Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Command / NavigationCommands.cs / 1 / NavigationCommands.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The NavigationCommands class defines a standard set of commands that act on Content Navigation // // See spec at : http://avalon/CoreUI/Specs%20%20Eventing%20and%20Commanding/CommandLibrarySpec.mht // // // History: // 03/31/2004 : chandras - Created // 04/28/2004 : Added Accelerator table loading from Resource // 02/02/2005 : Created NavigationCommands class from CommandLibrary class. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Collections; using System.ComponentModel; using System.Security; using System.Security.Permissions; using MS.Internal; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// NavigationCommands - Set of Standard Commands /// public static class NavigationCommands { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// BrowserBack Command /// public static RoutedUICommand BrowseBack { get { return _EnsureCommand(CommandId.BrowseBack); } } ////// BrowserForward Command /// public static RoutedUICommand BrowseForward { get { return _EnsureCommand(CommandId.BrowseForward); } } ////// BrowseHome Command /// public static RoutedUICommand BrowseHome { get { return _EnsureCommand(CommandId.BrowseHome); } } ////// BrowseStop Command /// public static RoutedUICommand BrowseStop { get { return _EnsureCommand(CommandId.BrowseStop); } } ////// Refresh Command /// public static RoutedUICommand Refresh { get { return _EnsureCommand(CommandId.Refresh); } } ////// Favorites Command /// public static RoutedUICommand Favorites { get { return _EnsureCommand(CommandId.Favorites); } } ////// Search Command /// public static RoutedUICommand Search { get { return _EnsureCommand(CommandId.Search); } } ////// IncreaseZoom Command /// public static RoutedUICommand IncreaseZoom { get { return _EnsureCommand(CommandId.IncreaseZoom); } } ////// DecreaseZoom Command /// public static RoutedUICommand DecreaseZoom { get { return _EnsureCommand(CommandId.DecreaseZoom); } } ////// Zoom Command /// public static RoutedUICommand Zoom { get { return _EnsureCommand(CommandId.Zoom); } } ////// NextPage Command /// public static RoutedUICommand NextPage { get {return _EnsureCommand(CommandId.NextPage);} } ////// PreviousPage Command /// public static RoutedUICommand PreviousPage { get {return _EnsureCommand(CommandId.PreviousPage);} } ////// FirstPage Command /// public static RoutedUICommand FirstPage { get {return _EnsureCommand(CommandId.FirstPage);} } ////// LastPage Command /// public static RoutedUICommand LastPage { get {return _EnsureCommand(CommandId.LastPage);} } ////// GoToPage Command /// public static RoutedUICommand GoToPage { get {return _EnsureCommand(CommandId.GoToPage);} } ////// NavigateJournal command. /// public static RoutedUICommand NavigateJournal { get { return _EnsureCommand(CommandId.NavigateJournal); } } #endregion Public Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static string GetPropertyName(CommandId commandId) { string propertyName = String.Empty; switch (commandId) { case CommandId.BrowseBack: propertyName = "BrowseBack"; break; case CommandId.BrowseForward: propertyName = "BrowseForward"; break; case CommandId.BrowseHome: propertyName = "BrowseHome"; break; case CommandId.BrowseStop: propertyName = "BrowseStop"; break; case CommandId.Refresh: propertyName = "Refresh"; break; case CommandId.Favorites: propertyName = "Favorites"; break; case CommandId.Search: propertyName = "Search"; break; case CommandId.IncreaseZoom: propertyName = "IncreaseZoom"; break; case CommandId.DecreaseZoom: propertyName = "DecreaseZoom"; break; case CommandId.Zoom: propertyName = "Zoom"; break; case CommandId.NextPage: propertyName = "NextPage"; break; case CommandId.PreviousPage: propertyName = "PreviousPage"; break; case CommandId.FirstPage: propertyName = "FirstPage"; break; case CommandId.LastPage: propertyName = "LastPage"; break; case CommandId.GoToPage: propertyName = "GoToPage"; break; case CommandId.NavigateJournal: propertyName = "NavigateJournal"; break; } return