Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Interaction / Model / ModelEditingScope.cs / 1305376 / ModelEditingScope.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Collections.Generic; using System.Globalization; using System.Activities.Presentation; using System.Diagnostics.CodeAnalysis; using System.Runtime; ////// A ModelEditingScope represents a group of changes to the /// editing store. Change groups are transactional: /// changes made under an editing scope can be committed /// or aborted as a unit. /// /// When an editing scope is committed, the editing store /// takes all changes that occurred within it and applies /// them to the model. If the editing scope’s Revert method /// is called, or the editing scope is disposed before Complete /// is called, the editing scope will instead reverse the /// changes made to the underlying objects, reapplying state /// from the editing store. This provides a solid basis for /// an undo mechanism. /// public abstract class ModelEditingScope : IDisposable { private string _description; private bool _completed; private bool _reverted; ////// Creates a new ModelEditingScope object. /// protected ModelEditingScope() { } ////// Describes the group. You may change this property /// anytime before the group is committed. /// public string Description { get { return (_description == null ? string.Empty : _description); } set { _description = value; } } ////// Completes the editing scope. If the editing scope has /// already been reverted or completed, this will throw an /// InvalidOperationException. Calling Complete calls the /// protected OnComplete method. /// ///If ModelEditingScope /// has already been complted or reverted. [SuppressMessage("Microsoft.Usage", "CA1816:CallGCSuppressFinalizeCorrectly", Justification = "By design.")] [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes", Justification = "By design.")] public void Complete() { if (_reverted) throw FxTrace.Exception.AsError(new InvalidOperationException(System.Activities.Presentation.Internal.Properties.Resources.Error_EditingScopeReverted)); if (_completed) throw FxTrace.Exception.AsError(new InvalidOperationException(System.Activities.Presentation.Internal.Properties.Resources.Error_EdtingScopeCompleted)); if (CanComplete()) { bool successful = false; _completed = true; // prevent recursive commits try { OnComplete(); successful = true; } catch (Exception e) { _completed = false; Revert(); if (Fx.IsFatal(e)) { throw; } if (!OnException(e)) { throw; } } finally { if (successful) { GC.SuppressFinalize(this); } else { _completed = false; } } } else Revert(); // We are not allowed to do completion, revert the change. } ////// Abandons the changes made during the editing scope. If the /// group has already been committed or aborted, this will /// throw an InvalidOperationException. Calling Revert calls /// the protected OnRevert with “false” for the /// finalizing parameter. /// ///If ModelEditingScope /// has already been committed. [SuppressMessage("Microsoft.Usage", "CA1816:CallGCSuppressFinalizeCorrectly", Justification = "By design.")] [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes", Justification = "By design.")] public void Revert() { if (_completed) throw FxTrace.Exception.AsError(new InvalidOperationException(System.Activities.Presentation.Internal.Properties.Resources.Error_EdtingScopeCompleted)); if (_reverted) return; bool successful = false; _reverted = true; try { OnRevert(false); successful = true; } catch(Exception e) { _reverted = false; if (Fx.IsFatal(e)) { throw; } if (!OnException(e)) { throw; } } finally { if (successful) { GC.SuppressFinalize(this); } else { _reverted = false; } } } ////// Implements IDisposable.Dispose as follows: /// /// 1. If the editing scope has already been completed or reverted, do nothing. /// 2. Revert the editing scope. /// /// GC.SuppressFinalize(this) is called through Revert(), so suppress the FxCop violation. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1816:DisposeMethodsShouldCallSuppressFinalize")] public void Dispose() { Dispose(true); } ////// Disposes this object by aborting changes. /// /// protected virtual void Dispose(bool disposing) { if (!_completed && !