Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Collections / Generic / DebugView.cs / 1 / DebugView.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Collections.ObjectModel; using System.Security.Permissions; using System.Diagnostics; // // VS IDE can't differentiate between types with the same name from different // assembly. So we need to use different names for collection debug view for // collections in mscorlib.dll and system.dll. // internal sealed class Mscorlib_CollectionDebugView{ private ICollection collection; public Mscorlib_CollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryKeyCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryValueCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryDebugView { private IDictionary dict; public Mscorlib_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.dictionary); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_KeyedCollectionDebugView { private KeyedCollection kc; public Mscorlib_KeyedCollectionDebugView(KeyedCollection keyedCollection) { if (keyedCollection == null) { throw new ArgumentNullException("keyedCollection"); } kc = keyedCollection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[kc.Count]; kc.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Collections.ObjectModel; using System.Security.Permissions; using System.Diagnostics; // // VS IDE can't differentiate between types with the same name from different // assembly. So we need to use different names for collection debug view for // collections in mscorlib.dll and system.dll. // internal sealed class Mscorlib_CollectionDebugView { private ICollection collection; public Mscorlib_CollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryKeyCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryValueCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryDebugView { private IDictionary dict; public Mscorlib_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.dictionary); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_KeyedCollectionDebugView { private KeyedCollection kc; public Mscorlib_KeyedCollectionDebugView(KeyedCollection keyedCollection) { if (keyedCollection == null) { throw new ArgumentNullException("keyedCollection"); } kc = keyedCollection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[kc.Count]; kc.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
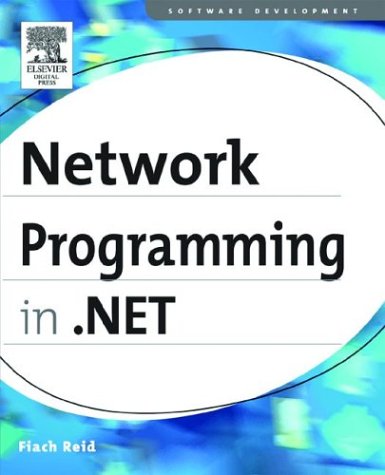
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReadingWritingEntityEventArgs.cs
- SQLByte.cs
- DataShape.cs
- EntityDataSourceState.cs
- AuthorizationSection.cs
- ChannelTerminatedException.cs
- WebBaseEventKeyComparer.cs
- DebugView.cs
- RSAPKCS1SignatureFormatter.cs
- DateTimeOffsetConverter.cs
- Gdiplus.cs
- TextFindEngine.cs
- ContextDataSourceView.cs
- KeyPullup.cs
- SystemFonts.cs
- EntityViewGenerator.cs
- MdiWindowListItemConverter.cs
- QueueProcessor.cs
- UTF8Encoding.cs
- XmlSchemaAttributeGroupRef.cs
- CodeCompiler.cs
- DataGridDesigner.cs
- CorrelationHandle.cs
- ButtonChrome.cs
- GacUtil.cs
- LinqDataSourceView.cs
- RotateTransform.cs
- ConfigXmlText.cs
- objectquery_tresulttype.cs
- SiteMapNodeCollection.cs
- _AcceptOverlappedAsyncResult.cs
- IPEndPointCollection.cs
- Set.cs
- WebAdminConfigurationHelper.cs
- COM2IProvidePropertyBuilderHandler.cs
- DropSource.cs
- DataGridViewRowsRemovedEventArgs.cs
- SafeMILHandle.cs
- _DomainName.cs
- XmlToDatasetMap.cs
- xsdvalidator.cs
- OdbcParameter.cs
- MethodBody.cs
- Oid.cs
- SoapClientProtocol.cs
- DataGridViewCellFormattingEventArgs.cs
- GridViewEditEventArgs.cs
- TemplateControlParser.cs
- TextServicesCompartmentEventSink.cs
- SetterBaseCollection.cs
- WebPartCollection.cs
- PassportIdentity.cs
- ConnectionProviderAttribute.cs
- Link.cs
- ByteStack.cs
- HttpCachePolicy.cs
- AccessedThroughPropertyAttribute.cs
- FontFamilyValueSerializer.cs
- Imaging.cs
- GridViewSelectEventArgs.cs
- Rotation3DAnimationBase.cs
- PackageDigitalSignatureManager.cs
- ComplexLine.cs
- Matrix3D.cs
- TextHidden.cs
- VisualStyleRenderer.cs
- securitycriticaldataClass.cs
- DesignTimeParseData.cs
- TypeValidationEventArgs.cs
- D3DImage.cs
- ManifestResourceInfo.cs
- PasswordBox.cs
- StreamInfo.cs
- MouseBinding.cs
- DSASignatureFormatter.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- XmlAttribute.cs
- DBCSCodePageEncoding.cs
- BuildProviderAppliesToAttribute.cs
- Menu.cs
- CrossSiteScriptingValidation.cs
- LineMetrics.cs
- GridViewRowPresenterBase.cs
- HtmlElementEventArgs.cs
- TextContainerChangedEventArgs.cs
- TableLayoutSettings.cs
- ItemsControl.cs
- Pens.cs
- SqlReorderer.cs
- InvocationExpression.cs
- Matrix3DStack.cs
- RefreshPropertiesAttribute.cs
- StorageSetMapping.cs
- FontFamilyConverter.cs
- MissingMethodException.cs
- ProgressBarAutomationPeer.cs
- EmptyEnumerable.cs
- SqlRowUpdatedEvent.cs
- HuffmanTree.cs
- HttpValueCollection.cs