propertyName; } internal static string GetUIText(byte commandId) { string uiText = String.Empty; switch ((CommandId)commandId) { case CommandId.BrowseBack: uiText = SR.Get(SRID.BrowseBackText); break; case CommandId.BrowseForward: uiText = SR.Get(SRID.BrowseForwardText); break; case CommandId.BrowseHome: uiText = SR.Get(SRID.BrowseHomeText); break; case CommandId.BrowseStop: uiText = SR.Get(SRID.BrowseStopText); break; case CommandId.Refresh: uiText = SR.Get(SRID.RefreshText); break; case CommandId.Favorites: uiText = SR.Get(SRID.FavoritesText); break; case CommandId.Search: uiText = SR.Get(SRID.SearchText); break; case CommandId.IncreaseZoom: uiText = SR.Get(SRID.IncreaseZoomText); break; case CommandId.DecreaseZoom: uiText = SR.Get(SRID.DecreaseZoomText); break; case CommandId.Zoom: uiText = SR.Get(SRID.ZoomText); break; case CommandId.NextPage: uiText = SR.Get(SRID.NextPageText); break; case CommandId.PreviousPage: uiText = SR.Get(SRID.PreviousPageText); break; case CommandId.FirstPage: uiText = SR.Get(SRID.FirstPageText); break; case CommandId.LastPage: uiText = SR.Get(SRID.LastPageText); break; case CommandId.GoToPage: uiText = SR.Get(SRID.GoToPageText); break; case CommandId.NavigateJournal: uiText = SR.Get(SRID.NavigateJournalText); break; } return uiText; } internal static InputGestureCollection LoadDefaultGestureFromResource(byte commandId) { InputGestureCollection gestures = new InputGestureCollection(); //Standard Commands switch ((CommandId)commandId) { case CommandId.BrowseBack: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseBackKey), SR.Get(SRID.BrowseBackKeyDisplayString), gestures); break; case CommandId.BrowseForward: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseForwardKey), SR.Get(SRID.BrowseForwardKeyDisplayString), gestures); break; case CommandId.BrowseHome: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseHomeKey), SR.Get(SRID.BrowseHomeKeyDisplayString), gestures); break; case CommandId.BrowseStop: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseStopKey), SR.Get(SRID.BrowseStopKeyDisplayString), gestures); break; case CommandId.Refresh: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.RefreshKey), SR.Get(SRID.RefreshKeyDisplayString), gestures); break; case CommandId.Favorites: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.FavoritesKey), SR.Get(SRID.FavoritesKeyDisplayString), gestures); break; case CommandId.Search: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.SearchKey), SR.Get(SRID.SearchKeyDisplayString), gestures); break; case CommandId.IncreaseZoom: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.IncreaseZoomKey), SR.Get(SRID.IncreaseZoomKeyDisplayString), gestures); break; case CommandId.DecreaseZoom: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.DecreaseZoomKey), SR.Get(SRID.DecreaseZoomKeyDisplayString), gestures); break; case CommandId.Zoom: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.ZoomKey), SR.Get(SRID.ZoomKeyDisplayString), gestures); break; case CommandId.NextPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.NextPageKey), SR.Get(SRID.NextPageKeyDisplayString), gestures); break; case CommandId.PreviousPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.PreviousPageKey), SR.Get(SRID.PreviousPageKeyDisplayString), gestures); break; case CommandId.FirstPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.FirstPageKey), SR.Get(SRID.FirstPageKeyDisplayString), gestures); break; case CommandId.LastPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.LastPageKey), SR.Get(SRID.LastPageKeyDisplayString), gestures); break; case CommandId.GoToPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.GoToPageKey), SR.Get(SRID.GoToPageKeyDisplayString), gestures); break; case CommandId.NavigateJournal: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.NavigateJournalKey), SR.Get(SRID.NavigateJournalKeyDisplayString), gestures); break; } return gestures; } private static RoutedUICommand _EnsureCommand(CommandId idCommand) { if (idCommand >= 0 && idCommand < CommandId.Last) { lock (_internalCommands.SyncRoot) { if (_internalCommands[(int)idCommand] == null) { RoutedUICommand newCommand = CommandLibraryHelper.CreateUICommand( GetPropertyName(idCommand), typeof(NavigationCommands), (byte)idCommand, null); _internalCommands[(int)idCommand] = newCommand; } } return _internalCommands[(int)idCommand]; } return null; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // these constants will go away in future, its just to index into the right one. private enum CommandId : byte { // Formatting BrowseBack = 1, BrowseForward = 2, BrowseHome = 3, BrowseStop = 4, Refresh = 5, Favorites = 6, Search = 7, IncreaseZoom = 8, DecreaseZoom = 9, Zoom = 10, NextPage = 11, PreviousPage = 12, FirstPage = 13, LastPage = 14, GoToPage = 15, NavigateJournal = 16, // Last Last = 17 } private static RoutedUICommand[] _internalCommands = new RoutedUICommand[(int)CommandId.Last]; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The NavigationCommands class defines a standard set of commands that act on Content Navigation // // See spec at : http://avalon/CoreUI/Specs%20%20Eventing%20and%20Commanding/CommandLibrarySpec.mht // // // History: // 03/31/2004 : chandras - Created // 04/28/2004 : Added Accelerator table loading from Resource // 02/02/2005 : Created NavigationCommands class from CommandLibrary class. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Collections; using System.ComponentModel; using System.Security; using System.Security.Permissions; using MS.Internal; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// NavigationCommands - Set of Standard Commands /// public static class NavigationCommands { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// BrowserBack Command /// public static RoutedUICommand BrowseBack { get { return _EnsureCommand(CommandId.BrowseBack); } } ////// BrowserForward Command /// public static RoutedUICommand BrowseForward { get { return _EnsureCommand(CommandId.BrowseForward); } } ////// BrowseHome Command /// public static RoutedUICommand BrowseHome { get { return _EnsureCommand(CommandId.BrowseHome); } } ////// BrowseStop Command /// public static RoutedUICommand BrowseStop { get { return _EnsureCommand(CommandId.BrowseStop); } } ////// Refresh Command /// public static RoutedUICommand Refresh { get { return _EnsureCommand(CommandId.Refresh); } } ////// Favorites Command /// public static RoutedUICommand Favorites { get { return _EnsureCommand(CommandId.Favorites); } } ////// Search Command /// public static RoutedUICommand Search { get { return _EnsureCommand(CommandId.Search); } } ////// IncreaseZoom Command /// public static RoutedUICommand IncreaseZoom { get { return _EnsureCommand(CommandId.IncreaseZoom); } } ////// DecreaseZoom Command /// public static RoutedUICommand DecreaseZoom { get { return _EnsureCommand(CommandId.DecreaseZoom); } } ////// Zoom Command /// public static RoutedUICommand Zoom { get { return _EnsureCommand(CommandId.Zoom); } } ////// NextPage Command /// public static RoutedUICommand NextPage { get {return _EnsureCommand(CommandId.NextPage);} } ////// PreviousPage Command /// public static RoutedUICommand PreviousPage { get {return _EnsureCommand(CommandId.PreviousPage);} } ////// FirstPage Command /// public static RoutedUICommand FirstPage { get {return _EnsureCommand(CommandId.FirstPage);} } ////// LastPage Command /// public static RoutedUICommand LastPage { get {return _EnsureCommand(CommandId.LastPage);} } ////// GoToPage Command /// public static RoutedUICommand GoToPage { get {return _EnsureCommand(CommandId.GoToPage);} } ////// NavigateJournal command. /// public static RoutedUICommand NavigateJournal { get { return _EnsureCommand(CommandId.NavigateJournal); } } #endregion Public Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static string GetPropertyName(CommandId commandId) { string propertyName = String.Empty; switch (commandId) { case CommandId.BrowseBack: propertyName = "BrowseBack"; break; case CommandId.BrowseForward: propertyName = "BrowseForward"; break; case CommandId.BrowseHome: propertyName = "BrowseHome"; break; case CommandId.BrowseStop: propertyName = "BrowseStop"; break; case