_reverted) { if (disposing) { Revert(); } else { OnRevert(true); } } } ////// Performs the actual complete of the editing scope. /// protected abstract void OnComplete(); ////// Determines if OnComplete should be called, or if the change should instead be reverted. Reasons /// for reverting may include file cannot be checked out of a source control system for modification. /// ///Returns true if completion can occur, false if the change should instead revert protected abstract bool CanComplete(); ////// Performs the actual revert of the editing scope. /// /// /// True if the abort is ocurring because the object /// is being finalized. Some undo systems may attempt to /// abort in this case, while others may abandon the /// change and record it as a reactive undo. /// protected abstract void OnRevert(bool finalizing); ////// Handle the exception during Complete and Revert. /// /// /// The exception to handle. /// ////// True if the exception is handled, false if otherwise /// protected abstract bool OnException(Exception exception); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Collections.Generic; using System.Globalization; using System.Activities.Presentation; using System.Diagnostics.CodeAnalysis; using System.Runtime; ////// A ModelEditingScope represents a group of changes to the /// editing store. Change groups are transactional: /// changes made under an editing scope can be committed /// or aborted as a unit. /// /// When an editing scope is committed, the editing store /// takes all changes that occurred within it and applies /// them to the model. If the editing scope’s Revert method /// is called, or the editing scope is disposed before Complete /// is called, the editing scope will instead reverse the /// changes made to the underlying objects, reapplying state /// from the editing store. This provides a solid basis for /// an undo mechanism. /// public abstract class ModelEditingScope : IDisposable { private string _description; private bool _completed; private bool _reverted; ////// Creates a new ModelEditingScope object. /// protected ModelEditingScope() { } ////// Describes the group. You may change this property /// anytime before the group is committed. /// public string Description { get { return (_description == null ? string.Empty : _description); } set { _description = value; } } ////// Completes the editing scope. If the editing scope has /// already been reverted or completed, this will throw an /// InvalidOperationException. Calling Complete calls the /// protected OnComplete method. /// ///If ModelEditingScope /// has already been complted or reverted. [SuppressMessage("Microsoft.Usage", "CA1816:CallGCSuppressFinalizeCorrectly", Justification = "By design.")] [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes", Justification = "By design.")] public void Complete() { if (_reverted) throw FxTrace.Exception.AsError(new InvalidOperationException(System.Activities.Presentation.Internal.Properties.Resources.Error_EditingScopeReverted)); if (_completed) throw FxTrace.Exception.AsError(new InvalidOperationException(System.Activities.Presentation.Internal.Properties.Resources.Error_EdtingScopeCompleted)); if (CanComplete()) { bool successful = false; _completed = true; // prevent recursive commits try { OnComplete(); successful = true; } catch (Exception e) { _completed = false; Revert(); if (Fx.IsFatal(e)) { throw; } if (!OnException(e)) { throw; } } finally { if (successful) { GC.SuppressFinalize(this); } else { _completed = false; } } } else Revert(); // We are not allowed to do completion, revert the change. } ////// Abandons the changes made during the editing scope. If the /// group has already been committed or aborted, this will /// throw an InvalidOperationException. Calling Revert calls /// the protected OnRevert with “false” for the /// finalizing parameter. /// ///If ModelEditingScope /// has already been committed. [SuppressMessage("Microsoft.Usage", "CA1816:CallGCSuppressFinalizeCorrectly", Justification = "By design.")] [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes", Justification = "By design.")] public void Revert() { if (_completed) throw FxTrace.Exception.AsError(new InvalidOperationException(System.Activities.Presentation.Internal.Properties.Resources.Error_EdtingScopeCompleted)); if (_reverted) return; bool successful = false; _reverted = true; try { OnRevert(false); successful = true; } catch(Exception e) { _reverted = false; if (Fx.IsFatal(e)) { throw; } if (!OnException(e)) { throw; } } finally { if (successful) { GC.SuppressFinalize(this); } else { _reverted = false; } } } ////// Implements IDisposable.Dispose as follows: /// /// 1. If the editing scope has already been completed or reverted, do nothing. /// 2. Revert the editing scope. /// /// GC.SuppressFinalize(this) is called through Revert(), so suppress the FxCop violation. