cs
- TCEAdapterGenerator.cs
- BlurBitmapEffect.cs
- KeyedPriorityQueue.cs
- CharacterMetrics.cs
- MemberBinding.cs
- StreamWithDictionary.cs
- _SslSessionsCache.cs
- _HeaderInfo.cs
- XmlSchemaAny.cs
- UICuesEvent.cs
- SymbolMethod.cs
- DependencyPropertyDescriptor.cs
- BinaryQueryOperator.cs
- BitmapFrameDecode.cs
- Padding.cs
- DbParameterCollectionHelper.cs
- OdbcStatementHandle.cs
- EventDescriptor.cs
- ResourceIDHelper.cs
- CodeDOMUtility.cs
- SequentialOutput.cs
- CheckBoxDesigner.cs
- Font.cs
- DataGridViewAutoSizeModeEventArgs.cs
- BuildProvidersCompiler.cs
- VSDExceptions.cs
- ZoneIdentityPermission.cs
- PermissionAttributes.cs
- HMAC.cs
- EndCreateSecurityTokenRequest.cs
- SmiEventSink_DeferedProcessing.cs
- GeometryDrawing.cs
- CryptoKeySecurity.cs
- SqlGatherProducedAliases.cs
- ShutDownListener.cs
- DatatypeImplementation.cs
- TitleStyle.cs
- PolicyFactory.cs
- AsyncOperationManager.cs
- SqlDataSourceParameterParser.cs
- SHA1CryptoServiceProvider.cs
- GZipUtils.cs