CommandId.Refresh: propertyName = "Refresh"; break; case CommandId.Favorites: propertyName = "Favorites"; break; case CommandId.Search: propertyName = "Search"; break; case CommandId.IncreaseZoom: propertyName = "IncreaseZoom"; break; case CommandId.DecreaseZoom: propertyName = "DecreaseZoom"; break; case CommandId.Zoom: propertyName = "Zoom"; break; case CommandId.NextPage: propertyName = "NextPage"; break; case CommandId.PreviousPage: propertyName = "PreviousPage"; break; case CommandId.FirstPage: propertyName = "FirstPage"; break; case CommandId.LastPage: propertyName = "LastPage"; break; case CommandId.GoToPage: propertyName = "GoToPage"; break; case CommandId.NavigateJournal: propertyName = "NavigateJournal"; break; } return propertyName; } internal static string GetUIText(byte commandId) { string uiText = String.Empty; switch ((CommandId)commandId) { case CommandId.BrowseBack: uiText = SR.Get(SRID.BrowseBackText); break; case CommandId.BrowseForward: uiText = SR.Get(SRID.BrowseForwardText); break; case CommandId.BrowseHome: uiText = SR.Get(SRID.BrowseHomeText); break; case CommandId.BrowseStop: uiText = SR.Get(SRID.BrowseStopText); break; case CommandId.Refresh: uiText = SR.Get(SRID.RefreshText); break; case CommandId.Favorites: uiText = SR.Get(SRID.FavoritesText); break; case CommandId.Search: uiText = SR.Get(SRID.SearchText); break; case CommandId.IncreaseZoom: uiText = SR.Get(SRID.IncreaseZoomText); break; case CommandId.DecreaseZoom: uiText = SR.Get(SRID.DecreaseZoomText); break; case CommandId.Zoom: uiText = SR.Get(SRID.ZoomText); break; case CommandId.NextPage: uiText = SR.Get(SRID.NextPageText); break; case CommandId.PreviousPage: uiText = SR.Get(SRID.PreviousPageText); break; case CommandId.FirstPage: uiText = SR.Get(SRID.FirstPageText); break; case CommandId.LastPage: uiText = SR.Get(SRID.LastPageText); break; case CommandId.GoToPage: uiText = SR.Get(SRID.GoToPageText); break; case CommandId.NavigateJournal: uiText = SR.Get(SRID.NavigateJournalText); break; } return uiText; } internal static InputGestureCollection LoadDefaultGestureFromResource(byte commandId) { InputGestureCollection gestures = new InputGestureCollection(); //Standard Commands switch ((CommandId)commandId) { case CommandId.BrowseBack: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseBackKey), SR.Get(SRID.BrowseBackKeyDisplayString), gestures); break; case CommandId.BrowseForward: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseForwardKey), SR.Get(SRID.BrowseForwardKeyDisplayString), gestures); break; case CommandId.BrowseHome: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseHomeKey), SR.Get(SRID.BrowseHomeKeyDisplayString), gestures); break; case CommandId.BrowseStop: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.BrowseStopKey), SR.Get(SRID.BrowseStopKeyDisplayString), gestures); break; case CommandId.Refresh: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.RefreshKey), SR.Get(SRID.RefreshKeyDisplayString), gestures); break; case CommandId.Favorites: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.FavoritesKey), SR.Get(SRID.FavoritesKeyDisplayString), gestures); break; case CommandId.Search: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.SearchKey), SR.Get(SRID.SearchKeyDisplayString), gestures); break; case CommandId.IncreaseZoom: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.IncreaseZoomKey), SR.Get(SRID.IncreaseZoomKeyDisplayString), gestures); break; case CommandId.DecreaseZoom: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.DecreaseZoomKey), SR.Get(SRID.DecreaseZoomKeyDisplayString), gestures); break; case CommandId.Zoom: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.ZoomKey), SR.Get(SRID.ZoomKeyDisplayString), gestures); break; case CommandId.NextPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.NextPageKey), SR.Get(SRID.NextPageKeyDisplayString), gestures); break; case CommandId.PreviousPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.PreviousPageKey), SR.Get(SRID.PreviousPageKeyDisplayString), gestures); break; case