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1816:DisposeMethodsShouldCallSuppressFinalize")] public void Dispose() { Dispose(true); } ////// Disposes this object by aborting changes. /// /// protected virtual void Dispose(bool disposing) { if (!_completed && !_reverted) { if (disposing) { Revert(); } else { OnRevert(true); } } } ////// Performs the actual complete of the editing scope. /// protected abstract void OnComplete(); ////// Determines if OnComplete should be called, or if the change should instead be reverted. Reasons /// for reverting may include file cannot be checked out of a source control system for modification. /// ///Returns true if completion can occur, false if the change should instead revert protected abstract bool CanComplete(); ////// Performs the actual revert of the editing scope. /// /// /// True if the abort is ocurring because the object /// is being finalized. Some undo systems may attempt to /// abort in this case, while others may abandon the /// change and record it as a reactive undo. /// protected abstract void OnRevert(bool finalizing); ////// Handle the exception during Complete and Revert. /// /// /// The exception to handle. /// ////// True if the exception is handled, false if otherwise /// protected abstract bool OnException(Exception exception); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
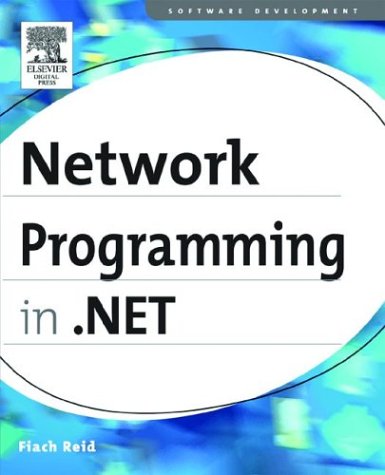
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TempFiles.cs
- DataGridViewControlCollection.cs
- BufferModesCollection.cs
- SqlDataReaderSmi.cs
- Helpers.cs
- SoapTransportImporter.cs
- _UriTypeConverter.cs
- FlowDocumentReaderAutomationPeer.cs
- ELinqQueryState.cs
- SafeProcessHandle.cs
- CqlParserHelpers.cs
- OleDbWrapper.cs
- Sql8ConformanceChecker.cs
- FixedSOMTableRow.cs
- ConstraintStruct.cs
- MediaSystem.cs
- TextAdaptor.cs
- BasicHttpBindingElement.cs
- SmiGettersStream.cs
- SqlProvider.cs
- BinaryConverter.cs
- GenericAuthenticationEventArgs.cs
- CompilationSection.cs
- PartitionResolver.cs
- UInt64.cs
- Int32EqualityComparer.cs
- ReachDocumentPageSerializerAsync.cs
- NumberSubstitution.cs
- Compilation.cs
- ExtendedPropertiesHandler.cs
- XmlHierarchicalDataSourceView.cs
- ToolStripManager.cs
- BigInt.cs
- XsdDateTime.cs
- ipaddressinformationcollection.cs
- RawAppCommandInputReport.cs
- SqlConnectionHelper.cs
- TextPattern.cs
- XsdBuildProvider.cs
- WorkflowViewService.cs
- NullRuntimeConfig.cs
- FileDialogCustomPlacesCollection.cs
- ListControlBuilder.cs
- CustomError.cs
- InvalidCommandTreeException.cs
- ClientSettingsSection.cs
- GenerateTemporaryTargetAssembly.cs
- RepeatInfo.cs
- SQLString.cs
- TableLayoutCellPaintEventArgs.cs
- TrustLevel.cs
- DoubleKeyFrameCollection.cs
- COM2Properties.cs
- UInt64Converter.cs
- LocatorBase.cs
- JsonSerializer.cs
- ScriptReference.cs
- Literal.cs
- CommandTreeTypeHelper.cs
- TemplateControl.cs
- DataList.cs
- Roles.cs
- ValidationSummary.cs
- WindowsTokenRoleProvider.cs
- MobileContainerDesigner.cs
- DefaultTextStoreTextComposition.cs
- BitmapMetadataEnumerator.cs
- HttpResponseInternalWrapper.cs
- ArrayMergeHelper.cs
- LambdaCompiler.Generated.cs
- KeyGesture.cs
- ProxyHwnd.cs
- Sql8ConformanceChecker.cs
- UrlAuthorizationModule.cs
- XmlSchemaCollection.cs
- FormCollection.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- CaseCqlBlock.cs
- RolePrincipal.cs
- DataGridViewColumnCollection.cs
- OutputCacheModule.cs
- SrgsNameValueTag.cs
- CompilationPass2TaskInternal.cs
- InfoCardTraceRecord.cs
- _HTTPDateParse.cs
- TouchEventArgs.cs
- WebPartEventArgs.cs
- XmlWrappingReader.cs
- ProtocolsConfigurationHandler.cs
- NetDataContractSerializer.cs
- System.Data_BID.cs
- RepeatInfo.cs
- ProcessThread.cs
- SelectedDatesCollection.cs
- ListViewHitTestInfo.cs
- SQLBoolean.cs
- SharedHttpsTransportManager.cs
- Evidence.cs
- GrammarBuilderDictation.cs
- FileRecordSequence.cs