CommandId.FirstPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.FirstPageKey), SR.Get(SRID.FirstPageKeyDisplayString), gestures); break; case CommandId.LastPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.LastPageKey), SR.Get(SRID.LastPageKeyDisplayString), gestures); break; case CommandId.GoToPage: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.GoToPageKey), SR.Get(SRID.GoToPageKeyDisplayString), gestures); break; case CommandId.NavigateJournal: KeyGesture.AddGesturesFromResourceStrings( SR.Get(SRID.NavigateJournalKey), SR.Get(SRID.NavigateJournalKeyDisplayString), gestures); break; } return gestures; } private static RoutedUICommand _EnsureCommand(CommandId idCommand) { if (idCommand >= 0 && idCommand < CommandId.Last) { lock (_internalCommands.SyncRoot) { if (_internalCommands[(int)idCommand] == null) { RoutedUICommand newCommand = CommandLibraryHelper.CreateUICommand( GetPropertyName(idCommand), typeof(NavigationCommands), (byte)idCommand, null); _internalCommands[(int)idCommand] = newCommand; } } return _internalCommands[(int)idCommand]; } return null; } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields // these constants will go away in future, its just to index into the right one. private enum CommandId : byte { // Formatting BrowseBack = 1, BrowseForward = 2, BrowseHome = 3, BrowseStop = 4, Refresh = 5, Favorites = 6, Search = 7, IncreaseZoom = 8, DecreaseZoom = 9, Zoom = 10, NextPage = 11, PreviousPage = 12, FirstPage = 13, LastPage = 14, GoToPage = 15, NavigateJournal = 16, // Last Last = 17 } private static RoutedUICommand[] _internalCommands = new RoutedUICommand[(int)CommandId.Last]; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
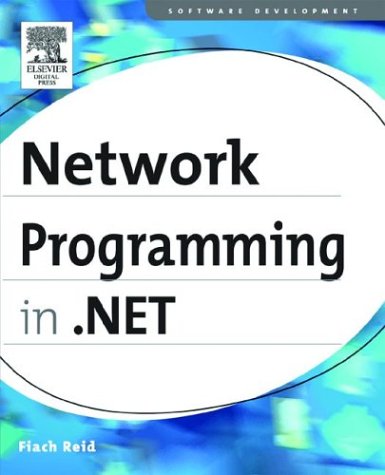
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DictionaryKeyPropertyAttribute.cs
- Transform.cs
- MembershipSection.cs
- ServiceThrottlingElement.cs
- httpserverutility.cs
- OpCodes.cs
- GenericParameterDataContract.cs
- TextViewDesigner.cs
- ClientSideQueueItem.cs
- DESCryptoServiceProvider.cs
- Transform3DCollection.cs
- DataObjectMethodAttribute.cs
- BitmapEditor.cs
- JsonWriterDelegator.cs
- SchemaElement.cs
- HttpException.cs
- WindowProviderWrapper.cs
- DataControlReferenceCollection.cs
- AtomEntry.cs
- RepeaterItemEventArgs.cs
- KnownColorTable.cs
- StandardOleMarshalObject.cs
- SecUtil.cs
- XmlSchemaChoice.cs
- dbenumerator.cs
- HtmlHistory.cs
- MemberMaps.cs
- Assert.cs
- ping.cs
- CodeDefaultValueExpression.cs
- Button.cs
- CodePropertyReferenceExpression.cs
- SystemEvents.cs
- WizardStepBase.cs
- IisTraceListener.cs
- TextTrailingWordEllipsis.cs
- OuterGlowBitmapEffect.cs
- EmissiveMaterial.cs
- IdentityManager.cs
- DecoratedNameAttribute.cs
- Menu.cs
- CodeStatement.cs
- oledbmetadatacollectionnames.cs
- SafeNativeMethods.cs
- UnsafeNetInfoNativeMethods.cs
- MatrixCamera.cs
- CryptoHandle.cs
- DataAdapter.cs
- ToolbarAUtomationPeer.cs
- unsafeIndexingFilterStream.cs
- WeakRefEnumerator.cs
- TripleDESCryptoServiceProvider.cs
- Comparer.cs
- NegatedCellConstant.cs
- VisualCollection.cs
- CodeAttributeDeclaration.cs
- GridViewCommandEventArgs.cs
- Selector.cs
- SpinWait.cs
- ExpressionBuilderContext.cs
- AxisAngleRotation3D.cs
- ArcSegment.cs
- CrossAppDomainChannel.cs
- RectangleHotSpot.cs
- ServiceRoute.cs
- ListDataHelper.cs
- XmlTextWriter.cs
- odbcmetadatacolumnnames.cs
- GetIndexBinder.cs
- XmlElementAttributes.cs
- NetworkInformationPermission.cs
- HandlerBase.cs
- ProxyWebPart.cs
- RetrieveVirtualItemEventArgs.cs
- TileModeValidation.cs
- Section.cs
- TextTreeInsertUndoUnit.cs
- DocumentApplicationDocumentViewer.cs
- InputReportEventArgs.cs
- GeneralTransformCollection.cs
- UiaCoreProviderApi.cs
- EventLogPermission.cs
- CacheHelper.cs
- MatrixAnimationUsingPath.cs
- TemplateBindingExtensionConverter.cs
- _SpnDictionary.cs
- BaseAddressElement.cs
- PrinterResolution.cs
- ContentType.cs
- NavigationWindowAutomationPeer.cs
- QuadraticBezierSegment.cs
- MediaPlayerState.cs
- OpacityConverter.cs
- HwndSourceParameters.cs
- ScrollBarAutomationPeer.cs
- HtmlControlPersistable.cs
- LogStore.cs
- InvokeHandlers.cs
- TextUtf8RawTextWriter.cs
- XmlNamedNodeMap.cs